Why Do Developers Prefer call? A Deep Dive into Ethereum's Send, Transfer, and Call Functions
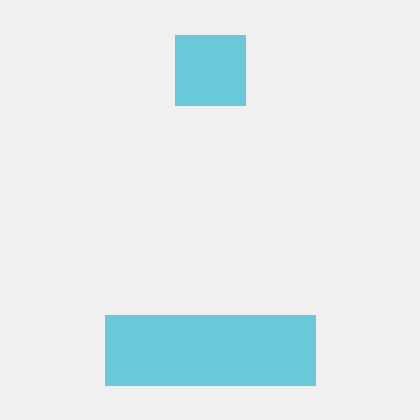
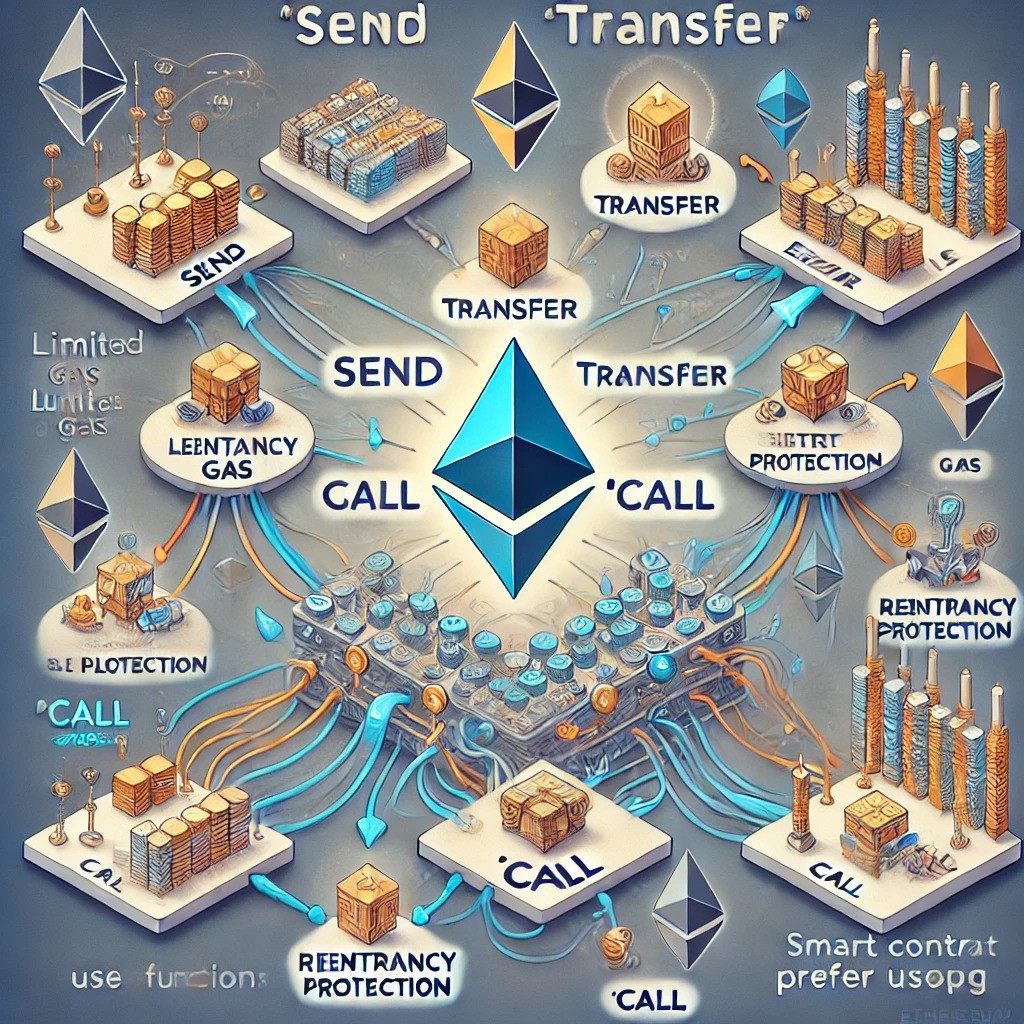
When working with Ethereum smart contracts, it's crucial to understand the nuances of different functions available for transferring Ether. The three primary methods used for sending Ether between contracts are send
, transfer
, and call
. While they might seem similar at first glance, each has distinct characteristics and use cases that developers need to consider when designing and interacting with smart contracts. In this article, we will explore the differences between send
, transfer
, and call
, and explain why call
is often preferred in modern smart contract development.
