Transforming Infrared Images to Visible Light Using AWS and cGANs

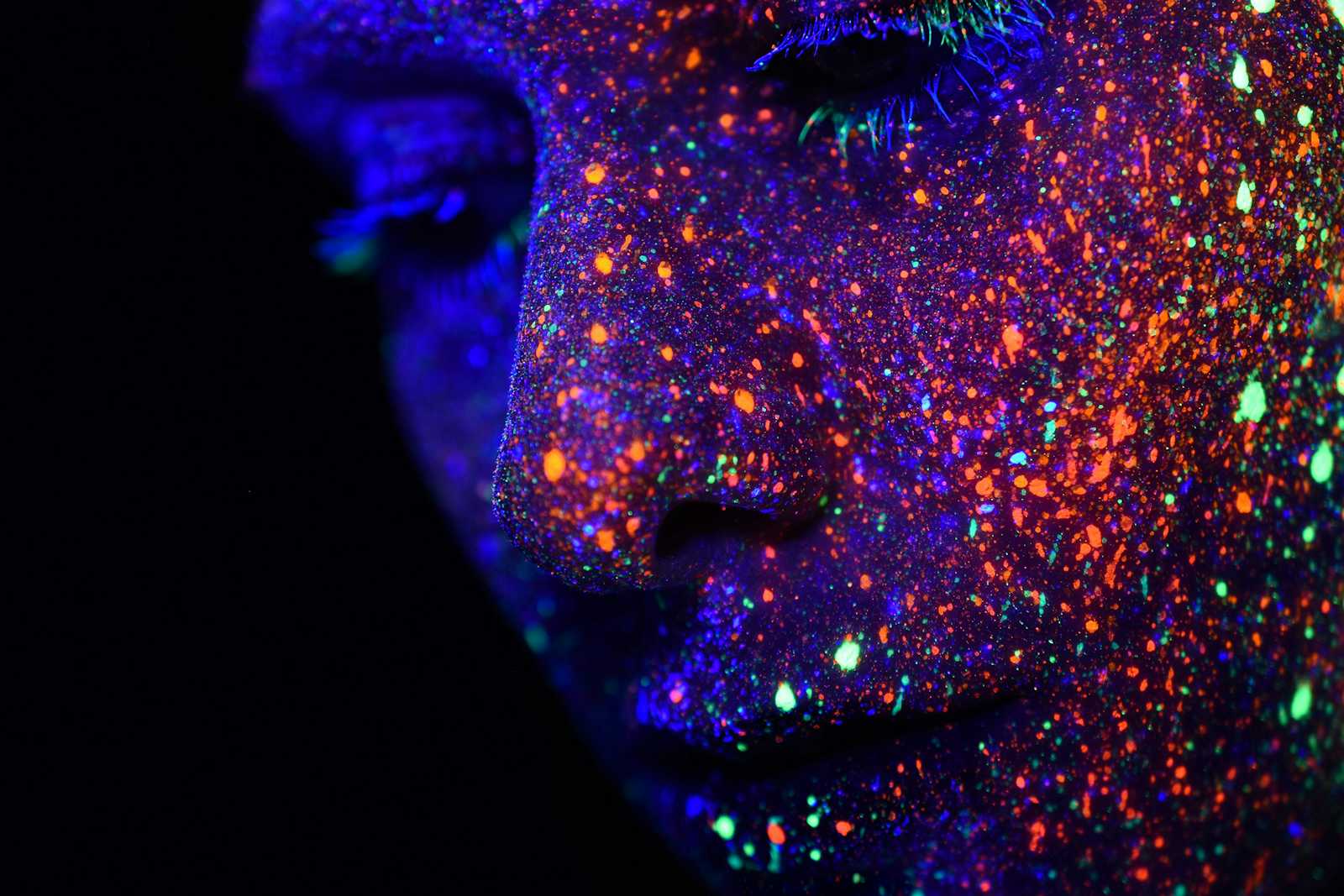
Introduction
Infrared imaging is a powerful tool used in various industries, from remote sensing to medical diagnostics. However, interpreting these images can be challenging because they capture data outside the visible spectrum. Converting infrared (IR) images to the visible light spectrum makes the data more accessible and easier to analyze. In this article, we’ll walk through how to build a scalable architecture on AWS to automate this conversion process using a Conditional Generative Adversarial Network (cGAN). We’ll dive deep into each step of the process, detailing the AWS services used and how they come together to create a robust solution.
