Create a Python Tool for Analyzing Personal Fitness Data
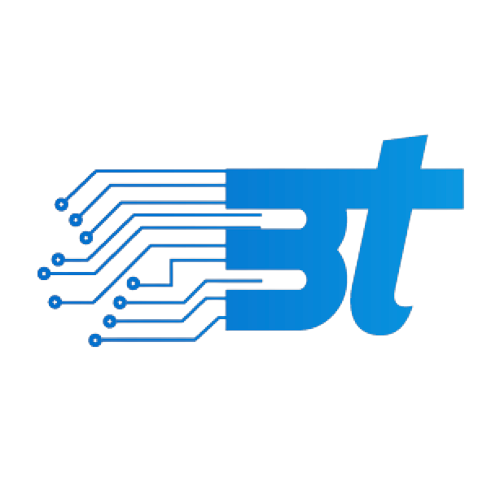
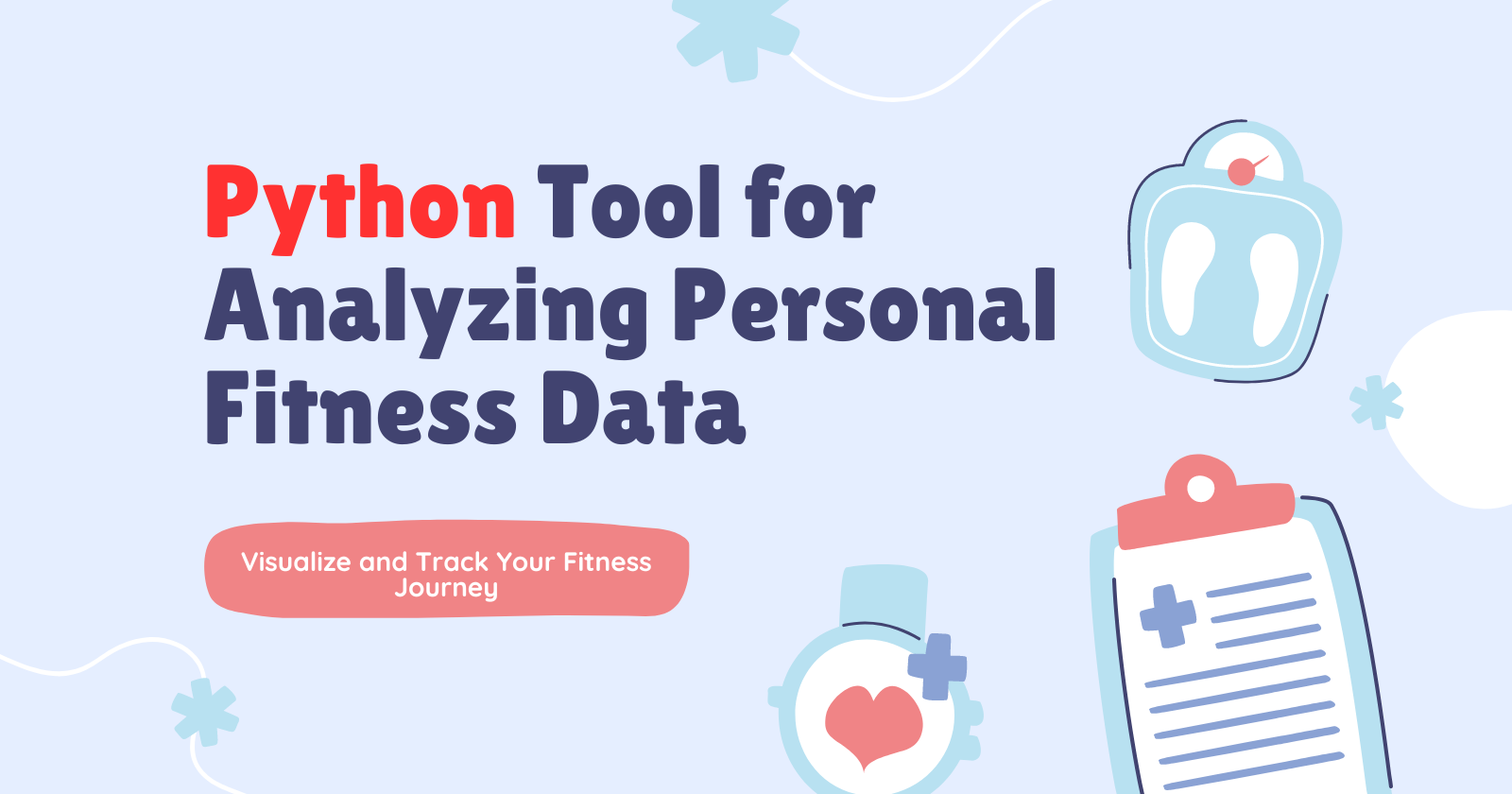
Tracking your fitness progress is essential to staying motivated and achieving your goals. By analyzing personal fitness data, you can identify trends, understand your strengths, and improve your weaknesses. In this blog post, we will guide you through creating a Python tool to analyze personal fitness data, leveraging libraries like Pandas for data manipulation, Matplotlib and Seaborn for visualization, and NumPy for numerical operations.
1. Prerequisites
Before diving into this tutorial, make sure you have the following:
Basic knowledge of Python programming.
Familiarity with libraries like Pandas, Matplotlib, and NumPy.
