Implementing Microservices Architecture with ASP.NET Core Web APIs
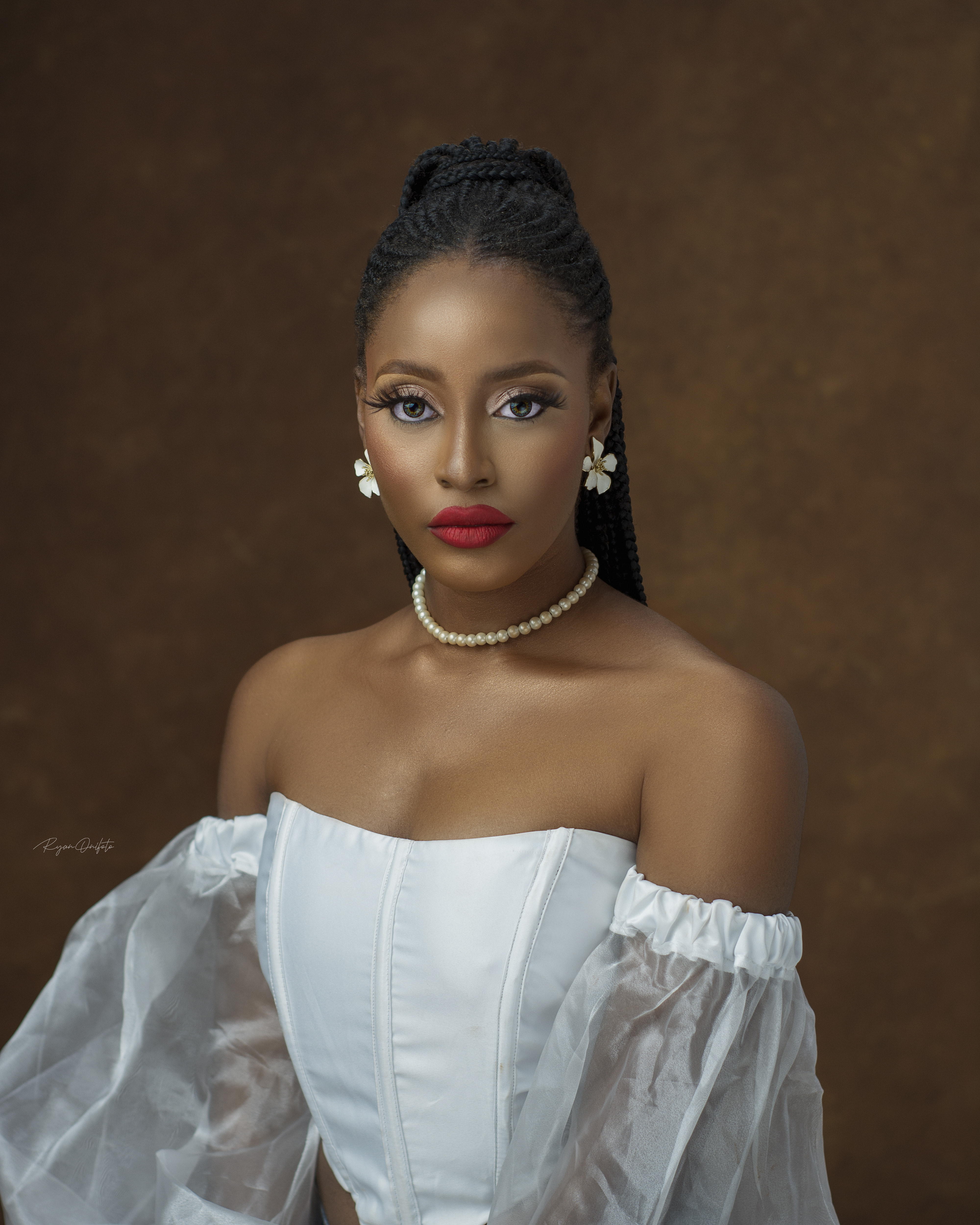
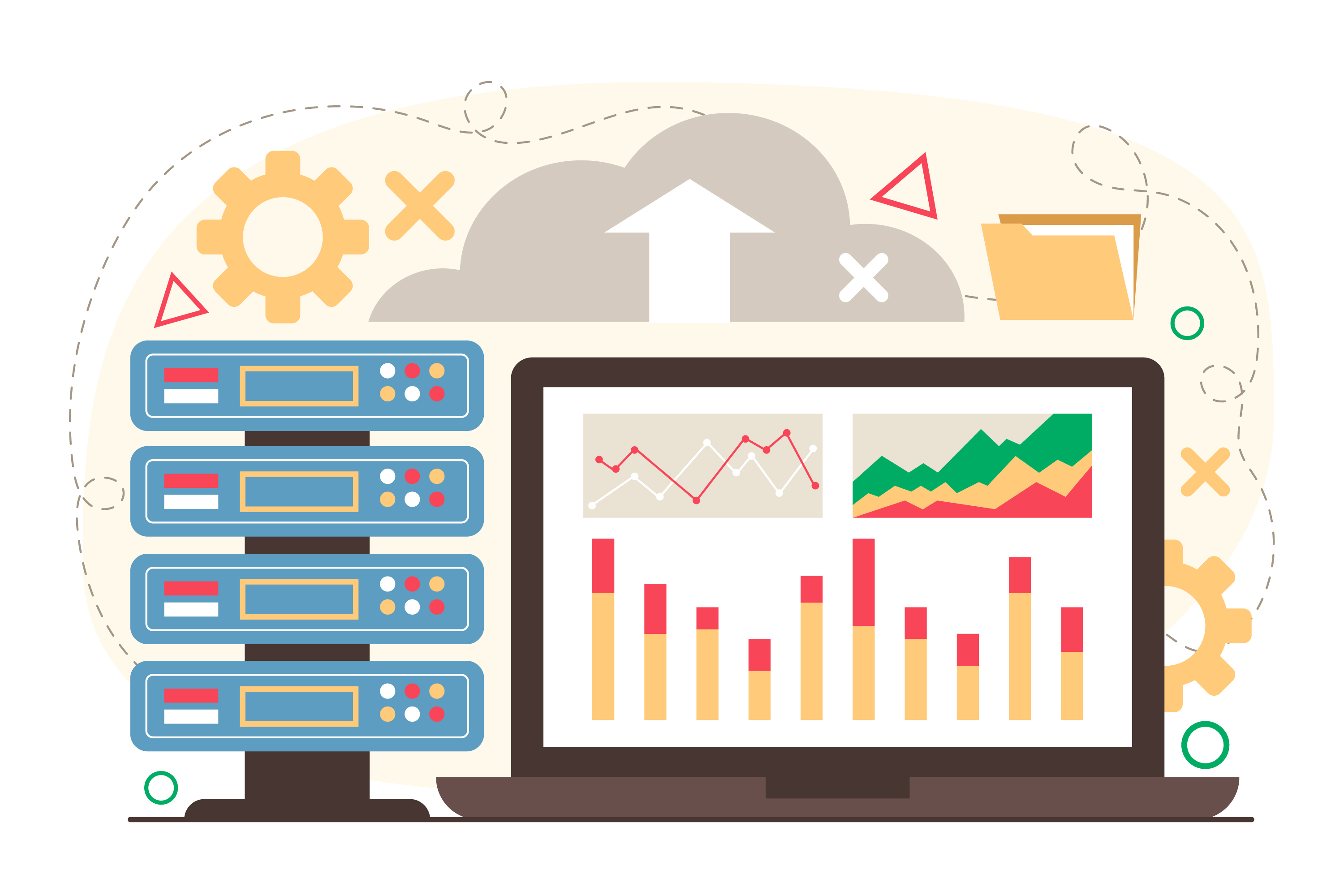
Hello! Have you ever wondered what a microservice architecture is or how to use Web APIs for microservices? Don’t worry—you're in the right place!
Think of a microservices architecture as a big machine made up of many small, specialized parts. Each part works independently without relying too much on the others. In software, these parts are called "microservices."
Pre-requisites
To fully benefit from this article, readers should have the following prerequisites:
Basic Understanding of C# and .NET Core
- Familiarity with C# syntax, object-oriented programming concepts, and the .NET Core framework.
Experience with ASP.NET Core Web APIs
- Ability to create and work with ASP.NET Core Web API projects, including routing, controllers, and dependency injection.
Knowledge of RESTful APIs
- Understanding of REST principles, HTTP methods (GET, POST, PUT, DELETE), and how to design and consume RESTful APIs.
Fundamental Concepts of Distributed Systems
- Awareness of what distributed systems are, including basic concepts like network communication, latency, and fault tolerance.
Familiarity with Docker and Containerization (Optional but Recommended)
- Understanding how to containerize applications using Docker, as this is often used in microservices architecture.
Basic Knowledge of Databases
- Experience working with relational databases (e.g., SQL Server) and basic CRUD operations.