Why Convert Infrared to Visible Light?
Infrared images capture thermal information, which is invaluable in scenarios like night vision, detecting heat leaks in buildings, and identifying health issues. However, these images are not intuitive for human interpretation. Converting them to the visible spectrum can enhance their usability, making it easier for analysts to draw meaningful conclusions.
Overview of the Architecture
We’ll use a combination of AWS services to create an end-to-end pipeline for converting infrared images to visible light images. Here’s a high-level overview of the process:
Data Storage with Amazon S3
Data Preprocessing with AWS Lambda
Model Training with Amazon SageMaker
Model Deployment with SageMaker Endpoints
Real-Time Inference Triggered by AWS Lambda
Visualization and Analysis with Amazon QuickSight
Monitoring with Amazon CloudWatch
Step-by-Step Implementation
Let’s break down each component of the architecture in detail.
1. Data Storage: Amazon S3
Purpose: Store infrared images and corresponding visible light images.
Setting Up S3 Buckets:
Create two S3 buckets: one for raw infrared images and another for processed visible light images.
Use S3’s folder structure to organize data by type, project, or any other logical segmentation.
Security and Access Control:
Apply IAM roles and policies to control access to the S3 buckets.
Use S3 encryption features to protect sensitive data at rest.
Versioning and Lifecycle Management:
Enable versioning to track changes to your data over time.
Implement lifecycle policies to automatically transition old data to lower-cost storage classes like S3 Glacier.
2. Data Preprocessing: AWS Lambda
Purpose: Preprocess the images before feeding them into the machine learning model.
Lambda Function Setup:
Write a Lambda function in Python (or another supported language) to handle image preprocessing tasks such as resizing, normalization, and data augmentation.
Use the
boto3
library to interact with S3, downloading images, processing them, and uploading the results back to S3.
Triggering the Function:
Configure S3 event notifications to trigger the Lambda function whenever a new image is uploaded to the infrared image bucket.
Set up error handling within the function to ensure robustness and reliability.
Code:
pythonCopy codeimport boto3 from PIL import Image import io s3 = boto3.client('s3') def lambda_handler(event, context): bucket_name = event['Records'][0]['s3']['bucket']['name'] key = event['Records'][0]['s3']['object']['key'] # Download the image from S3 s3_object = s3.get_object(Bucket=bucket_name, Key=key) image_content = s3_object['Body'].read() # Open the image using PIL image = Image.open(io.BytesIO(image_content)) # Preprocess the image (resize, normalize, etc.) image = image.resize((256, 256)) image = image.convert('RGB') # Save the processed image back to S3 buffer = io.BytesIO() image.save(buffer, 'JPEG') buffer.seek(0) s3.put_object(Bucket=bucket_name, Key=f'processed/{key}', Body=buffer)
3. Model Training: Amazon SageMaker
Purpose: Train the cGAN model on the preprocessed images.
Data Preparation:
- Use AWS Glue to create a data catalog and manage your dataset. Glue can help you transform and move data between S3 buckets, making it easier to manage your training data.
Building the cGAN Model:
Use SageMaker’s built-in notebook instances to develop your cGAN model. You can use frameworks like TensorFlow or PyTorch, both of which are supported natively in SageMaker.
