How to Create a Python Weather App
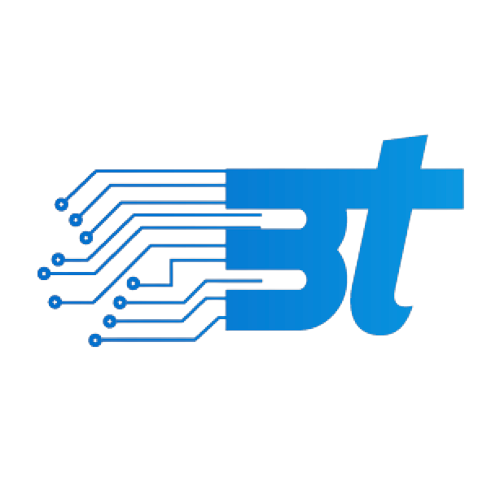
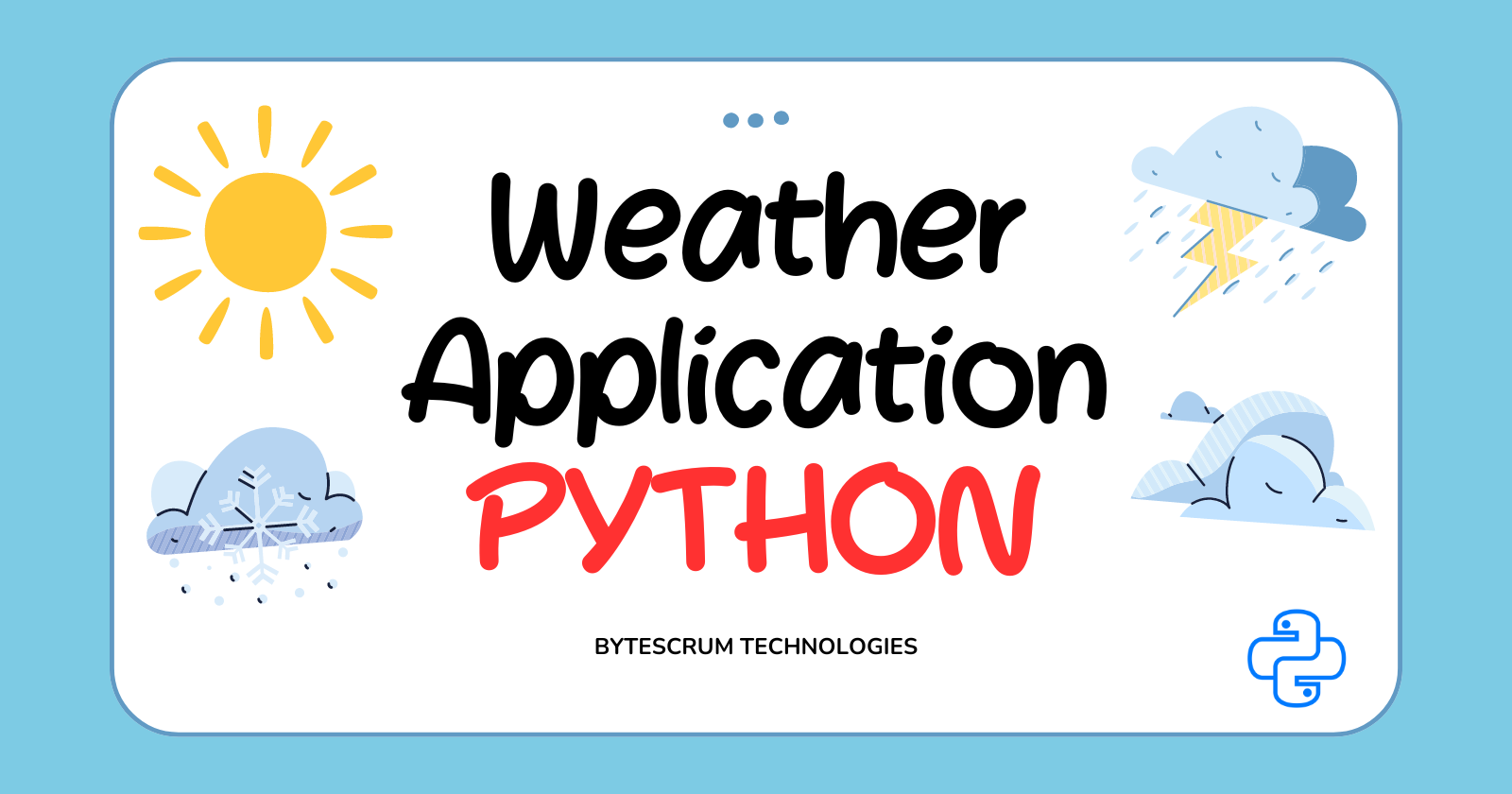
Building a Python Weather App is a fantastic project for beginners and intermediate programmers alike. It helps you learn how to interact with external APIs, handle JSON data, and create a simple graphical user interface (GUI) using tkinter
. In this guide, we will walk you through the steps to create a weather app that fetches real-time weather information from an online API and displays it in a user-friendly manner.
Why Build a Weather App?
Learn API Integration: Practice using third-party APIs to fetch and manipulate data.
Understand JSON Data: Work with JSON data formats, a crucial skill for handling data in web and app development.
GUI Development: Gain experience in creating a graphical interface using Python's
tkinter
library.Practical Usage: Create a tool that provides real-time weather information, which can be genuinely useful in daily life.
