NestJS Authentication and Authorization with Auth0
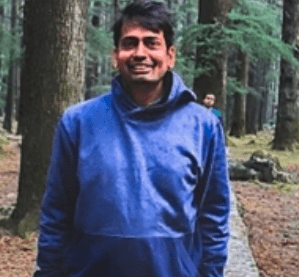
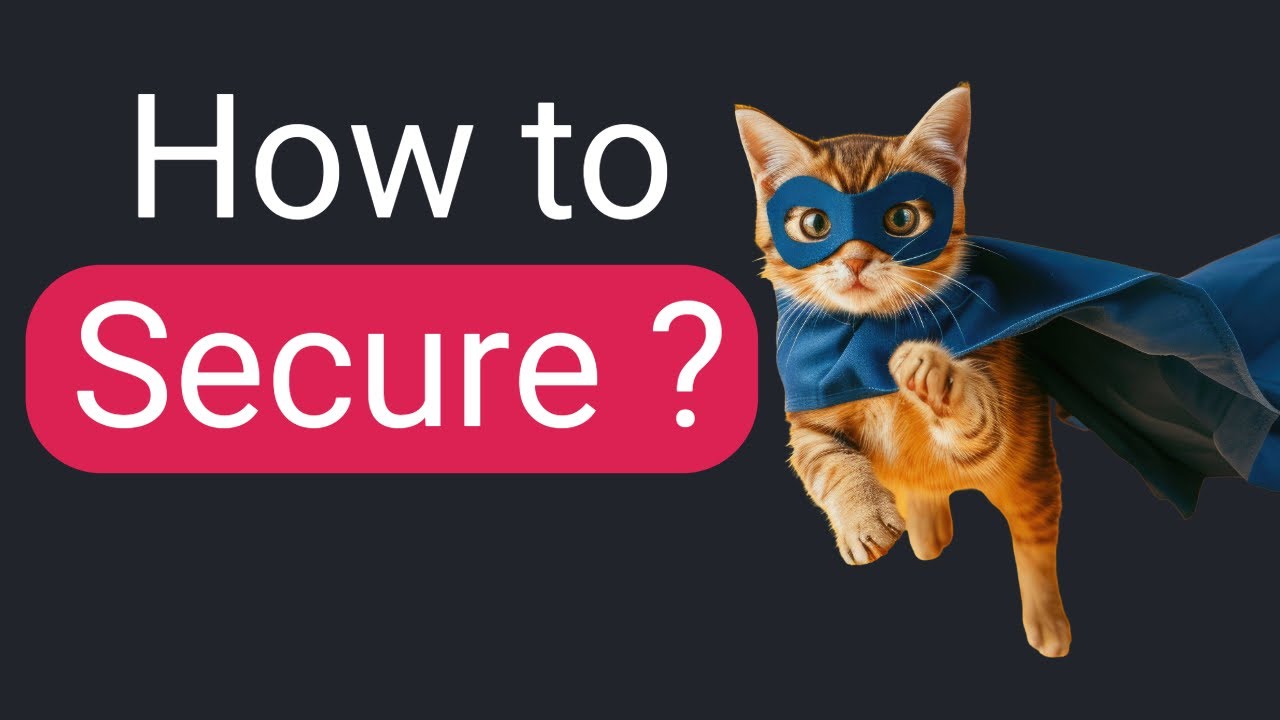
%[https://www.youtube.com/watch?v=1P44lR6JBzA]
NestJS Authentication and Authorization with Auth0: A Comprehensive Guide

Introduction
Implementing robust authentication and authorization is crucial for any web application. NestJS, a popular Node.js framework, provides a solid foundation for building secure and scalable applications. In this blog post, we'll explore how to integrate Auth0, a leading identity management platform, with NestJS to streamline the authentication process and enhance security.
Understanding Auth0
Auth0 is a cloud-based identity and access management platform that simplifies the process of adding authentication and authorization to your applications. It supports a wide range of authentication methods, including social logins, password-based authentication, and custom authentication flows. Auth0 also provides features like multi-factor authentication, single sign-on, and role-based access control.
Setting Up Auth0
Create an Auth0 account: Sign up for a free or paid Auth0 account.
Create a new application: Specify the application's name, callback URL, and other relevant settings.
Generate API credentials: Obtain your client ID and client secret.
Integrating Auth0 with NestJS
Install the
@auth0/auth0-spa
package:npm install @auth0/auth0-spa
Create an authentication service:
import { Injectable } from '@nestjs/common'; import { Auth0Client } from '@auth0/auth0-spa'; @Injectable() export class AuthService { auth0Client: Auth0Client; constructor() { this.auth0Client = new Auth0Client({ domain: 'your-domain.auth0.com', clientId: 'your-client-id', redirectUri: 'http://localhost:3000/callback', }); } login() { this.auth0Client.loginWithRedirect({ redirectUri: window.location.origin }); } }
Protect routes: Use NestJS's built-in guards to protect routes based on user authentication and authorization. For example, you can use the
AuthGuard
to require authentication for specific routes:import { Controller, Get, UseGuards } from '@nestjs/common'; import { AuthGuard } from '@nestjs/passport'; @Controller('profile') @UseGuards(AuthGuard()) export class ProfileController { @Get() getProfile() { // Access user data here } }
Additional Considerations
Handle authentication errors: Implement error handling to gracefully deal with authentication failures.
Implement role-based authorization: Use Auth0's rules engine to define access control policies based on user roles.
Secure your application: Follow best practices for protecting your application from security vulnerabilities.
Consider using a library like
@nestjs/passport
: This library provides pre-built strategies for various authentication providers, simplifying integration.
By following these steps and leveraging Auth0's powerful features, you can effectively implement authentication and authorization in your NestJS applications, ensuring a secure and user-friendly experience.
Subscribe to my newsletter
Read articles from tkssharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
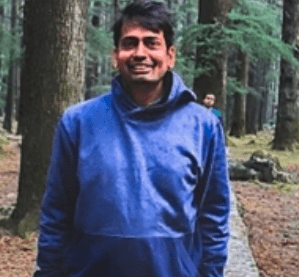
tkssharma
tkssharma
I'm a full-stack software developer creating open-source projects and writing about modern JavaScript client-side and server-side. Working remotely from India.