An Advanced Guide For JUnit Testing
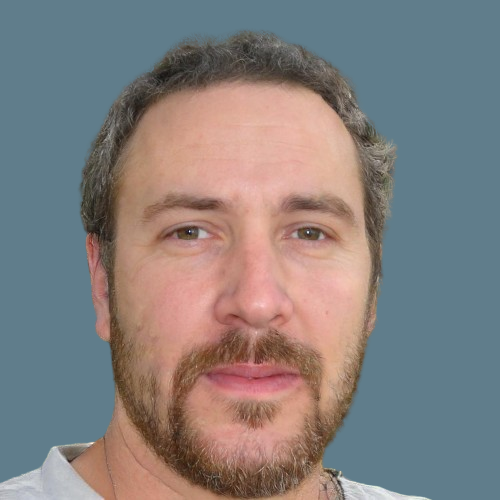
In the dynamic landscape of software development, ensuring the robustness and reliability of applications is paramount. As projects grow in complexity, maintaining high-quality code becomes increasingly challenging. This is where unit testing steps in, serving as a foundational practice to verify that individual components function as intended. Among the various testing frameworks available, JUnit stands out as the go-to choice for Java developers.
JUnit, an open-source testing framework, has been a cornerstone for software testing in Java for over two decades. It provides a structured way to write and run repeatable tests, enabling developers and testers to detect and fix bugs early in the development cycle. By isolating and testing individual units of code, JUnit helps ensure that each part of the application behaves correctly, fostering greater confidence in code changes and refactoring efforts.
This advanced guide to JUnit will delve deep into how to set up, write, and automate tests, offering insights into best practices and advanced techniques. Whether you’re a seasoned developer looking to refine your testing strategy or a newcomer eager to understand the intricacies of unit testing, this guide will equip you with the knowledge and tools to master JUnit. Join us as we explore how to leverage JUnit to enhance the quality, reliability, and maintainability of your Java applications.
What is JUnit?
JUnit is a unit testing framework for the Java programming language that is used to write and execute automated test scripts. It provides a structured way to write and run repeatable tests, enabling developers and testers to detect and fix bugs early in the development cycle.
JUnit testing is typically used for unit testing, although it may also be used to perform functional and integration tests. Functional tests evaluate the functioning of a system. They differ from unit tests in examining the system as a whole rather than individual components. Integration tests evaluate the integration between two or more systems. JUnit tests differ from unit tests in that they assess how well the elements of a system work together compared to separately.
JUnit has two major versions: JUnit 4 and JUnit 5. JUnit 4 is compatible with all JDKs released after 2006. JUnit 5 requires Java 8 or higher and is not backward compatible with older JDKs.
How Does JUnit Testing Work?
JUnit is a powerful framework that allows developers to test their Java applications effectively. By enabling tests to be written and executed within the Java platform, JUnit testing ensures that software behaves as intended and helps catch errors early in the development process. This early error detection aligns with the principle of fixing bugs before they become more complicated and costly to resolve.
The framework simplifies test creation with annotations like @Test
for marking test methods, @Before
and @After
for setting up and tearing down test environments, and assertions like assertEquals
and assertTrue
for validating expected outcomes. These features ensure that individual units of code function correctly, fostering confidence in code changes and refactoring efforts.
JUnit testing also supports different types of tests, including unit tests that focus on individual code snippets, integration tests that assess component interactions, and system tests that examine entire systems. Its test runners execute these tests and report results, while test suites allow grouping related tests for efficient execution. JUnit’s built-in reporter provides detailed insights into test execution, highlighting passed, failed, and skipped tests, which aids in quick and accurate debugging.
By integrating seamlessly with IDEs like Eclipse and IntelliJ IDEA and supporting command-line execution, JUnit testing enhances the efficiency of testing processes. Its capability to run multiple tests simultaneously is particularly beneficial for automation in continuous integration/continuous deployment (CI/CD) pipelines. Overall, JUnit testing’s structured approach helps developers maintain high-quality code, leading to robust and reliable Java applications.
