Leveraging AI for Climate Change: Analyzing Climate Data with Spatiotemporal Models

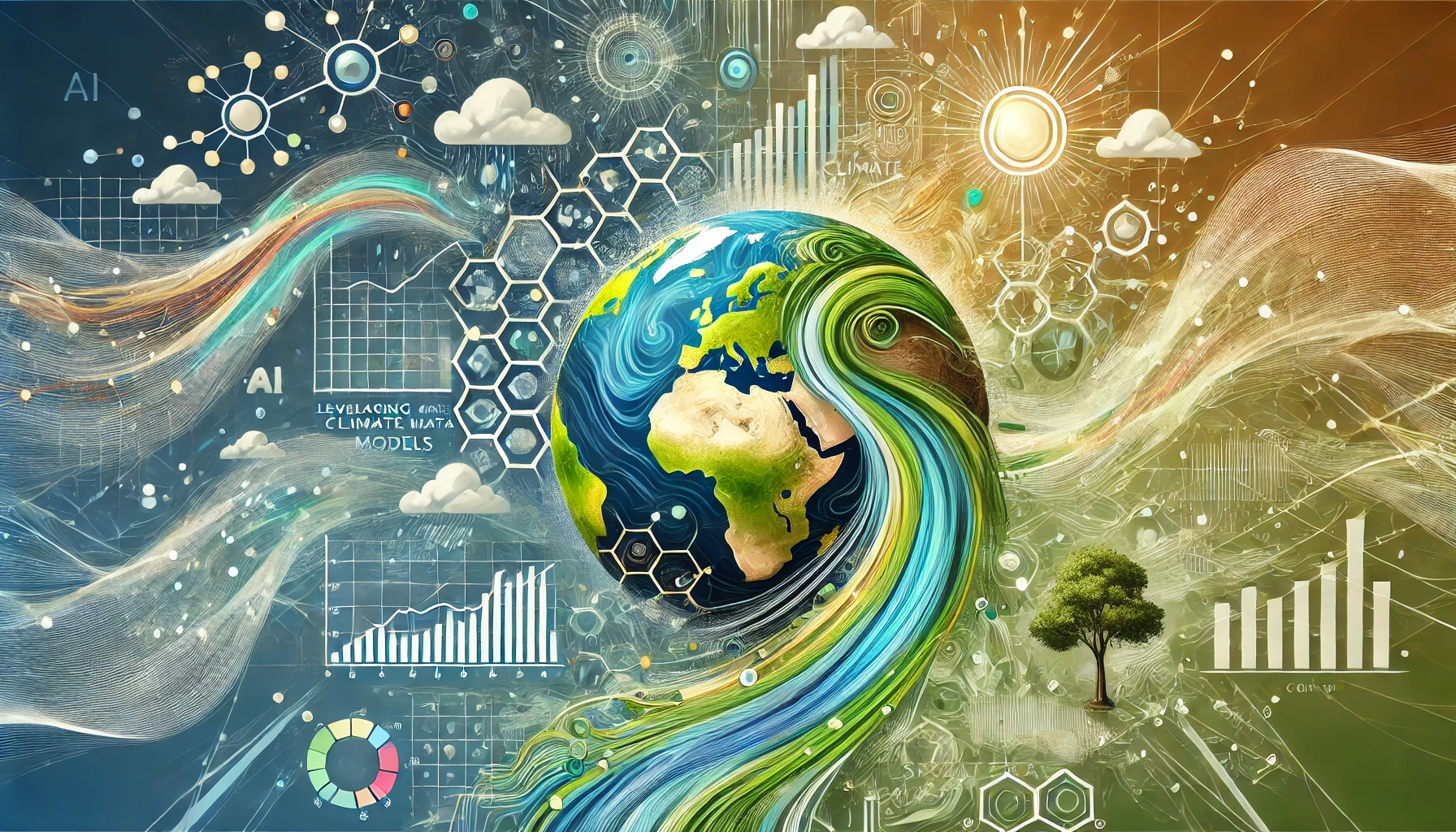
We all know it's horrible out there, and things are getting worse by the day. Climate change is one of the most pressing issues of our time, affecting every aspect of our planet. Its impacts are far-reaching, profoundly influencing ecosystems, human health, and global economies. Understanding these impacts is crucial for developing effective strategies to mitigate them. To achieve this, analyzing climate data becomes essential.
Climate data is inherently complex and characterized by its spatiotemporal nature, meaning it varies across both space and time. This complexity necessitates the use of advanced modeling techniques to interpret the data accurately. Traditional methods often fail to capture the intricate patterns and trends present in climate data.
In this article, we will explore the use of spatiotemporal AI models to analyze climate data. These models are specifically designed to handle the unique challenges posed by spatiotemporal data, allowing for more accurate and insightful analysis. We will provide detailed code examples to demonstrate how these models can be implemented and references for further reading to deepen your understanding.
By the end of this article, you will have a fundamental understanding of how spatiotemporal AI models can be leveraged to analyze climate data, enabling you to contribute to the ongoing efforts to address climate change. Understanding Spatiotemporal Models
Spatiotemporal models are designed to analyze data that changes over space and time. These models benefit climate science, where temperature, precipitation, and other climate variables vary across different regions and periods.
Key Concepts:
Spatial Data: Data with a geographic or spatial component, such as latitude and longitude.
Temporal Data: Data that changes over time, often represented in time series.
Spatiotemporal Data: Data that changes across both space and time.
Applications of Spatiotemporal Models in Climate Science
Spatiotemporal models are used in climate science for various applications, such as:
Climate Pattern Analysis: Identifying and understanding climate patterns, such as the El Niño Southern Oscillation (ENSO).
