Best Practices for Securing Node.js Applications in Production
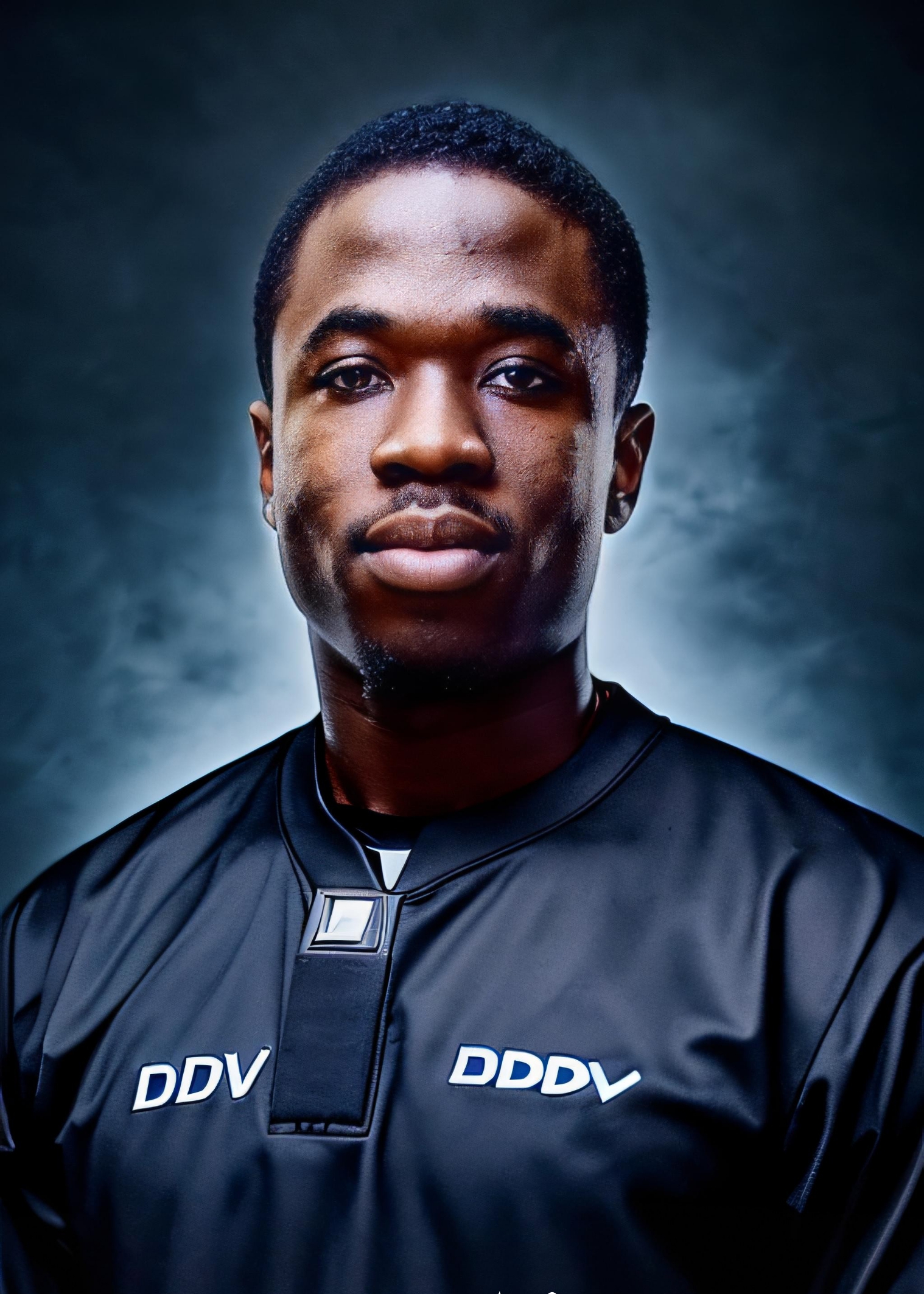
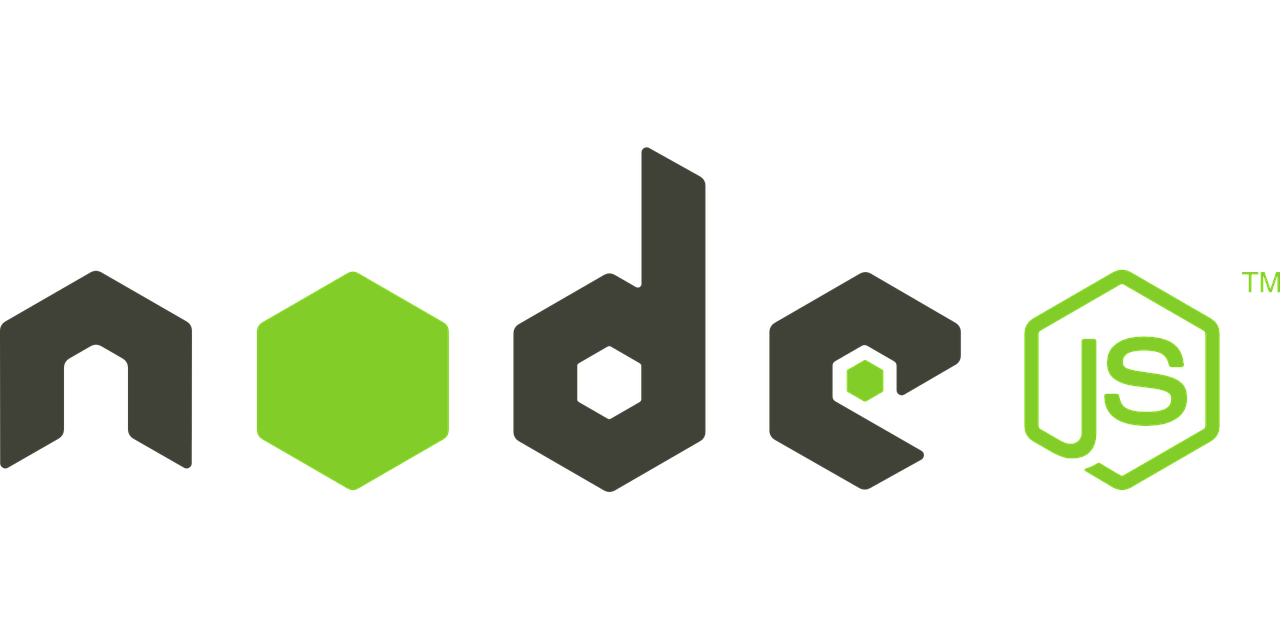
Node.js is a powerful and popular platform for building scalable server-side applications. However, like any other technology, it comes with its own set of security concerns that developers must address to ensure their applications are secure in production. This article will cover best practices for securing Node.js applications in production, focusing on common vulnerabilities and how to mitigate them.
1. Keep Node.js and Dependencies Updated
Why it Matters: Security vulnerabilities are regularly discovered in software, including Node.js itself and its dependencies. Failing to update can leave your application exposed to known exploits.
Best Practice: Regularly update Node.js to the latest stable version. Use tools like
npm audit
andsnyk
to scan for vulnerabilities in your dependencies. Automate dependency updates using tools likeRenovate
orDependabot
.
2. Use HTTPS and Secure Network Connections
Why it Matters: Data transmitted over unencrypted channels can be intercepted and altered by attackers, leading to potential data breaches and man-in-the-middle attacks.
Best Practice: Always use HTTPS to encrypt data in transit. Obtain and manage SSL/TLS certificates using tools like Let's Encrypt and automate certificate renewal. Ensure all internal microservices also communicate over HTTPS or secure channels.
3. Implement Strong Authentication and Authorization
Why it Matters: Weak authentication mechanisms can allow unauthorized access to your application, while improper authorization controls can lead to privilege escalation attacks.
Best Practice: Implement strong authentication mechanisms such as OAuth2, JWT (JSON Web Tokens), or multi-factor authentication (MFA). Ensure that roles and permissions are clearly defined and enforced throughout the application.