JUnit Architecture
We will learn about the JUnit 5 architecture in this section of the JUnit guide. JUnit 5 is organized into multiple modules that are divided among three different sub-projects, each with a separate function.
JUnit Platform
The JUnit Platform serves as the foundation for running testing frameworks on the Java Virtual Machine (JVM). It provides a robust interface that integrates JUnit with users and various build tools, enabling easy discovery and execution of tests. A key feature of the platform is the TestEngine API, which allows developers to create custom TestEngines, integrating third-party testing libraries seamlessly into the JUnit environment.
JUnit Jupiter
The Jupiter module introduces new programming and extension models specifically designed for JUnit 5. It includes innovative annotations that enhance the test definition process compared to JUnit 4. Important annotations include:
@TestFactory: Marks a method as a factory for dynamic tests.
@DisplayName: Sets a custom display name for a test class or method.
@Nested: Denotes a nested, non-static test class.
@Tag: Allows tagging for test filtering.
@ExtendWith: Registers custom extensions.
@BeforeEach: Runs before each test method (replaces @Before).
@AfterEach: Runs after each test method (replaces @After).
@BeforeAll: Executes before all test methods in the class (replaces @BeforeClass).
@AfterAll: Executes after all test methods in the class (replaces @AfterClass).
@Disabled: Disables a test class or method (replaces @Ignore).
JUnit Vintage
JUnit Vintage ensures compatibility for running tests written with JUnit 3 and JUnit 4 within the JUnit 5 platform. This allows for smooth migration of projects using older versions of JUnit.
In summary, JUnit 5’s architecture is divided into the Platform, Jupiter, and Vintage modules. This structure provides developers with flexibility, compatibility, and enhanced features for testing Java applications.
How to Install the JUnit Testing Framework
To install JUnit in Eclipse IDE, follow these steps:
Step 1: Open Eclipse and start it with the latest version.
Step 2: Go to the “Help” menu and select “Install New Software…”.
Step 3: Click on the “Add” button and search for the JUnit repository at JUnit GitHub.
Step 4: Select JUnit from the list of choices and click “Next.”
Step 5: If you are using macOS, select the macOS target; otherwise, just hit “Next.” After selecting your target system, you will be guided through the installation process.
Step 6: Close the Installation Wizard window once JUnit is installed.
Step 7: Double-click on the JUnit icon located in the ‘Run’ dialog box.
Step 8: If you want to install a specific version of JUnit (e.g., JUnit 4), you need to find it manually. Click the ‘Install’ button and follow the prompts.
Step 9: After installation, close the Installation Wizard window.
Step 10: Double-click on the JUnit icon in the ‘Run’ dialog box and follow the instructions to start JUnit in Eclipse.
Once JUnit is started, you can run your test cases as expected.
Supported OS/Platforms: macOS 10.2 or later, Windows 7.0 or later, Linux 2.6 or later (including 64-bit Linux).
Annotations used in JUnit Testing
JUnit provides a range of annotations to simplify the process of writing and managing tests. These annotations help structure test cases, define test setup and teardown routines, and control test execution. Here’s a comprehensive look at the essential JUnit annotations:
@Test
The @Test annotation marks a method as a test case. It is the most fundamental annotation in JUnit, indicating that the method should be executed as a test.
@Test |
@BeforeEach
The @BeforeEach annotation is used to specify a method that runs before each test method. It is commonly used for setup operations that need to be performed before every test.
@BeforeEach |
@AfterEach
The @AfterEach annotation indicates that a method should run after each test method. It is typically used for cleanup operations.
@AfterEach |
@BeforeAll
The @BeforeAll annotation marks a method to be run once before all test methods in the class. The method must be static.
@BeforeAll |
@AfterAll
The @AfterAll annotation specifies a method that runs once after all test methods in the class. Like @BeforeAll, the method must be static.
@AfterAll |
@DisplayName
The @DisplayName annotation is used to provide a custom name for a test class or method, making test reports more readable.
@DisplayName(“Addition Test”) |
@Nested
The @Nested annotation allows for defining nested, non-static test classes. This is useful for grouping related tests together in an organized manner.
