Master the Art of Extracting Image URLs from Any Website Using DevTools A Step-by-Step Guide
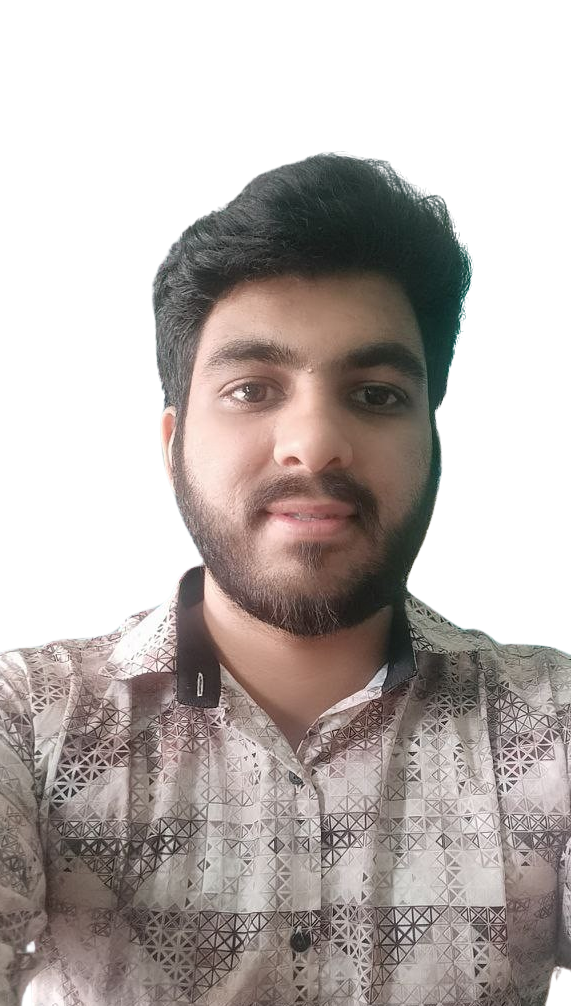
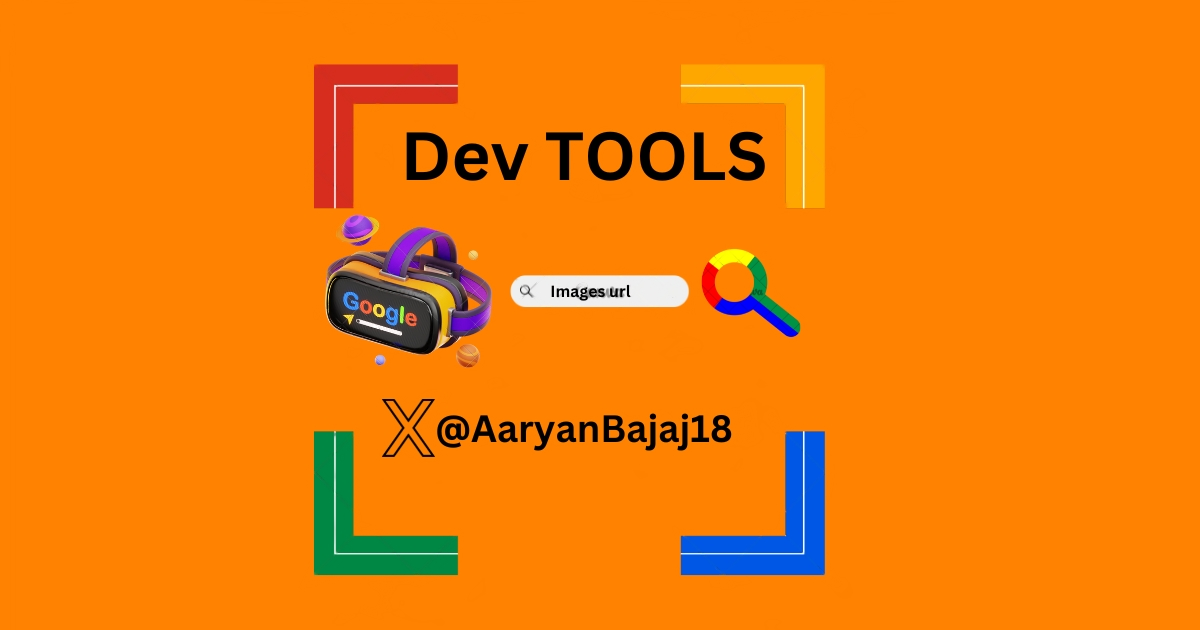
Introduction
Have you ever stumbled upon a website filled with stunning images and wished you could get your hands on those image URLs without the tedious task of right clicking and saving each one ⌛
Well, I've got a trick up my sleeve that will make this process as smooth as butter
In this blog, I’ll guide you through a fascinating method to extract all image URLs from any website directly through your browser's console
Steps
Step 1: Open the Website
First things first, navigate to the website that contains the images you want to extract. Make sure all the images you want to capture are loaded on the page. This is crucial because our trick will pull URLs directly from what's visible (and sometimes hidden) in your browser.
