Exploring Python’s zip() Function: Efficiently Pairing Iterables 🔗🐍

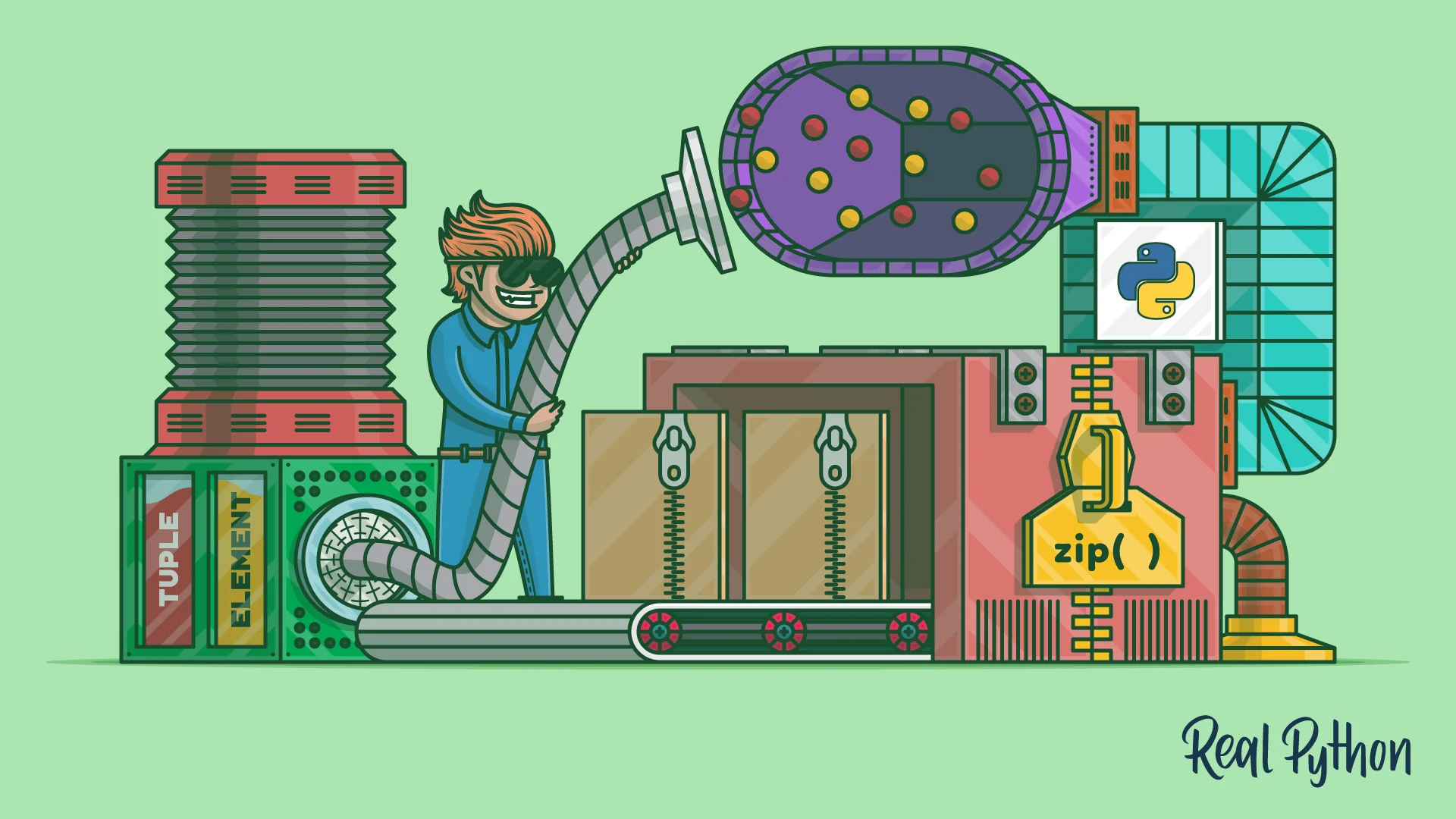
Introduction
Python is packed with useful built-in functions that simplify coding tasks, and one of these is the zip()
function. zip()
allows you to take two or more iterables—like lists, tuples, or strings—and combine them into a single iterable of tuples, pairing corresponding elements. It’s a versatile tool that can save time and make your code cleaner. In this blog, we’ll dive into the workings of the zip()
function and explore various practical use cases.
How Does zip()
Work?
The zip()
function takes multiple iterables as arguments and returns an iterator of tuples, where each tuple contains elements from the iterables at the same position.
Basic Usage
Let’s start with a simple example:
In this example, zip()
pairs elements from list1
and list2
into tuples, resulting in a list of paired tuples.
Handling Different Lengths
When the iterables passed to zip()
are of different lengths, zip()
stops creating tuples once the shortest iterable is exhausted.
If you need to handle cases where iterables have different lengths, you might want to use the itertools.zip
_longest()
function from the itertools
module.
Practical Applications of zip()
The zip()
function is highly useful in various scenarios. Let’s explore some common use cases.
1. Looping Over Multiple Iterables
If you need to iterate over multiple lists simultaneously, zip()
makes it straightforward:
This example pairs each name with its corresponding score and prints them together.
2. Unzipping a List of Tuples
You can also use zip()
to unzip a list of tuples back into individual lists:
Here, the *
operator unpacks the list of tuples, and zip()
recombines the elements into separate tuples.
3. Creating Dictionaries
You can create dictionaries from two lists using zip()
:
This is a quick and readable way to pair keys and values into a dictionary.
4. Simultaneous Sorting
Another interesting application is sorting one list based on the values of another:
In this example, zip()
pairs scores with names, sorts the pairs by scores, and then unzips them into sorted lists.
Using zip()
with More Than Two Iterables
The zip()
function isn’t limited to two iterables—you can pass as many as you like:
Each tuple will contain one element from each iterable, making it easy to group related data.
Subscribe to my newsletter
Read articles from Shrey Dikshant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shrey Dikshant
Shrey Dikshant
Aspiring data scientist with a strong foundation in adaptive quality techniques. Gained valuable experience through internships at YT Views, focusing on operation handling. Proficient in Python and passionate about data visualization, aiming to turn complex data into actionable insights.