How to Use Python for Batch Image Resizing and Compression
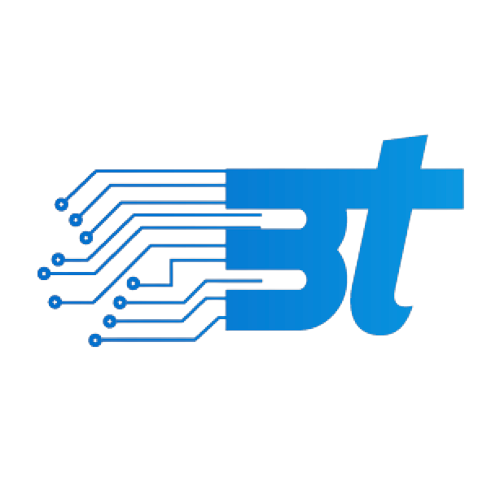
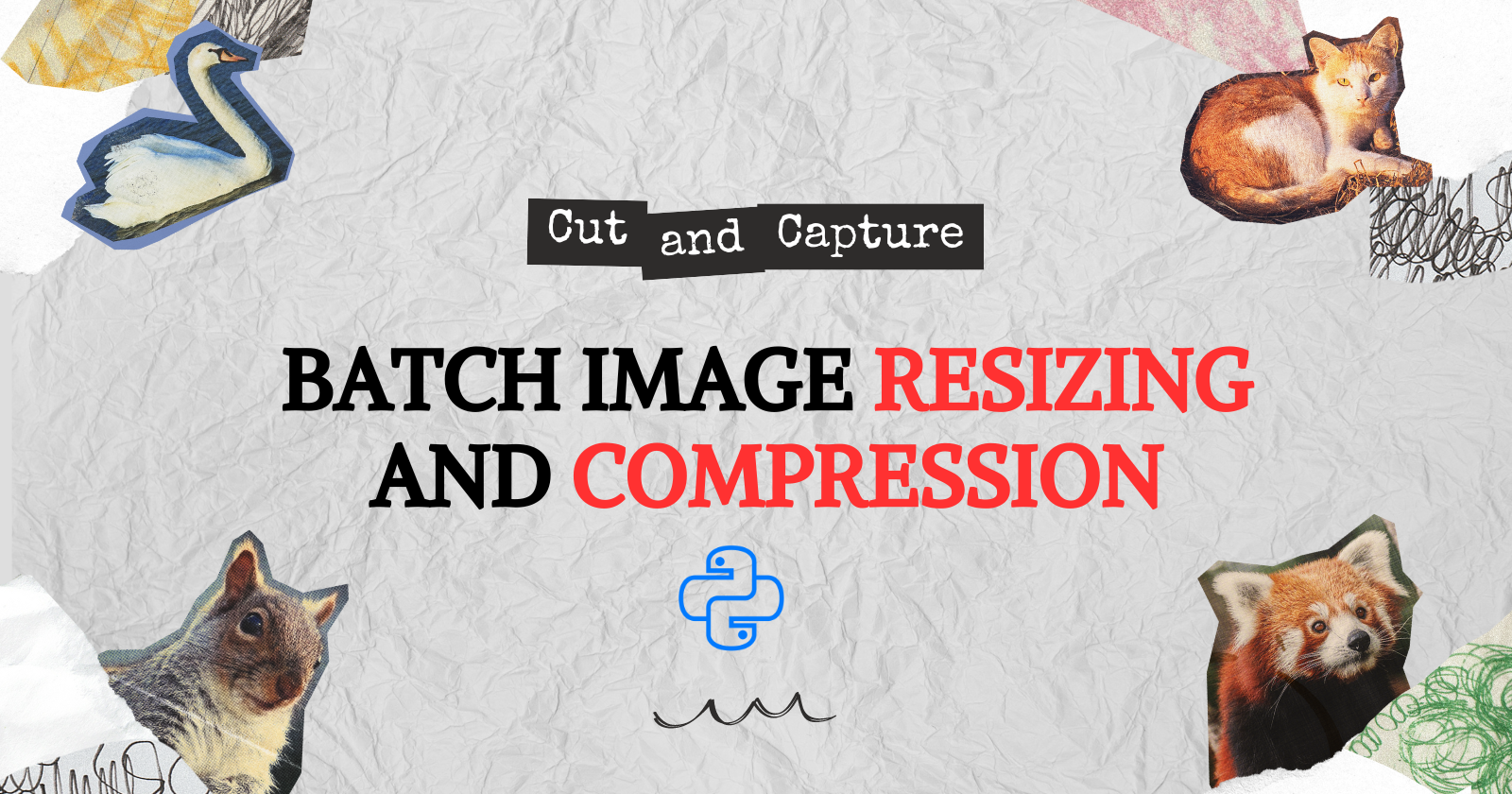
Managing a large collection of high-resolution images can be challenging, especially when storage space is limited, or you're preparing images for web use. Resizing and compressing images in bulk can save space and make your files easier to share or upload. In this tutorial, we’ll build a Python script to resize and compress images in bulk using the PIL
(Python Imaging Library) module, now known as Pillow
.