4. Validate and Sanitize User Input
Why it Matters: Unsanitized user input is a common attack vector for injection attacks such as SQL injection, NoSQL injection, and Cross-Site Scripting (XSS).
Best Practice: Validate all incoming data against expected formats and types. Sanitize input to remove or escape special characters that can be used in injection attacks. Use libraries like
validator.js
for validation andDOMPurify
for sanitization.
5. Protect Against Cross-Site Scripting (XSS)
Why it Matters: XSS attacks can lead to the execution of malicious scripts in the user's browser, compromising sensitive data such as cookies, session tokens, and more.
Best Practice: Use a templating engine that automatically escapes output, such as Handlebars. Apply Content Security Policy (CSP) headers to restrict the sources from which scripts can be loaded. Sanitize user-generated content before displaying it.
6. Secure Session Management
Why it Matters: Poor session management can lead to session hijacking, fixation, and replay attacks, compromising user accounts.
Best Practice: Use secure, HttpOnly, and SameSite cookies for session management. Implement short session expiration times and regenerate session IDs on each request. Consider using token-based authentication mechanisms like JWT for stateless sessions.
7. Limit Rate and Resource Usage
Why it Matters: Unrestricted resource usage can make your application vulnerable to Denial of Service (DoS) attacks, where an attacker overwhelms your server with requests.
Best Practice: Implement rate limiting and throttling to control the number of requests a client can make within a specified timeframe. Use libraries like
express-rate-limit
to set up rate limiting. Monitor resource usage and apply circuit breakers or graceful degradation techniques to handle load spikes.
8. Handle Errors and Logging Securely
Why it Matters: Improper error handling can expose sensitive information, such as stack traces and database queries, to attackers.
Best Practice: Avoid exposing detailed error messages to end-users. Use generic error messages for users and log detailed errors on the server side. Ensure logs do not contain sensitive information like passwords, tokens, or PII (Personally Identifiable Information). Use log management tools like ELK Stack or Papertrail for centralized logging.
9. Use Environment Variables Securely
Why it Matters: Hardcoding sensitive information such as API keys, database credentials, and encryption keys in your source code can lead to exposure if the code is compromised.
Best Practice: Store sensitive information in environment variables, and use tools like
dotenv
to manage these variables securely. Ensure environment variables are not exposed to the client side. Avoid committing.env
files to version control systems.
10. Implement Content Security Policies (CSP)
Why it Matters: CSPs help prevent a variety of attacks, including XSS and data injection attacks, by controlling which resources can be loaded on a webpage.
Best Practice: Define a strict Content Security Policy that restricts the sources of scripts, styles, and other resources. Continuously monitor and update your CSP to adapt to new threats and changes in your application.
11. Regular Security Audits and Penetration Testing
Why it Matters: Security is not a one-time task. Regular audits and testing help identify new vulnerabilities and ensure your application remains secure over time.
Best Practice: Conduct regular security audits and penetration testing using automated tools like OWASP ZAP and manual testing by security professionals. Address identified vulnerabilities promptly and update your security policies and practices accordingly.
12. Monitor and Respond to Security Incidents
Why it Matters: Quick detection and response to security incidents can minimize the impact of an attack and prevent further damage.
Best Practice: Implement real-time monitoring and alerting for suspicious activities using tools like New Relic, Datadog, or AWS CloudWatch. Have an incident response plan in place and conduct regular drills to ensure your team is prepared to respond effectively.
13. Secure Your Development and Deployment Pipeline
Why it Matters: Compromising your CI/CD pipeline can lead to unauthorized code changes and the deployment of malicious software.
Best Practice: Use secure CI/CD practices, such as signed commits, branch protection, and code reviews. Ensure that build and deployment servers are secured with strong authentication and are isolated from public networks. Regularly audit the pipeline for security vulnerabilities.