Introduction to Cloud Services (Optional)
- Awareness of cloud platforms (e.g., Azure, AWS) and their role in hosting and scaling microservices.
Table of Contents
Introduction to Microservices Architecture
Setting Up Your ASP.NET Core Project
Decoupling Monolithic Applications into Microservices
Design Patterns for Communication Between Microservices
Challenges and Best Practices for Managing Microservices at Scale
Testing and Debugging Microservices
Conclusion and Further Resources
Introduction to Microservices Architecture
What is Microservices Architecture?
Microservices architecture is a way of designing software where an application is broken down into smaller, independent services, each responsible for a specific function. Imagine a big machine made up of many small, specialized parts, where each part can work on its own without depending too much on others. In the world of software, these parts are called "microservices."
Each microservice in this architecture does one thing well and communicates with other microservices through simple, well-defined channels, usually over the internet using APIs. This approach makes it easier to develop, manage, and scale large applications because you can focus on improving or fixing one microservice at a time without affecting the others.
Benefits of Using Microservices
Flexibility in Development:
- Developers can work on different microservices independently, allowing teams to develop, test, and deploy features faster.
Scalability:
- Since each microservice is independent, you can scale specific parts of your application without needing to scale the entire system. This means you can allocate resources where they are most needed.
Resilience:
- If one microservice fails, it doesn’t bring down the entire application. Other services can continue to run, reducing the risk of complete system failures.
Technology Diversity:
- Different microservices can use different technologies or programming languages, enabling you to choose the best tool for each specific job.
Easier Maintenance and Updates:
- With microservices, you can update, fix, or improve one service without disrupting the whole application. This makes maintenance simpler and reduces downtime.
Microservices architecture offers a modern approach to building applications that are easier to manage, scale, and adapt to changing needs.
Setting Up Your ASP.NET Core Project
In this section, we'll walk through creating a new ASP.NET Core Web API project and installing the necessary packages and tools. By the end, you'll have a basic project set up and ready for implementing microservices.
Creating a New ASP.NET Core Web API Project
First, we'll create a new ASP.NET Core Web API project using Visual Studio. If you're using Visual Studio Code or the .NET CLI, the steps will be slightly different but still easy to follow.
Step 1: Open Visual Studio
- Launch Visual Studio and select "Create a new project" from the start screen.
Step 2: Choose the ASP.NET Core Web API Template
In the project templates, search for "ASP.NET Core Web API" and select it.
Click Next to continue.
Step 3: Configure Your Project
Give your project a name (e.g.,
MicroservicesDemo
).Choose a location to save your project.
Click Next to proceed.
Step 4: Select Target Framework
Choose
.NET 7.0
(or the latest stable version).Leave the other options as default and click Create.
Visual Studio will now create a new ASP.NET Core Web API project for you, with some basic starter code already in place.
Code Sample: Basic WeatherForecast
API
When your project is created, you'll find a WeatherForecastController
with some sample code:
[ApiController]
[Route("[controller]")]
public class WeatherForecastController : ControllerBase
{
private static readonly string[] Summaries = new[]
{
"Freezing", "Bracing", "Chilly", "Cool", "Mild", "Warm", "Balmy", "Hot", "Sweltering", "Scorching"
};
private readonly ILogger<WeatherForecastController> _logger;
public WeatherForecastController(ILogger<WeatherForecastController> logger)
{
_logger = logger;
}
[HttpGet]
public IEnumerable<WeatherForecast> Get()
{
return Enumerable.Range(1, 5).Select(index => new WeatherForecast
{
Date = DateTime.Now.AddDays(index),
TemperatureC = Random.Shared.Next(-20, 55),
Summary = Summaries[Random.Shared.Next(Summaries.Length)]
})
.ToArray();
}
}
This is a simple API that returns weather data. You can run the project and test this API by pressing F5
or clicking the Run button.
Installing Necessary Packages and Tools
Next, let's install some packages that you'll need as you develop your microservices.
Step 1: Open the NuGet Package Manager
Right-click on the project in Solution Explorer.
Select Manage NuGet Packages.