Implement a cGAN where the generator takes an infrared image and outputs a visible light image, while the discriminator tries to distinguish between real and fake visible light images.
cGAN Architecture in PyTorch:
pythonCopy codeimport torch import torch.nn as nn class Generator(nn.Module): def __init__(self): super(Generator, self).__init__() self.main = nn.Sequential( nn.Conv2d(3, 64, 4, 2, 1, bias=False), nn.ReLU(True), # Additional layers here nn.ConvTranspose2d(64, 3, 4, 2, 1, bias=False), nn.Tanh() ) def forward(self, input): return self.main(input) class Discriminator(nn.Module): def __init__(self): super(Discriminator, self).__init__() self.main = nn.Sequential( nn.Conv2d(6, 64, 4, 2, 1, bias=False), nn.LeakyReLU(0.2, inplace=True), # Additional layers here nn.Conv2d(64, 1, 4, 1, 0, bias=False), nn.Sigmoid() ) def forward(self, input): return self.main(input)
Training the Model:
Use SageMaker’s managed training feature to scale your training across multiple GPUs or instances if needed.
Implement callbacks or logging to track the training progress using SageMaker’s built-in metrics.
Hyperparameter Tuning:
- Leverage SageMaker’s hyperparameter tuning to optimize the learning rate, batch size, and other critical parameters for better model performance.
4. Model Deployment: SageMaker Endpoint
Purpose: Deploy the trained cGAN model for real-time inference.
Creating the Endpoint:
After training, deploy the model directly from SageMaker by creating an endpoint. SageMaker handles the underlying infrastructure, allowing you to focus on deployment without worrying about server management.
Specify the instance type for your endpoint based on your performance needs. For example, if you require fast inference, choose a GPU instance like
ml.p2.xlarge
.
Setting Up Autoscaling:
- Enable autoscaling for your endpoint to handle variable traffic. SageMaker can automatically scale the number of instances based on demand, ensuring that your endpoint remains responsive even during peak usage.
Security:
Secure your endpoint by applying IAM policies that control which users or services can invoke it.
Use SSL/TLS to encrypt data in transit between your application and the endpoint.
5. Real-Time Inference: AWS Lambda
Purpose: Automate the inference process for new infrared images.
Integrating Lambda with the SageMaker Endpoint:
Write a Lambda function that triggers when a new infrared image is uploaded to the S3 bucket.
The function should retrieve the image, invoke the SageMaker endpoint, and store the generated visible light image back in S3.
Inference Code:
pythonCopy codeimport boto3 import json from io import BytesIO runtime = boto3.client('runtime.sagemaker') s3 = boto3.client('s3') def lambda_handler(event, context): bucket_name = event['Records'][0]['s3']['bucket']['name'] key = event['Records'][0]['s3']['object']['key'] # Download the infrared image from S3 s3_object = s3.get_object(Bucket=bucket_name, Key=key) image_content = s3_object['Body'].read() # Invoke the SageMaker endpoint response = runtime.invoke_endpoint(EndpointName='your-endpoint-name', ContentType='application/x-image', Body=image_content) # Process the response and store the generated image generated_image = response['Body'].read() s3.put_object(Bucket=bucket_name, Key=f'visible/{key}', Body=generated_image)
6. Visualization and Analysis: Amazon QuickSight
Purpose: Visualize the converted images and analyze the performance of the model.
Connecting QuickSight to S3:
- Use Amazon QuickSight to connect to your S3 buckets where the images are stored. QuickSight can read the data directly from S3, making it easy to visualize and analyze.
Creating Dashboards:
Create a dashboard in QuickSight that shows side-by-side comparisons of the infrared images and their converted visible light counterparts.
Add metrics to evaluate the model’s performance, such as the accuracy of the discriminator or the visual quality of the generated images.
Sharing Insights:
- QuickSight allows you to share your dashboards with team members or stakeholders, providing a collaborative environment for analyzing the results.
7. Monitoring and Logging: Amazon CloudWatch
Purpose: Monitor the performance of the Lambda functions and SageMaker endpoints.
