Profiling Tools and Techniques for Node.js Applications
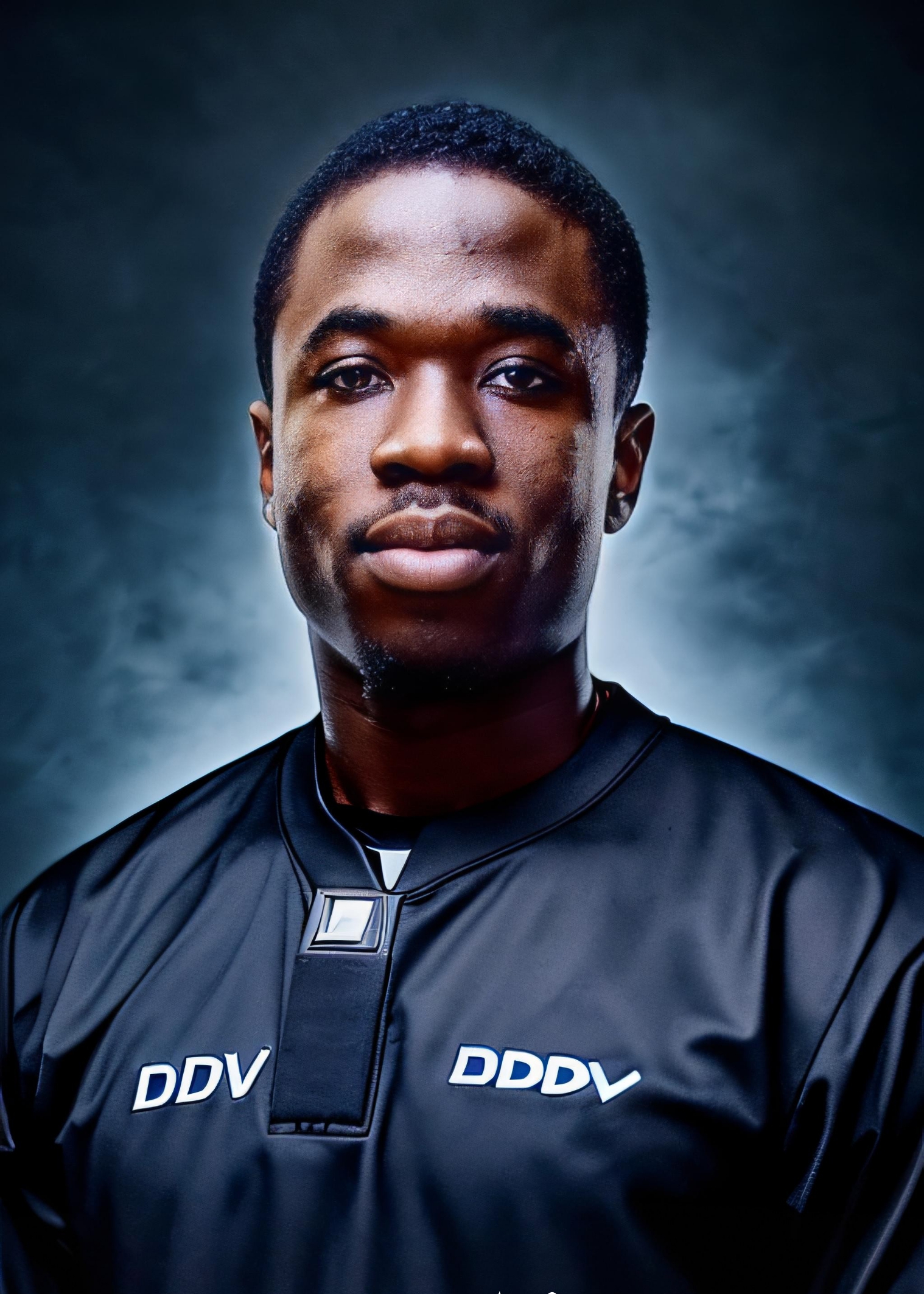
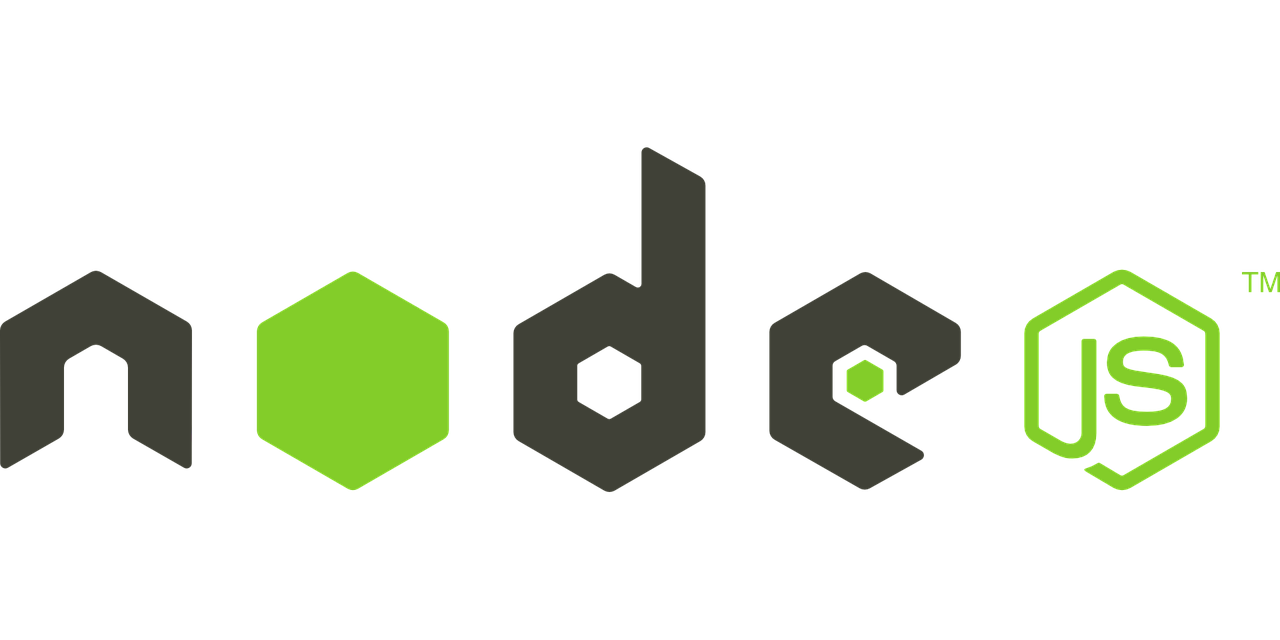
Node.js is a powerful platform for building server-side applications due to its asynchronous, non-blocking architecture. However, as applications scale, performance bottlenecks can arise, leading to increased latency and degraded user experience. Profiling is a crucial process that helps developers identify and address these bottlenecks. This article explores the tools and techniques available for profiling Node.js applications, providing insights on how to optimize performance.
Understanding Profiling
Profiling involves collecting data on the performance characteristics of an application. This data includes CPU usage, memory consumption, and execution times for various parts of the code. Profiling helps identify the following common performance issues:
CPU-bound processes: Functions that consume excessive CPU resources.
Memory leaks: Memory that is not properly released, leading to increased memory consumption over time.
Blocking code: Code that prevents the event loop from processing other tasks, leading to increased response times.
Key Profiling Tools for Node.js
Several tools are available for profiling Node.js applications, each offering different levels of granularity and ease of use. Below are some of the most popular and effective tools:
Node.js Built-in Profiler
Overview: Node.js comes with a built-in profiler that provides basic profiling capabilities using the V8 engine's
--prof
flag. This generates a log file that can be analyzed to understand where your application spends most of its time.Usage:
node --prof app.js
Analysis: The log file can be processed using the
node --prof-process
command, which generates a human-readable summary of the profile data.Strengths: It's readily available and integrated with Node.js, making it easy to use without additional setup.
