Inline Caching in Node.js: A Journey to Better Performance
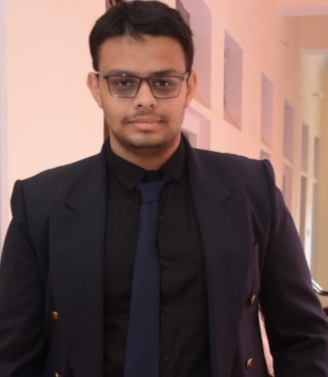
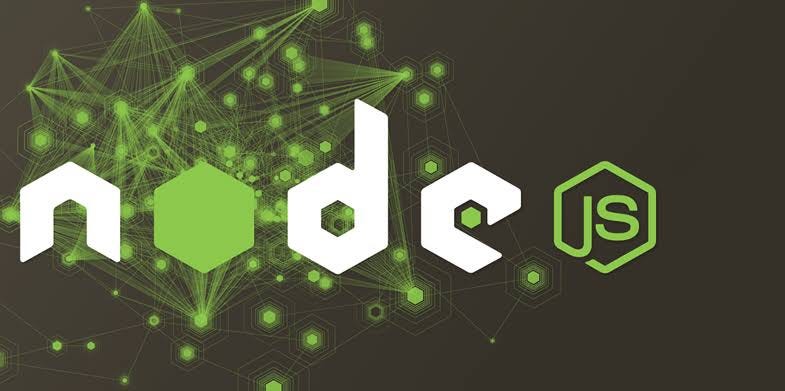
When I first started working with JavaScript and Node.js, I quickly realized how important performance optimization was for scaling applications. In my quest to understand how JavaScript engines make code run faster, I came across the concept of inline caching. Although it sounded like a complex and low-level optimization technique at first, diving into it changed my perspective on how JavaScript engines, like V8, optimize our code under the hood.
Let me take you through what I learned about inline caching and why it's so crucial for JavaScript performance.
What is Inline Caching?
Inline caching is a performance optimization technique used by JavaScript engines to speed up the access of object properties. At its core, inline caching is all about caching the locations of properties within objects so that future accesses are faster.
To put it simply, when JavaScript code accesses a property on an object, the engine first has to figure out where that property is located. The process of finding and accessing the property can be slow, especially if it involves navigating the object's prototype chain. Inline caching helps by storing the location of the property once it’s been accessed, so subsequent accesses can be much faster.
My First Encounter with Inline Caching
I remember working on a Node.js project that involved processing a large amount of data and frequently accessing object properties. Initially, everything was running fine, but as the data set grew, the application started to slow down significantly. That’s when I started reading about different optimization techniques, and I stumbled upon inline caching.
Inline caching seemed like a hidden gem. It wasn't something I could directly implement or tweak, but understanding how it worked helped me write better code that played nicely with the JavaScript engine’s optimization strategies.
How Inline Caching Works
Let me break down how inline caching works, using a simple example:
function getValue(obj) {
return obj.value;
}
let obj1 = { value: 42 };
let obj2 = { value: 17 };
console.log(getValue(obj1)); // First access
console.log(getValue(obj2)); // Second access
First Access: When the
getValue
function is called withobj1
, the JavaScript engine does not know where thevalue
property is located. It has to search for it, a process that involves checking the object itself and possibly walking up the prototype chain. This initial lookup is slower because it needs to determine the location ofvalue
.Caching: After finding
value
inobj1
, the engine stores (or caches) this information, including the object's shape (which properties it has and their types).Subsequent Access: When
getValue
is called again withobj2
, ifobj2
has the same shape asobj1
, the engine uses the cached information. It already knows where to findvalue
inobj2
, so the lookup is much faster.
Why Inline Caching Matters
I found that inline caching is a game-changer for performance because it reduces the need for repetitive and expensive property lookups. By caching the location of properties, the JavaScript engine can optimize code execution paths, leading to faster and more efficient code.
In the project I was working on, understanding this allowed me to structure my objects and their properties in a way that was more predictable for the engine. I started to notice performance improvements, especially in loops and functions that were accessing object properties frequently. This wasn’t just about writing efficient code—it was about writing code that the engine could optimize effectively.
My Key Takeaways:
Consistency is Key: When writing JavaScript, if you can maintain consistent object shapes (the properties and their types), you’re helping the JavaScript engine optimize with inline caching. Avoid frequently changing the structure of objects dynamically, as this can invalidate caches and slow down execution.
Frequent Property Access: If your code frequently accesses properties on objects, inline caching will naturally kick in and optimize these accesses. It's one of those things that just works behind the scenes, making your code run faster without any manual intervention.
Writing Engine-Friendly Code: Knowing about inline caching has influenced how I write JavaScript. I’m more mindful of how I structure objects and how I access their properties. Writing engine-friendly code isn’t about knowing all the deep internals of V8, but rather understanding key concepts like inline caching and aligning your code with them.
Conclusion
Inline caching might seem like an advanced optimization technique reserved for the inner workings of JavaScript engines, but as developers, we benefit greatly from understanding it. By keeping our code predictable and consistent, we allow JavaScript engines to do what they do best: optimize.
For me, learning about inline caching was an eye-opener. It’s a reminder of how much thought and complexity goes into making JavaScript fast and efficient. Even though it’s not something we control directly, understanding it helps us write better code, and that’s a powerful tool to have as a developer.
So, the next time you're optimizing your Node.js application, remember that sometimes the best optimizations come from simply understanding how the language and its engines work. Happy coding!
Subscribe to my newsletter
Read articles from SANKALP HARITASH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
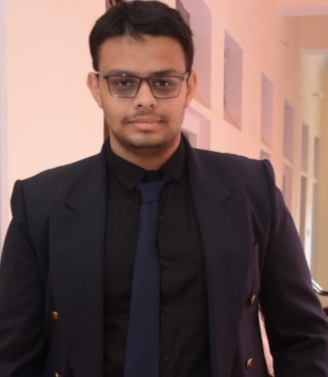
SANKALP HARITASH
SANKALP HARITASH
Hey 👋🏻, I am , a Software Engineer from India. I am interested in, write about, and develop (open source) software solutions for and with JavaScript, ReactJs. 📬 Get in touch Twitter: https://x.com/SankalpHaritash Blog: https://sankalp-haritash.hashnode.dev/ LinkedIn: https://www.linkedin.com/in/sankalp-haritash/ GitHub: https://github.com/SankalpHaritash21 📧 Sign up for my newsletter: https://sankalp-haritash.hashnode.dev/newsletter