Database Modeling in Django (Models)
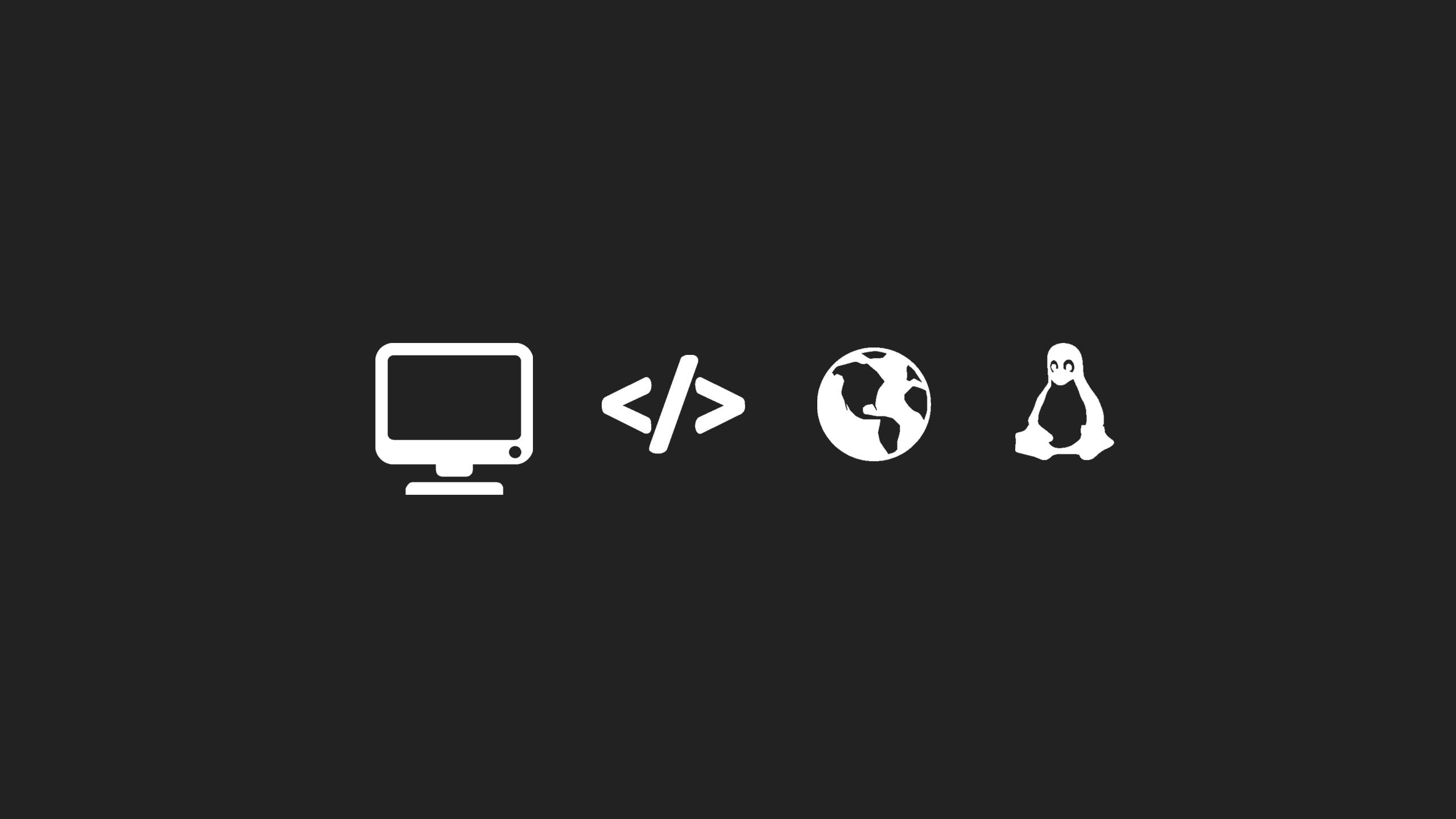
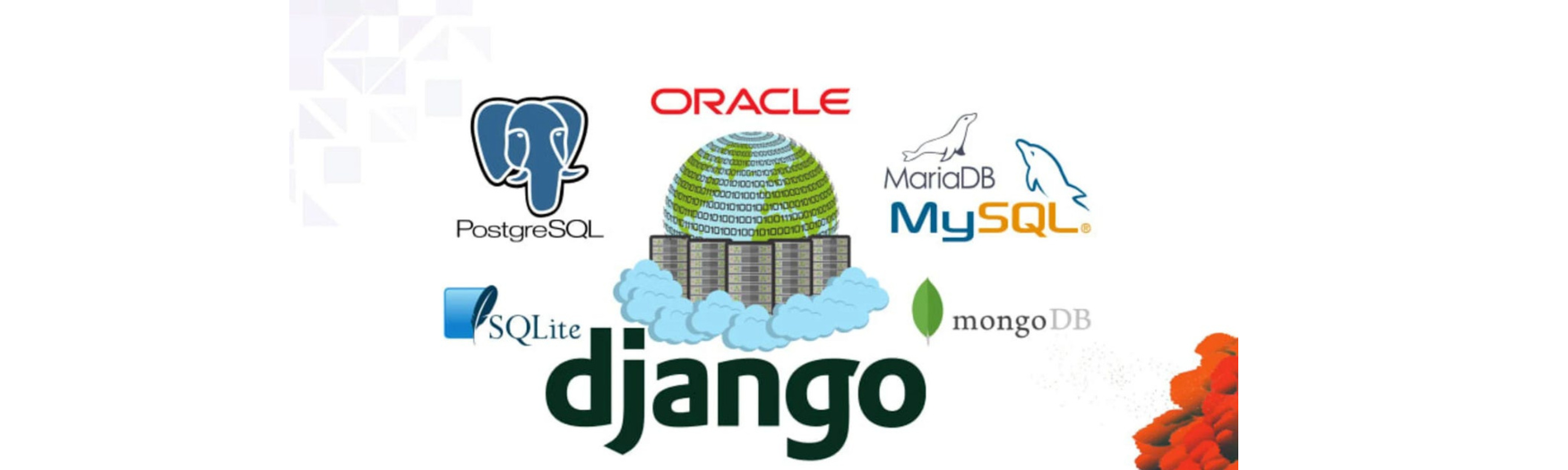
Before delving into the concept of Database Modeling in Django we must know the basic of MTV architecture that is followed by Django , MTV stands for Model , Template & View where Templates are the skeletal structure of your basic Web page format and View is the rendering program that will accept the Request from User via Browser and Map/Parse the requested URL / Page in the form of HTTP Response.
The term "Model" will be our main point of focus in the current blog , Model is basically the definition of Fields and Behaviors of the data we are storing.
What a Model Can DO ?
It creates Tables and Define the Relationship between them
Helps to Modify Tables and can change the Relationship between them
Can access data stored in tables using Django ORM (Object Relational Mapping) without writing raw SQL
Configuring Database :
Before creating Models we must configure our desired Database with Django , We can do this by simple steps :
1.) Open Settings.py file that is available in sitewide django_project , scroll and find the "DATABASE" variable
2.) The "ENGINE" will specify the type of database backend Django is using ,( By default Django Use SQLite DB ).
Data Representation :
Before we code Models , lets analyze how the data we need to store in our Database , Suppose we are creating a Blog App , we would need to store some information about Following Entities
NOTE : Tables in DB are termed as Entities & Attributes of the Entities are termed as Fields
Ex: Post , Author , Category , Tag , these are the entities and lets say they have attributes as :-
Post
title
slug
content
publication date
Author
name
email
active
account creation date
Last login date
Category
name
slug
Tag
name
