Basics things I know about DOM manupulation

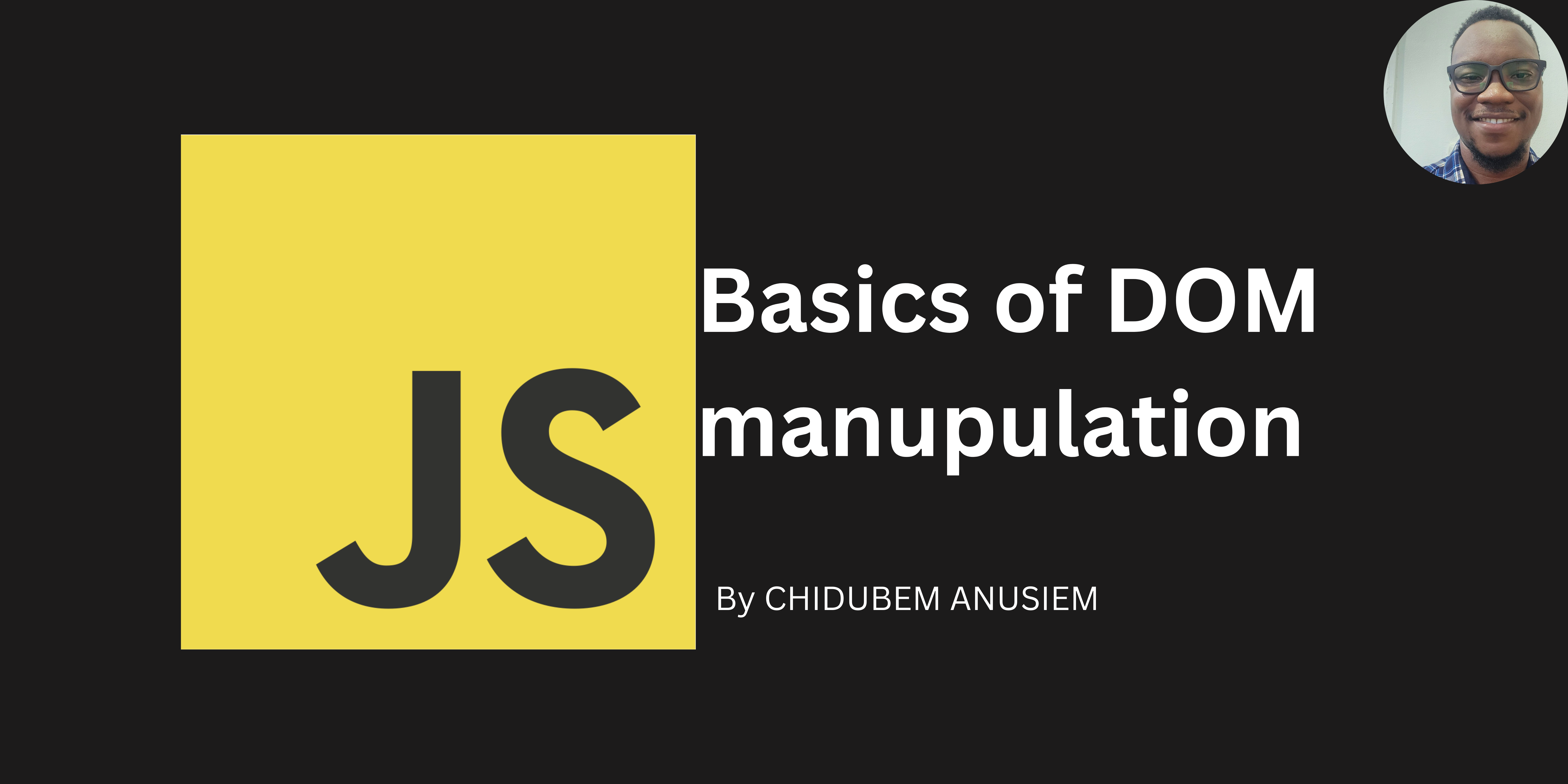
What is the DOM?
The DOM, or Document Object Model, is essentially the bridge between your HTML and JavaScript. Think of it as a tree-like structure that represents your web page. Each element in your HTML—like headings, paragraphs, buttons, and images—becomes a node in this tree. And with JavaScript, you can interact with these nodes to make your website dynamic and interactive.
Why is DOM Manipulation Important?
Imagine your website is a house, and the DOM is the blueprint. With DOM manipulation, you’re not just stuck with the original blueprint—you can add rooms, change paint colors, or even knock down walls without rebuilding the whole house! This means you can update content, change styles, and react to user interactions on the fly.
Basics of DOM Manipulation:
Selecting Elements:
Get Elements by ID:
To select a single element, use
document.getElementById('elementId')
.Example:
let header = document.getElementById('main-header');
Get Elements by Class Name:
To select multiple elements with the same class, use
document.getElementsByClassName('className')
.Example:
let buttons = document.getElementsByClassName('btn');
Query Selector:
For more flexibility, use
document.querySelector('.className')
for a single element ordocument.querySelectorAll('.className')
for multiple elements.Example:
let firstButton = document.querySelector('.btn');
Changing Content:
InnerHTML:
Modify the content inside an element with
element.innerHTML
.Example:
header.innerHTML = 'Welcome to My Website!';
Text Content:
For just the text without any HTML tags, use
element.textContent
.Example:
header.textContent = 'Hello World!';
Modifying Styles:
Inline Styles:
Change the style directly using
element.style
.Example:
header.style
.color = 'blue';
Class List:
Add, remove, or toggle CSS classes using
element.classList
.Example:
header.classList.add('highlight');
Creating and Adding Elements:
Create Element:
Dynamically create new elements with
document.createElement('tagName')
.Example:
let newDiv = document.createElement('div');
Append Child:
Add your new element to the DOM using
parentElement.appendChild(newElement)
.Example:
document.body.appendChild(newDiv);
Handling Events:
Add Event Listener:
Make your page interactive by responding to user actions using
element.addEventListener('event', function)
.Example:
let button = document.querySelector('.btn'); button.addEventListener('click', () => { alert('Button was clicked!'); });
Why Should You Care?
DOM manipulation is at the heart of creating interactive websites. Whether you're building a simple to-do list, a dynamic form, or a complex web app, knowing how to manipulate the DOM allows you to bring your ideas to life and enhance the user experience.
Subscribe to my newsletter
Read articles from Anusiem Chidubem directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
