The Truth Behind Every 'foreach' Loop

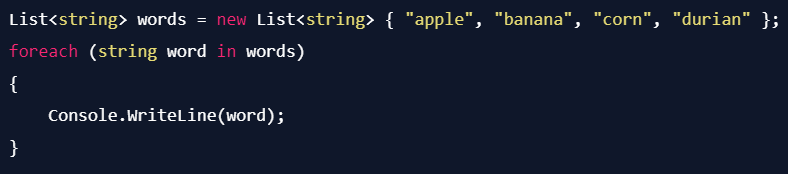
In the world of C#, one of the most common and elegant constructs is the foreach
loop. It’s simple, readable, and effective for iterating over collections. But have you ever wondered what makes this magic happen? The secret lies in the IEnumerable<T>
interface, a foundational part of .NET that quietly powers the foreach
loop behind the scenes.
The Magic Behind Foreach
Consider the following example:
List<string> words = new List<string> { "apple", "banana", "corn", "durian" };
foreach (string word in words)
{
Console.WriteLine(word);
}
This code snippet does exactly what you expect—it iterates through a list of strings and prints each one. Simple, right? But under the hood, the foreach
loop is doing more than just iterating. It’s interacting with something foundational in .NET: the IEnumerable<T>
interface.
What is IEnumerable?
IEnumerable<T>
is a simple yet powerful interface that defines what counts as a collection in .NET. At its core, IEnumerable<T>
provides a mechanism to inspect items one at a time, making it possible to loop through collections using a foreach
loop. Here’s what the interface looks like:
public interface IEnumerable<T>
{
IEnumerator<T> GetEnumerator();
}
So, what’s an enumerator? It’s the component that does the heavy lifting in a foreach
loop. The GetEnumerator
method returns an IEnumerator<T>
object, which allows you to access elements in the collection one by one.
Breaking Down IEnumerator
The IEnumerator<T>
interface is the backbone of this process. It provides the functionality needed to traverse a collection, one element at a time, with the ability to start over if needed. Here’s a simplified version of the IEnumerator<T>
interface:
public interface IEnumerator<T>
{
T Current { get; }
bool MoveNext();
void Reset();
}
Current
: This property returns the current element in the collection.MoveNext
: This method advances the enumerator to the next element in the collection and returnstrue
if there is another element to process.Reset
: This method brings the enumerator back to its initial position, which is before the first element in the collection.
While IEnumerator<T>
is rarely used directly, understanding how it works gives you insight into what happens during a foreach
loop.
The Foreach Loop Unveiled
To truly grasp how a foreach
loop works, let’s rewrite the earlier example using IEnumerator<T>
directly:
List<string> words = new List<string> { "apple", "banana", "corn", "durian" };
IEnumerator<string> iterator = words.GetEnumerator();
while (iterator.MoveNext())
{
string word = iterator.Current;
Console.WriteLine(word);
}
As you can see, the foreach
loop is just syntactic sugar for this more verbose iteration process. The loop hides the complexity of dealing with IEnumerator<T>
directly, making our code cleaner and easier to read.
This interface is so fundamental that it’s implemented by virtually every collection type in .NET, for example, List<T>
and arrays. Understanding IEnumerable<T>
helps you appreciate the elegance of C# collections and the power that comes with the simplicity of the foreach
loop.
If you read this till the end, I hope you have learned something useful! 😊✨
Subscribe to my newsletter
Read articles from emptycodes directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
