Functions in Rust
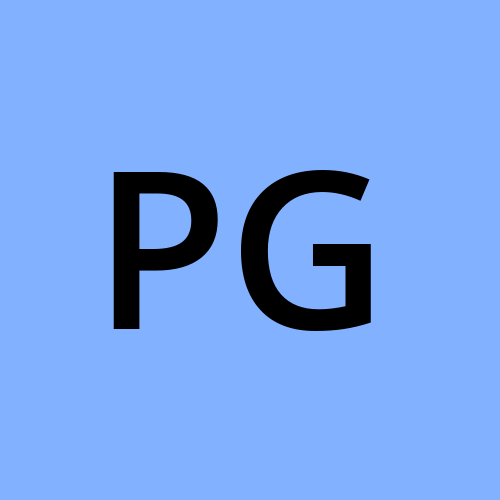
The main function present in code is an entry point for the compiler, so it is compulsory to have one main function in code.
We use fn keyword to declare functions in Rust. The functions follow snake case conventional style for naming i.e, where all the characters are lowercase and separated through underscores.
Basic Code Example:
fn main() {
println!("Hello, world!");
my_function() //fn calling
}
// fn declaration
fn my_function(){
println!("Hello from my function")
}
The Rust compiler runs the main function so we can declare remaining functions either below or above the main function. Compilation of the code is only affected by the order in which we are calling the functions.
Parameters
The main use of functions is to stop the programmer from rewriting same piece of code again and again, therefore it can take parameters and return outputs accordingly.
For example if i want to calculate the sum of two numbers at different instances in my code then i can create a standard function.
Example Code:
fn main() {
println!("Hello, world!");
sumtwo(3,5); //fn calling
}
fn sumtwo(first:i32,second:i32){
let sum=first+second;
println!("The sum of given numbers is = {}",sum);
}
Code Output:
Subscribe to my newsletter
Read articles from Pulkit Govrani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
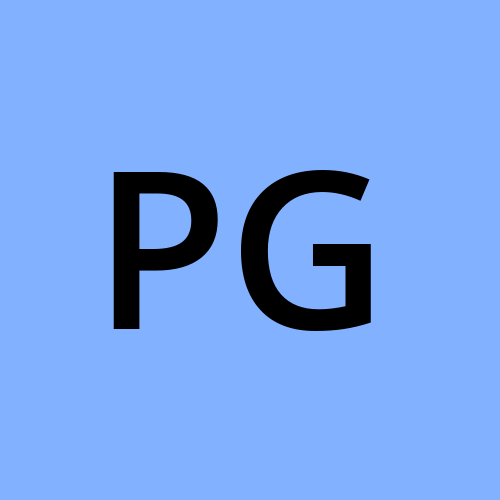