Debounce vs Throttle: Understanding the Differences and When to Use Them
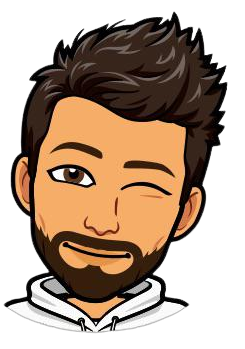
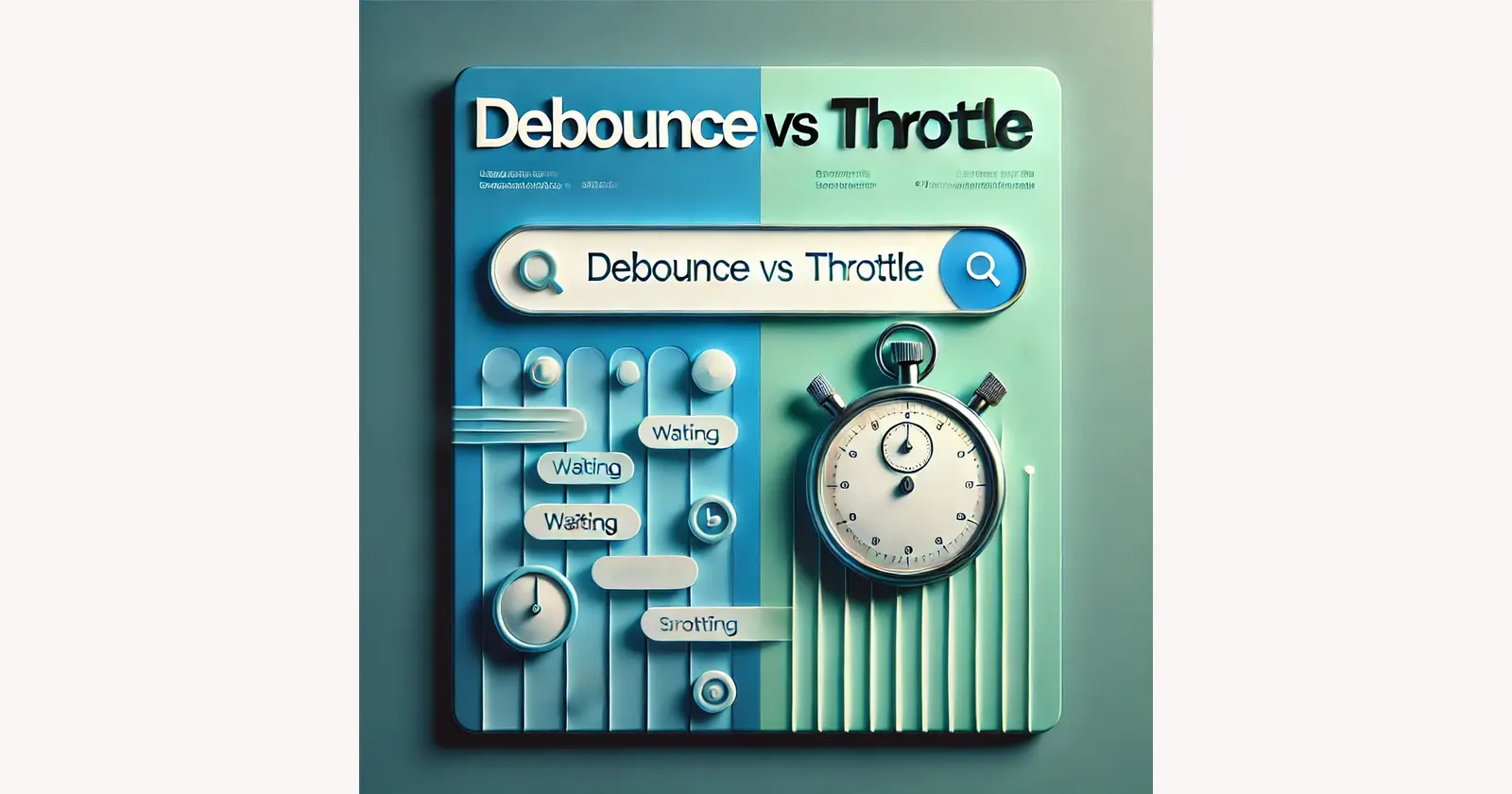
In modern web development, managing user interactions efficiently is crucial for ensuring a smooth and responsive user experience. Two techniques that are often employed to control the frequency of function executions in response to user actions are debouncing and throttling. Both serve to optimize performance by limiting the number of times a function is invoked, but they do so in different ways and are suited for different use cases. Understanding when and how to use each can make a significant difference in the performance of your application.
