Solidity: Send, Transfer, Call Explained
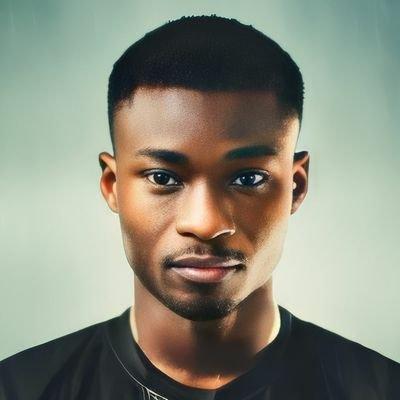
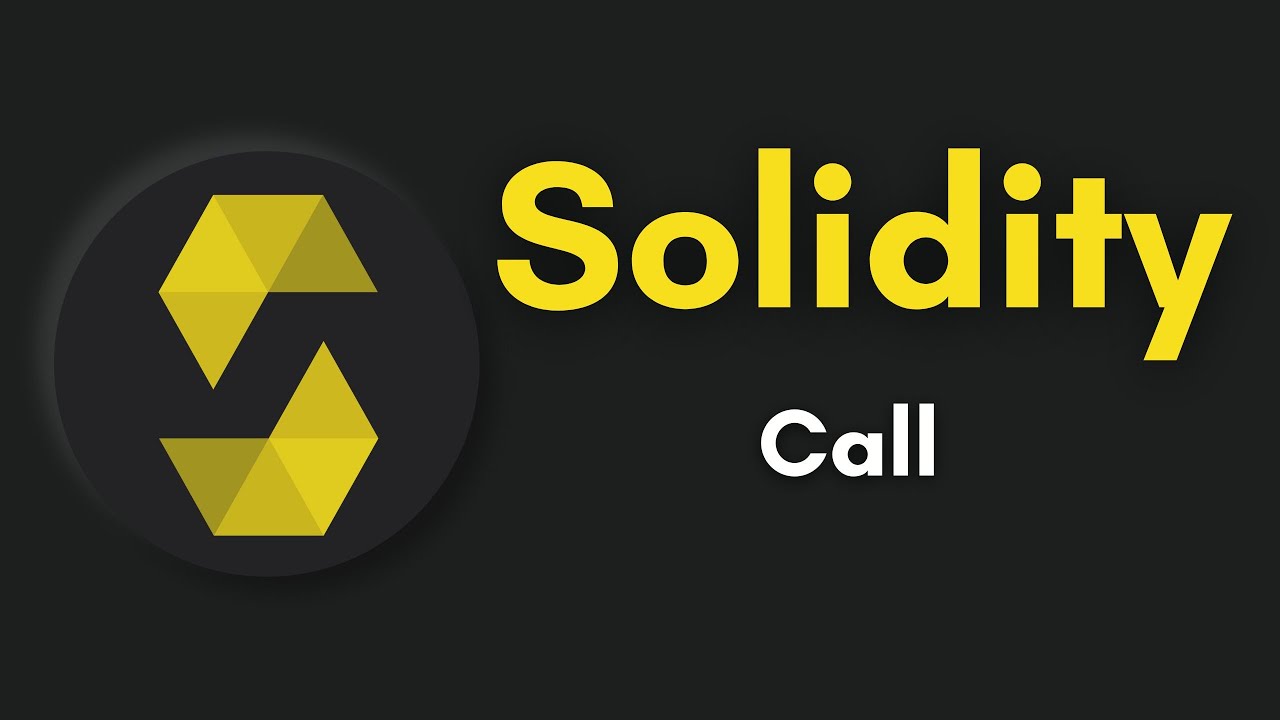
Smart contracts changed the blockchain industry by enabling self-executing code that automatically enforces rules and obligations. Solidity, the primary programming language for Ethereum smart contracts, provides powerful constructs for interacting with the blockchain, including methods for transferring Ether. This article attempts to making of calls in Solidity by simply focusing on the transfer of Ether through three main methods - send
, transfer
, and call
- and discuss their advantages and disadvantages.
Understanding Ether Transfers in Solidity
Before proceeding further into the specifics of these methods, it's important to understand why Ether transfers are unique compared to regular account transfers:
Gas Requirements: Unlike traditional account transfers, which are handled by the network, Ether transfers requires the forwarding of gas - gas to be paid upfront.
Security Considerations: When transferring/sending Ether, there's a risk of losing funds if the recipient's address is incorrect or if the transaction fails.
Flexibility: Solidity offers different approaches to handling Ether transfers, allowing developers to choose the most suitable method based on their needs.
