Rendering Patterns Demystified
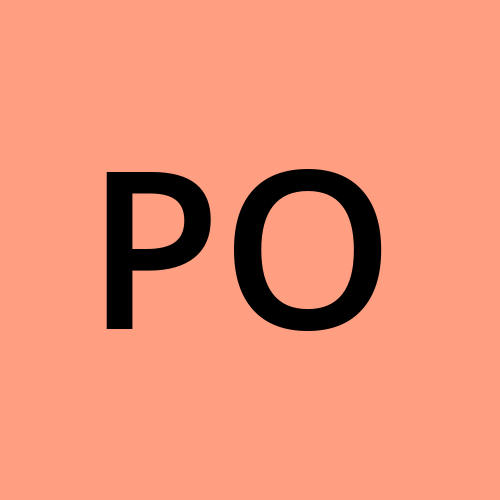
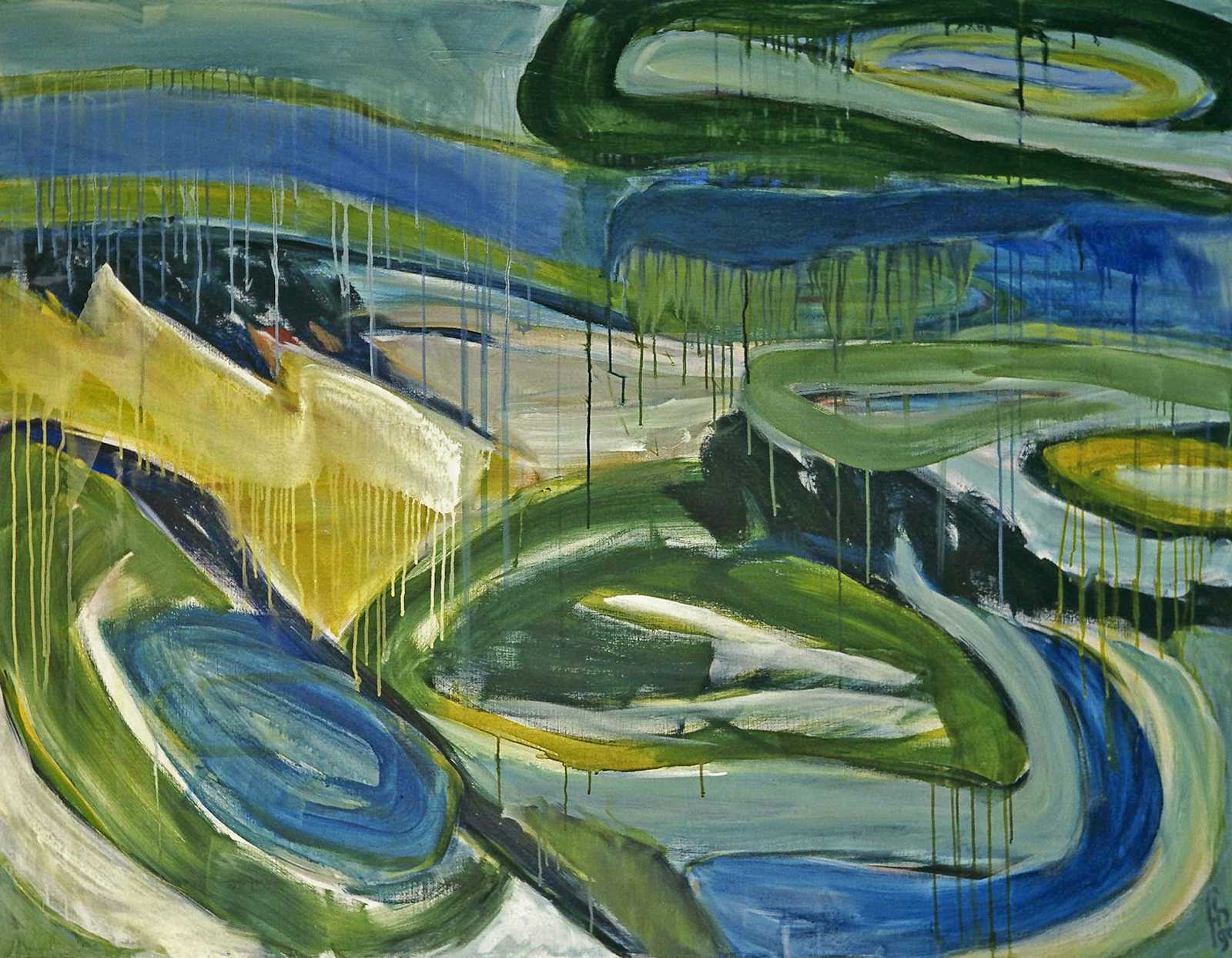
In web development, choosing the right rendering pattern is crucial for optimizing performance, user experience, and search engine optimization (SEO). This article will demystify common rendering patterns used in modern web applications, explaining their pros, cons, and use cases.
What are Rendering Patterns?