Step 2: Inspect the Page
Now, we’ll move into the behind-the-scenes world of your browser. Right-click anywhere on the page and select “Inspect” or simply press F12
on your keyboard. This will open up the DevTools panel, your gateway to everything happening under the hood of the website.
Step 3: Navigate to the Console
In the DevTools panel, you’ll see various tabs like Elements, Network, and Sources. What we’re interested in is the "Console" tab. This is where all the magic happens. Click on it, and you’re ready for the next step.
Step 4: Enter the Magic Code
// Function to get the true image source
function getTrueImageSrc(img) {
// Check for data-src attribute (commonly used for lazy loading)
if (img.getAttribute('data-src')) {
return img.getAttribute('data-src');
}
// Check for srcset attribute
if (img.srcset) {
// Get the highest resolution image from srcset
const srcset = img.srcset.split(',').map(s => s.trim().split(' '));
const highestRes = srcset.reduce((prev, current) => {
const prevSize = parseInt(prev[1]) || 0;
const currentSize = parseInt(current[1]) || 0;
return currentSize > prevSize ? current : prev;
});
return highestRes[0];
}
// Fallback to src attribute
return img.src;
}
// Function to extract and log image links
function extractImageLinks() {
const images = document.getElementsByTagName('img');
const imageLinks = Array.from(images).map(img => getTrueImageSrc(img));
console.log('Image links found on this page:');
imageLinks.forEach((link, index) => {
console.log(`${index + 1}. ${link}`);
});
console.log(`\nTotal images found: ${imageLinks.length}`);
console.log('You can copy these links and open them in new tabs manually.');
}
// Run the function
extractImageLinks();
Once you have the code ready, paste it directly into the Console tab and press Enter. If you face any issues with pasting the code, you might see a message about security. No worries—just type "allow pasting" into the console and hit enter, then paste the code again.
What Does the Code Do?
This script works by:
Defining a Function: It creates a function that meticulously checks each image element on the page to determine the correct source URL. It considers various attributes like
data-src
andsrcset
, which are often used for responsive images.Collecting Image Elements: It gathers all
<img>
elements from the page, ensuring nothing is missed.Extracting True Source URLs: The script then extracts the actual source URL for each image, whether it’s hidden behind a lazy-load attribute or served in multiple resolutions.
Logging URLs: Finally, it logs each image URL to the console, neatly numbered for easy reference. This way, you can quickly copy and use them as you wish.
Conclusion: The Power of DevTools
With just a few clicks and a simple script, you can unlock a treasure trove of image URLs from any website. This method not only saves you time but also gives you a deeper insight into how websites manage their visual content. Whether you're a developer, designer or just a curious mind, this technique will definitely come in handy.
So, the next time you find yourself mesmerized by the visuals on a webpage, remember you now have the power to capture it all in just a few seconds!
That’s all for today.
Thanks for reading.
For more content like this, click here.
You can also follow me on X(Twitter) for daily web development tips.
Keep Coding!!
Subscribe to my newsletter
Read articles from AARYAN BAJAJ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
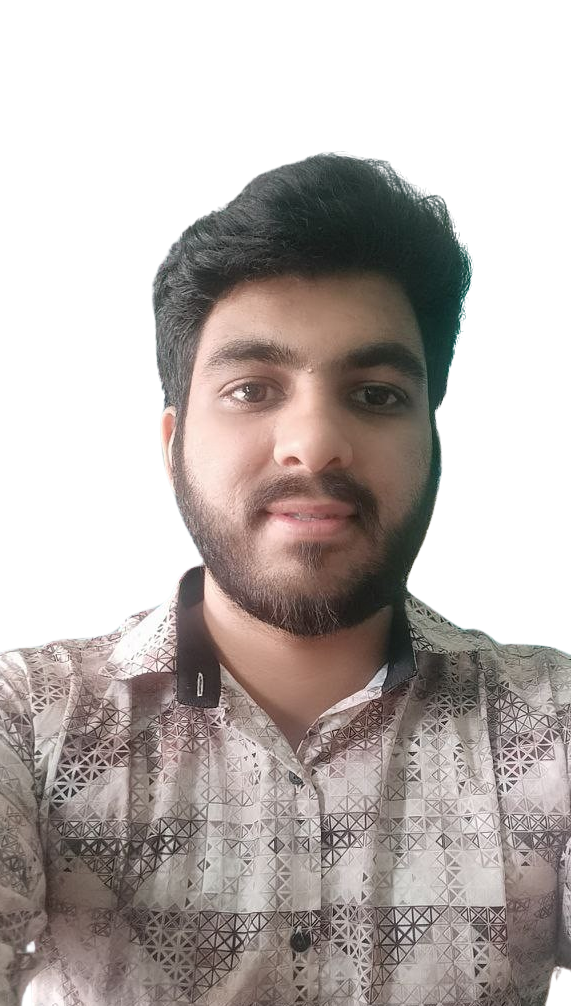
AARYAN BAJAJ
AARYAN BAJAJ
Hi Everyone 👋🏻 , I'm Aaryan Bajaj , a Full Stack Web Developer from India(🇮🇳). I share my knowledge through engaging technical blogs on Hashnode, covering web development.