The Basics of Ether Transfers in Smart Contracts
Before diving into the differences, let's briefly review what these functions are designed to do. In Ethereum, smart contracts often need to transfer Ether to other contracts or externally owned accounts (EOAs). This transfer can be executed using one of the three methods:
send
: A lower-level function that sends a fixed amount of gas with the transaction.transfer
: A safer method that also sends a fixed amount of gas but reverts if the transaction fails.call
: A more flexible and lower-level function that allows for arbitrary data and gas amounts to be sent, offering greater control over the transaction.
Detailed Comparison
1. Send
The send
function is a low-level function that transfers Ether but does so with a significant limitation: it only forwards 2300 gas units to the receiving contract. This gas amount is sufficient for basic operations but not for more complex tasks. If the receiving contract's fallback or receive function requires more gas than the provided 2300 units, the transaction will fail, but send
will return false
instead of reverting the transaction. This behavior requires the developer to explicitly handle failures, which can lead to vulnerabilities if not properly managed.
Key Characteristics:
Sends 2300 gas units.
Returns
false
on failure.Requires manual handling of failure cases.
Use Cases:
Suitable for contracts where the receiving function is simple and does not require much gas.
Historically used in simple payment scenarios.
Example Code for
send
The
send
function sends Ether but requires manual handling of failure cases.pragma solidity ^0.8.0; contract ExampleSend { function sendEther(address payable recipient) public payable { // Sending Ether with the send function bool success = recipient.send(msg.value); // Handle failure case if (!success) { // Optionally revert the transaction or handle the failure revert("Send failed"); } } }
Explanation:
The
sendEther
function sends the amount of Ether passed inmsg.value
to therecipient
.If the transaction fails (e.g., due to running out of gas), the
success
variable will befalse
.The failure is handled by explicitly checking the
success
variable, and optionally reverting the transaction or taking other actions.
The main drawback of using the send
function in Ethereum smart contracts is its limited gas allowance and the resulting manual failure handling. Here are the specific cons associated with send
:
1. Limited Gas Allowance (2300 Gas)
Description: The
send
function only forwards 2300 gas units to the receiving contract. This amount of gas is sufficient for basic operations like logging events or updating a single state variable but is not enough for more complex logic.Impact: If the receiving contract's fallback or receive function requires more than 2300 gas units, the operation will fail. This makes
send
unsuitable for interacting with contracts that perform more complex operations on receiving Ether.
2. Manual Failure Handling
Description: When
send
fails (e.g., due to insufficient gas or other reasons), it does not automatically revert the transaction. Instead, it returnsfalse
, and it is up to the developer to check this return value and handle the failure appropriately.Impact: This can lead to potential vulnerabilities if the developer forgets to or improperly handles the failure. For example, funds might not be correctly refunded, or the contract might end up in an unexpected state if the failure is not adequately managed.
3. Less Safe Compared to Transfer
Description: Since
send
requires manual handling of failures, it is inherently less safe thantransfer
, which automatically reverts the transaction upon failure.Impact: This increases the risk of bugs and security issues, especially in complex contracts or situations where Ether transfers are a critical part of the contract's logic.
4. Deprecated Usage in Modern Contracts
Description: With the emergence of more complex decentralized applications (dApps) and the need for more reliable Ether transfers, the use of
send
has become less common. Modern best practices favor usingcall
for its flexibility ortransfer
for its safety, depending on the scenario.Impact: Relying on
send
in modern contract development may be seen as outdated or risky, especially as the ecosystem evolves and better alternatives are available.
Summary of Send
Drawbacks:
Gas Limitation: Only provides 2300 gas, insufficient for complex operations.
Manual Failure Handling: Developers must explicitly handle the possibility of failure.
Increased Risk: Higher potential for bugs or vulnerabilities due to manual error handling.