Step 2: Install the Required Packages
Search for and install the following packages:
Swashbuckle.AspNetCore: This package allows you to add Swagger support, which provides a UI for testing your APIs.
dotnet add package Swashbuckle.AspNetCore
Microsoft.EntityFrameworkCore: This is required if you plan to use a database with your microservices.
dotnet add package Microsoft.EntityFrameworkCore.SqlServer
Polly: This package is useful for handling transient faults and adding resilience to your microservices.
dotnet add package Polly
Step 3: Update Your Project to Use Swagger
To enable Swagger, modify the Startup.cs
or Program.cs
file, depending on your project setup:
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddSwaggerGen();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
app.UseSwagger();
app.UseSwaggerUI(c => c.SwaggerEndpoint("/swagger/v1/swagger.json", "MicroservicesDemo v1"));
}
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
}
Step 4: Run Your Project with Swagger
Press
F5
to run your project.You'll see the Swagger UI at
http://localhost:5000/swagger
, where you can test your API endpoints.
You've successfully set up your ASP.NET Core Web API project, installed necessary packages, and configured Swagger for API testing. Now you're ready to start implementing microservices.
Decoupling Monolithic Applications into Microservices
Monolithic applications are single, large applications where all components are tightly integrated. This can lead to challenges as the application grows. Microservices break down this large application into smaller, independent services that communicate with each other.
Identifying Monolithic Components
Monolithic Component Example: Imagine you have an application that handles user management, product management, and order processing all in one codebase.
// Monolithic Controller Example
public class MainController : ControllerBase
{
[HttpGet("users")]
public IActionResult GetUsers() { /*...*/ }
[HttpGet("products")]
public IActionResult GetProducts() { /*...*/ }
[HttpGet("orders")]
public IActionResult GetOrders() { /*...*/ }
}
Identify Components: Look for distinct functionalities that can be separated. In the example above:
User Management
Product Management
Order Processing
Strategies for Breaking Down a Monolith
Step-by-Step Approach:
Define Boundaries: Determine what each microservice will handle. For instance:
UserService
for user managementProductService
for product managementOrderService
for order processing
Create Separate Projects: Create separate ASP.NET Core projects for each microservice.
Refactor Code: Move the relevant code into these projects.
Example: Original Monolithic Controller:
// UserService Example - Refactored
public class UserController : ControllerBase
{
[HttpGet]
public IActionResult GetUsers() { /*...*/ }
}
ProductService Example:
// ProductController Example - Refactored
public class ProductController : ControllerBase
{
[HttpGet]
public IActionResult GetProducts() { /*...*/ }
}
OrderService Example:
// OrderController Example - Refactored
public class OrderController : ControllerBase
{
[HttpGet]
public IActionResult GetOrders() { /*...*/ }
}
Creating Microservices from Existing Code
Steps to Create Microservices:
Extract and Create Projects: For each component identified (User, Product, Order), create a new ASP.NET Core Web API project.
Move Code: Move the relevant controllers and services to the new projects.
Set Up Communication: Implement communication between services, usually through HTTP APIs.
Example Communication Using HTTP:
UserService API:
// Fetch user details from ProductService
public async Task<Product> GetProductById(int id)
{
using (var client = new HttpClient())
{
var response = await client.GetAsync($"http://productservice/api/products/{id}");
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsAsync<Product>();
}
}
ProductService API:
[HttpGet("{id}")]
public IActionResult GetProduct(int id) { /*...*/ }
Summary
By breaking down a monolithic application into microservices:
Each service handles a specific task (e.g., user management).
Services communicate through APIs (e.g., HTTP requests).
Each microservice can be developed, deployed, and scaled independently.
This approach helps manage complexity and improves maintainability.
Design Patterns for Communication Between Microservices
When building a microservices architecture, you'll need to choose how your services communicate with each other. Here are some explanations and code samples for common design patterns used in microservices communication:
RESTful APIs and HTTP Communication
RESTful APIs are a popular way for microservices to communicate over HTTP. Each microservice exposes a set of endpoints that other services or clients can call using standard HTTP methods like GET, POST, PUT, and DELETE.
Example: Creating a Simple RESTful API
Let's say you have two microservices: OrderService and CustomerService.
OrderService - This service manages orders and needs to fetch customer details from CustomerService.