The Three Methods ( Send, Transfer and Call)
1. Send Method
This is the oldest method used in sending Ether in Solidity. When using send
, only 2,300 gas is sent along with the transaction. This limited gas is sufficient for simple operations, such as updating a balance or logging an event in the receiving contract, but not for more complex operations. It is characterised by the following:
It returns a boolean indicating whether the transaction was successful.
It uses a fixed gas limit of 2300 units regardless of the transaction size. This means If a transaction contains complex logic and the gas required is more than 2300 units of gas, then the transaction fails.
It doesn't return any value or revert state changes if the transaction fails.
// where `to` is the recipient
address to;
uint256 amount;
to.send(amount);
Advantages:
It is quite simple to use
It doesn't throw errors if the recipient(`address to`) is a contract
It does protect against re-entrancy attack.
Disadvantages:
Fixed gas cost regardless of transaction size
Due to the fixed gas, it is a waste especially when running simple logic.
No ability to specify custom gas limits
2. Transfer Method
The transfer
method is an improvement over the send
method but it still forwards only 2,300 gas, just like send
. However, the improvement is seen in the reverts of transactions if the transfer fails, making it a lot safer than the `send` method.
// where `to` is the recipient
address to;
uint256 amount;
to.transfer(amount);
Advantages:
Throws an error if the recipient is a contract
Simpler syntax than
call
Disadvantages:
It still uses a fixed gas limit of 2300gas
Can't handle complex transactions
3. Call Method
The call method is a low-level call. It is the most flexible and powerful method for sending Ether. Unlike send and transfer, `call` allows you to specify the amount of gas to forward for that transaction, which means you can send all available gas or specify a custom amount of gas, depending on the needs of the receiving contract. In the situation where the gas forwarded is not fully consumed, it refunds the sender the unused gas. An example is of this: if gasAmount
is set to 2,300 but the receiving contract only needs 1,000 gas to execute its logic, the remaining 1,300 gas is returned to the sender after the execution is complete.
// where `to` is the recipient
address to;
uint256 amount;
(bool success, ) = recipient.call{value: amount}("");
require(success, "Transaction failed");
function sendEtherWithCustomGas(address payable to, uint256 amount, uint256 gasAmount) external payable {
(bool success, ) = to.call{value: amount, gas: gasAmount}("");
require(success, "Call failed");
}
Advantages:
It gives full control over gas usage
It can interact with smart contracts
Its more secure than
send
andtransfer
Disadvantages:
Requires manual error checking
Its does have a more complex syntax
Pros and Cons of Using the Call Function
Pros:
Gas Control: Allows the sender to specify custom gas limits, potentially saving on unnecessary gas costs.
Flexibility: Enables interaction with smart contracts and complex transactions.
Error Handling: Provides more granular control over error management.
Security: Reduces the risk of losing funds due to unexpected behaviour from the recipient contract.
Cons:
Complexity: Requires more careful coding and error handling.
Manual Success Check: Developers need to manually check the success of the operation.
Potential for Reentrancy Attacks: If not implemented correctly, it can be vulnerable to reentrancy attacks.
Why the Call is Preferable
The call
method is often considered the most preferable option for several reasons:
Custom Gas Limits: It allows developers to optimize gas usage based on the actual requirements of the transaction.
Interoperability: It enables seamless interaction with other smart contracts, enhancing the functionality of decentralized applications.
Security: By providing more control over the execution environment, it reduces the risk of unintended consequences.
Future-proofing: As the complexity of smart contracts increases,
call
provides a more adaptable framework for handling various types of transactions.
Conclusion
While all three methods (send
, transfer
, and call
) serve the purpose of sending Ether, the call
method stands out as the most versatile and secure option. Its flexibility in gas usage, ability to interact with smart contracts, and enhanced security features make it the preferred choice for many developers working with Ethereum-based projects. Also, it's crucial to note that the choice of method should depend on the specific requirements of the application, considering factors such as gas efficiency, security concerns, and the nature of the transaction being performed.
Subscribe to my newsletter
Read articles from Jeremiah Samuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
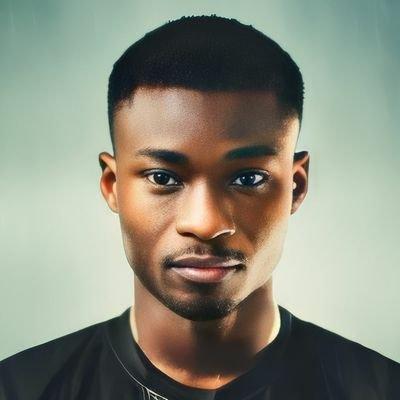