@Nested |
@Tag
The @Tag annotation allows you to tag test methods or classes, enabling filtering and categorization of tests.
@Tag(“fast”) |
@ExtendWith
The @ExtendWith annotation is used to register extensions for a test class or method. Extensions provide additional functionality, such as parameter injection or lifecycle callbacks.
@ExtendWith(MockitoExtension.class) |
@Disabled
The @Disabled annotation is used to disable a test class or method, preventing it from being executed.
@Disabled(“Test is under development”) |
@TestFactory
The @TestFactory annotation is used for dynamic tests, which are generated at runtime. These tests are useful for scenarios where the exact number of tests or their parameters are not known beforehand.
@TestFactory |
These annotations, individually and in combination, provide a powerful way to structure and manage tests in JUnit, making the testing process more efficient and maintainable.
How to perform automated JUnit Testing with Selenium?
JUnit and Selenium are a powerful combination for performing automated testing of web applications. Selenium provides tools for interacting with web elements, while JUnit manages the execution of tests and the reporting of results. Here, we’ll provide an example of how to write tests using JUnit and Selenium.
Prerequisites
Before you begin, ensure you have the following:
Java Development Kit (JDK) installed
Eclipse IDE or any other Java IDE installed
Selenium WebDriver and JUnit libraries added to your project
Step-by-Step Guide
- Set Up Your Project: Create a new Java project in your IDE and add the Selenium and JUnit dependencies to your project. You can add these dependencies via Maven by adding the following to your pom.xml:
<dependencies> |
- Write the JUnit Test Class: Create a new Java class for your test cases. In this example, we will create a test that verifies the title of a webpage.
import org.junit.jupiter.api.AfterEach; |
Explanation of the Code
Imports: Import the necessary JUnit and Selenium classes.
WebDriver Setup: In the setUp method, initialize the WebDriver. Here, we are using ChromeDriver, so you need to set the path to the ChromeDriver executable.
Test Method: The testGoogleTitle method opens the Google homepage and verifies that the page title is “Google”.
Teardown: The tearDown method closes the browser after each test to ensure a clean state for subsequent tests.
Conclusion
By now, you should have a solid grasp of JUnit’s fundamental concepts and its pivotal role in automated testing. This guide has highlighted JUnit’s key features, demonstrated the differences between its versions, and explained various annotations to streamline test creation.
Understanding JUnit’s capabilities and structure enables you to effectively write and manage your tests, ensuring your Java applications are robust and reliable.
To further enhance your testing strategy, consider integrating TestGrid with JUnit and Selenium. TestGrid offers a powerful cloud-based platform that scales your testing efforts, supports cross-browser testing, and accelerates test execution through parallel processing. By leveraging TestGrid’s infrastructure, you can effortlessly extend your testing coverage and improve the efficiency of your continuous integration/continuous deployment (CI/CD) pipeline.9999
Source: This article was originally published at https://testgrid.io/blog/junit-testing/
Subscribe to my newsletter
Read articles from Jace Reed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
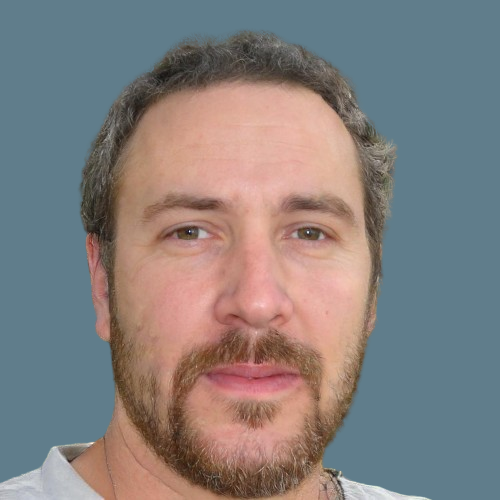
Jace Reed
Jace Reed
Senior Software Tester with 7+ years' experience. Expert in automation, API, and Agile. Boosted test coverage by 30%. Focused on delivering top-tier software.