Setting Up CloudWatch Alarms:
Configure CloudWatch alarms to monitor key metrics like Lambda execution time, SageMaker endpoint latency, and S3 bucket activity.
Set up notifications to alert you if any metric crosses a defined threshold, ensuring that you can respond quickly to issues.
Custom Metrics:
Create custom metrics in CloudWatch to track the specific performance of your cGAN model, such as inference times or the accuracy of the generated images.
Use these metrics to optimize the model and the overall pipeline.
Log Management:
- Use CloudWatch Logs to store logs from your Lambda functions and SageMaker endpoint invocations. These logs are invaluable for debugging and understanding the behavior of your system.
Deep Dive into Conditional GANs (cGANs)
What is a cGAN?
A Conditional Generative Adversarial Network (cGAN) is an extension of the traditional GAN, where the generation of images is conditioned on additional input. This input can be any data that the model uses to guide the generation process, such as an image, label, or text.
In our case, the input is an infrared image, and the cGAN is tasked with generating a corresponding visible light image.
How Does a cGAN Work?
Generator (G): The generator creates a visible light image conditioned on the infrared image. The generator’s goal is to produce an image that is indistinguishable from real visible light images.
Discriminator (D): The discriminator evaluates pairs of images (infrared and visible) to determine whether the visible image is real or generated. The discriminator tries to correctly classify real versus fake images.
The generator and discriminator are trained together in an adversarial manner:
The generator improves by learning to fool the discriminator.
The discriminator improves by becoming better at distinguishing real images from fake ones.
This adversarial training process continues until the generator produces highly realistic visible light images.
Benefits of Using cGANs
Conditional Control: By conditioning the image generation on the infrared input, cGANs ensure that the output image closely matches the features of the input image.
High-Quality Output: cGANs are known for producing realistic images that can be directly used in practical applications.
Implementation Workflow Recap
Here’s a recap of the complete implementation workflow:
Data Collection and Storage: Use Amazon S3 to store your dataset of paired infrared and visible light images.
Data Preprocessing: Employ AWS Lambda to preprocess images for model training.
Model Development: Build and train a cGAN model using Amazon SageMaker, optimizing hyperparameters for best performance.
Model Deployment: Deploy the trained cGAN as a SageMaker Endpoint for real-time inference.
Automated Inference: Set up AWS Lambda to trigger the inference process whenever new infrared images are uploaded to S3.
Visualization and Analysis: Use Amazon QuickSight to visualize and analyze the results, ensuring the model meets your quality standards.
Monitoring and Optimization: Implement comprehensive monitoring with Amazon CloudWatch to track performance and identify areas for improvement.
Conclusion
Converting infrared images to the visible light spectrum is a complex task that can be effectively handled using AWS services and a cGAN. This architecture provides a scalable, automated solution that leverages the full power of AWS, from data storage and processing to model training and deployment. By following the detailed steps outlined in this article, you can build a robust pipeline that transforms infrared images into visually interpretable data, unlocking new possibilities in various industries.
This idea not only showcases the synergy between advanced machine learning models like cGANs and AWS cloud services but also provides a clear, practical guide for implementing such a solution. Whether you're working in fields like remote sensing, medical imaging, or any other domain that relies on infrared data, this architecture can be tailored to meet your specific needs.
For more awesome projects about AWS and its services, follow this blog page, also consider following me on LinkedIn. Want to know more about me!! follow me on Instagram!!
Subscribe to my newsletter
Read articles from Shreyas Ladhe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shreyas Ladhe
Shreyas Ladhe
I am Shreyas Ladhe a pre final year student, an avid cloud devops enthusiast pursuing my B Tech in Computer Science at Indian Institute of Information Technology Vadodara ICD. I love to learn how DevOps tools help automate complex and recurring tasks. I also love to share my knowledge and my project insights openly to promote the open source aspect of the DevOps community.