Building FullStack Clone Application with Nextjs and NextAuth Prisma
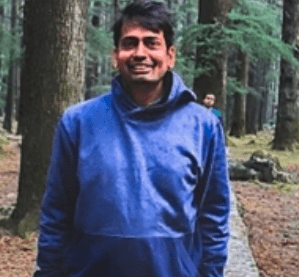
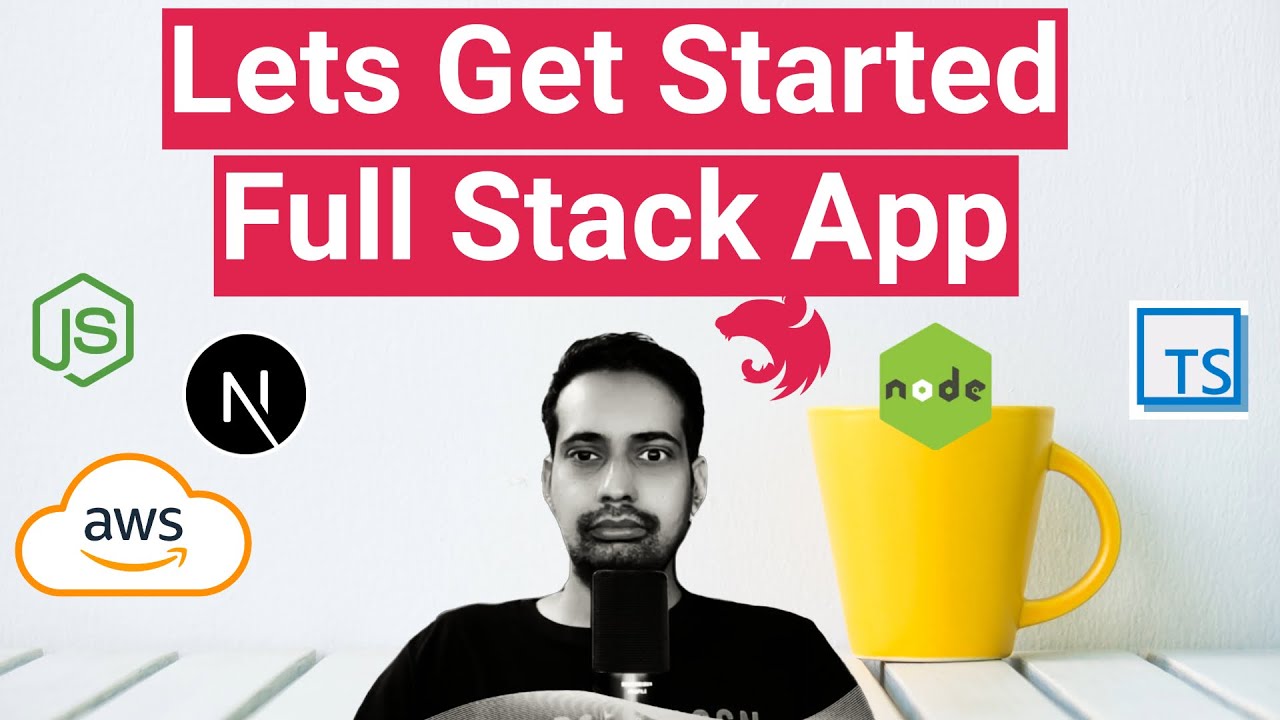
Building a Full-Stack Clone Application with Next.js, NextAuth.js, and Prisma
Introduction
In this blog post, we'll explore how to build a full-stack clone application using the powerful combination of Next.js, NextAuth.js, and Prisma. These tools offer a streamlined and efficient way to develop modern web applications with robust features.
Plsylist Link
https://www.youtube.com/watch?v=-AsbruYYFpU&list=PLU-FndeNRY4Cw7Kq7SHtqpI5bK06s8wt0
Github link
https://github.com/tkssharma/buddy-clone/tree/develop
Understanding the Tools
Next.js: A popular React framework for building server-rendered or static websites and web applications. It offers features like server-side rendering, automatic code splitting, and hybrid static & server rendering.
NextAuth.js: A simple, flexible, and easy-to-use authentication library for Next.js applications. It supports various authentication providers like Google, GitHub, Facebook, and more.
Prisma: A modern ORM (Object-Relational Mapper) that simplifies database interactions. It generates type-safe database clients and provides features like migrations, querying, and relations.
Setting Up the Project
Create a new Next.js project: Bash
npx create-next-app my-app
Install required dependencies: Bash
cd my-app npm install next-auth @prisma/client
Set up Prisma:
Create a
.env
file with your Prisma database connection details.Run
npx prisma init
to initialize Prisma.Create your Prisma schema file (
schema.prisma
).
Implementing Authentication with NextAuth.js
Configure NextAuth.js:
Create a
pages/api/auth/[...nextauth].js
file.Configure the providers you want to support (e.g., Google, GitHub).
Set up callbacks for sign-in, sign-out, and error handling.
Protect routes:
- Use the
getServerSideProps
method in your pages to check for authentication and redirect if necessary.
- Use the
Using Prisma for Data Management
Define your Prisma schema:
- Define your data models, fields, and relationships in the
schema.prisma
file.
- Define your data models, fields, and relationships in the
Generate database clients:
- Run
npx prisma generate
to generate TypeScript types and database clients.
- Run
Interact with the database:
- Use the generated Prisma client to query, create, update, and delete data.
Building Your Application
Create pages and components:
- Structure your application using Next.js's routing system and component-based architecture.
Fetch data:
- Use Prisma to fetch data from the database and pass it to your components.
Handle authentication:
- Use the
getServerSideProps
method to check for authentication and redirect if necessary.
- Use the
Implement features:
- Build your application's features using Next.js, React, and other necessary libraries.
Example Code Snippet
JavaScript
// pages/api/auth/[...nextauth].js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.GitHub({
clientId: process.env.GITHUB_ID,
clientSecret: process.env.GITHUB_SECRET,
}),
// ... other providers
],
});
// pages/index.js
import { getSession } from 'next-auth/react';
export default function Home({ session }) {
return (
<div>
{session ? (
<p>Signed in as {session.user.name}</p>
) : (
<p>Not signed in</p>
)}
</div>
);
}
export async function getServerSideProps(context) {
const session = await getSession(context);
return {
props: { session },
};
}
Conclusion
By leveraging Next.js, NextAuth.js, and Prisma, you can efficiently build full-stack applications with robust authentication, data management, and a great user experience. This powerful combination provides a solid foundation for creating scalable and maintainable web applications.
Subscribe to my newsletter
Read articles from tkssharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
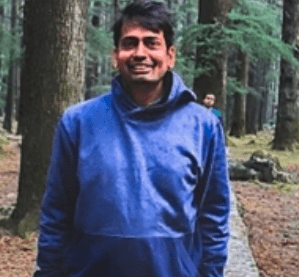
tkssharma
tkssharma
I'm a full-stack software developer creating open-source projects and writing about modern JavaScript client-side and server-side. Working remotely from India.