Features of the Python Weather App
Real-Time Weather Data: Fetches current weather data for any city.
Simple GUI: Easy-to-use interface for entering city names and displaying results.
Error Handling: Alerts users when an invalid city name is entered or when data fetching fails.
Cross-Platform: Runs on any operating system that supports Python.
Getting Started
To build this Python Weather App, we will need:
Python: Make sure you have Python installed on your system. You can download it from python.org.
API Key: Sign up for a free API key from a weather data provider like OpenWeatherMap.
Step 1: Install Required Libraries
We will use the requests
library to make HTTP requests to the weather API and tkinter
for the GUI.
pip install requests
brew install python-tk@3.10
Step 2: Get Your API Key
Sign up at OpenWeatherMap and obtain your free API key. This key will allow you to make requests to their weather data service. Api activation may take some time.
Step 3: Write the Python Script
Here’s how you can write the script for your weather app:
import tkinter as tk
from tkinter import messagebox
import requests
# Replace 'your_api_key_here' with your actual OpenWeatherMap API key
API_KEY = "your_api_key_here"
BASE_URL = "http://api.openweathermap.org/data/2.5/weather"
def get_weather(city):
"""
Fetches the weather data for a given city from OpenWeatherMap.
Parameters:
- city (str): The name of the city for which to fetch the weather.
Returns:
- dict: A dictionary containing weather information, or None if an error occurs.
"""
try:
# Construct the URL for the API request
url = f"{BASE_URL}?q={city}&appid={API_KEY}&units=metric"
response = requests.get(url)
data = response.json()
# Check if the response contains valid weather data
if data['cod'] == 200:
return data
else:
messagebox.showerror("Error", f"City '{city}' not found.")
return None
except Exception as e:
messagebox.showerror("Error", "Failed to fetch weather data. Please try again.")
return None
def show_weather():
"""
Retrieves weather data for the city entered by the user and updates the GUI.
"""
city = city_entry.get()
weather_data = get_weather(city)
if weather_data:
city_name = weather_data['name']
temperature = weather_data['main']['temp']
description = weather_data['weather'][0]['description']
humidity = weather_data['main']['humidity']
wind_speed = weather_data['wind']['speed']
weather_info = f"City: {city_name}\nTemperature: {temperature}°C\n" \
f"Weather: {description.capitalize()}\nHumidity: {humidity}%\n" \
f"Wind Speed: {wind_speed} m/s"
weather_label.config(text=weather_info)
def create_gui():
"""
Creates the main GUI window for the weather app.
"""
global city_entry, weather_label
window = tk.Tk()
window.title("Weather App")
window.geometry("300x250")
# Create and place the widgets
city_entry = tk.Entry(window, font=("Arial", 14))
city_entry.pack(pady=10)
get_weather_button = tk.Button(window, text="Get Weather", command=show_weather)
get_weather_button.pack(pady=5)
weather_label = tk.Label(window, font=("Arial", 12), justify="left")
weather_label.pack(pady=20)
window.mainloop()
if __name__ == "__main__":
create_gui()
How the Script Works
API Request: The
get_weather()
function constructs the request URL using the city name and your API key. It then sends a request to the OpenWeatherMap API and parses the JSON response.Data Handling: If the city is found, it extracts relevant weather information such as temperature, weather description, humidity, and wind speed.
Error Handling: If the city is not found or an error occurs, it displays an error message using
tkinter.messagebox
.GUI Components: The
create_gui()
function sets up a simpletkinter
GUI with an entry widget for the city name, a button to fetch weather data, and a label to display the weather information.Event Binding: The "Get Weather" button is bound to the
show_weather()
function, which retrieves and displays the weather information.
Running the Weather App
Run the Script: Execute the Python script from your terminal or command prompt:
python weather_app.py
Enter City Name: In the GUI window, enter the name of the city you want to check the weather for and click "Get Weather".
View Weather Data: The app will fetch and display the weather information for the entered city.
Enhancing the Weather App
To make your weather app even more feature-rich and user-friendly, consider implementing the following enhancements:
Add Forecasts: Integrate a 5-day weather forecast feature using OpenWeatherMap’s forecast API.
Improve UI/UX: Design a more attractive and user-friendly interface with additional styling and layout customization.
Save Locations: Allow users to save frequently searched cities and quickly access their weather information.
Geolocation: Automatically detect the user's location using their IP address and display the weather information for their current location.
Error Messages: Add more detailed error messages for network errors, incorrect API keys, etc.
Unit Conversion: Allow users to toggle between Celsius and Fahrenheit for temperature display.
Weather Alerts: Add functionality to alert users of severe weather conditions like storms or heavy rainfall.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
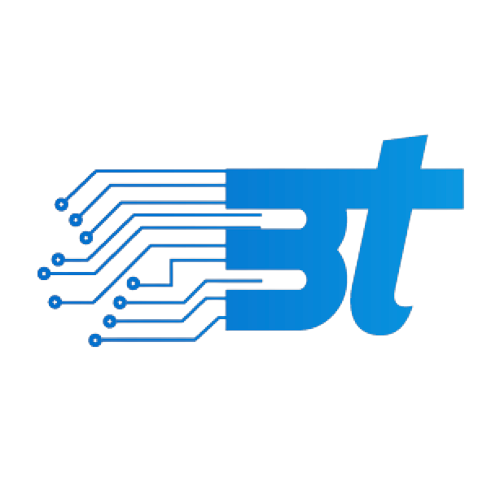
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.