Nestjs File Upload to AWS S3 & Azure Blob
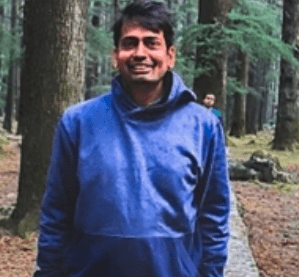
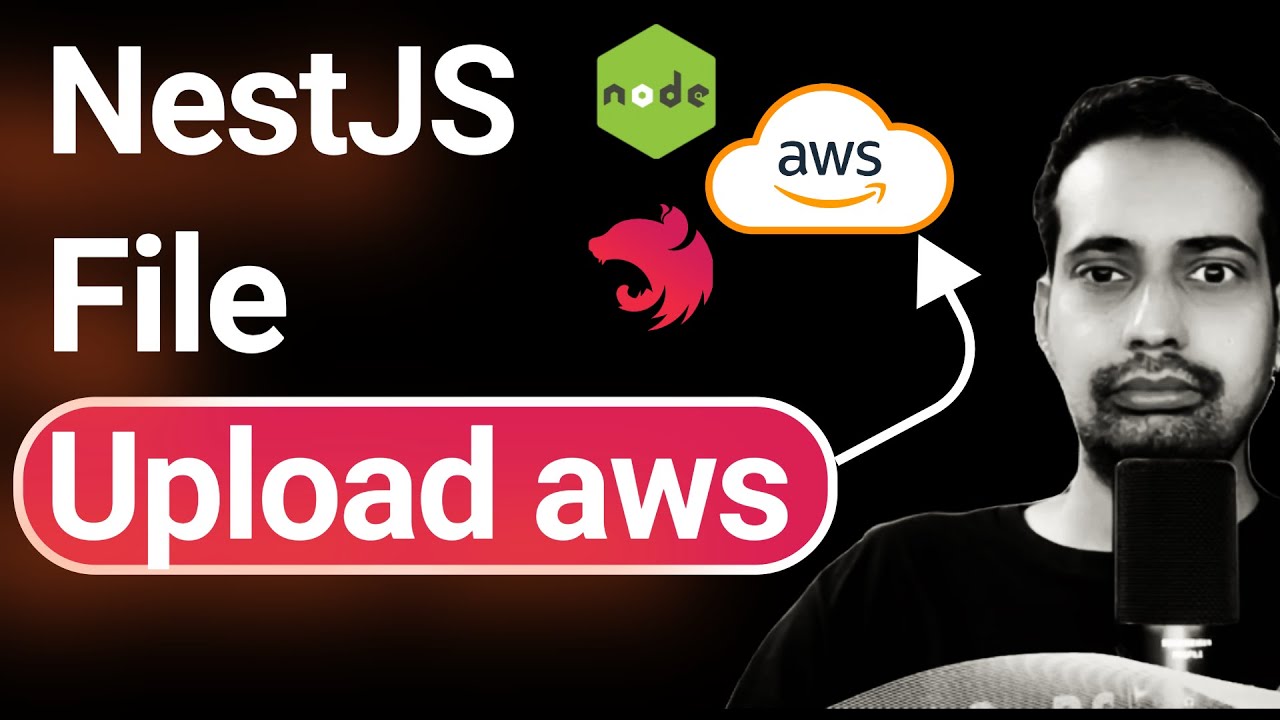

Part 1 & Part-2
Introduction
File uploads are a common requirement in many web applications. NestJS, a popular Node.js framework, provides a flexible and efficient way to handle file uploads. In this blog post, we'll explore how to integrate AWS S3 and Azure Blob Storage with NestJS to securely store and manage uploaded files.
AWS S3 Integration
Create an AWS account and S3 bucket: Set up your AWS account and create an S3 bucket to store your uploaded files.
Install the AWS SDK: Bash
npm install @nestjs/aws-sdk/s3
Configure AWS credentials: Create a
.env
file with your AWS access key ID and secret access key.Create an S3 service: TypeScript
import { Injectable } from '@nestjs/common'; import { S3 } from '@nestjs/aws-sdk/s3'; @Injectable() export class S3Service { constructor(private readonly s3: S3) {} async uploadFile(file: Express.Multer.File) { const params = { Bucket: 'your-bucket-name', Key: file.originalname, Body: file.buffer, ContentType: file.mimetype, }; await this.s3.upload(params).promise(); } }
Azure Blob Storage Integration
Create an Azure account and storage account: Set up your Azure account and create a storage account.
Install the Azure Storage SDK: Bash
npm install @azure/storage-blob
Configure Azure credentials: Create a
.env
file with your Azure storage account connection string.Create a Blob service: TypeScript
import { Injectable } from '@nestjs/common'; import { BlobServiceClient, ContainerClient, BlockBlobClient } from '@azure/storage-blob'; @Injectable() export class BlobStorageService { private blobServiceClient: BlobServiceClient; private containerClient: ContainerClient; constructor() { this.blobServiceClient = new BlobServiceClient(process.env.AZURE_STORAGE_CONNECTION_STRING); this.containerClient = this.blobServiceClient.getContainerClient('your-container-name'); } async uploadFile(file: Express.Multer.File) { const blockBlobClient = this.containerClient.getBlockBlobClient(file.originalname); await blockBlobClient.uploadData(file.buffer); } }
Key Considerations
File format and size: Ensure compatibility with the chosen storage provider.
Access control: Implement appropriate access controls to protect your files.
Performance optimization: Consider caching and CDN strategies for improved performance.
Cost management: Monitor usage and costs associated with storage and data transfer.
Choosing Between AWS S3 and Azure Blob Storage
The best choice for your project depends on various factors, including your existing cloud infrastructure, pricing, and specific requirements. Both AWS S3 and Azure Blob Storage offer robust features and scalability for handling file uploads in NestJS applications.
By following these steps and considering the key factors, you can effectively integrate file uploads into your NestJS applications using either AWS S3 or Azure Blob Storage.
Subscribe to my newsletter
Read articles from tkssharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
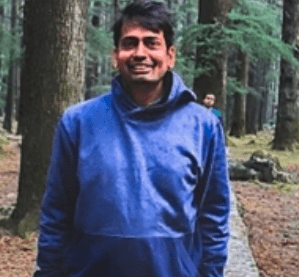
tkssharma
tkssharma
I'm a full-stack software developer creating open-source projects and writing about modern JavaScript client-side and server-side. Working remotely from India.