Automate Data Backup and Sync Across Devices Using Python
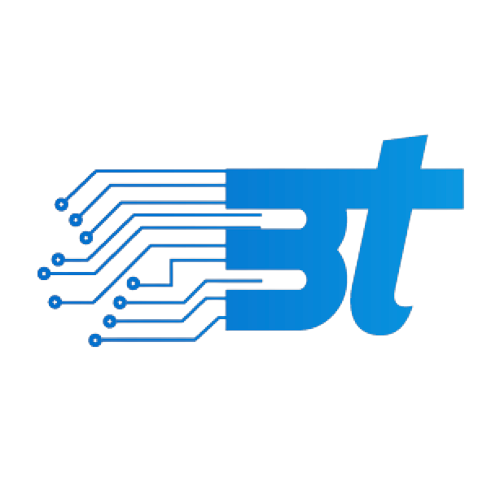
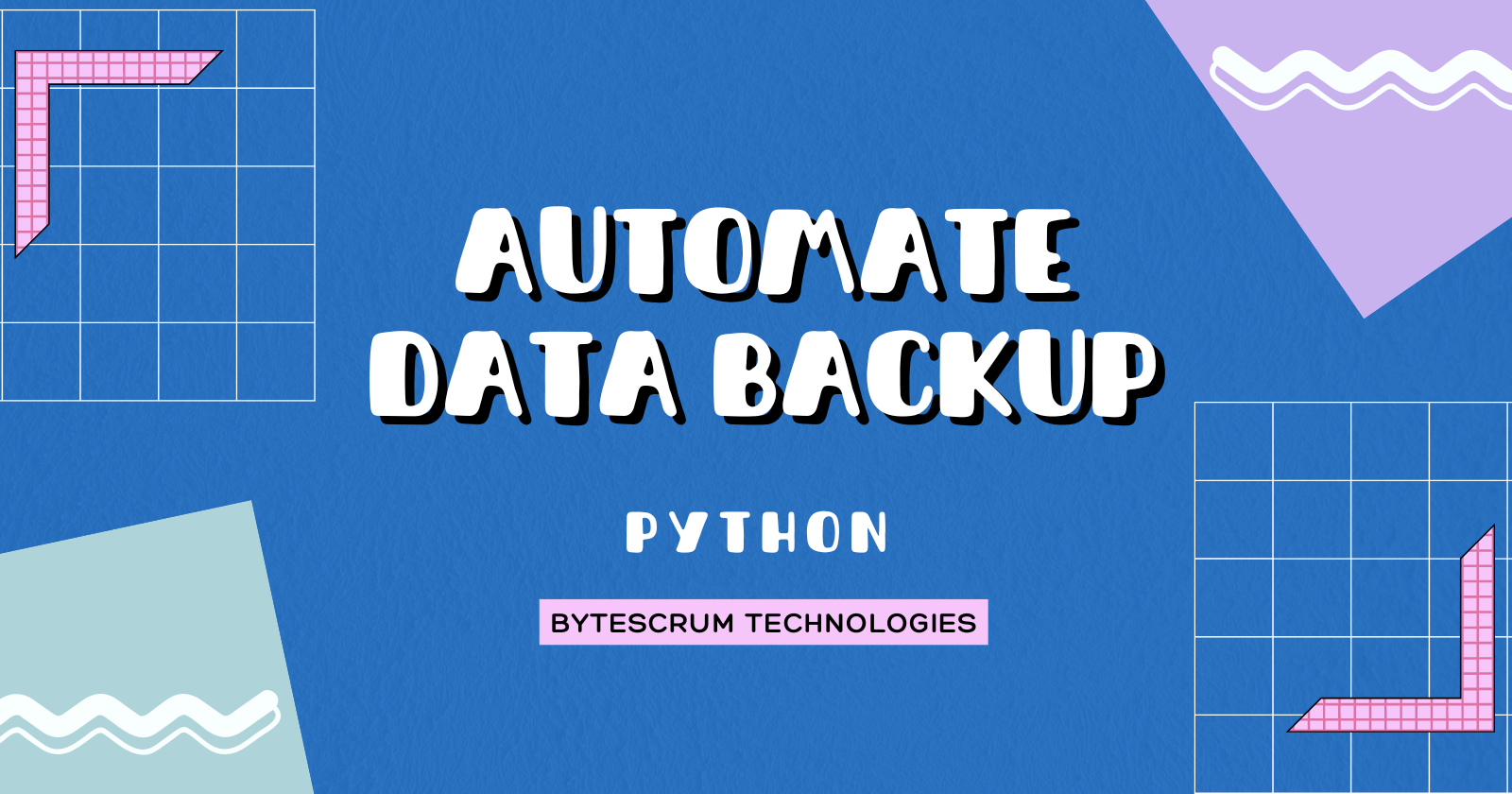
Data loss can be catastrophic, whether due to accidental deletion, hardware failure, or malicious attacks. Regular backups and synchronization across devices are essential practices to safeguard your files. Automating this process with Python not only saves time but also ensures consistency across all your devices. In this guide, we'll walk you through creating a Python script to automate data backup and synchronization across multiple devices.
Why Automate Data Backup and Sync?
Data Security: Regular backups protect against data loss due to hardware failures or accidental deletions.
Convenience: Automated processes run without manual intervention, ensuring backups are always up-to-date.
Consistency: Keep files synchronized across multiple devices, such as laptops, desktops, and external drives.
Redundancy: Having multiple copies of your data reduces the risk of losing important files.
Getting Started
To create an automated backup and sync script in Python, we’ll use the following libraries:
shutil
: For high-level file operations.os
: To interact with the operating system.datetime
: To timestamp backups.subprocess
: For running system commands likersync
(useful for synchronization).logging
: For creating logs to track the backup and sync processes.
Step 1: Install Required Libraries
Most of the libraries (shutil
, os
, datetime
, subprocess
, logging
) are part of Python’s standard library, so no additional installation is necessary. However, for more advanced use cases, you might consider libraries like rsync