OrderController.cs in OrderService:
using Microsoft.AspNetCore.Mvc;
using System.Net.Http;
using System.Threading.Tasks;
[ApiController]
[Route("api/[controller]")]
public class OrderController : ControllerBase
{
private readonly HttpClient _httpClient;
public OrderController(HttpClient httpClient)
{
_httpClient = httpClient;
}
[HttpGet("{id}")]
public async Task<IActionResult> GetOrder(int id)
{
// Fetch customer details from CustomerService
var customerResponse = await _httpClient.GetStringAsync($"http://customerservice/api/customers/{id}");
// Process order and customer details
return Ok(new { OrderId = id, CustomerDetails = customerResponse });
}
}
CustomerController.cs in CustomerService:
using Microsoft.AspNetCore.Mvc;
[ApiController]
[Route("api/[controller]")]
public class CustomerController : ControllerBase
{
[HttpGet("{id}")]
public IActionResult GetCustomer(int id)
{
// Return dummy customer details
return Ok(new { CustomerId = id, Name = "John Doe" });
}
}
Note: For real-world scenarios, use configuration settings for service URLs and handle errors and retries.
Message Brokers and Asynchronous Communication
Message Brokers allow services to communicate asynchronously by sending messages to a queue. This helps to decouple services and manage communication without requiring real-time responses.
Example: Using RabbitMQ for Asynchronous Communication
ProducerService - Sends messages to the queue.
OrderProducer.cs:
using RabbitMQ.Client;
using System.Text;
public class OrderProducer
{
public void SendOrder(string orderDetails)
{
var factory = new ConnectionFactory() { HostName = "localhost" };
using (var connection = factory.CreateConnection())
using (var channel = connection.CreateModel())
{
channel.QueueDeclare(queue: "orderQueue",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null);
var body = Encoding.UTF8.GetBytes(orderDetails);
channel.BasicPublish(exchange: "",
routingKey: "orderQueue",
basicProperties: null,
body: body);
}
}
}
ConsumerService - Receives messages from the queue.
OrderConsumer.cs:
using RabbitMQ.Client;
using RabbitMQ.Client.Events;
using System;
using System.Text;
public class OrderConsumer
{
public void StartConsuming()
{
var factory = new ConnectionFactory() { HostName = "localhost" };
using (var connection = factory.CreateConnection())
using (var channel = connection.CreateModel())
{
channel.QueueDeclare(queue: "orderQueue",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null);
var consumer = new EventingBasicConsumer(channel);
consumer.Received += (model, ea) =>
{
var body = ea.Body.ToArray();
var message = Encoding.UTF8.GetString(body);
Console.WriteLine("Received: {0}", message);
};
channel.BasicConsume(queue: "orderQueue",
autoAck: true,
consumer: consumer);
Console.WriteLine("Press [enter] to exit.");
Console.ReadLine();
}
}
}
Service Discovery and API Gateways
Service Discovery helps microservices locate each other dynamically. API Gateways provide a single entry point for clients to interact with multiple microservices, handling tasks like routing, load balancing, and security.
Example: Using Consul for Service Discovery
Registering Services with Consul:
using Consul;
public class ServiceRegistration
{
public void RegisterService()
{
var client = new ConsulClient();
var registration = new AgentServiceRegistration()
{
ID = "order-service",
Name = "order-service",
Address = "localhost",
Port = 5000
};
client.Agent.ServiceRegister(registration).Wait();
}
}
API Gateway using Ocelot: In ocelot.json
configuration file:
{
"ReRoutes": [
{
"DownstreamPathTemplate": "/api/orders/{everything}",
"DownstreamScheme": "http",
"DownstreamHostAndPorts": [
{
"Host": "localhost",
"Port": 5000
}
],
"UpstreamPathTemplate": "/orders/{everything}",
"UpstreamHttpMethod": [ "Get" ]
}
],
"GlobalConfiguration": {
"BaseUrl": "http://localhost:5001"
}
}
In Startup.cs
:
public void ConfigureServices(IServiceCollection services)
{
services.AddOcelot();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseOcelot().Wait();
}
These examples cover basic concepts of communication patterns in microservices. As you advance, you might explore more complex scenarios and tools, but these provide a solid foundation.
Challenges and Best Practices for Managing Microservices at Scale
Handling Inter-Service Communication Failures
Challenge: When you have multiple microservices interacting with each other, there's a chance that one service might fail or become unreachable. Handling these failures gracefully is crucial to maintaining a reliable system.
Best Practice: Use Retry and Circuit Breaker Patterns
Retry Pattern: Automatically retry failed requests a few times before giving up.
Circuit Breaker Pattern: Stop making requests to a failing service and retry only after a timeout period.
