Episode Two - Oops, I DDD It Again (Part 1) - A New Hope for Clean Architecture
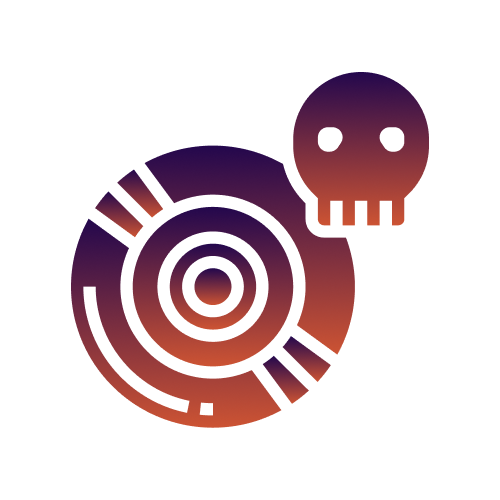
Table of contents
- A Quick Note from Your Friendly Neighborhood Rambler
- The Great GraphQL Detour
- DDD: The Plot Twist in Our Coding Saga
- From Monolith Maze to Green Field Dreams
- Setting the Stage: A Few Candid Notes
- Domain-Driven Design: Starting with the Basics
- What is a Domain?
- Subdomains: Breaking Down the Big Picture
- Diving into Domain-Driven Design: Key Concepts
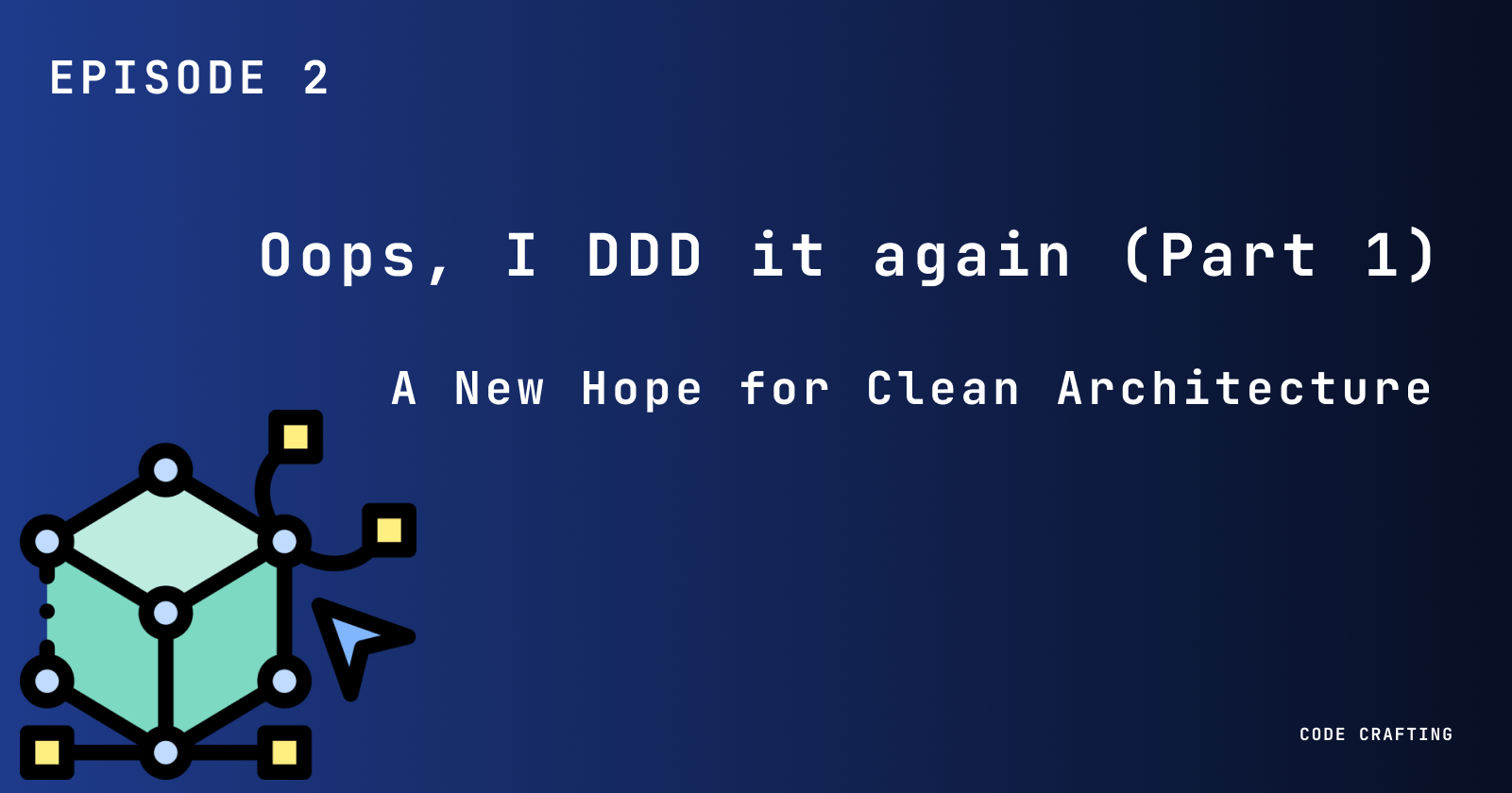
Hey there, fellow code wranglers! π Welcome back to The Kollabs Chronicle. Grab your favorite debugging beverage, because we're about to embark on an unexpected journey into the world of Domain-Driven Design (DDD)!
