Mastering Python List Comprehensions
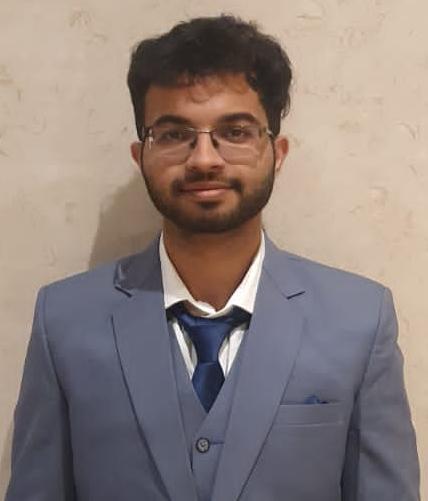
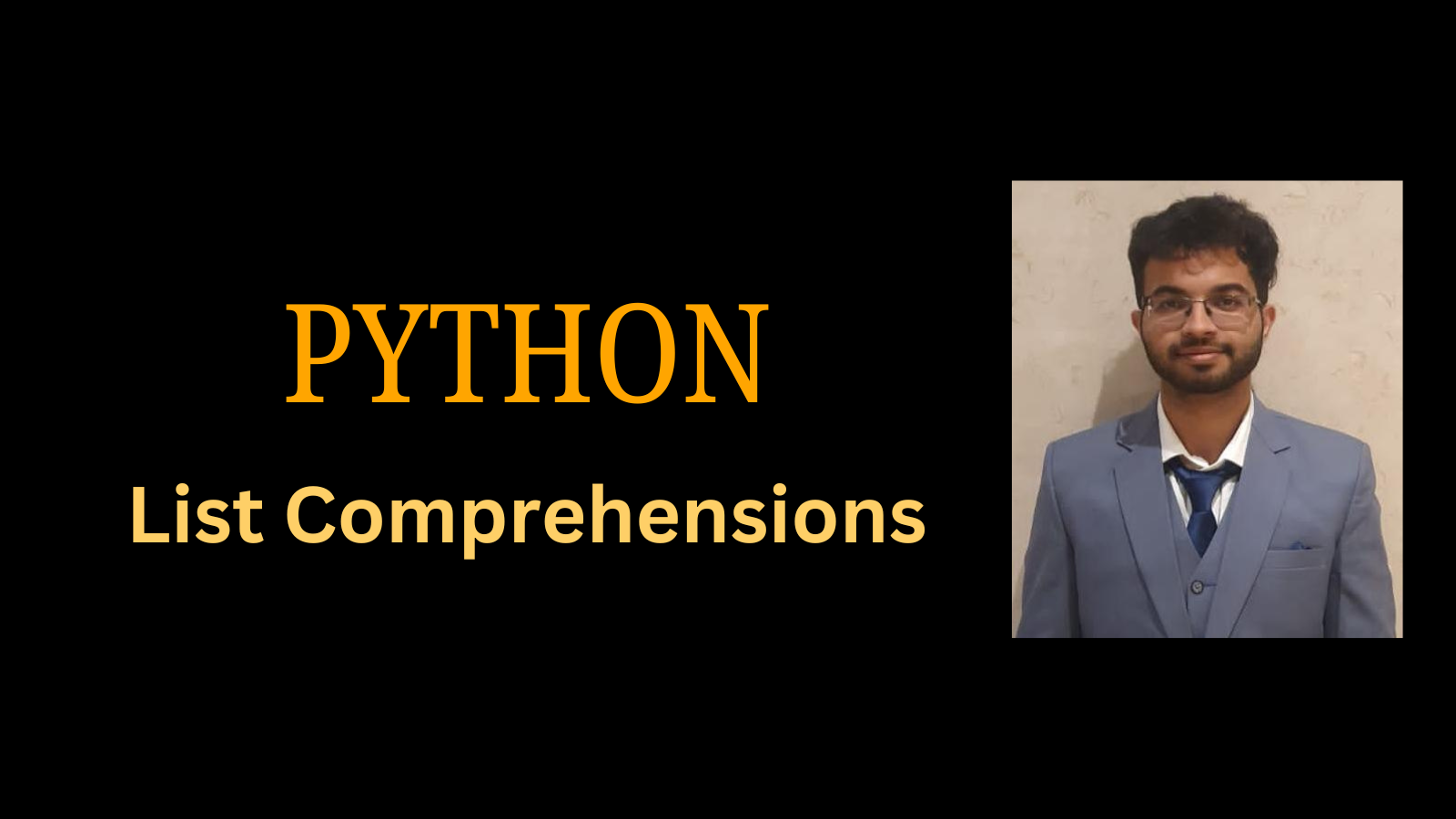
Python is renowned for its simplicity and readability, and one of the features that make it so elegant is List Comprehensions. Whether youβre a beginner or a seasoned developer, mastering this powerful feature can significantly enhance your coding efficiency and readability.