slug
The 4 Entities are Post , Author , Category & Tag. In context of Database , each of these entities corresponds to a table and each attribute corresponds to a column.
By default, Django Framework adds a primary key field called id or pk. The key automatically increments every time a new record is added.
Relationship b/w Entities :
A relationship can exist between entities, A single entity can related to single , many . similarly many entities can be related to many.
like a post may belong to any one author but an author can write may blog posts , so One-to-many relationship exists between post and author
A post may be belonging to one category but a category can have multiple posts , so again One-to-Many Relationship
We can solve the issues by creating / adding a Foreign Key on the many side of the Relationship
Creating Models :
In order to create a model , open models.py file inside the blog application that you have created .
A Model is simply a python class which inherits from models.model class ,
from django.db import models
class Author(models.Model):
pass
syntactically Author is a valid Model , although it doesn't have any attributes or fields , We can add the fields via the following methods :
from django.db import models
class Author(models.Model):
name = models.CharField(max_length = 100 , unique = True)
If you have some basic knowledge of SQL raw commands which allows us to create Database and tables like for the above Author Table we want to create an attribute called 'name' we would be using the command :
CREATE TABLE Author(name Varchar(10));
INSERT INTO Author(name)
VALUES ( "Surya" );
The above SQL Command creates a Table called Author with an attribute called name i.e Author's Name , but Django gives us the Freedom to write this without implementing it raw using SQL
WHY ?