Rendering patterns determine how and where your web application's content is generated and served to the user. The choice of rendering pattern can significantly impact factors such as load time, interactivity, and SEO.
Client-Side Rendering (CSR)
Client-Side Rendering is a pattern where the browser downloads a minimal HTML page, along with JavaScript files. The JavaScript then renders the content in the browser.
Example of a CSR Application
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>CSR App</title>
</head>
<body>
<div id="root"></div>
<script src="app.js"></script>
</body>
</html>
// app.js
document.addEventListener('DOMContentLoaded', () => {
const root = document.getElementById('root');
root.innerHTML = '<h1>Hello, Client-Side Rendering!</h1>';
});
In this example, the initial HTML is minimal, and the content is rendered by JavaScript after the page loads.
Pros of CSR:
Rich interactions and dynamic updates
Reduced server load
Faster subsequent page loads
Cons of CSR:
Slower initial page load
Poor SEO if not implemented correctly
May not work well with JavaScript disabled
Server-Side Rendering (SSR)
Server-Side Rendering generates the full HTML for a page on the server in response to a user's request.
Example of SSR with Node.js and Express
const express = require('express');
const app = express();
app.get('/', (req, res) => {
const html = `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>SSR App</title>
</head>
<body>
<h1>Hello, Server-Side Rendering!</h1>
</body>
</html>
`;
res.send(html);
});
app.listen(3000, () => console.log('Server running on port 3000'));
This code generates and serves a complete HTML page from the server.
Pros of SSR:
Better initial page load performance
Improved SEO
Works without JavaScript
Cons of SSR:
Increased server load
Slower page transitions
More complex development process
Static Site Generation (SSG)
Static Site Generation pre-renders pages at build time, creating static HTML files that can be served quickly.
Example using Next.js
// pages/index.js
export default function Home({ posts }) {
return (
<div>
<h1>My Blog</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
}
export async function getStaticProps() {
const posts = await fetchBlogPosts();
return {
props: {
posts,
},
};
}
In this Next.js example, getStaticProps
fetches data at build time, which is then used to generate static HTML pages.
Pros of SSG:
Excellent performance
Good for SEO
Reduced server costs
Cons of SSG:
Not suitable for highly dynamic content
Requires rebuilding for content updates
Can be slow for large sites
Incremental Static Regeneration (ISR)
ISR is a hybrid approach that combines the benefits of static generation and server-side rendering. It allows you to update static content without rebuilding the entire site.
Example using Next.js
// pages/product/[id].js
export default function Product({ product }) {
return (
<div>
<h1>{product.name}</h1>
<p>Price: ${product.price}</p>
</div>
);
}
export async function getStaticProps({ params }) {
const product = await fetchProduct(params.id);
return {
props: {
product,
},
revalidate: 60, // Regenerate page every 60 seconds
};
}
export async function getStaticPaths() {
const products = await fetchProducts();
const paths = products.map((product) => ({
params: { id: product.id.toString() },
}));
return { paths, fallback: 'blocking' };
}
This Next.js code generates static pages for products but revalidates them every 60 seconds, allowing for updated content without a full rebuild.
Pros of ISR:
Combines benefits of static and dynamic rendering
Good for frequently updated content
Scalable for large sites
Cons of ISR:
More complex setup
Potential for stale data
Requires careful cache management
Conclusion
Choosing the right rendering pattern depends on your specific use case, considering factors such as content dynamism, SEO requirements, and performance needs. Often, modern web applications use a combination of these patterns to optimize different parts of the application.
Use CSR for highly interactive, dynamic applications where SEO is less critical.
Choose SSR for content-heavy sites that require good SEO and fast initial loads.
Opt for SSG for mostly static sites with infrequent updates.
Consider ISR for large sites with frequently updated content that still benefit from static generation.
Remember, these patterns are not mutually exclusive. Many modern frameworks, like Next.js, allow you to mix and match these rendering strategies within a single application, giving you the flexibility to optimize each page or component individually.
As web technologies continue to evolve, new rendering patterns may emerge. Stay informed about the latest developments to ensure you're always using the best approach for your projects.
Subscribe to my newsletter
Read articles from Precious Okeren directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
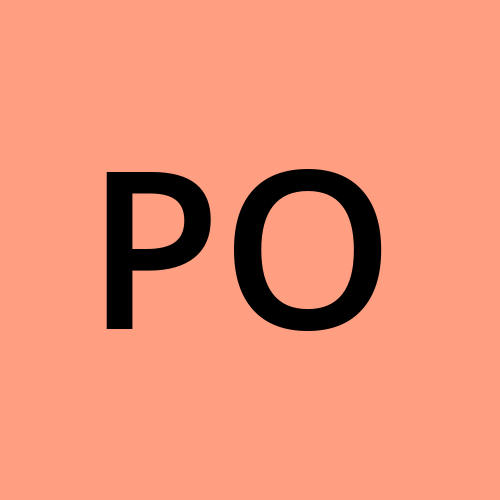