A Quick Note from Your Friendly Neighborhood Rambler
Before we dive in, I've got a confession to make. This post was originally a behemoth - we're talking "War and Peace" of coding blogs. But then I remembered: I'm a storyteller, not a technical manual writer. And let's face it, attention spans these days are shorter than a Vine video (RIP Vine, you were too pure for this world).
So, in the spirit of not boring you to tears, I've decided to break this DDD saga into a trilogy. Think "Lord of the Rings," but with more semicolons and fewer elves. Why? Because I believe in the power of storytelling, even when it comes to coding. I didn't want to just throw technical jargon at you like I'm a walking Stack Overflow. Nope, I wanted to take you on this journey with me, complete with all the twists, turns, and "how did I end up here?" moments.
Plus, let's be real - I love to ramble. It's like my superpower, but instead of fighting crime, I fight boredom with tangents and pop culture references. So buckle up, buttercup! We're about to take a wild ride through the land of DDD, with plenty of pit stops for humor, sarcasm, and the occasional gif that perfectly captures the mood of debugging at 2 AM.
Now, without further ado, let's dive into how I accidentally stumbled into the galaxy of Domain-Driven Design for this post!
The Great GraphQL Detour
Hereβs the truth: I sat down to write a tutorial about implementing a GraphQL API in a monolithic application. You know, the kind of tech wizardry that impresses your peers and makes your code reviews a bit more interesting.
But as I started setting up a simple playground project to showcase GraphQL, something clicked. It was one of those classic "aha!" moments. "What if," I thought, "we structure this using Domain-Driven Design principles from the start?"
And just like that, our GraphQL tutorial took a sharp left turn into DDD territory. Oops, I DDD it again! (Cue the Britney Spears soundtrack π΅)
DDD: The Plot Twist in Our Coding Saga
Now, you might be wondering, "Christina, why the sudden passion for DDD?" Well, let me break it down for you:
Real-world Complexity: Professional development often involves navigating domains more tangled than your earbuds after being in your pocket for 5 seconds (yeah, I just outed myself as a millennial there). DDD offers us a map and compass for these treacherous terrains.
Future-Proofing: By starting with DDD, we're not just coding; we're architecting for maintainability. It's like planting a tree - the best time was 20 years ago, the second best time is now.
Business-Code Synergy: DDD bridges the gap between business ideas and software design. It's our toolkit for building systems that truly reflect and support real-world operations.
Don't worry, GraphQL hasn't fallen off our radar. Think of this DDD exploration as laying the groundwork. By the time we circle back to GraphQL, our project will be so well-structured that implementing the API will feel like a breeze. (Okay, maybe a gentle wind. Let's not get too carried away.)
From Monolith Maze to Green Field Dreams
Here's where things get interesting. Up until now, I've been applying DDD principles in bits and pieces, shoehorning them into new features of the monolithic project I'm working on. Imagine trying to renovate a house while people are still living in it β you can make improvements, but you're always constrained by the existing structure.
But creating something from scratch using DDD? That's a whole different ball game. It's like being handed a blank canvas and a full set of paints after years of only being allowed to touch up existing artwork. This exercise isn't just about building a new project β it's about uncovering different layers of DDD that are hard to see when you're neck-deep in legacy code.
My hope? That this exploration will not only teach us about building with DDD from the ground up but also shine a light on new ways we could refactor and improve existing systems. Who knows? We might just discover some techniques to breathe new life into that monolith back at work. Think of it as mastering the art of cooking gourmet meals from scratch β suddenly, you see a hundred ways to spice up those leftovers in your fridge!
Setting the Stage: A Few Candid Notes
Before we dive in, let's set some ground rules:
Keeping It Simple: We're focusing on core DDD concepts. No tests or fancy tools in this post β we'll get there, but one step at a time!
Perfection? Not Our Goal: This code won't be production-ready, and that's perfectly fine. We're here to learn and explore.
