Packet Sniffing in Action: A Hands-On Guide Using Python and Scapy
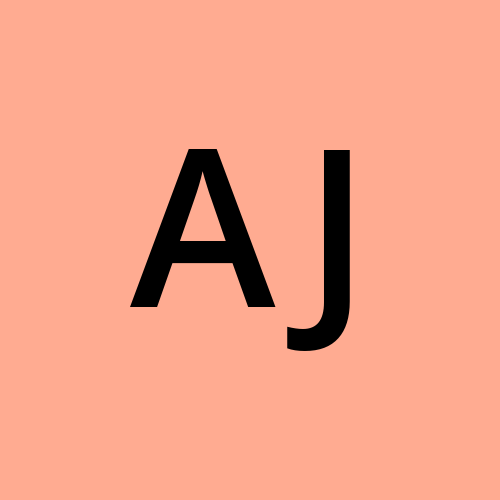
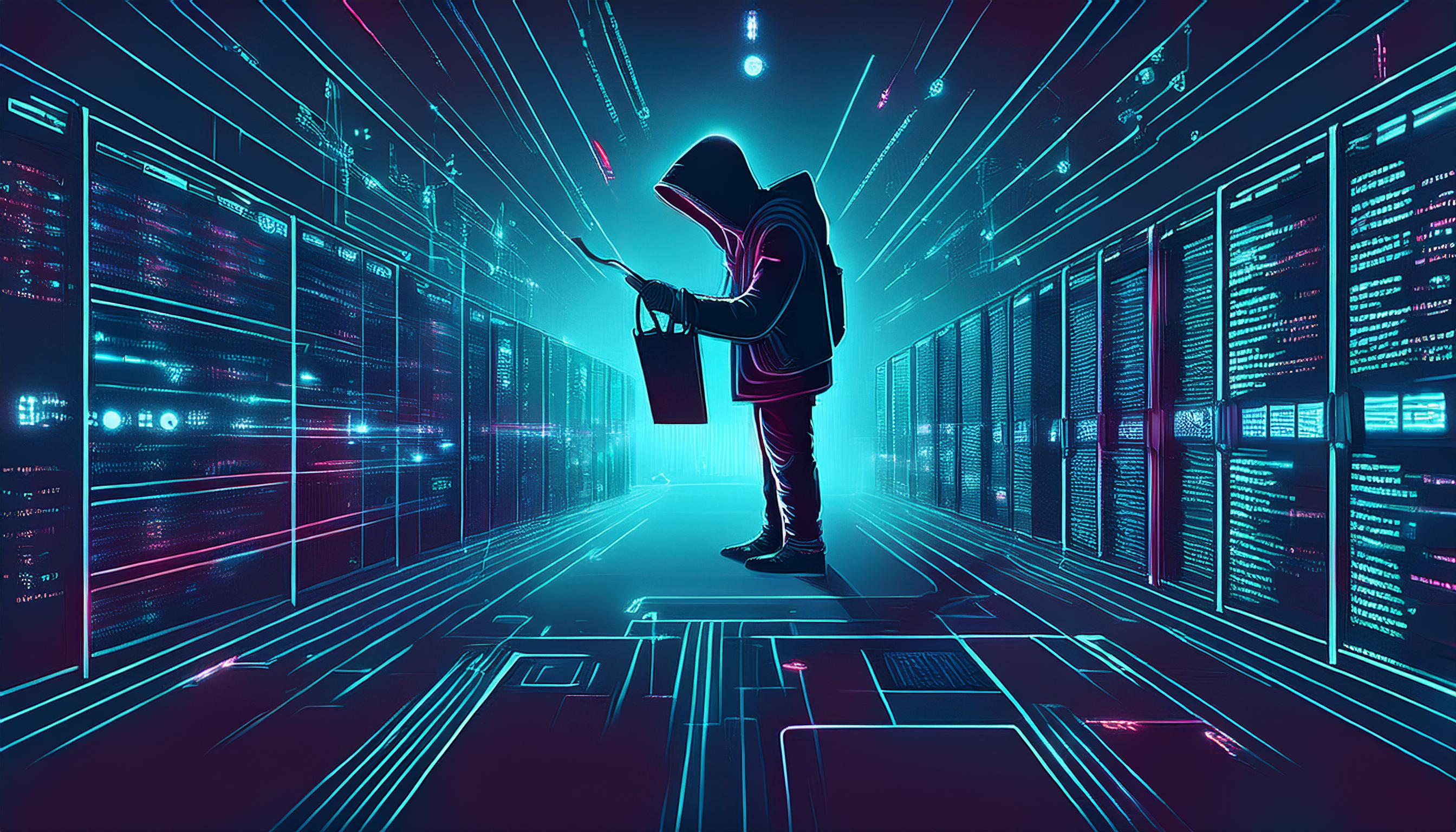
Imagine you’re curious about the data flowing through your network—what’s being sent, where it’s going, and who’s communicating with who?. Packet sniffing is a powerful technique that can help you answer all these questions. In this blog, we’ll dive deeper into packet sniffing, but this time with a hands-on approach. We’ll walk through the steps of using Python and the Scapy library to sniff network packets.