This serves two important purposes :
Validate data before saving into the database
They also control how to display the data in the form
Getting over head right ? Do not worry , at first the concepts seems cloudy but as we delve deeper into the topics we can understand how these are related to each other & their applications :)
Subscribe to my newsletter
Read articles from Surya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
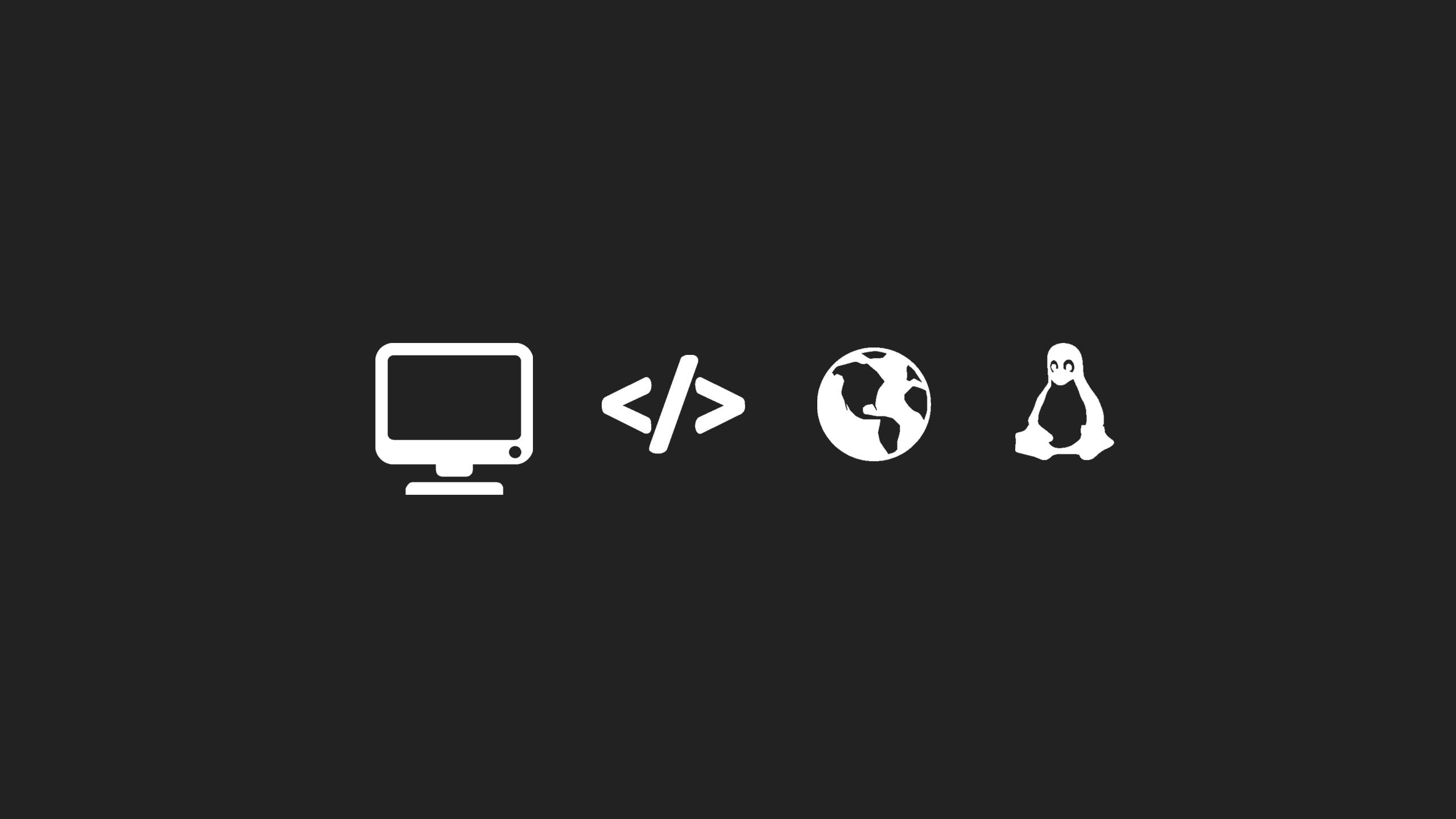