2 . PixelPlotter: AI Tool for Crafting Stories and Game Plots

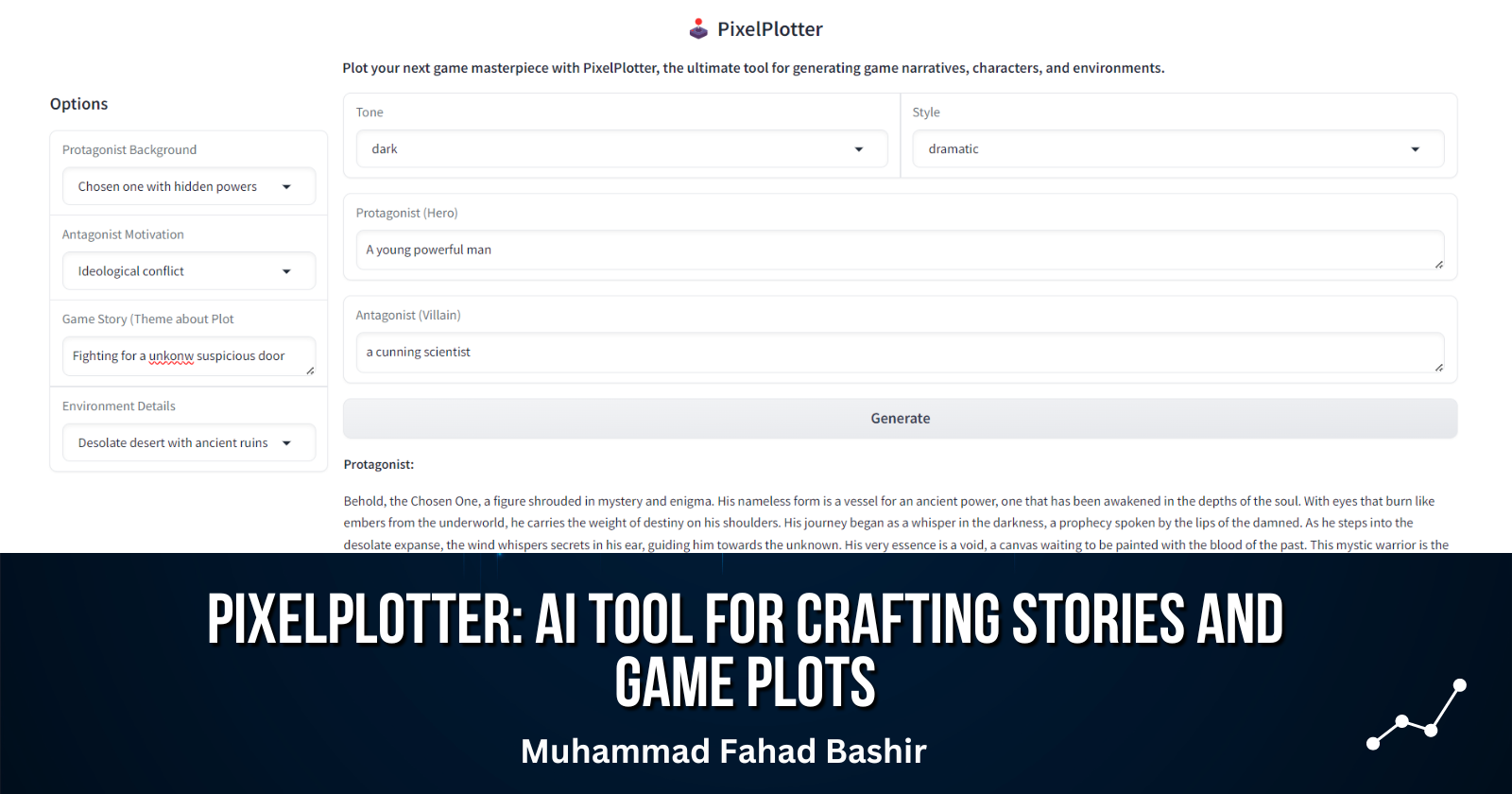
PixelPlotter is an innovative AI-powered tool designed for game developers, storytellers, and creative minds. Whether you're developing the next big indie game or crafting an epic narrative for your gaming universe, PixelPlotter is here to simplify the creative process.
his application leverages the power of advanced language models to generate detailed game content, including characters, environments, and story plots. With PixelPlotter, you can transform your ideas into fully fleshed-out game narratives in just a few clicks.