Conclusion
Securing a Node.js application in production requires a holistic approach that covers everything from code quality to network security. By following these best practices, you can significantly reduce the risk of security breaches and ensure that your application remains robust and secure in the face of evolving threats. Remember, security is an ongoing process, and staying informed about the latest vulnerabilities and mitigation strategies is key to maintaining a secure application.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
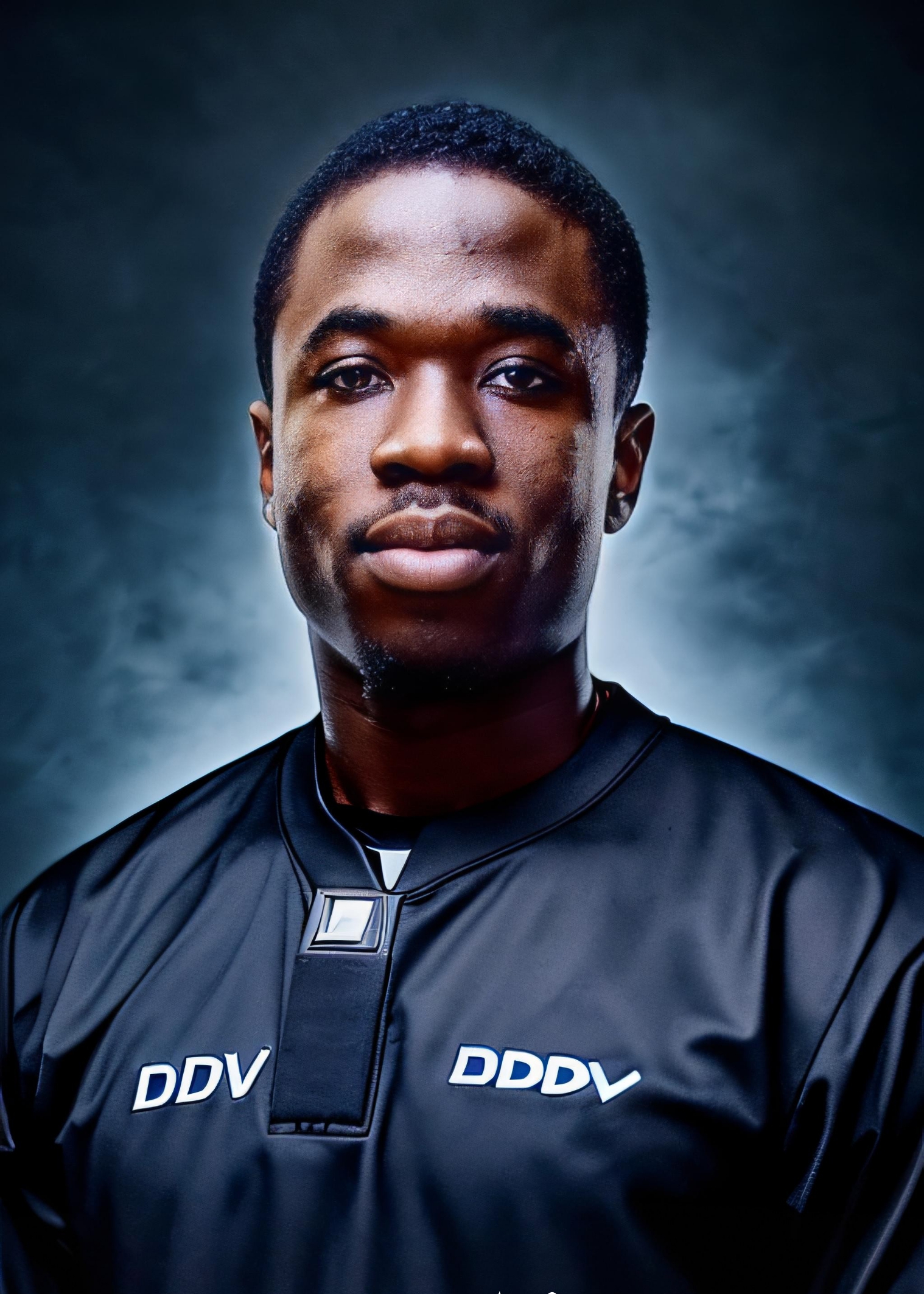
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.