Code Sample: Retry Pattern with Polly
using Polly;
using System.Net.Http;
public class MyServiceClient
{
private readonly HttpClient _httpClient;
public MyServiceClient(HttpClient httpClient)
{
_httpClient = httpClient;
}
public async Task<string> GetDataAsync()
{
var policy = Policy
.Handle<HttpRequestException>()
.WaitAndRetryAsync(
retryCount: 3,
sleepDurationProvider: retryAttempt => TimeSpan.FromSeconds(Math.Pow(2, retryAttempt)),
onRetry: (exception, timeSpan, retryCount, context) =>
{
Console.WriteLine($"Retry {retryCount} due to: {exception.Message}");
});
return await policy.ExecuteAsync(() => _httpClient.GetStringAsync("http://example.com/data"));
}
}
Code Sample: Circuit Breaker with Polly
using Polly;
using System.Net.Http;
public class MyServiceClient
{
private readonly HttpClient _httpClient;
public MyServiceClient(HttpClient httpClient)
{
_httpClient = httpClient;
}
public async Task<string> GetDataAsync()
{
var policy = Policy
.Handle<HttpRequestException>()
.CircuitBreakerAsync(
handledEventsAllowedBeforeBreaking: 2,
durationOfBreak: TimeSpan.FromMinutes(1),
onBreak: (exception, duration) =>
{
Console.WriteLine($"Circuit broken due to: {exception.Message}");
},
onReset: () =>
{
Console.WriteLine("Circuit reset");
});
return await policy.ExecuteAsync(() => _httpClient.GetStringAsync("http://example.com/data"));
}
}
Monitoring and Logging Microservices
Challenge: With multiple microservices, tracking what’s happening across all services and identifying issues can become complex.
Best Practice: Use Centralized Logging and Monitoring Tools
Centralized Logging: Collect logs from all microservices into a central location for easier access and analysis.
Monitoring Tools: Use tools to visualize metrics and set up alerts for unusual behavior.
Code Sample: Basic Logging with Serilog
Install Serilog via NuGet:
dotnet add package Serilog dotnet add package Serilog.Sinks.Console
Configure Serilog in
Program.cs
:using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Hosting; using Serilog; public class Program { public static void Main(string[] args) { Log.Logger = new LoggerConfiguration() .WriteTo.Console() .CreateLogger(); CreateHostBuilder(args).Build().Run(); } public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .UseSerilog() .ConfigureWebHostDefaults(webBuilder => { webBuilder.UseStartup<Startup>(); }); }
Log messages in your services:
using Microsoft.Extensions.Logging; public class MyService { private readonly ILogger<MyService> _logger; public MyService(ILogger<MyService> logger) { _logger = logger; } public void DoWork() { _logger.LogInformation("Doing some work."); // Perform work here } }
Scaling and Load Balancing
Challenge: As traffic increases, your microservices need to handle more requests. Scaling and balancing the load are essential to prevent bottlenecks.
Best Practice: Use Load Balancers and Auto-Scaling
Load Balancers: Distribute incoming requests across multiple instances of your services.
Auto-Scaling: Automatically adjust the number of service instances based on traffic.
Code Sample: Load Balancing with Kubernetes (Conceptual)
Define a service in Kubernetes to balance the load:
apiVersion: v1 kind: Service metadata: name: myservice spec: selector: app: myservice ports: - protocol: TCP port: 80 targetPort: 8080
Define a deployment with multiple replicas:
apiVersion: apps/v1 kind: Deployment metadata: name: myservice spec: replicas: 3 selector: matchLabels: app: myservice template: metadata: labels: app: myservice spec: containers: - name: myservice image: myservice:latest ports: - containerPort: 8080
These practices and code samples should help beginners understand and handle common challenges in managing microservices.
Testing and Debugging Microservices
Unit Testing Microservices
Unit testing checks if individual parts of your microservice work correctly in isolation. Think of it as testing a single function or method to ensure it behaves as expected.
Example:
Suppose you have a microservice with a method that calculates the total price of an order. Here's a simple unit test for this method.
Code:
// OrderService.cs
public class OrderService
{
public decimal CalculateTotalPrice(decimal price, int quantity)
{
return price * quantity;
}
}
// OrderServiceTests.cs
using Xunit;
public class OrderServiceTests
{
[Fact]
public void CalculateTotalPrice_ShouldReturnCorrectValue()
{
// Arrange
var service = new OrderService();
decimal price = 10m;
int quantity = 5;
// Act
var result = service.CalculateTotalPrice(price, quantity);
// Assert
Assert.Equal(50m, result);
}
}
In this example:
OrderService
is the class being tested.CalculateTotalPrice_ShouldReturnCorrectValue
is a unit test that ensures theCalculateTotalPrice
method works correctly.