What is Debouncing?
Debouncing is a technique used to delay the execution of a function until a certain amount of time has passed since the last time it was invoked. If the function is triggered again before the delay has expired, the timer is reset. This ensures that the function is executed only once after the user has stopped triggering it.
Example Use Case:
A common scenario for using debouncing is in search input fields. When a user types into a search box, each keystroke could potentially trigger an API call to fetch search results. However, if you debounce the input, the API call will only be made after the user has stopped typing for a specified duration, preventing unnecessary requests and reducing the load on the server.
Implementation:
Here’s a basic example of how debouncing can be implemented in JavaScript:
function debounce(func, delay) {
let timeoutId;
return function(...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => func.apply(this, args), delay);
};
}
// Usage
const handleSearch = debounce((query) => {
// API call to fetch search results
console.log(`Searching for ${query}`);
}, 300);
// Triggered on every keyup event in an input field
document.querySelector('input').addEventListener('keyup', (e) => {
handleSearch(e.target.value);
});
In this example, the handleSearch
function will only execute 300 milliseconds after the user stops typing.
What is Throttling?
Throttling is a technique that limits the execution of a function to once every specified period, regardless of how often the event occurs. This ensures that the function is called at regular intervals, even if the event is triggered many times in rapid succession.
Example Use Case:
Throttling is ideal for scenarios like handling window resize or scroll events, where the event can fire numerous times per second. By throttling the event handler, you ensure that the function is only called at a fixed rate, reducing the strain on the browser and improving performance.
Implementation:
Here’s how throttling can be implemented:
function throttle(func, limit) {
let lastFunc;
let lastRan;
return function(...args) {
const context = this;
if (!lastRan) {
func.apply(context, args);
lastRan = Date.now();
} else {
clearTimeout(lastFunc);
lastFunc = setTimeout(function() {
if ((Date.now() - lastRan) >= limit) {
func.apply(context, args);
lastRan = Date.now();
}
}, limit - (Date.now() - lastRan));
}
};
}
// Usage
const handleScroll = throttle(() => {
// Logic to handle scroll event
console.log('Scroll event handled');
}, 1000);
// Triggered on every scroll event
window.addEventListener('scroll', handleScroll);
In this example, the handleScroll
function will only be executed once every 1000 milliseconds, even if the scroll event fires continuously.
Debounce vs Throttle: Key Differences
Timing:
Debounce: The function executes only after a specified delay has passed without further invocations. It’s ideal for scenarios where you want to wait for a user to stop performing an action before executing the function.
Throttle: The function executes at regular intervals, ensuring it’s called at most once every specified period, regardless of how frequently the event occurs.
Use Cases:
Debounce: Best for user input events, such as search fields or resize events, where you want to wait until the user is finished before acting.
Throttle: Ideal for events that occur frequently and where performance is critical, such as scrolling, resizing, or mouse movements.
Implementation:
Debounce resets the delay timer each time the event is triggered, so the function is only called after the events stop.
Throttle limits the function execution to a set rate, so the function is called at a consistent interval, regardless of how often the event is triggered.
When to Use Debounce vs Throttle
Debounce:
When you want to ensure that a function is executed only after a series of rapid events has ended.
Examples: Search input, form validation, resizing elements.
Throttle:
When you want to ensure that a function is executed at regular intervals during a series of rapid events.
Examples: Scroll events, API polling, rate-limiting user actions.
Conclusion
Both debouncing and throttling are essential techniques for optimizing performance and improving user experience in web applications. By understanding the differences and knowing when to use each, you can write more efficient and responsive code. Whether you're handling user input, API requests, or frequent browser events, these techniques can help you avoid unnecessary function calls and ensure your application runs smoothly.
Subscribe to my newsletter
Read articles from Shubham Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
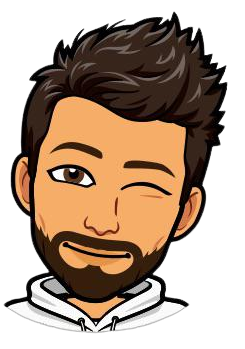
Shubham Jain
Shubham Jain
I work as an SDE-1 in frontend role at a healthcare startup. I am always curious to learn new technologies and share my learnings. I love development and love to code.