Extreme Weather Event Prediction: Predicting the likelihood and impact of extreme weather events like hurricanes, floods, and heatwaves.
Long-Term Climate Projections: Projecting future climate conditions based on historical data and climate models.
Implementing a Spatiotemporal Model in Python
A. Data Preparation
Let's use a dataset of global temperature anomalies to demonstrate how to implement a spatiotemporal model. We'll use Python and popular libraries like xarray
and PyTorch
.
Code Example:
import xarray as xr
import torch
import torch.nn as nn
# Load climate data (e.g., global temperature anomalies)
ds = xr.open_dataset("path_to_climate_data.nc")
# Extract relevant variables (e.g., temperature)
temperature = ds['temperature_anomaly']
# Convert the data to a PyTorch tensor
temperature_tensor = torch.tensor(temperature.values, dtype=torch.float32)
# Normalize the data
temperature_tensor = (temperature_tensor - temperature_tensor.mean()) / temperature_tensor.std()
print(temperature_tensor.shape) # Should show (time, latitude, longitude)
B. Building the Spatiotemporal Model
We'll use PyTorch to build a simple spatiotemporal neural network model. This model will input the temperature anomaly data and predict future temperature anomalies.
Code Example:
class SpatiotemporalModel(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(SpatiotemporalModel, self).__init__()
self.conv1 = nn.Conv2d(in_channels=1, out_channels=16, kernel_size=3, padding=1)
self.lstm = nn.LSTM(input_size=hidden_size, hidden_size=hidden_size, num_layers=2, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
x = torch.relu(self.conv1(x))
x, _ = self.lstm(x)
x = self.fc(x[:, -1, :])
return x
# Define model parameters
input_size = temperature_tensor.shape[-1] # Number of spatial features (e.g., grid points)
hidden_size = 128
output_size = 1 # Predicting one step ahead
# Initialize and train the model
model = SpatiotemporalModel(input_size, hidden_size, output_size)
# Dummy forward pass
output = model(temperature_tensor.unsqueeze(0).unsqueeze(0))
print(output)
C. Training and Evaluating the Model
We'll use historical temperature data to train the model to predict future anomalies. After training, the model can be evaluated on a test set to assess its predictive accuracy.
Code Example:
# Define loss function and optimizer
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
# Dummy training loop (for illustration)
for epoch in range(100):
model.train()
optimizer.zero_grad()
output = model(temperature_tensor.unsqueeze(0).unsqueeze(0))
loss = criterion(output, temperature_tensor[-1].unsqueeze(0)) # Predicting the last time step
loss.backward()
optimizer.step()
print(f'Epoch {epoch+1}, Loss: {loss.item()}')
Real-Life Example: Predicting Temperature Anomalies
Let's consider a real-life application in which this model could predict temperature anomalies for the next year based on historical data. This type of prediction is crucial for planning in agriculture, energy, and disaster management.
Scenario:
A government agency wants to predict temperature anomalies to plan for potential heatwaves in the coming year. Using the spatiotemporal model built above, they can input historical temperature data and generate predictions for the next year.
Spatiotemporal models are powerful tools for analyzing and predicting climate data. By leveraging these models, data scientists can gain deeper insights into climate patterns and make more accurate predictions about future climate conditions. Analyzing data across space and time is particularly valuable in understanding complex phenomena like climate change.
Further Reading and Resources
Spatiotemporal Data Science with Python by O'Reilly Media ( I use this as my reference guide)
xarray Documentation for working with multi-dimensional arrays in Python
PyTorch Documentation for building and training neural networks
NASA Earthdata for accessing climate data (Thank god this dataset exists!!!)
I hope you enjoyed reading this article and learned something new! If you have any questions or comments, please let me know.
Subscribe to my newsletter
Read articles from Madhusudhan Anand directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Madhusudhan Anand
Madhusudhan Anand
Embarking on a Journey: Unveiling My Passions and Pursuits Greetings from Bangalore, India! My name is Madhusudhan Anand, and life has been a beautiful ride of experiences and challenges. Growing up, my family's nomadic nature led us to traverse various cities in Karnataka, immersing me in the rich tapestry of diverse cultures. These encounters have left an indelible mark on my journey, shaping my passions across four distinctive realms: product development, teaching, problem-solving, and writing. As the co-founder of Ambee, a vibrant climate tech startup, my forte is transforming promising ideas into tangible, revenue-generating products. I channel my creative energy, technical expertise, and entrepreneurial spirit with every project to make a meaningful impact. Teaching has become more than just a hobby—it has become a way for me to ignite a spark of knowledge and inspiration in others. Over the years, I've had the privilege of mentoring and training over 2000 programmers worldwide. Sharing my insights and empowering aspiring talents in the world of data science and programming has been a profitable endeavor. Problem-solving is the fuel that drives my passion. With an optimistic and multidimensional perspective, I approach every challenge as an opportunity for growth. From my roots in data science and remote sensing to exploring the realms of climate change, IoT, and AI, I've harnessed my problem-solving prowess to create innovative products at Ambee. Writing has always been my sanctuary—an avenue to channel my thoughts, emotions, and ideas. I am captivated by the power of the written word to inspire, educate, and connect. Through my blog, I promise to deliver authentic, informative, and infused content with my personal touch. I'll share insights from my journey, staying true to my values and unwavering commitment to honesty. As I embark on this blogging adventure, I dedicate this platform to my late father, a constant source of inspiration and strength. His memory will forever reside in my heart, guiding me to be true to myself and positively impact the world. Join me on this exhilarating journey of exploration, learning, and growth. Let's delve into the fascinating realms of technology, data science, and personal reflections. Welcome to my world!