Build a Password Manager with Python
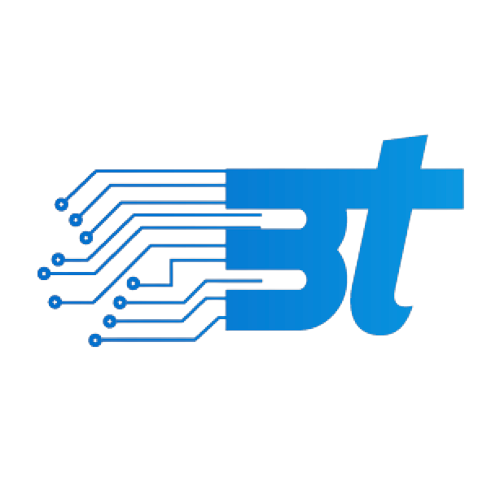
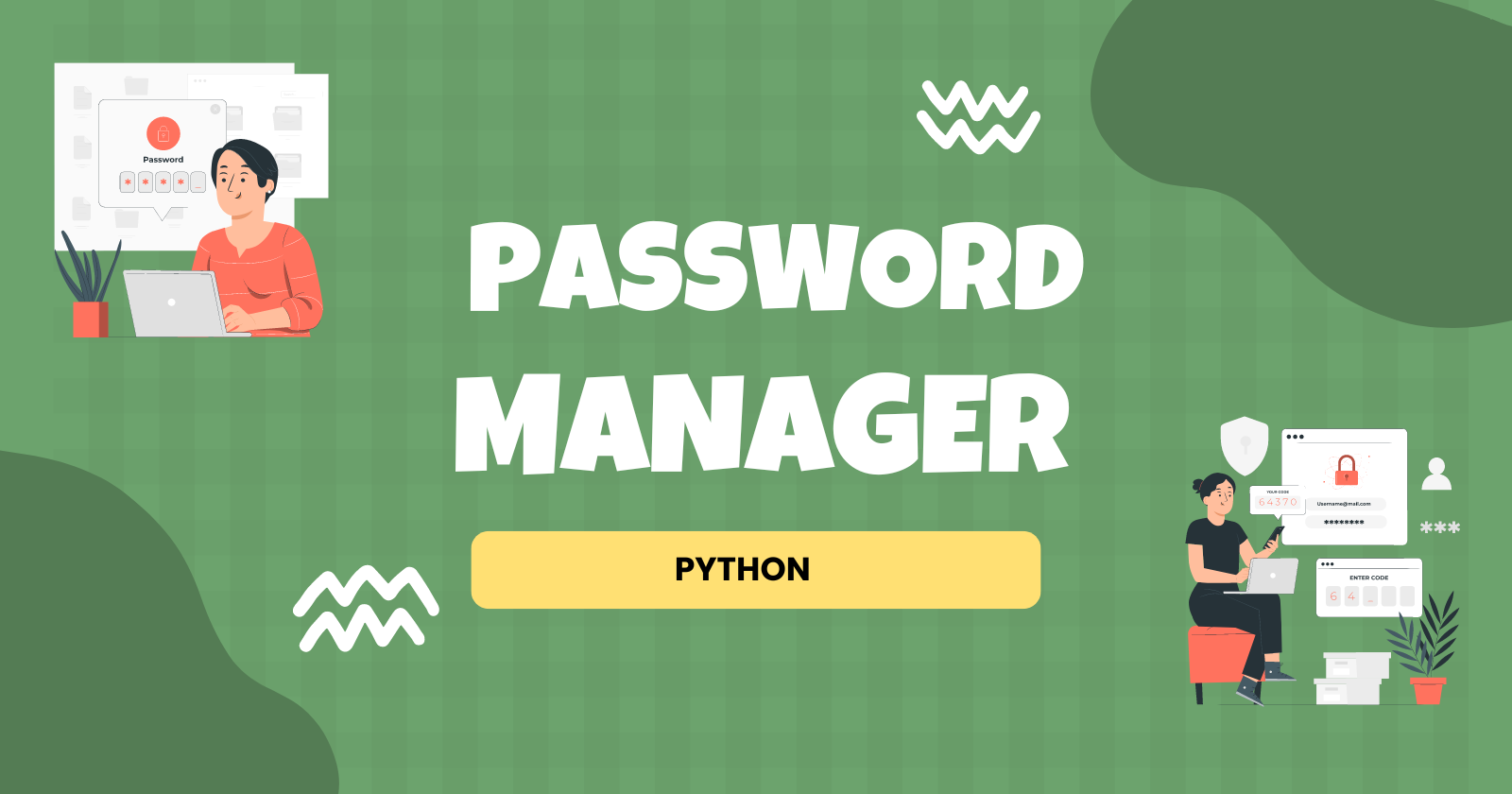
In today's digital world, managing multiple strong passwords is essential for protecting personal and professional data. A password manager helps you generate, store, and manage complex passwords securely. While there are many password managers available, building your own with Python offers a deeper understanding of data security and gives you full control over your personal information. In this guide, we'll create a simple yet effective password manager using Python.
