Understanding Ether Transfers in Ethereum: transfer(), send(), and call()

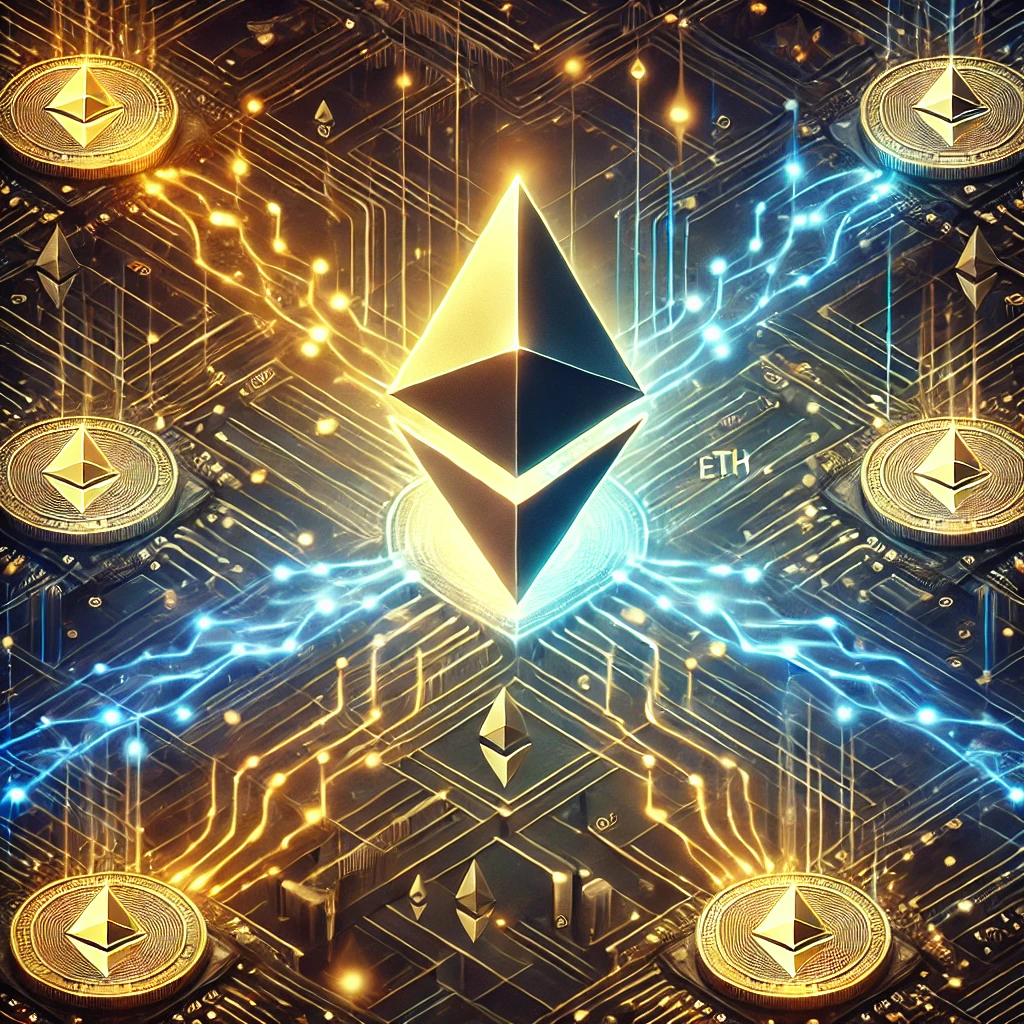
The exchange of value is one of the fundamental aspects of blockchain technology, particularly in the Ethereum ecosystem. Every cryptocurrency, including Ether (ETH), has its own mechanisms for transferring value between accounts. While many people know that Ether can be sent from one address to another, the underlying mechanisms and their trade-offs are not always well understood.
This article explores the different methods for transferring Ether between Ethereum accounts and discusses their respective trade-offs.
Ethereum Accounts: EOAs and Contract Accounts
In Ethereum, there are two types of accounts: Externally Owned Accounts (EOAs) and Contract Accounts.
Externally Owned Accounts (EOAs): These are accounts controlled by private keys. Transactions from EOAs are signed using the account's private key.
Contract Accounts: These are accounts that contain code (smart contracts) and are controlled by the logic defined within the contract. Transactions to and from Contract Accounts are initiated by EOAs or other contracts and are executed according to the contract's code.
While EOA uses private to sign transactions, the contract uses the transfer(), send() and call to send Ether to other accounts. This article will explain how the send(), transfer() and the call() method works.
Ether Transfer Methods: transfer(), send(), and call()
transfer()
The transfer()
method is a simple and straightforward way to transfer Ether from one account to another. This method:
Gas Cost: It forwards exactly 2300 gas to the receiving contract, which is enough to allow the receiving contract to execute a limited set of operations, such as logging an event.
Safety: The fixed gas limit prevents re-entrancy attacks, which is a common vulnerability in smart contracts.
Reverts on Failure: If the transfer fails (e.g., if the receiving contract runs out of gas), the transaction is automatically reverted, meaning that any changes made by the transaction up to that point are undone.
Example of using transfer
:
// Function to transfer Ether using transfer() method
function transferEther(address payable recipient, uint256 amount) public {
// transfer automatically reverts if it fails
recipient.transfer(amount);
emit TransferLog(recipient, amount, true, "transfer");
}
send()
The send()
method is similar to transfer()
in that it also forwards 2300 gas to the receiving contract. However, it has some key differences:
Gas Cost: Like
transfer()
, it also sends only 2300 gas to the receiving contract.Returns a Boolean: The
send()
method returns a boolean value (true
orfalse
) indicating whether the transfer was successful.No Revert on Failure: If the transfer fails, the transaction is not automatically reverted. This means that any state changes made before the
send()
call will persist. This requires the developer to handle errors explicitly.Recommendation: The
send()
method is no longer recommended for sending Ether due to its limitations and the introduction of more flexible alternatives likecall()
.function sendEther(address payable recipient, uint256 amount) public returns (bool) { // send returns false if it fails bool success = recipient.send(amount); emit TransferLog(recipient, amount, success, "send"); return success; }
call()
The
call()
method is the most flexible and recommended way to send Ether in modern smart contracts. It allows the sender to specify the exact amount of gas to forward and includes more detailed control over the transaction:Gas Cost: The sender can specify the amount of gas to send, making it more flexible than
transfer()
andsend()
.Flexibility:
call()
can trigger the fallback function of the receiving address, which allows for a wider range of operations to be executed.Returns a Boolean: Like
send()
, thecall()
method also returns a boolean value indicating success or failure. Additionally, it returns data from the called function, which can be useful in some cases.Low-Level Call:
call()
is a low-level function, meaning that it does not perform any checks or safety mechanisms on its own. This flexibility comes with risks, as it can be exploited by malicious actors if not used carefully.
// Function to transfer Ether using call() method
function callEther(address payable recipient, uint256 amount) public returns (bool) {
// call returns a boolean value indicating success or failure
(bool success, ) = recipient.call{value: amount}("");
emit TransferLog(recipient, amount, success, "call");
return success;
}
Understanding the differences between transfer()
, send()
, and call()
is crucial for developers working with Ethereum smart contracts. Each method has its own use cases, advantages, and trade-offs. While transfer()
is simple and safe, it lacks flexibility. send()
offers error handling but is generally outdated. call()
provides maximum flexibility but requires careful handling to avoid security vulnerabilities.
- I hope this article helps in understanding, transfer(), send() and call functions.
Subscribe to my newsletter
Read articles from Habeeblai musa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