Less Preferred in Modern Development: Considered outdated and less reliable compared to
call
ortransfer
.
2. Transfer
The transfer
function is similar to send
but with a crucial difference: it reverts the transaction if the transfer fails. Like send
, it also forwards only 2300 gas units to the receiving contract. This feature makes transfer
a safer option because it avoids scenarios where a transfer failure could go unnoticed, potentially leading to unexpected contract states.
Key Characteristics:
Sends 2300 gas units.
Reverts on failure.
Safer than
send
due to automatic failure handling.
Use Cases:
Preferred in scenarios where failure should immediately halt the transaction, preventing further execution.
Used in contracts where safety and simplicity are prioritized.
Example Code for
transfer
The
transfer
function is similar tosend
, but it automatically reverts the transaction if it fails, making it safer and easier to use.pragma solidity ^0.8.0; contract ExampleTransfer { function transferEther(address payable recipient) public payable { // Sending Ether with the transfer function recipient.transfer(msg.value); // No need to handle failure explicitly as transfer reverts automatically on failure } }
Explanation:
The
transferEther
function sends the amount of Ether inmsg.value
to therecipient
.If the transfer fails (e.g., due to insufficient gas), the transaction automatically reverts, ensuring that the failure is handled without requiring additional code.
The transfer
function, while safer than send
, also has its own cons. Drawbacks of using the transfer
function in Ethereum smart contracts:
1. Fixed Gas Limit (2300 Gas)
Description: Like
send
, thetransfer
function forwards a fixed amount of 2300 gas units to the receiving contract.Impact: This gas limit is intended to prevent reentrancy attacks by restricting the complexity of the operations that can be performed in the receiving contract's fallback or receive function. However, it also limits the receiving contract's ability to execute more complex logic. If the receiving contract needs more gas to complete its operations, the transaction will fail and revert.
2. Lack of Flexibility
Description: The
transfer
function does not allow developers to specify the amount of gas to forward with the transaction.Impact: This lack of flexibility can be a significant limitation when interacting with contracts that require more gas for their execution. For example, if the receiving contract performs more than basic operations (such as calling other contracts, performing multiple state changes, or complex calculations), the transfer will fail due to insufficient gas.
3. Automatic Reversion on Failure
Description: While the automatic reversion on failure is generally a safety feature, it can be a drawback in certain scenarios.
Impact: In some cases, developers might prefer to handle failures more gracefully, such as by logging an event or taking alternative actions rather than having the entire transaction revert. The automatic reversion of
transfer
limits the developer's ability to implement custom error handling and fallback strategies.
4. Incompatibility with Some Contract Patterns
Description: The 2300 gas limit can cause compatibility issues with some contract patterns, especially as decentralized applications become more complex.
Impact: If a receiving contract is designed to perform more complex operations or interacts with other contracts upon receiving Ether, the use of
transfer
might lead to unexpected reverts, breaking the expected flow of the application.
5. Deprecated in Complex Scenarios
Description: As the Ethereum ecosystem has evolved,
transfer
is seen as less suitable for complex contract interactions. The emergence of thecall
function, which offers more control over gas and error handling, has madetransfer
less favored in advanced contract development.Impact: Using
transfer
in complex scenarios might be seen as outdated, especially when more robust and flexible options likecall
are available. This can lead to suboptimal design choices in modern contract development.
Summary of Transfer
Drawbacks:
Gas Limitation: Like
send
,transfer
is limited to 2300 gas, which is insufficient for complex operations.Lack of Flexibility: Developers cannot adjust the gas limit, limiting its use in scenarios requiring more than basic operations.
Automatic Reversion: While safer, it restricts the ability to handle failures in a custom manner.
Compatibility Issues: May not work well with more complex contract patterns or those requiring more gas.
Less Suitable for Modern Contracts: Viewed as less robust compared to
call
in advanced scenarios.
3. Call
The call
function is the most versatile and powerful of the three. It allows the developer to specify the exact amount of gas to forward and supports sending arbitrary data along with the Ether. This flexibility makes call
more suitable for complex interactions between contracts. Importantly, call
does not impose a strict gas limit, allowing receiving contracts to execute more complex logic without running out of gas.