Your personal fitness data (e.g., CSV file with daily steps, calories burned, exercise duration).
2. Setting Up the Environment
Let's start by setting up a virtual environment and installing the necessary Python packages:
# Create a new directory for the project
mkdir fitness_data_analysis
cd fitness_data_analysis
# Set up a virtual environment
python -m venv venv
source venv/bin/activate # On Windows use `venv\Scripts\activate`
# Install required libraries
pip install pandas matplotlib seaborn numpy
3. Preparing Your Fitness Data
You’ll need a CSV file containing your fitness data. This could be exported from a fitness tracker or manually logged. Make sure your data includes columns such as:
Date
Steps
Calories burned
Exercise duration (minutes)
Sleep duration (hours)
Example of fitness_data.csv
:
Date | Steps | Calories | Exercise Duration (min) | Sleep Duration (hours) |
2024-08-01 | 10500 | 2500 | 45 | 7.5 |
2024-08-02 | 8000 | 2000 | 30 | 6.0 |
... | ... | ... | ... | ... |
4. Loading and Cleaning Data with Pandas
Load your data into a Pandas DataFrame and perform basic data cleaning:
# fitness_analysis.py
import pandas as pd
# Load the fitness data CSV file into a DataFrame
df = pd.read_csv('fitness_data.csv')
# Display the first few rows of the DataFrame
print(df.head())
# Convert the 'Date' column to datetime format
df['Date'] = pd.to_datetime(df['Date'])
# Check for missing values
print(df.isnull().sum())
# Fill missing values with appropriate methods or drop rows if necessary
df.fillna(method='ffill', inplace=True)
5. Analyzing Fitness Metrics
Now that we have our data ready, let’s analyze some key fitness metrics:
- Total Steps per Month:
# Group data by month and calculate the total steps
df['Month'] = df['Date'].dt.to_period('M')
total_steps_per_month = df.groupby('Month')['Steps'].sum()
print(total_steps_per_month)
- Average Calories Burned per Week:
# Calculate the weekly average of calories burned
df['Week'] = df['Date'].dt.isocalendar().week
average_calories_per_week = df.groupby('Week')['Calories'].mean()
print(average_calories_per_week)
- Daily Exercise Duration Analysis:
# Basic statistics for daily exercise duration
exercise_stats = df['Exercise Duration (min)'].describe()
print(exercise_stats)
6. Visualizing Your Progress
Visualization helps you understand your data better. We will use Matplotlib and Seaborn to create insightful charts.
- Line Chart for Steps Progress Over Time:
import matplotlib.pyplot as plt
plt.figure(figsize=(10, 5))
plt.plot(df['Date'], df['Steps'], marker='o', linestyle='-')
plt.title('Daily Steps Over Time')
plt.xlabel('Date')
plt.ylabel('Steps')
plt.grid(True)
plt.show()
- Bar Chart for Monthly Calories Burned:
import seaborn as sns
plt.figure(figsize=(10, 5))
sns.barplot(x=total_steps_per_month.index.astype(str), y=total_steps_per_month.values)
plt.title('Total Steps Per Month')
plt.xlabel('Month')
plt.ylabel('Total Steps')
plt.xticks(rotation=45)
plt.show()
- Histogram for Exercise Duration:
plt.figure(figsize=(8, 4))
plt.hist(df['Exercise Duration (min)'], bins=20, color='skyblue', edgecolor='black')
plt.title('Distribution of Daily Exercise Duration')
plt.xlabel('Exercise Duration (min)')
plt.ylabel('Frequency')
plt.show()
7. Advanced Analytics with Python
Explore more advanced analytics using Python. For example, identifying trends in your fitness data or using machine learning to predict future fitness outcomes.
# Example: Calculate 7-day moving average for steps
df['7-Day Moving Average'] = df['Steps'].rolling(window=7).mean()
plt.figure(figsize=(10, 5))
plt.plot(df['Date'], df['Steps'], label='Daily Steps', alpha=0.7)
plt.plot(df['Date'], df['7-Day Moving Average'], label='7-Day Moving Average', color='red')
plt.title('Daily Steps and 7-Day Moving Average')
plt.xlabel('Date')
plt.ylabel('Steps')
plt.legend()
plt.show()
8. Deploying and Sharing Your Tool
Once your tool is complete, you can share it with others by creating a Python package, a Jupyter Notebook, or a web application using Flask or Django. You can also deploy your project on platforms like GitHub or create an interactive dashboard using Streamlit or Dash.
Conclusion
Happy coding, and stay fit!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
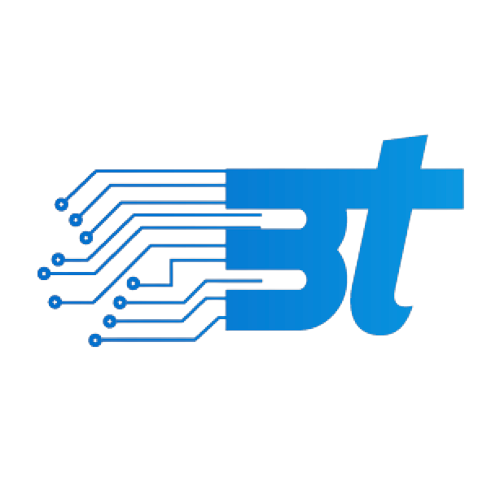
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.