Why Packet Sniffing?
Packet sniffing is an essential skill for network administrators and cybersecurity professionals. It allows you to capture and analyze data packets traveling through a network, providing valuable insights into network performance, security vulnerabilities, and potential threats. However, it’s important to remember that while packet sniffing can be used for legitimate purposes, it can also be exploited for malicious activities. Ethical use and legal considerations are crucial.
Getting Started: Tools You’ll Need
For this tutorial, you’ll need:
Python installed on your system.
Scapy, a powerful Python library for network packet manipulation.
Access to two network hosts (we’ll will be referring them as hostA and hostB).
Step 1: Setting Up Your Sniffer
We’ll start by writing a simple Python program named sniffer.py
using the Scapy library. This program will capture and display 10 network packets that match specific criteria, such as ICMP (ping) or UDP packets.
Here’s the code:
#!/usr/bin/python3
from scapy.all import *
# Sniff 10 packets from the network interface 'enp0s3'
# Filter for ICMP (ping) or UDP packets
pkt = sniff(iface='enp0s3', filter='icmp or udp', count=10)
# Display a summary of the captured packets
pkt.summary()
In this code:
sniff()
is the function used to capture packets.iface='enp0s3'
specifies the network interface to sniff on.filter='icmp or udp'
filters packets to only capture ICMP (often used for pings) and UDP packets.count=10
tells Scapy to stop sniffing after capturing 10 packets.pkt.summary()
provides a summary of the captured packets.
Step 2: Running the Sniffer
Once your sniffer.py
script is ready, it’s time to run it on hostA with root privileges. This will allow the program to capture packets that require higher-level permissions.
sudo python3 sniffer.py
As the sniffer runs on hostA, open another terminal to access hostB and send some packets over the network. For example, you can use the ping
command to generate ICMP packets:
ping -c 15 10.55.0.5
This command will send 15 ping requests from hostB to hostA.
Step 3: Analyzing the Output
With the sniffer running on hostA, you should see the captured packets displayed in the terminal. The pkt.summary()
function will give you a brief overview of each packet, showing essential information such as the source and destination IP addresses, protocol type, and more.
Now, take a look at both terminals:
hostA (Sniffer Terminal): You should see a summary of the captured ICMP or UDP packets.
hostB (Ping Terminal): This terminal will display the ping statistics, showing the number of packets sent, received, and any packet loss.
Understanding the Results
You might notice that the number of packets captured by the sniffer on hostA doesn’t exactly match the number of packets sent by hostB. This discrepancy can occur due to several factors, such as packet loss, network congestion, or differences in how each host handles network traffic.
Conclusion
Packet sniffing is a fascinating and practical way to explore the data flowing through your network. With Python and Scapy, you have the tools to capture, analyze, and understand these packets in detail. Whether you’re troubleshooting a network issue or learning more about cybersecurity, packet sniffing provides valuable insights.
Remember, while packet sniffing is a powerful technique, it comes with ethical responsibilities. Always ensure you have permission to sniff a network and use your skills for good.
Happy Sniffing!
Subscribe to my newsletter
Read articles from Akash Reddy Jammula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
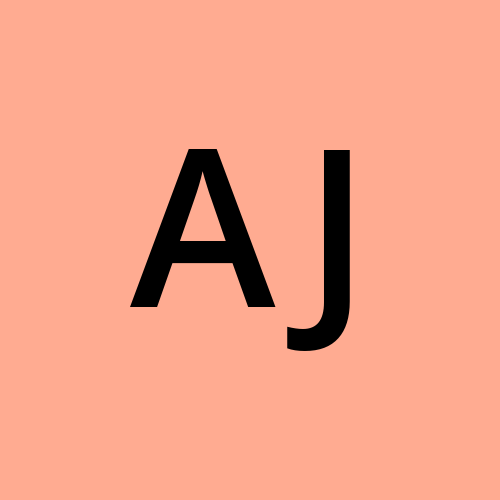
Akash Reddy Jammula
Akash Reddy Jammula
Hello 👋🏻 , I am a curiosity-driven software engineer who likes to write. I have a passion for discovering and working with cutting-edge technology.