Chapter - 1

Table of contents
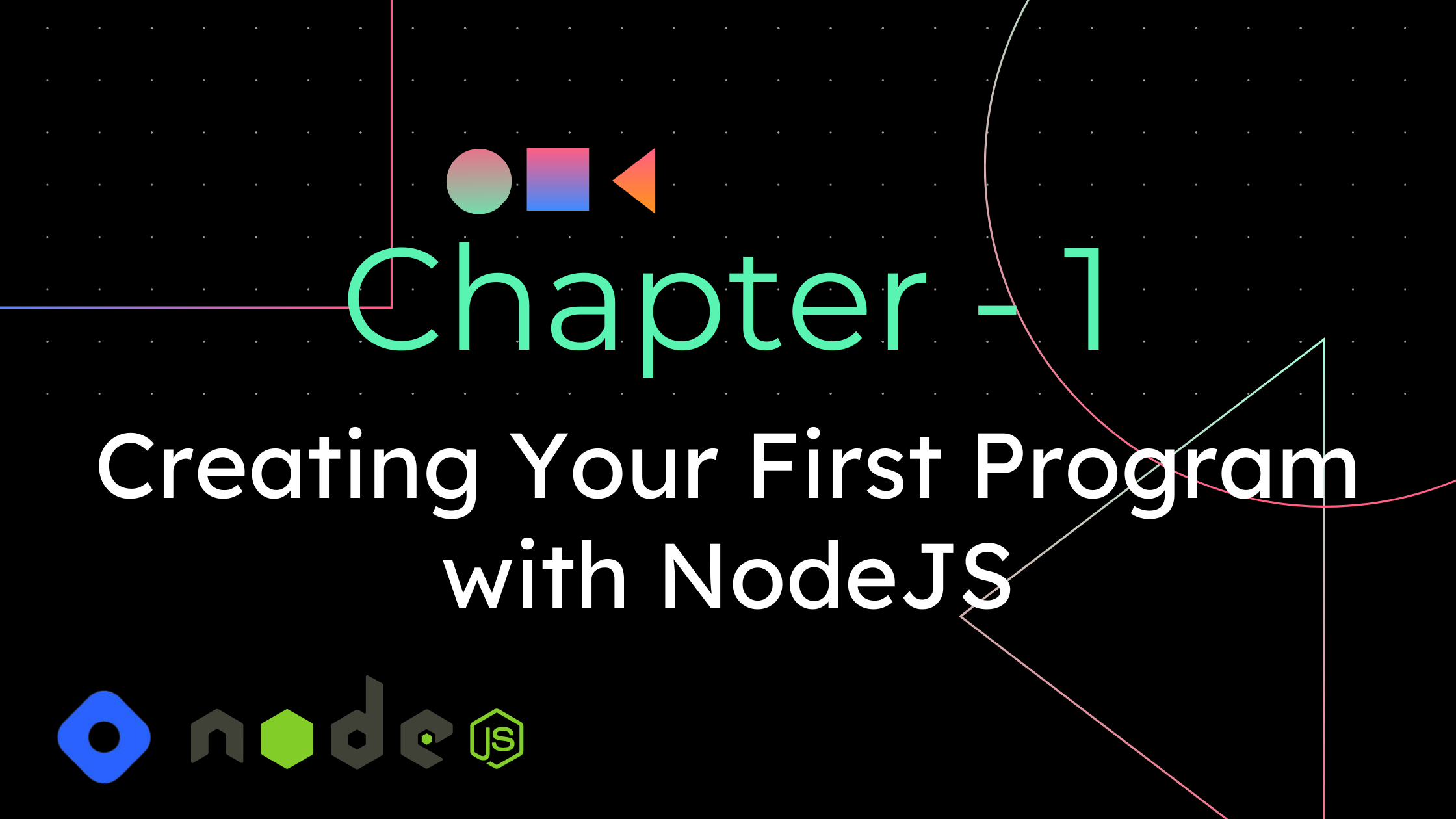
To initialize the Node JS project, run the following command in the terminal:
$ npm init
It will prompt you some questions (name, version, description, entry point, etc.). You can either answer them or press Enter
to accept the defaults.
At the end, it will generate a package.json
file that looks something like this:
{
"name": "my-node-project",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": // in this we can also add our own scripts
{
"start":"node index.js" //eg -> now we canrun our file using npm start command
"test": "echo "Error: no test specified" && exit 1"
},
"author": "",
"license": "ISC"
}
If you want to skip all prompts and create a package.json
with default values, use:
npm init -y
The default entry point for a Node.js project is index.js
, as specified in the package.json
file ("main": "index.js"
).
Create this file in your project directory
Add some basic code to the index.js
file, like:
console.log("Hello, Node.js!");
To run the project, use the following command:
node inde.js
or npm start
(added in the scripts in package.json earlier)
Modules in NodeJS
In Node, we do modular programming that allows you to organize your code into reusable pieces. A module in Node.js is essentially a JavaScript file that can export variables, functions, objects, or classes for use in other files.
Core Modules: These are modules provided by Node.js itself. They are built-in and do not require installation.
Examples:
fs
: (File System): Handles file operations.http
: Used to create HTTP servers and clients.path
: Provides utilities for working with file and directory paths.os
: Provides information about the operating system.
Local Modules:
These are custom modules that you create within your Node.js project. They usually contain code to be reused in different parts of your application.
Any JavaScript file in your project can be a module by exporting values or functions.
Creating a Local Module:
// File: myModule.js function greet(name) { return `Hello, ${name}!`; } module.exports = greet; // Export the function
Using a Local Module:
// File: app.js const greet = require('./myModule'); // Import the local module console.log(greet('Kartik')); // Outputs: Hello, Kartik!
