Summer of Bitcoin 2024: My Experience

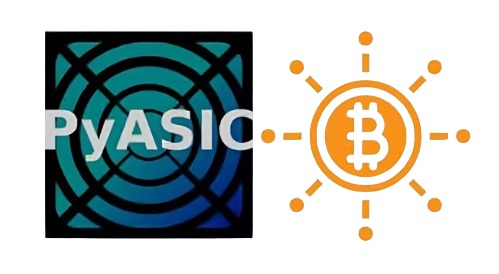
Hey everyone, welcome back! In this blog, we'll cover the progress I’ve made on the project. If you haven’t read my first blog, I recommend checking it out here an introduction to the project. For more details about my organization and the project background, be sure to read my second blog here.
My Project: Adding Firmware Update Functionality to ASIC Miners
In the rapidly evolving world of Bitcoin mining, keeping your mining hardware up to date is crucial for maintaining efficiency and competitiveness. My project focuses on enhancing the capabilities of ASIC miners by adding firmware update functionality through a user-facing API. This update will simplify the process of managing and upgrading mining hardware, ensuring that miners can stay ahead in the race for profitability.
Key Project Goals
Add Firmware Update Functionality: The primary objective is to introduce a robust mechanism for updating the firmware of various types of ASIC miners. This feature will allow users to easily upgrade their mining hardware, improving performance and security.
Develop a User-Facing API: The project will also involve creating a user-friendly API for updating firmware across different miner types. Initially, this API will take a file location as input, enabling users to upload firmware files directly. However, the ultimate goal is to integrate the API with an internal PyASIC server (e.g., firmware.pyasic.org) that will automatically retrieve the appropriate firmware files for supported miners.
Handle Backend Complexities: The API will abstract away the complexities of sending update files to the miners, making the update process seamless for users. The backend will manage the communication between the API and the mining hardware, ensuring that the updates are applied correctly and efficiently.
Understanding Bitcoin Mining
Before diving into the technical details of the project, it's essential to grasp the basics of Bitcoin mining.
Bitcoin Mining is the process through which new transactions are added to the blockchain, and new bitcoins are introduced into circulation. Miners use specialized hardware and software to solve complex cryptographic puzzles. The first miner to solve the puzzle earns a reward in bitcoins, motivating miners to participate in securing the network.
ASIC Mining: To stay competitive, most miners now use Application-Specific Integrated Circuit (ASIC) miners. These are custom-built machines designed solely for mining specific cryptocurrencies like Bitcoin. ASIC miners are far more efficient than general-purpose hardware, making them indispensable for large-scale mining operations.
Understanding Firmware Updates and Their Importance in ASIC Mining
In cryptocurrency mining, where technology and efficiency are constantly advancing, keeping your mining hardware updated is crucial. One of the most important aspects of maintaining ASIC miners is regularly updating their firmware. But what exactly is firmware, and why is it so important?
What is Firmware?
Firmware is specialized software that provides low-level control for a device's hardware. In ASIC miners, it governs how components like chips and fans operate, ensuring optimal performance and efficient handling of mining algorithms.
The Importance of Firmware Updates
Regularly updating your ASIC miners' firmware is vital for several reasons:
Enhanced Security: Updates often include patches that fix vulnerabilities, protecting your mining hardware from potential security threats.
Performance Improvements: New firmware versions may improve hash rates, reduce power consumption, or speed up processing, directly impacting your mining profitability.
New Features and Functionalities: Firmware updates can add new capabilities, such as support for additional mining algorithms, better user interfaces, or enhanced management tools.
Problem Resolution: Updates address known issues, ensuring your miners run smoothly with minimal interruptions.
1. Basic Firmware Upgrade Method
The upgrade_firmware
function is an asynchronous method designed to handle the firmware upgrade process for a device. It takes two parameters: file
, which is the path to the firmware file, and keep_settings
, a boolean that determines whether the device’s current settings should be retained after the upgrade. The function first checks if the file
parameter is provided and raises a ValueError
if it’s missing. It then calls the update_firmware
method to perform the actual firmware upgrade, awaiting the result. Depending on the outcome, the function returns a success message if the upgrade completes successfully or a failure message that includes any error details received.
async def upgrade_firmware(self, file: Path, keep_settings: bool = True) -> str:
if not file:
raise ValueError("File location must be provided for firmware upgrade.")
result = await self.web.update_firmware(file=file, keep_settings=keep_settings)
if result.get("success"):
return "Firmware upgrade completed successfully."
else:
return f"Firmware upgrade failed. Response: {result.get('message', 'Unknown error')}"
2. Sending a Command to a Device
The send_command
function is responsible for sending commands to the device via HTTP, utilizing digest authentication for security. It takes a command
parameter, which specifies the CGI command to be executed, and accepts additional parameters to be included in the request. The function constructs the appropriate URL using the device’s IP and port, and then sends an HTTP POST
request to the device with the given command and parameters. If the response status is 200, indicating success, it attempts to parse and return the JSON response. Otherwise, it returns a failure message, signaling that the command execution failed.
async def send_command(self, command: str, **parameters: Any) -> dict:
url = f"http://{self.ip}:{self.port}/cgi-bin/{command}.cgi"
auth = httpx.DigestAuth(self.username, self.password)
async with httpx.AsyncClient() as client:
response = await client.post(url, auth=auth, files=parameters)
if response.status_code == 200:
return response.json()
return {"success": False, "message": "Failed to execute command"}
3. Handling Firmware File Upload
The update_firmware
function focuses on the process of uploading the firmware file to the device and initiating the firmware upgrade. It takes the file
path and the keep_settings
boolean as input. The function reads the firmware file asynchronously to ensure the event loop is not blocked. After reading the file, it prepares the necessary parameters, including the firmware content and the keep_settings
flag, to be sent to the device. The send_command
function is then called with the upgrade
command and these parameters, which triggers the firmware upgrade process on the device.
async def update_firmware(self, file: Path, keep_settings: bool = True) -> dict:
async with aiofiles.open(file, "rb") as firmware:
file_content = await firmware.read()
parameters = {
"file": (file.name, file_content, "application/octet-stream"),
"keep_settings": keep_settings
}
return await self.send_command(command="upgrade", **parameters)
4. Error Handling in the Firmware Upgrade Process
This snippet demonstrates how to manage errors during the firmware upgrade process. The function attempts to upgrade the firmware by calling the update_firmware
method. If the upgrade fails, indicated by a lack of success in the result, the function raises an exception with the error message. The except
block catches any exceptions that occur during this process, logs the error along with detailed traceback information for debugging purposes, and then re-raises the exception to ensure the error is propagated up the call stack. This robust error handling ensures that any issues during the firmware upgrade are properly logged and managed.
try:
result = await self.web.update_firmware(file=file, keep_settings=keep_settings)
if not result.get("success"):
raise Exception(result.get("message", "Unknown error"))
except Exception as e:
logging.error(f"An error occurred: {e}", exc_info=True)
raise
Subscribe to my newsletter
Read articles from ABHISHEK PATIDAR directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