However, call
introduces its own complexities. Since call
returns a boolean value indicating success or failure and does not automatically revert the transaction on failure, developers need to handle these scenarios manually. This increased responsibility comes with the benefit of having complete control over the transaction process.
Key Characteristics:
Can send an arbitrary amount of gas.
Allows sending data along with Ether.
Returns
true
on success andfalse
on failure, without reverting automatically.Requires careful handling of success and failure conditions.
Use Cases:
Ideal for interactions with other contracts that require complex execution logic.
Preferred in modern development due to its flexibility and ability to work around the 2300 gas limit imposed by
send
andtransfer
.Certainly! Let's add example code snippets for each of the functions (
send
,transfer
, andcall
) to illustrate how they work and when you might use them.Example Code for
call
The
call
function is more flexible, allowing you to specify the gas amount and send data along with the Ether. However, it requires careful handling of success and failure.pragma solidity ^0.8.0; contract ExampleCall { function callEther(address payable recipient) public payable { // Sending Ether with the call function (bool success, ) = recipient.call{value: msg.value, gas: 5000}(""); // Handle failure case if (!success) { // Optionally revert the transaction or handle the failure revert("Call failed"); } } function callWithFunction(address payable recipient, bytes memory data) public payable { // Sending Ether and calling a function on the recipient contract (bool success, ) = recipient.call{value: msg.value}(data); // Handle failure case if (!success) { // Optionally revert the transaction or handle the failure revert("Call with function failed"); } } }
Explanation:
The
callEther
function sends Ether to therecipient
with 5000 gas units, much more than the 2300 gas limit ofsend
andtransfer
.The success of the operation is determined by the
success
variable, and if the transaction fails, the code can handle it as needed.The
callWithFunction
function illustrates howcall
can be used to send Ether along with data, such as calling a specific function on the recipient contract. Again, the success is checked and handled appropriately.
Why Call
is More Preferred
In recent years, call
has become the preferred method for Ether transfers in smart contracts. This shift is primarily due to the increasing complexity of decentralized applications (dApps) and the limitations imposed by the 2300 gas limit of send
and transfer
. Here’s why call
is favored:
Flexibility:
Call
allows developers to specify the exact amount of gas needed for the transaction, which is crucial for contracts that require more complex execution logic. This flexibility helps avoid failures due to gas limitations.Safety: Although
call
does not automatically revert on failure, its ability to handle custom logic allows developers to implement safer, more sophisticated error handling mechanisms. By carefully checking the returned boolean value, developers can ensure that failures are managed appropriately.Gas Considerations: With the increased complexity of smart contracts, 2300 gas units are often insufficient, leading to failed transactions when using
send
ortransfer
.Call
does not impose this limitation, making it more reliable for modern applications.Future-Proofing: As Ethereum evolves, gas costs and contract complexities are likely to increase. Using
call
prepares contracts for future changes by allowing them to adapt to varying gas requirements.
Understanding the differences between send
, transfer
, and call
is essential for any Ethereum developer. While send
and transfer
offer simplicity and safety for basic transfers, their rigid gas limits make them less suitable for more complex operations. Call
, on the other hand, provides the flexibility and control needed for modern smart contracts, making it the preferred choice for most developers today. However, with this flexibility comes the responsibility to carefully handle errors and ensure contract security, which is why developers must thoroughly understand the implications of each method when designing their smart contracts.
By choosing the appropriate function based on the specific needs of your contract, you can ensure efficient, secure, and reliable Ether transfers within your decentralized applications.
Subscribe to my newsletter
Read articles from Samuel Aleonomoh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
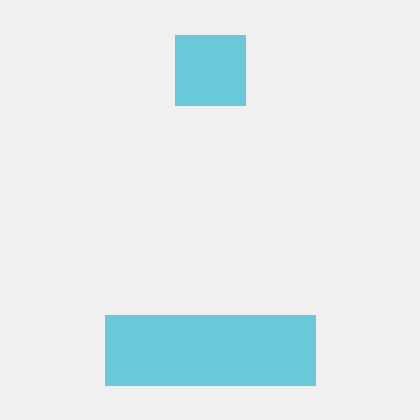