Third-Party Modules (npm modules):
These are modules created by the community and shared via npm (Node Package Manager). You can install these modules using npm and use them in your project.
Examples:
express
: A web framework for building web applications.lodash
: A utility library that provides helpful functions for working with arrays, objects, etc.mongoose
: An ODM (Object Data Modeling) library for MongoDB.Installing and using a third-party module:
npm install express
const express = require('express'); // Import the installed module const app = express(); // Create an instance of an Express application app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(8000, () => { console.log('Server running on port 3000'); });
Exporting modules:
We can export the modules in two ways:
module.exports = add
module.exports = sub // now we can use only sub because it wil overwrite add
To prevent the overwriting:
module.exports = {
add,
sub,
}; // now we can use both add and sub
We can export the fn without making them separately:
exports.add = (a,b) => a+b
exports.sub = (a,b) => a-b
// These are now anonymous functions because we do not have given them any name
Importing modules:
Importing local module:
const math = require('path./math')
Importing built-in and third-party modules:
const fs = require('fs') // used in file system -> built-in
const express = require('express') // express module -> third-party
Handling files in Node JS
To handle the files we have to use the fs
module.
The fs
module is powerful and essential for handling file-related tasks in Node.js, making it a key tool for any server-side application.
To import fs
module:
const fs = require('fs');
There are two types of methods:
Synchronous Methods: These methods block the execution of your code until the operation is complete. They are easier to understand but can slow down your application if used excessively.
Asynchronous Methods: This allows your program to continue executing while the file operation is performed in the background. They use callbacks or Promises to handle the result.
Commonly used functions:
Reading Files:
// Asynchronous: const fs = require('fs'); fs.readFile('example.txt', 'utf8', (err, data) => { if (err) { console.error(err); return; } console.log(data); });
fs.readFile
reads the contents of a file asynchronously. The callback is called with an error (if any) and the data from the file.// Synchronous: const fs = require('fs'); try { const data = fs.readFileSync('example.txt', 'utf8'); console.log(data); } catch (err) { console.error(err); }
fs.readFileSync
reads the file synchronously and returns the data directly. If an error occurs, it must be caught usingtry-catch
.Writing Files:
// Asynchronous: const fs = require('fs'); const content = 'This is some content'; fs.writeFile('example.txt', content, err => { if (err) { console.error(err); return; } console.log('File written successfully'); });
fs.writeFile
writes data to a file asynchronously. If the file does not exist, it is created. The callback is executed after the file is written.
// Synchronous:
const fs = require('fs');
const content = 'This is some content';
try {
fs.writeFileSync('example.txt', content);
console.log('File written successfully');
} catch (err) {
console.error(err);
}
fs.writeFileSync
writes data to a file synchronously. It blocks the code execution until the operation is complete.
Appending to Files:
// Asynchronous: const fs = require('fs'); const content = 'This content will be appended'; fs.appendFile('example.txt', content, err => { if (err) { console.error(err); return; } console.log('Content appended successfully'); });
fs.appendFile
appends data to the end of a file asynchronously. If the file does not exist, it is created.// Synchronous: const fs = require('fs'); const content = 'This content will be appended'; try { fs.appendFileSync('example.txt', content); console.log('Content appended successfully'); } catch (err) { console.error(err); }
fs.appendFileSync
appends data to a file synchronously.
Deleting Files:
// Asynchronous: const fs = require('fs'); fs.unlink('example.txt', err => { if (err) { console.error(err); return; } console.log('File deleted successfully'); });
fs.unlink
deletes a file asynchronously. The callback is executed after the file is deleted.// Synchronous: const fs = require('fs'); try { fs.unlinkSync('example.txt'); console.log('File deleted successfully'); } catch (err) { console.error(err); }
fs.unlinkSync
deletes a file synchronously.Renaming Files:
// Asynchronous: const fs = require('fs'); fs.rename('oldname.txt', 'newname.txt', err => { if (err) { console.error(err); return; } console.log('File renamed successfully'); });
fs.rename
renames a file asynchronously. The callback is executed after the file is renamed.// Synchronous: const fs = require('fs'); try { fs.renameSync('oldname.txt', 'newname.txt'); console.log('File renamed successfully'); } catch (err) { console.error(err); }
fs.renameSync
renames a file synchronously.Checking if a File Exists:
// Asynchronous: const fs = require('fs'); fs.access('example.txt', fs.constants.F_OK, (err) => { console.log(`${err ? 'File does not exist' : 'File exists'}`); });
fs.access
check if a file exists and if the program has permission to read, write, or execute it. In this example,F_OK
check if the file exists.// Synchronous: const fs = require('fs'); try { fs.accessSync('example.txt', fs.constants.F_OK); console.log('File exists'); } catch (err) { console.log('File does not exist'); }
fs.accessSync
does the same check synchronously.Working with Directories:
Creating a Directory:
fs.mkdir('newDir', { recursive: true }, (err) => { if (err) throw err; console.log('Directory created'); });
fs.mkdir
creates a new directory. Therecursive: true
option allows creating nested directories if they don't exist.Reading a Directory:
fs.readdir('someDir', (err, files) => { if (err) throw err; console.log(files); });
fs.readdir
reads the contents of a directory and returns an array of filenames.Removing a Directory:
fs.rmdir('newDir', (err) => { if (err) throw err; console.log('Directory removed'); });
fs.rmdir
removes an empty directory.
Subscribe to my newsletter
Read articles from Kartik Sangal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
