Comprehensive Guide to Iteration ,Iterables, and Iterators in Python

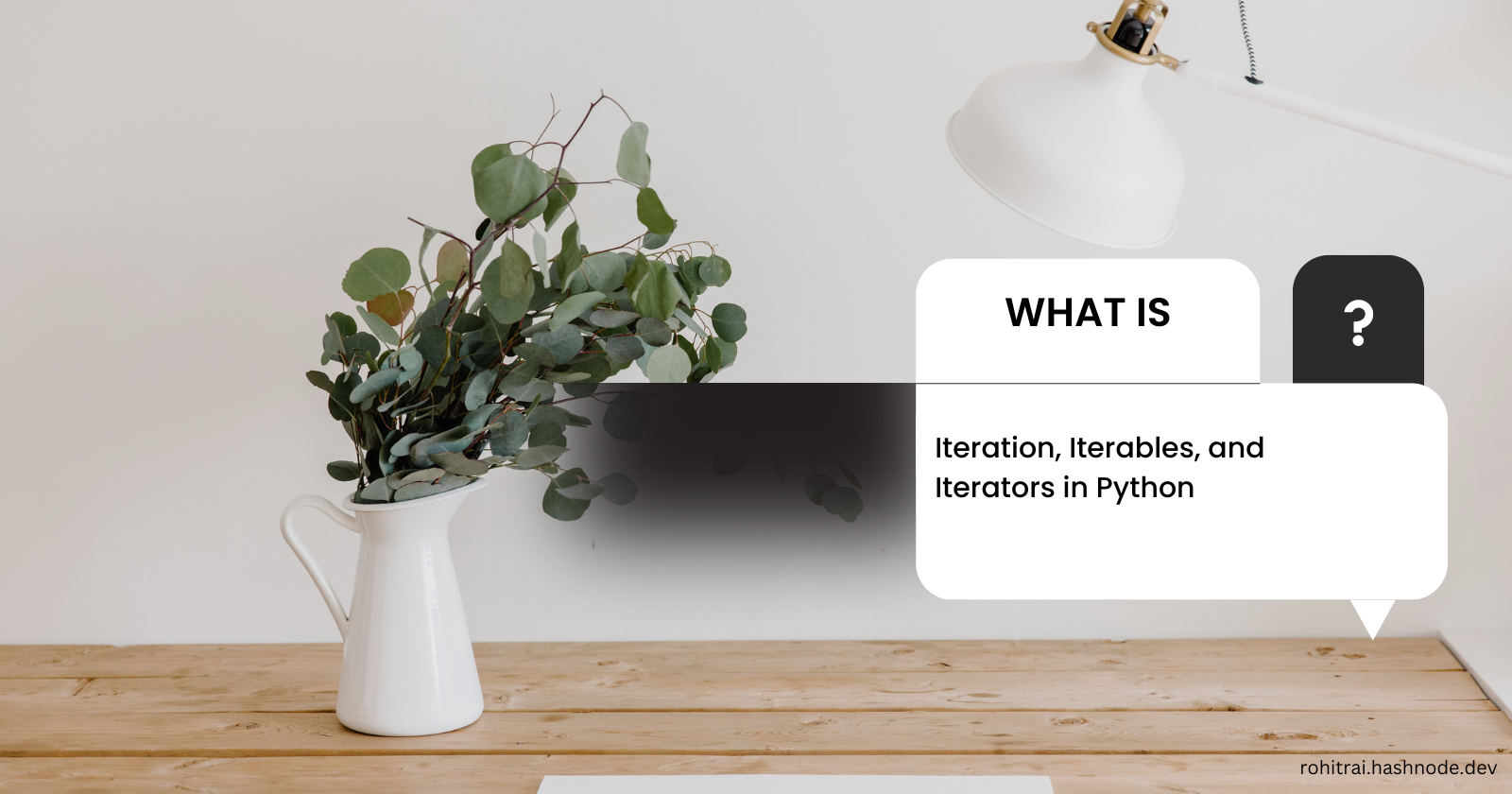
Introduction
Iteration is a fundamental concept in Python, allowing you to loop over data structures like lists, tuples, and dictionaries. Understanding how iteration works, along with the concepts of iterables and iterators, is crucial for writing efficient and readable Python code.
This guide will break down these concepts into simple terms, complete with examples to help you grasp them quickly.
What is an Iterable?
An iterable is any Python object that can be looped over (i.e., iterated). Common examples include lists, strings, and dictionaries. These objects have a special method called __iter__()
that returns an iterator.
Example:
my_list = [1, 2, 3]
for item in my_list:
print(item)
Here, my_list
is an iterable, and the for
loop is used to iterate over its elements.
What is an Iterator?
An iterator is an object that represents a stream of data. It is obtained by calling iter()
on an iterable. Iterators have a method called __next__()
, which returns the next item in the sequence. When there are no more items to return, a StopIteration
exception is raised.
Example:
my_list = [1, 2, 3]
iterator = iter(my_list)
print(next(iterator)) # Output: 1
print(next(iterator)) # Output: 2
print(next(iterator)) # Output: 3
In this example, iterator
is an iterator created from my_list
. Each call to next()
retrieves the next item.
The Relationship Between Iterables and Iterators
Every iterator is an iterable, but not every iterable is an iterator. When you use a for
loop in Python, it automatically calls iter()
to get an iterator from the iterable, then repeatedly calls next()
to get each item.
Example:
my_string = "Hello"
for char in my_string:
print(char)
Here, my_string
is an iterable, and Python handles the creation of an iterator behind the scenes.
Custom Iterators
You can create your own iterator by defining a class with __iter__()
and __next__()
methods.
Example:
class MyRange:
def __init__(self, start, end):
self.current = start
self.end = end
def __iter__(self):
return self
def __next__(self):
if self.current >= self.end:
raise StopIteration
current = self.current
self.current += 1
return current
for num in MyRange(1, 4):
print(num)
This custom iterator mimics Python's built-in range()
function, allowing you to iterate over a sequence of numbers.
Recap of Key Points
Iterable: Any object in Python that can be looped over (e.g., lists, strings). It must implement the
__iter__()
method.Iterator: An object that represents a stream of data, produced by calling
iter()
on an iterable. It must implement the__next__()
method.Iteration: The process of looping over an iterable to access its elements. Python handles the iteration process automatically with
for
loops.Custom Iterators: You can create your own iterators by defining classes with
__iter__()
and__next__()
methods, giving you control over how data is accessed.
Conclusion
Iteration, iterables, and iterators are key concepts in Python that enable efficient looping and data processing. Understanding these concepts helps you write cleaner and more Pythonic code. Whether you're using built-in iterables or creating your own custom iterators, this knowledge will serve as a foundation for mastering Python.
Subscribe to my newsletter
Read articles from Rohit Rai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rohit Rai
Rohit Rai
๐ DevOps Engineer at IBM With a solid background in both on-premise and public cloud environments, I specialize in OpenStack, AWS, and IBM Cloud. My expertise spans across infrastructure provisioning using ๐ ๏ธ Terraform, configuration management with ๐ง Ansible, and orchestration through โ๏ธ Kubernetes and OpenShift. Iโm skilled in scripting with ๐ Python and ๐ฅ๏ธ Bash, and have deep knowledge of Linux administration. Passionate about driving efficiency through automation, I strive to optimize and scale cloud operations seamlessly. ๐ Additionally, I have a keen interest in data science and am excited about exploring how data-driven insights can further enhance cloud solutions and operational strategies. ๐๐