for Unix-based systems, or paramiko
for SSH-based remote backups.
Step 2: Write the Python Script
Here’s a script to automate the backup and synchronization of files:
import os
import shutil
import datetime
import subprocess
import logging
def setup_logging():
"""
Set up logging configuration to log backup and sync processes.
"""
logging.basicConfig(
filename='backup_sync.log',
level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s',
)
def backup_files(source_dirs, backup_dir):
"""
Back up files from the source directories to the backup directory.
Parameters:
- source_dirs: List of directories to back up.
- backup_dir: Directory where backups will be stored.
"""
# Ensure the backup directory exists
os.makedirs(backup_dir, exist_ok=True)
# Timestamp for the backup folder
timestamp = datetime.datetime.now().strftime('%Y-%m-%d_%H-%M-%S')
backup_path = os.path.join(backup_dir, f"backup_{timestamp}")
# Create the timestamped backup directory
os.makedirs(backup_path, exist_ok=True)
for source_dir in source_dirs:
if os.path.exists(source_dir):
# Copy each directory to the backup location
try:
shutil.copytree(source_dir, os.path.join(backup_path, os.path.basename(source_dir)))
logging.info(f"Backed up {source_dir} to {backup_path}")
except Exception as e:
logging.error(f"Failed to back up {source_dir}: {e}")
else:
logging.warning(f"Source directory {source_dir} does not exist. Skipping.")
def sync_directories(source, target):
"""
Synchronize files between the source and target directories.
Parameters:
- source: Source directory to sync from.
- target: Target directory to sync to.
