Safe Optional Chaining (?.) vs. Try-Catch Block in JavaScript

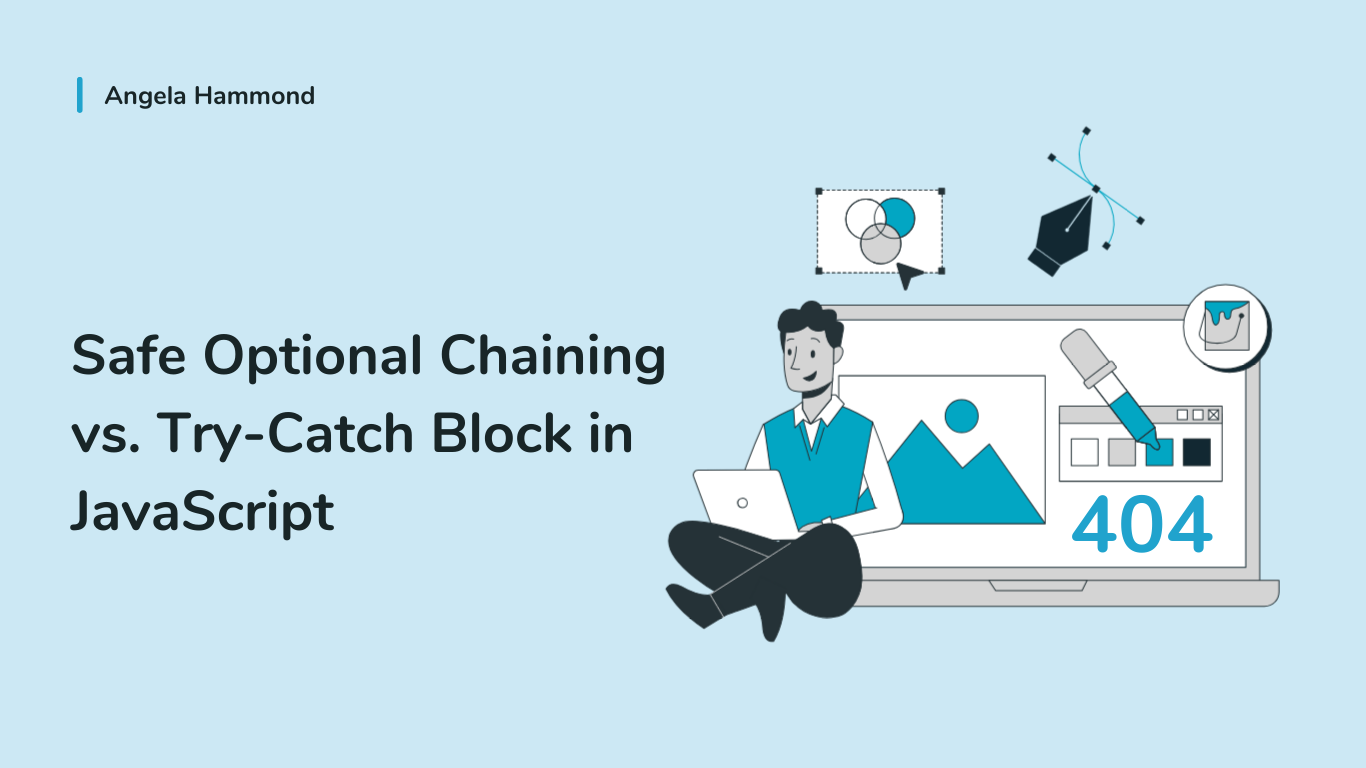
JavaScript is a flexible and dynamic language, but with this flexibility comes the need to handle potential errors, especially when dealing with complex objects or uncertain data structures. Two common approaches to error handling in JavaScript are Safe Optional Chaining (?.
) and the Try-Catch Block. Understanding when and how to use these techniques is essential for writing reliable and maintainable code in the world of newspioneers.
In this article, we'll explore these two methods, compare their use cases, and provide beginner-friendly examples to help you grasp their differences and applications.
Understanding Safe Optional Chaining (?.
)
Optional chaining is a feature introduced in ECMAScript 2020 (ES11) that allows you to safely access deeply nested properties of an object without worrying about whether intermediate properties are null
or undefined
. Before this feature, you often had to write multiple checks to avoid runtime errors.
Syntax:
let result = obj?.property?.nestedProperty;
If any part of the chain is null
or undefined
, the expression short-circuits and returns undefined
instead of throwing an error.
Example:
let user = {
name: "John",
address: {
city: "New York"
}
};
console.log(user?.address?.city); // Output: New York
console.log(user?.address?.zipcode); // Output: undefined
console.log(user?.contact?.phone); // Output: undefined
In this example, the optional chaining operator ?.
ensures that the code does not throw an error if address
or contact
is undefined
.
Understanding Try-Catch Blocks
The try-catch
block is a traditional way to handle errors in JavaScript. It allows you to "try" a block of code and "catch" any errors that occur during execution. This is useful when you expect that a block of code might throw an exception, and you want to handle it gracefully.