Story Behind PixelPlotter
Imagine you're a game developer with an incredible concept but struggling to bring your characters, environments, and storylines to life. That’s where PixelPlotter comes in. PixelPlotter allows you to focus on what you do best—innovating and designing—while leaving the heavy lifting of content generation to advanced AI.
PixelPlotter was created to bridge the gap between imagination and execution, making it easier than ever to produce compelling game content.
Key Features of PixelPlotter
Character and Environment Customization 🎮
Generate detailed descriptions for protagonists, antagonists, and environments.
Customize the tone, style, and background to match your game’s theme.
Dynamic Story Plot Generation 📜
Automatically generate engaging story plots based on your input.
Perfect for creating unique game narratives that resonate with your target audience.
Flexible Input Options 🎨
Choose from a variety of backgrounds, motivations, and environments, or input your own custom details.
The system supports different tones and styles, allowing you to craft content that’s dark, humorous, epic, or light-hearted.
User-Friendly Interface 🖱️
Intuitive and easy to use, even for those with minimal technical experience.
Integrated with Gradio, making the application accessible directly from your browser.
Instant Feedback ⏱️
- Real-time content generation allows you to see your ideas come to life immediately.
Example Use Case
Let’s say you’re designing a fantasy RPG game. You want a dark, epic storyline with a hardened warrior protagonist and a power-hungry antagonist. The environment is a stormy mountain range filled with hidden dangers. With PixelPlotter, you can input these details, select the desired tone and style, and click “Generate.” In moments, you’ll receive a rich, detailed description of your characters and environment, along with a plot that ties everything together—ready to be incorporated into your game.
Building and Deploying PixelPlotter
Creating PixelPlotter was an exciting journey, from idea to deployment. The application was built using the LLaMA model from Groq for content generation, Gradio for the user interface, and Google Colab for testing and development. Finally, I deployed the tool on Hugging Face Spaces for easy access.
Tools Used:
LLaMA (Groq): Powered the AI-driven content generation.
Gradio: Provided an interactive user interface.
Google Colab: Facilitated development and testing.
Hugging Face Spaces: Enabled quick and easy deployment.
Development Process
1. Initial Testing: I started by testing the core idea-generating game content using AI—in Google Colab.
2. Iteration and Refinement: As I developed the tool, I encountered challenges, such as formatting the output correctly. After multiple iterations and adjustments, I managed to customize the output to enhance readability and usability.
3. Final Deployment: Once the tool was polished, I deployed PixelPlotter on Hugging Face Spaces, making it available for users to create their own game content.
I am sharing my initial output and final output
Initial output
Final output
Try It Yourself! 🛠️
Experience the power of PixelPlotter firsthand with our Hugging Face Space link.
https://huggingface.co/spaces/mfahadkhan/PixelPlotter
Load the application, input your custom details, and watch as PixelPlotter generates the game content you’ve been dreaming of.
Code
import os
from groq import Groq
import gradio as gr
# Set your API key
os.environ['GROQ_API_KEY'] = "YOUR API KEY HERE"
# Initialize the Groq client
client = Groq(
api_key=os.environ.get("GROQ_API_KEY"),
)
def generate_game_content(protagonist, antagonist, environment, tone, style, protagonist_background, antagonist_motivation, environment_detail):
# Create a prompt that includes character details and environment details
messages = [
{
"role": "user",
"content": f"Create detailed descriptions for the following game elements in a {tone}, {style} style:\n\n\
**Protagonist**: {protagonist} (Background: {protagonist_background})\n\
**Antagonist**: {antagonist} (Motivation: {antagonist_motivation})\n\
**Environment**: {environment} (Details: {environment_detail})\n\n\
Also, provide a brief plot for the game."