"""
# Using rsync to sync directories (Linux/macOS)
try:
subprocess.run(["rsync", "-av", source, target], check=True)
logging.info(f"Synchronized {source} to {target}")
except subprocess.CalledProcessError as e:
logging.error(f"Error during synchronization: {e}")
except Exception as e:
logging.error(f"Unexpected error during synchronization: {e}")
if __name__ == "__main__":
setup_logging()
source_directories = ["/path/to/source1", "/path/to/source2"] # Directories to back up
backup_directory = "/path/to/backup" # Backup destination
sync_source = "/path/to/source" # Source for synchronization
sync_target = "/path/to/target" # Target for synchronization
# Perform backup
backup_files(source_directories, backup_directory)
# Synchronize directories
sync_directories(sync_source, sync_target)
How the Script Works
Importing Libraries: The script uses
os
,shutil
,datetime
,logging
, andsubprocess
to handle file operations, generate timestamps, log activities, and execute system commands.Setting Up Logging:
setup_logging
initializes logging to keep track of the backup and sync processes.Logs are saved in a file called
backup_sync.log
to help you monitor activities and debug issues.
Backup Files:
The
backup_files
function iterates over a list of source directories.Each directory is copied to a backup location, which is timestamped to differentiate backups.
shutil.copytree()
is used to copy entire directories recursively. Logging is used to record successes and errors.
Synchronize Directories:
The
sync_directories
function uses thersync
command to synchronize files between two directories.rsync
is a robust and efficient utility for file synchronization, particularly on Linux and macOS.The
subprocess.run()
function executesrsync
with the-av
options (archive mode and verbose output).Logging is used to capture the outcome of synchronization.
Main Function:
Specifies the directories to back up and sync.
Calls the
backup_files
andsync_directories
functions.
Enhancing the Script for More Robust Backups
To make your backup and synchronization script even more powerful, consider the following enhancements:
Incremental Backups: Modify the script to perform incremental backups, only copying files that have changed since the last backup. This can be done by comparing file modification times or using a tool like
rsync
with the--update
or-u
flag.Compression: Compress backups to save space. Python’s
shutil
module provides themake_archive()
function to create compressed zip or tar files.Encryption: For sensitive data, encrypt backups before storing them. Use Python libraries like
cryptography
orPyCrypto
to encrypt files.Remote Backup: Implement remote backups using SSH and tools like
scp
orrsync
over SSH. For cloud storage, use APIs likeboto3
for AWS S3 orgoogle-cloud-storage
for Google Cloud.Backup Verification: Verify the integrity of backups by comparing checksums (using
hashlib
) of the original and backed-up files.Notification System: Set up email notifications or integrate with messaging apps (like Slack or Telegram) to receive alerts about backup status or errors.
Running the Script
Set Your Directories: Replace the placeholders (
/path/to/source1
,/path/to/backup
, etc.) with your actual directories.Adjust Backup and Sync Settings: Customize the script to include or exclude specific directories and files.
Automate Execution: Schedule the script to run automatically at regular intervals:
Linux/macOS: Use cron jobs (
crontab -e
to edit) to schedule the script.Windows: Use Task Scheduler to set up a recurring task for the script.
Monitor Logs: Check the
backup_sync.log
file regularly to ensure backups and synchronization are running smoothly and address any issues promptly.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
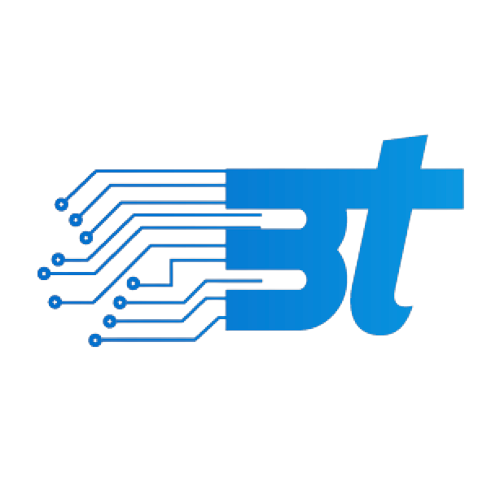
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.