Tools:
- Use testing frameworks like xUnit or NUnit for writing and running tests.
Integration Testing Strategies
Integration testing checks how different parts of your microservices work together. This often involves testing the interactions between your microservice and external dependencies like databases or other services.
Example:
Suppose your microservice saves orders to a database. Here's a basic integration test that checks if an order is saved correctly.
Code:
// OrderServiceIntegrationTests.cs
using Xunit;
using Microsoft.Extensions.DependencyInjection;
using YourNamespace.Data;
using YourNamespace.Services;
public class OrderServiceIntegrationTests : IClassFixture<TestFixture>
{
private readonly OrderService _orderService;
private readonly ApplicationDbContext _context;
public OrderServiceIntegrationTests(TestFixture fixture)
{
_context = fixture.Context;
_orderService = new OrderService(_context);
}
[Fact]
public void SaveOrder_ShouldPersistOrderInDatabase()
{
// Arrange
var order = new Order { Price = 100m, Quantity = 2 };
// Act
_orderService.SaveOrder(order);
var savedOrder = _context.Orders.Find(order.Id);
// Assert
Assert.NotNull(savedOrder);
Assert.Equal(order.Price, savedOrder.Price);
Assert.Equal(order.Quantity, savedOrder.Quantity);
}
}
// TestFixture.cs
public class TestFixture : IDisposable
{
public ApplicationDbContext Context { get; private set; }
public TestFixture()
{
// Set up an in-memory database or test database
var options = new DbContextOptionsBuilder<ApplicationDbContext>()
.UseInMemoryDatabase("TestDatabase")
.Options;
Context = new ApplicationDbContext(options);
}
public void Dispose()
{
Context.Dispose();
}
}
In this example:
OrderServiceIntegrationTests
sets up an integration test to check if theOrderService.SaveOrder
method correctly saves an order to an in-memory database.
Tools:
- Use xUnit for testing, and In-Memory Database for testing database interactions.
Debugging Techniques
Debugging helps you find and fix issues in your code. Here are some common techniques:
Breakpoints: Pause the execution of your code at a specific line to inspect variables and the flow of execution.
Logging: Add logging statements to your code to track what’s happening at runtime.
Example:
Code with Logging:
// OrderService.cs
public class OrderService
{
private readonly ILogger<OrderService> _logger;
public OrderService(ILogger<OrderService> logger)
{
_logger = logger;
}
public decimal CalculateTotalPrice(decimal price, int quantity)
{
_logger.LogInformation($"Calculating total price for price: {price}, quantity: {quantity}");
return price * quantity;
}
}
Using Visual Studio Debugger:
Set a breakpoint by clicking in the left margin of the code editor next to the line where you want to pause.
Run your application in Debug mode.
When execution hits the breakpoint, use the Locals and Watch windows to inspect variable values and step through your code.
Tools:
Visual Studio or Visual Studio Code for debugging and setting breakpoints.
Serilog or NLog for logging.
Conclusion and Further Resources
Summary of Key Points
In this guide, we explored how to implement a microservices architecture using ASP.NET Core Web APIs. Here's a quick recap of what we covered:
Introduction to Microservices Architecture
- We learned that microservices involve breaking down a large application into smaller, independently deployable services that communicate with each other.
Decoupling Monolithic Applications
- We discussed how to identify parts of a monolithic application and transform them into separate microservices. For example, if you have a monolithic e-commerce application, you could split it into services like Product, Order, and Customer.
Design Patterns for Communication
We covered different ways microservices can communicate. For instance:
// Using HTTP for communication [HttpGet("products/{id}")] public IActionResult GetProduct(int id) { // Fetch product details }
Challenges and Best Practices
- Managing microservices can be challenging, but best practices include handling communication failures gracefully and monitoring your services for performance and issues.
Testing and Debugging
- We talked about how to test microservices to ensure they work correctly and how to debug issues when they arise.
Recommended Reading and Tools
To deepen your understanding and expand your skills, consider exploring the following resources:
Books:
"Microservices Patterns: With examples in Java" by Chris Richardson – Provides a comprehensive guide to microservices patterns and practices.
"Designing Data-Intensive Applications" by Martin Kleppmann – Offers insights into building scalable and reliable systems, which is useful for understanding microservices.
Online Courses:
Microsoft Learn - Introduction to Microservices – A free, interactive course on microservices fundamentals.
Udemy - Microservices with ASP.NET Core – A paid course with practical examples and hands-on exercises.