Limitations: The output is somewhat cryptic and may require expertise to interpret effectively.
Clinic.js
Overview: Clinic.js is a suite of tools designed for profiling and diagnosing performance issues in Node.js applications. It includes tools like
clinic doctor
,clinic flame
, andclinic bubbleprof
.Usage:
npx clinic doctor -- node app.js
Key Features:
clinic doctor
: Provides a high-level overview and recommendations for improving performance.clinic flame
: Generates flame graphs that visualize the performance of various parts of your application.clinic bubbleprof
: Offers a unique visualization of asynchronous operations in your application.
Strengths: It provides a user-friendly interface and visualizations, making it easier to identify performance bottlenecks.
Limitations: It may require additional installation steps and some learning curve to fully leverage its capabilities.
Chrome DevTools
Overview: Chrome DevTools is a powerful suite of debugging tools built into the Chrome browser. It can be used to profile Node.js applications by connecting remotely.
Usage:
node --inspect app.js
Then, open Chrome and navigate to
chrome://inspect
to connect to the running Node.js process.Key Features:
CPU and Memory Profiling: Provides detailed breakdowns of CPU and memory usage.
Flame Charts: Visualize the time spent on various functions.
Heap Snapshots: Analyze memory usage and detect memory leaks.
Strengths: Integrated with Chrome, offering a rich set of features for detailed profiling.
Limitations: Requires familiarity with DevTools, and the setup can be complex for beginners.
V8 Profiler
Overview: The V8 Profiler is an advanced tool for profiling JavaScript code running in Node.js. It provides in-depth analysis of CPU usage and memory consumption.
Usage: The V8 Profiler can be integrated into your application via the
v8-profiler-node8
package.npm install v8-profiler-node8
Then, you can start and stop profiling within your application code.
Key Features:
CPU Profiles: Detailed reports on CPU usage.
Heap Snapshots: Analyze memory usage and detect leaks.
Strengths: Offers deep insights into V8 engine performance.
Limitations: Requires manual integration and is more complex to use.
Heapdump
Overview: Heapdump is a tool that captures heap snapshots of your Node.js application, which can be analyzed to understand memory usage patterns and detect memory leaks.
Usage:
npm install heapdump
Within your application, you can trigger a heap snapshot:
const heapdump = require('heapdump'); heapdump.writeSnapshot('snapshot.heapsnapshot');
Strengths: Provides detailed insights into memory allocation and usage.
Limitations: Snapshots can be large and difficult to analyze without the right tools.
Techniques for Effective Profiling
Profiling is not just about running tools—it's about using them effectively to gain actionable insights. Here are some best practices:
Baseline Performance: Before making any changes, establish a baseline performance metric for your application. This allows you to measure the impact of your optimizations.
Isolate Performance Issues: Use profiling tools to isolate specific performance issues. For example, identify whether the bottleneck is CPU-bound, I/O-bound, or memory-bound.
Focus on Hotspots: Concentrate on optimizing the hotspots—functions or modules where your application spends the most time. Small improvements in these areas can lead to significant overall performance gains.
Iterative Optimization: Optimization should be an iterative process. Profile your application, make targeted optimizations, and then profile again to measure the impact.
Monitor in Production: Performance issues may not always surface in development environments. Use monitoring tools like New Relic or Datadog to profile and monitor your application in production.
