Accessing deeply nested components in VueJS

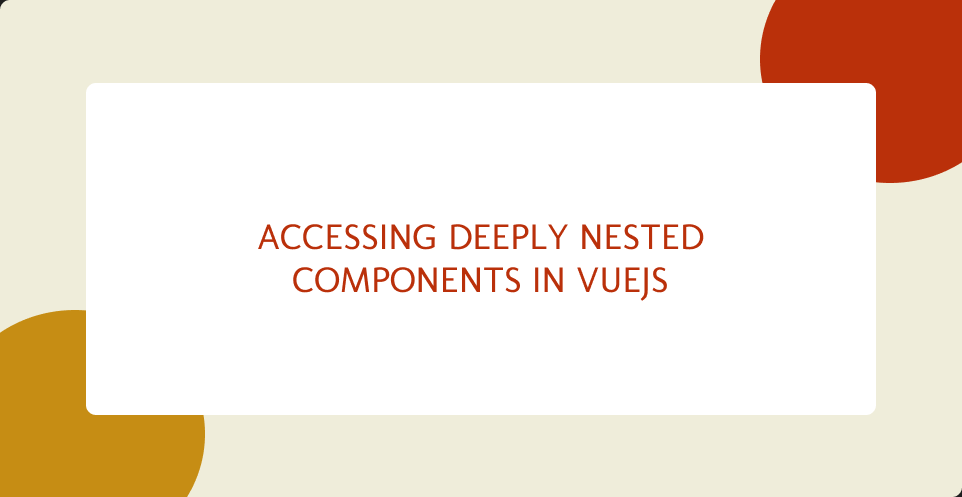
This article explores two advanced patterns for accessing deeply nested components: nested slots and the composition store.
- Nested Slots: Nested slots enable the creation of highly customisable and reusable components. By using scoped slots, you can pass data and functions from parent to child components. This approach is particularly useful for complex UIs where different parts of the component tree need to communicate or share state.
<!-- GrandchildComponent.vue -->
<template>
<div>
<slot>This is the text that is going to be
replaced by the grandparent component
</slot>
</div>
</template>
<!-- ChildComponent.vue -->
<template>
<grandchild-component>
<template v-slot>
<slot name="grandchild-component-slot"></slot>
</template>
</grandchild-component>
</template>
<!-- ParentComponent.vue -->
<template>
<ChildComponent>
<template v-slot:grandchild-component-slot>
This is going to replace the text in the grandchild component
</template>
</ChildComponent>
</template>
Here, ChildComponent
exposes a slot that ParentComponent
can use to render GrandChildComponent
, passing data down the chain.
- Composition Store: The composition store pattern centralizes state management using Vue's Composition API. This pattern is similar to Vuex but allows for more modular and granular state management within components.
// useStore.js
import { reactive } from 'vue';
const state = reactive({
data: null,
});
export function useStore() {
return {
state,
setData(newData) {
state.data = newData;
},
};
}
Components can import this store and interact with it to share state and actions:
import { useStore } from './useStore';
export default {
setup() {
const store = useStore();
return { store };
},
};
Conclusion
These advanced component composition patterns in Vue.js—nested slots and composition store— enhance the framework's flexibility and power, allowing for the creation of elegant, maintainable, and scalable applications. I will be updating this article as much as I can.
Subscribe to my newsletter
Read articles from Oluwaseyi Aimudo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Oluwaseyi Aimudo
Oluwaseyi Aimudo
Software Engineer with a knack for solving complex problems and building efficient, scalable solutions. Experienced in Javascript (VueJs). Currently learning C# so I will be documenting my journey here. I love turning ideas into reality through clean and maintainable code. Always learning, always coding.