Feedback is Gold: This is my first time fully applying DDD principles from scratch. Have ideas on how to do it better? Drop a comment. Let's make this a collaborative learning experience!
Domain-Driven Design: Starting with the Basics
Before we dive into the specifics of our Kollabs Book Network (aka our playground project), let's start with some fundamental concepts of Domain-Driven Design:
What is a Domain?
In software development, a "domain" refers to the subject area to which we apply our program. It's the sphere of knowledge and activity around which the application logic revolves.
In our case, the overall domain is book selling and management. This encompasses everything related to books, customers, orders, reviews, and the business of running a bookstore network.
Subdomains: Breaking Down the Big Picture
Large domains are often divided into smaller, more manageable subdomains. These are distinct areas of functionality within the main domain.
For the Kollabs Book Network (or as I like to call it, the Book Emporium in true Star Wars fashion), we have identified several subdomains:
Book Catalog: Managing the inventory of books (which is our focus for applying DDD in this series)
Order Management: Handling customer orders, payments, and shipping
User Management: Dealing with customer accounts, preferences, and authentication
Review System: Managing book reviews and ratings
Recommendation Engine: Suggesting books based on user behavior and preferences
By focusing on one subdomain at a time, we can manage complexity and create more focused, efficient systems. For now, we're concentrating on the Book Catalog subdomain, but it's important to keep the larger context in mind as we design our system.
Diving into Domain-Driven Design: Key Concepts
Let's break down some core DDD concepts and see how they apply to our Kollabs Book Network:
Bounded Context - The Bookshelf of Our Domain
Think of a Bounded Context as a bookshelf in our vast library of domain knowledge. It's where we group related concepts and models. For our Book Catalog, this shelf holds everything about managing books - their details and inventory.
Entities - The Main Characters of Our Story
Entities are the protagonists in our domain narrative. In our Book Catalog, a Book
is an entity. It has a unique identity (like an ISBN) that persists even if its details change. It's like how Harry Potter is still Harry Potter, whether he's in Year 1 or Year 7 at Hogwarts.
Value Objects - The Supporting Cast
Value Objects are the sidekicks that describe our entities. They don't have an identity of their own, but they're crucial to the story. In our catalog, Price
could be a value object. It doesn't matter which specific price object we use, as long as the value is correct.
Aggregates - Where the Plot Thickens
Aggregates are clusters of domain objects (entities and value objects) that can be treated as a single unit. An aggregate has a root entity and a boundary. In our Book Catalog, a BookInventoryItem
aggregate could bundle the Book
entity (with unchanging attributes like title and author) with state-dependent information such as current price and stock level. This structure manages the changing state of a book in our inventory over time, while keeping the core Book
entity stable.
Repositories - The Librarians
Repositories are our domain librarians. They know how to find and store our aggregates. A BookInventoryItemRepositoryInterface
is like a knowledgeable librarian who can fetch any book's inventory information we need, without us having to know exactly how it's stored or managed behind the scenes.
Domain Services - The Narrators
When an operation doesn't naturally fit within an entity or value object, we use a domain service. It's like the narrator in our story, describing actions that involve multiple characters. Our BookService
might handle complex operations like "find all books by this author in this price range".
This foundational information sets the stage for our DDD adventure. As we progress through this series, we'll build on these concepts to create a robust Book Catalog system. If you're eager to dive deeper into Domain-Driven Design before our next installment, here are some excellent resources that have been invaluable in shaping my understanding of DDD as a beginner:
Ozan Akman's series on Understanding Domain-Driven Design:
Naresh Bhatia's series on Domain-Driven Design
These resources offer valuable insights that complement our journey, and I hope you enjoy them as much as I did. In our next exciting episode, we'll take our first steps into a larger world as we start implementing these DDD concepts. Get ready to craft your first Entity and tame the wild Repository! May the Domain be with you.
Subscribe to my newsletter
Read articles from Christina Koleri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
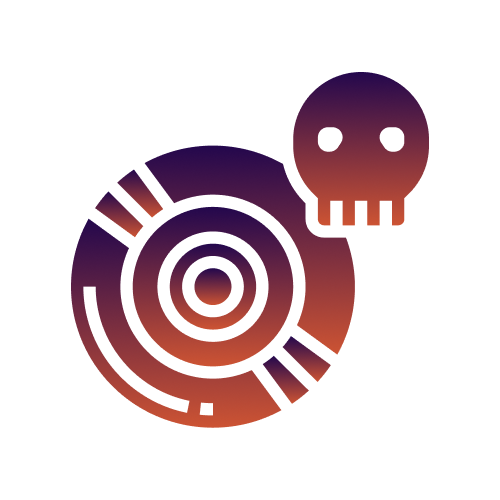