}
]
try:
chat_completion = client.chat.completions.create(
messages=messages,
model="llama3-8b-8192",
)
response = chat_completion.choices[0].message.content
protagonist_desc = "Protagonist Description:\n" + response.split("Antagonist Description:")[0].strip() if "Antagonist Description:" in response else response
antagonist_desc = "Antagonist Description:\n" + response.split("Antagonist Description:")[1].split("Environment Description:")[0].strip() if "Environment Description:" in response else ""
environment_desc = "Environment Description:\n" + response.split("Environment Description:")[1].split("Story Plot:")[0].strip() if "Story Plot:" in response else ""
story_plot = "Story Plot:\n" + response.split("Story Plot:")[1].strip() if "Story Plot:" in response else ""
except Exception as e:
protagonist_desc = "An error occurred while generating the content."
antagonist_desc = ""
environment_desc = ""
story_plot = ""
return protagonist_desc, antagonist_desc, environment_desc, story_plot
# Gradio interface
def gradio_interface():
with gr.Blocks() as demo:
with gr.Row():
gr.Markdown("<h2 style='text-align: center;'><img src='/content/image.jpg' width='200' height='100'> PixelPlotter</h2>")
with gr.Row():
gr.Markdown("<h2 style='text-align: center;'>🕹️ PixelPlotter</h2>")
with gr.Row():
gr.Markdown("<h4 style='text-align: center;'> Plot your next game masterpiece with PixelPlotter, the ultimate tool for generating game narratives, characters, and environments.</h4>")
with gr.Row():
with gr.Column(scale=1, min_width=150):
gr.Markdown("<h3 style='font-size: 18px; font-weight: bold;'>Options</h3>")
protagonist_background = gr.Dropdown(label="Protagonist Background", choices=["Orphaned hero", "Reluctant leader", "Chosen one with hidden powers", "Hardened warrior","Random"], value="Orphaned hero")
antagonist_motivation = gr.Dropdown(label="Antagonist Motivation", choices=["Revenge", "Thirst for power", "Ideological conflict", "Desire for chaos","Random"], value="Revenge")
environment = gr.Textbox(label="Game Environment")
environment_detail = gr.Dropdown(label="Environment Details", choices=["Stormy mountains", "Lush forests with hidden dangers", "Desolate desert with ancient ruins", "Frozen tundra with mystical creatures","random environment"], value="Stormy mountains")
with gr.Column(scale=4):
with gr.Row():
tone = gr.Dropdown(label="Tone", choices=["dark", "humorous", "epic", "light-hearted","Random"], value="epic")
style = gr.Dropdown(label="Style", choices=["formal", "casual", "dramatic", "whimsical","Random"], value="formal")
with gr.Row():
protagonist = gr.Textbox(label="Protagonist")
with gr.Row():
antagonist = gr.Textbox(label="Antagonist")
generate_button = gr.Button("Generate", elem_id="generate-button")
protagonist_output = gr.Markdown()
antagonist_output = gr.Markdown()
environment_output = gr.Markdown()
story_plot_output = gr.Markdown()
generate_button.click(
fn=generate_game_content,
inputs=[protagonist, antagonist, environment, tone, style, protagonist_background, antagonist_motivation, environment_detail],
outputs=[protagonist_output, antagonist_output, environment_output, story_plot_output]
)
# Add inline CSS to style elements
gr.Markdown("""
<style>
#generate-button {
background-color: #ff7f00;
color: white;
border-radius: 5px;
padding: 10px;
font-size: 16px;
}
</style>
""")
demo.launch()
gradio_interface()
My learning
Iterate and Improve: Each round of testing brings you closer to the final product.
Problem-Solving: Challenges are inevitable, but persistence leads to better solutions.
User Experience: Always focus on making the tool user-friendly and the output clear.
From concept to deployment, building PixelPlotter was a rewarding experience, and I’m eager to see how others will use it to craft unique game narratives.
Subscribe to my newsletter
Read articles from Muhammad Fahad Bashir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