Why Build Your Own Password Manager?
Customization: Tailor the manager to your specific needs and preferences.
Security: Avoid trusting third-party software with your sensitive data.
Learning: Gain valuable knowledge in Python programming and cybersecurity.
No Subscription Fees: Save money by avoiding subscription-based password managers.
Features of the Password Manager
Password Generation: Automatically generate strong passwords.
Secure Storage: Store passwords securely using encryption.
Password Retrieval: Retrieve passwords when needed.
User Authentication: Protect access to the password manager with a master password.
Cross-Platform: Run on any system that supports Python.
Getting Started
To build a password manager in Python, we'll use the following libraries:
cryptography
: For encryption and decryption of passwords.os
: To interact with the operating system.json
: For storing and managing data in a structured format.getpass
: To securely accept the master password from the user.
Step 1: Install Required Libraries
First, install the cryptography
library using pip:
pip install cryptography
Step 2: Write the Python Script
Here's the code for your password manager:
import json
import os
from getpass import getpass
from cryptography.fernet import Fernet
# File to store passwords
PASSWORD_FILE = 'passwords.json'
KEY_FILE = 'key.key'
def generate_key():
"""
Generate a new encryption key and save it to a file.
"""
key = Fernet.generate_key()
with open(KEY_FILE, 'wb') as key_file:
key_file.write(key)
def load_key():
"""
Load the encryption key from a file.
"""
return open(KEY_FILE, 'rb').read()
def encrypt_password(password, key):
"""
Encrypt a password using the provided key.
Parameters:
- password: The password to encrypt.
- key: The encryption key.
Returns:
- The encrypted password.
"""
f = Fernet(key)
return f.encrypt(password.encode())
def decrypt_password(encrypted_password, key):
"""
Decrypt a password using the provided key.
Parameters:
- encrypted_password: The encrypted password to decrypt.
- key: The encryption key.
Returns:
- The decrypted password.
"""
f = Fernet(key)
return f.decrypt(encrypted_password).decode()
def save_passwords(passwords):
"""
Save the password data to a JSON file.
Parameters:
- passwords: A dictionary containing account-password pairs.
"""
with open(PASSWORD_FILE, 'w') as file:
json.dump(passwords, file)
def load_passwords():
"""
Load the password data from a JSON file.
Returns:
- A dictionary containing account-password pairs.
"""
if os.path.exists(PASSWORD_FILE):
with open(PASSWORD_FILE, 'r') as file:
return json.load(file)
else:
return {}
def add_password(account, password, key):
"""
Add a new account and password to the password manager.
Parameters:
- account: The account name (e.g., website or app name).
- password: The password to store.
- key: The encryption key.
"""
passwords = load_passwords()
encrypted_password = encrypt_password(password, key)
passwords[account] = encrypted_password.decode()
save_passwords(passwords)
print(f"Password for {account} added successfully.")
def retrieve_password(account, key):
"""
Retrieve the password for a specific account.