Syntax:
try {
// Code that may throw an error
} catch (error) {
// Code to handle the error
}
Example:
try {
let result = JSON.parse('{"name": "John"}'); // Valid JSON
console.log(result.name); // Output: John
let invalidResult = JSON.parse('{name: John}'); // Invalid JSON
} catch (error) {
console.log("An error occurred:", error.message); // Output: An error occurred: Unexpected token n in JSON at position 1
}
In this example, the try-catch
block catches the error that occurs when trying to parse invalid JSON and prevents the program from crashing.
When to Use Safe Optional Chaining (?.
)
Optional chaining is most effective when you are dealing with objects that may or may not have certain properties. It's particularly useful in scenarios where you're working with data from external sources, such as APIs, where certain fields may not always be present.
Use Cases:
Accessing nested properties in objects when you are unsure if all intermediate properties exist.
Working with optional data, such as user profiles where certain details may be missing.
Avoiding verbose and repetitive
if
checks fornull
orundefined
.
Example:
let user = {
name: "Alice",
preferences: {
theme: "dark"
}
};
let theme = user?.preferences?.theme || "default"; // Output: dark
let language = user?.preferences?.language || "en"; // Output: en
In this example, optional chaining is used to safely access preferences.theme
and preferences.language
without worrying if the properties exist. If preferences
or language
is undefined
, it returns the fallback value.
When to Use Try-Catch Blocks
While optional chaining is great for avoiding errors due to missing properties, it doesn't handle other types of errors, such as those that occur during data processing or when dealing with unexpected input. For these situations, try-catch
is more appropriate.
Use Cases:
Handling errors during asynchronous operations (e.g., network requests, file reading).
Catching exceptions in code that may fail due to external factors (e.g., invalid user input).
Managing errors in complex operations, such as parsing, calculations, or data processing.
Example:
try {
let response = fetch("https://api.example.com/data");
let data = await response.json();
console.log(data.name); // This could throw an error if the response is not valid JSON
} catch (error) {
console.error("Failed to fetch data:", error.message);
}
Here, the try-catch
block ensures that any errors occurring during the fetching or parsing of data are caught and handled, preventing the application from crashing.
Comparing Safe Optional Chaining (?.
) and Try-Catch Blocks
Key Differences:
Scope of Use:
Optional Chaining (
?.
): Specifically designed for handlingnull
orundefined
values when accessing object properties. It doesn't catch other types of errors.Try-Catch Block: A general-purpose error handling mechanism that can catch any type of error, not just
null
orundefined
.
Code Complexity:
Optional Chaining (
?.
): Simplifies code by reducing the need for repetitive checks and conditions.Try-Catch Block: Requires more code to handle errors but provides greater flexibility and control over error management.
Performance:
Optional Chaining (
?.
): Slightly faster for property access checks since it's a simple conditional operation.Try-Catch Block: Involves a performance overhead due to the additional logic required to catch and handle errors.
When to Use Which:
Use Optional Chaining (
?.
) when you only need to check fornull
orundefined
during property access.Use Try-Catch Blocks when you're dealing with operations that may throw exceptions or need to handle errors beyond just missing properties.
Examples and Use Cases
Example 1: Handling User Profiles with Optional Chaining
let user = {
name: "Bob",
profile: {
age: 30
}
};
console.log(user?.profile?.age); // Output: 30
console.log(user?.profile?.gender); // Output: undefined
In this example, optional chaining is used to access the properties of the profile
object. If profile
doesn't exist, the code won't throw an error, and undefined
is returned.
Example 2: Handling JSON Parsing with Try-Catch
try {
let jsonData = '{"name": "Bob"}'; // Valid JSON
let user = JSON.parse(jsonData);
console.log(user.name); // Output: Bob
let invalidJsonData = '{name: Bob}'; // Invalid JSON
let invalidUser = JSON.parse(invalidJsonData); // This will throw an error
} catch (error) {
console.error("JSON Parsing Error:", error.message); // Output: JSON Parsing Error: Unexpected token n in JSON at position 1
}
In this case, the try-catch
block ensures that the program handles errors that occur during JSON parsing, a common source of runtime errors.
Conclusion
Both Safe Optional Chaining (?.
) and the Try-Catch Block are powerful tools for handling different types of errors in JavaScript. Understanding their differences and when to use each can help you write cleaner, more efficient, and more resilient code.
Use Safe Optional Chaining (
?.
) for safely accessing object properties without worrying aboutnull
orundefined
values. It's a great way to simplify your code and avoid repetitive checks.Use Try-Catch Blocks when dealing with operations that can throw exceptions, such as data parsing, network requests, or complex calculations. This approach allows you to catch and handle errors gracefully, preventing crashes.
By mastering these techniques, you'll be well-equipped to handle a wide range of error scenarios in your JavaScript applications, making your code more robust and user-friendly.
Subscribe to my newsletter
Read articles from Angela Hammond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