Why Resize and Compress Images?
Reduce File Size: Smaller images use less disk space and are faster to upload and download.
Improve Website Performance: Resized and compressed images load faster on web pages, improving user experience.
Optimize for Different Uses: Create image sizes suitable for various platforms like social media, web, or print.
Getting Started
To create a script that resizes and compresses images, you need to install the Pillow
library, a powerful tool for image manipulation in Python.
Step 1: Install Pillow
Install the Pillow library using pip:
pip install Pillow
Step 2: Write the Python Script
Here’s a Python script that resizes and compresses all images in a specified directory:
from PIL import Image
import os
def resize_and_compress_images(input_dir, output_dir, max_width, max_height, quality):
"""
Resize and compress images in the input directory and save them to the output directory.
Parameters:
- input_dir: Directory containing images to process.
- output_dir: Directory to save processed images.
- max_width: Maximum width for resizing.
- max_height: Maximum height for resizing.
- quality: Quality of the output image (1-100).
"""
# Ensure the output directory exists
os.makedirs(output_dir, exist_ok=True)
# Iterate through all files in the input directory
for filename in os.listdir(input_dir):
# Construct the full file path
file_path = os.path.join(input_dir, filename)
# Open the image file
with Image.open(file_path) as img:
# Resize the image
img.thumbnail((max_width, max_height))
# Save the compressed image to the output directory
output_path = os.path.join(output_dir, filename)
img.save(output_path, optimize=True, quality=quality)
print(f"Processed {filename} -> {output_path}")
if __name__ == "__main__":
input_directory = "images/input" # Directory with original images
output_directory = "images/output" # Directory for processed images
max_width = 800 # Max width for resizing
max_height = 600 # Max height for resizing
quality = 85 # Image quality (1-100)
resize_and_compress_images(input_directory, output_directory, max_width, max_height, quality)
How the Script Works
Importing Libraries: The script uses the
Image
class from Pillow to handle image processing.Setting Up Directories: The script takes an input directory containing the original images and an output directory where the resized and compressed images will be saved.
Resizing Images: The
thumbnail()
method is used to resize images while maintaining the aspect ratio, with the maximum width and height set by the user.Compressing Images: The
save()
method saves the resized image with the specified quality, optimizing the file size.Processing Each Image: The script iterates over all files in the input directory, processing each image file and saving the output to the specified directory.
Running the Script
Set the Input and Output Directories: Update the
input_directory
andoutput_directory
variables to point to your desired folders.Adjust the Resize and Compression Settings: Modify the
max_width
,max_height
, andquality
variables to match your needs.Execute the Script: Run the script in your Python environment to process all images in the specified directory.
Tips for Using the Script
Batch Processing: This script is ideal for batch processing large numbers of images, saving time and effort.
Different Image Formats: Pillow supports various image formats such as JPEG, PNG, GIF, and more, making it versatile for different use cases.
Custom Resizing: You can customize the resizing logic to crop images or apply different scaling techniques based on your requirements.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
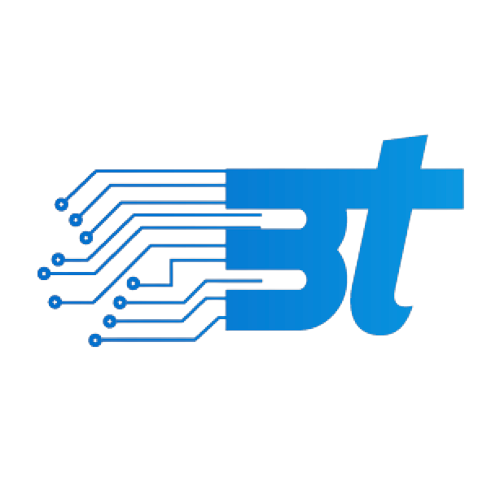
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.