Conclusion
Profiling is an essential aspect of maintaining and optimizing the performance of Node.js applications. By leveraging the right tools and techniques, developers can gain deep insights into the performance characteristics of their applications, identify bottlenecks, and make informed decisions on optimizations. Whether you're using built-in profilers, visual tools like Clinic.js, or advanced memory analysis tools, the key is to integrate profiling into your development workflow to ensure your Node.js applications run efficiently at scale.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
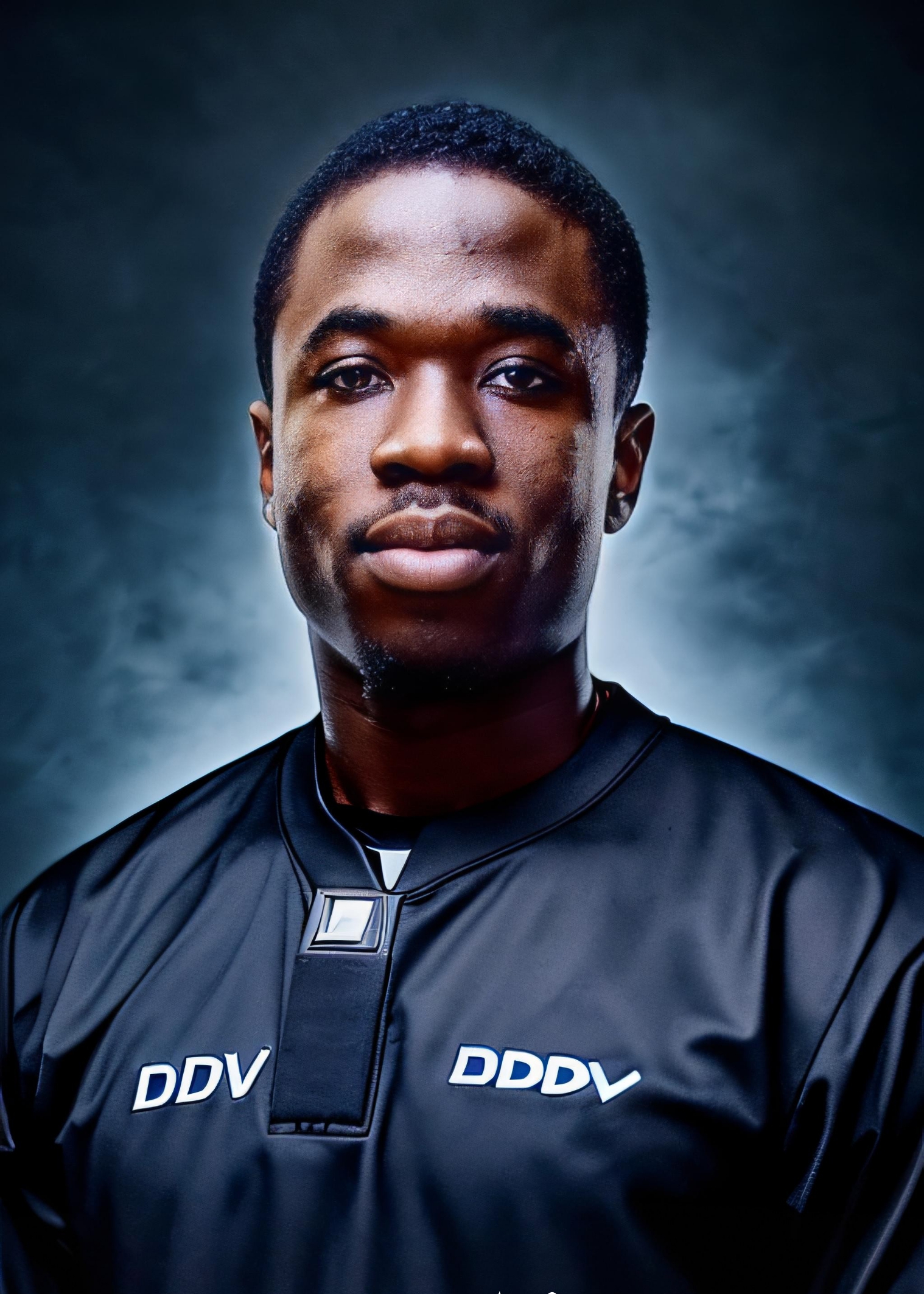
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.