Tools:
Docker: Helps in containerizing your microservices to run them consistently across different environments. Docker Documentation
Postman: Useful for testing and debugging your API endpoints. Postman Documentation
Kubernetes: A powerful tool for managing containerized applications, especially in microservices architecture. Kubernetes Documentation
By using these resources and tools, you'll be well-equipped to dive deeper into microservices and improve your skills in building robust and scalable applications. I hope you found this guide helpful and learned something new. Stay tuned for the next article in the Mastering C# series: In-depth Analysis of C# 10 and .NET 6 Features
Happy coding!
Subscribe to my newsletter
Read articles from Opaluwa Emidowo-ojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
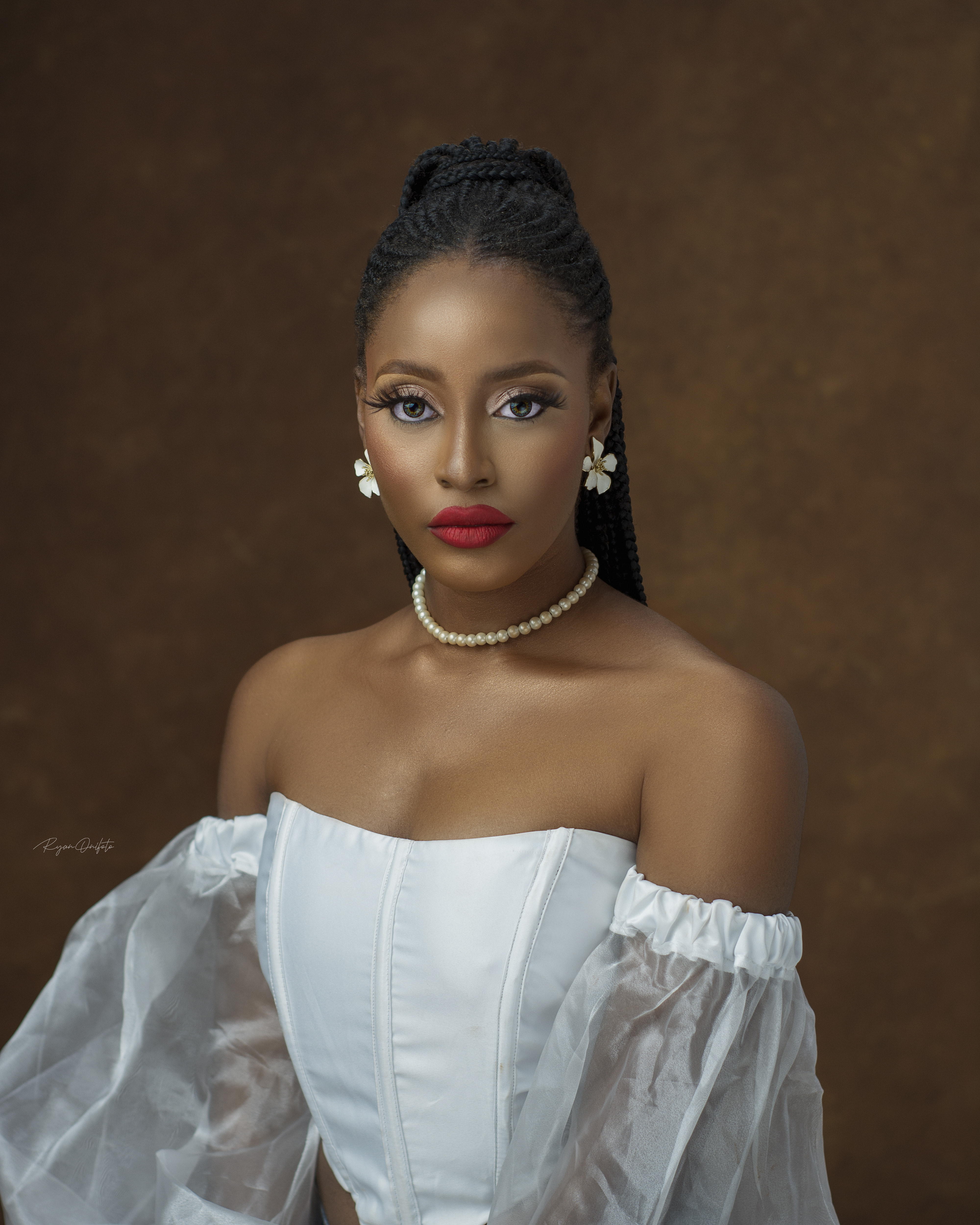