Parameters:
- account: The account name to retrieve the password for.
- key: The encryption key.
Returns:
- The decrypted password, if found.
"""
passwords = load_passwords()
if account in passwords:
encrypted_password = passwords[account].encode()
return decrypt_password(encrypted_password, key)
else:
print(f"No password found for account: {account}")
return None
def main():
if not os.path.exists(KEY_FILE):
print("Encryption key not found. Generating a new key...")
generate_key()
key = load_key()
while True:
print("\n--- Password Manager ---")
print("1. Add a new password")
print("2. Retrieve a password")
print("3. Quit")
choice = input("Enter your choice: ")
if choice == '1':
account = input("Enter the account name (e.g., website or app name): ")
password = getpass("Enter the password: ")
add_password(account, password, key)
elif choice == '2':
account = input("Enter the account name: ")
password = retrieve_password(account, key)
if password:
print(f"Password for {account}: {password}")
elif choice == '3':
print("Exiting the Password Manager.")
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
How the Script Works
Encryption Key Management:
Generate Key: The
generate_key()
function creates a new encryption key and saves it to a file (key.key
). This key is essential for encrypting and decrypting passwords.Load Key: The
load_key()
function reads the encryption key from the file.
Password Encryption and Decryption:
Encrypt Password: The
encrypt_password()
function encrypts a password using the encryption key and returns the encrypted password.Decrypt Password: The
decrypt_password()
function decrypts an encrypted password using the encryption key and returns the decrypted password.
Password Storage:
Save Passwords: The
save_passwords()
function writes the account-password pairs to a JSON file (passwords.json
).Load Passwords: The
load_passwords()
function reads the account-password pairs from the JSON file.
Adding and Retrieving Passwords:
Add Password: The
add_password()
function adds a new account and encrypted password to the password manager.Retrieve Password: The
retrieve_password()
function retrieves and decrypts the password for a specific account.
User Interface:
- The script provides a simple command-line interface for users to add new passwords, retrieve existing ones, or exit the program.
Enhancing the Password Manager
To make your password manager even more robust and user-friendly, consider implementing the following enhancements:
Master Password Protection: Protect access to the password manager itself with a master password that is verified before any operation.
Password Generation: Integrate a password generator to create strong, random passwords.
Advanced Encryption: Use more sophisticated encryption methods, like AES with a key derivation function, for additional security.
Secure Storage: Store encrypted passwords in a more secure database like SQLite or even an encrypted file system.
Cross-Platform GUI: Develop a graphical user interface (GUI) using
tkinter
orPyQt
for better usability.Password Health Check: Add functionality to check the strength of existing passwords and recommend changes.
Backup and Sync: Implement features to back up and synchronize passwords across devices securely.
Running the Password Manager
Initial Setup:
Run the script for the first time. If the encryption key does not exist, it will generate one.
Follow the prompts to add and retrieve passwords.
Secure Your Data:
Ensure the encryption key and password file are stored securely.
Consider storing the key on a USB drive or using environment variables for added security.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
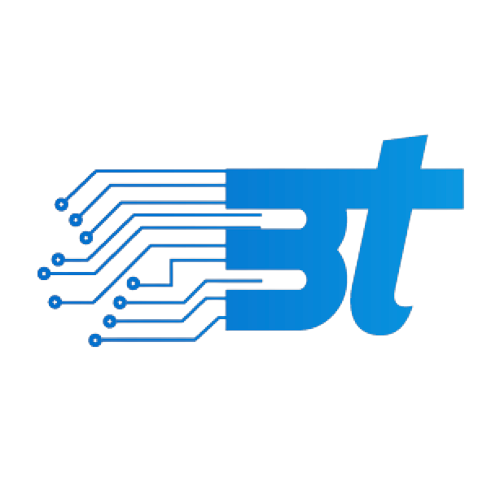
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.