What Are List Comprehensions? π€
List Comprehensions offer a concise way to create lists in Python. They allow you to generate a new list by applying an expression to each item in an existing iterable (like a list or range) and, optionally, filter items based on a condition.
The Basic Syntax π
pythonCopy codenew_list = [expression for item in iterable if condition]
expression: The operation or transformation to apply to each
item
.item: The current element from the
iterable
(e.g., list, range).iterable: The collection youβre iterating over.
condition (optional): A filter that determines if an
item
should be included.
Examples in Action π―
Basic List Transformation: Convert a list of numbers to their squares.
pythonCopy codenumbers = [1, 2, 3, 4, 5] squares = [x**2 for x in numbers] print(squares) # Output: [1, 4, 9, 16, 25]
Filtering with Conditions: Create a list of even numbers from a range.
pythonCopy codeeven_numbers = [x for x in range(10) if x % 2 == 0] print(even_numbers) # Output: [0, 2, 4, 6, 8]
Nested List Comprehensions: Flatten a 2D list into a 1D list.
pythonCopy codematrix = [[1, 2], [3, 4], [5, 6]] flattened = [num for row in matrix for num in row] print(flattened) # Output: [1, 2, 3, 4, 5, 6]
Applying Functions: Convert a list of strings to their uppercase form.
pythonCopy codefruits = ["apple", "banana", "cherry"] upper_fruits = [fruit.upper() for fruit in fruits] print(upper_fruits) # Output: ['APPLE', 'BANANA', 'CHERRY']
Benefits of Using List Comprehensions π‘
Conciseness: Write more in fewer lines.
Readability: A well-written list comprehension can be easier to understand than loops.
Performance: Often faster than traditional for-loops due to optimization in Python.
How to Learn List Comprehensions Easily π§
Start Simple: Begin by converting basic for-loops to list comprehensions.
Experiment: Practice with different data types and use cases.
Understand the Flow: Remember, the order is
[expression for item in iterable if condition]
.Read Pythonic Code: Examine how seasoned developers use list comprehensions in real projects.
Practice, Practice, Practice: The more you use them, the more intuitive they become.
Conclusion
That's it for now. Did you like this blog? Please let me know.
You can Buy Me a Coffee if you want to and please don't forget to follow me on Youtube, Twitter, and LinkedIn also.
Subscribe to my newsletter
Read articles from Saarthak Maini directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
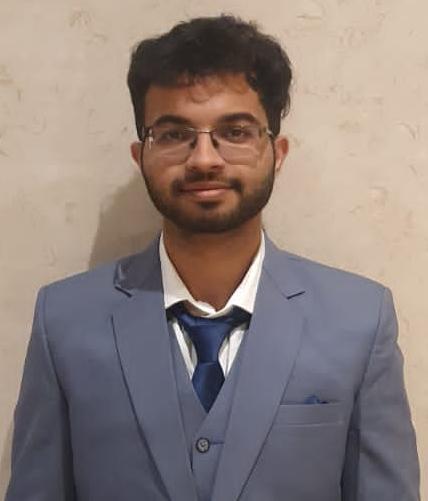