The .transfer(), .send(), and .call() function Used in Solidity For Ether Transfer

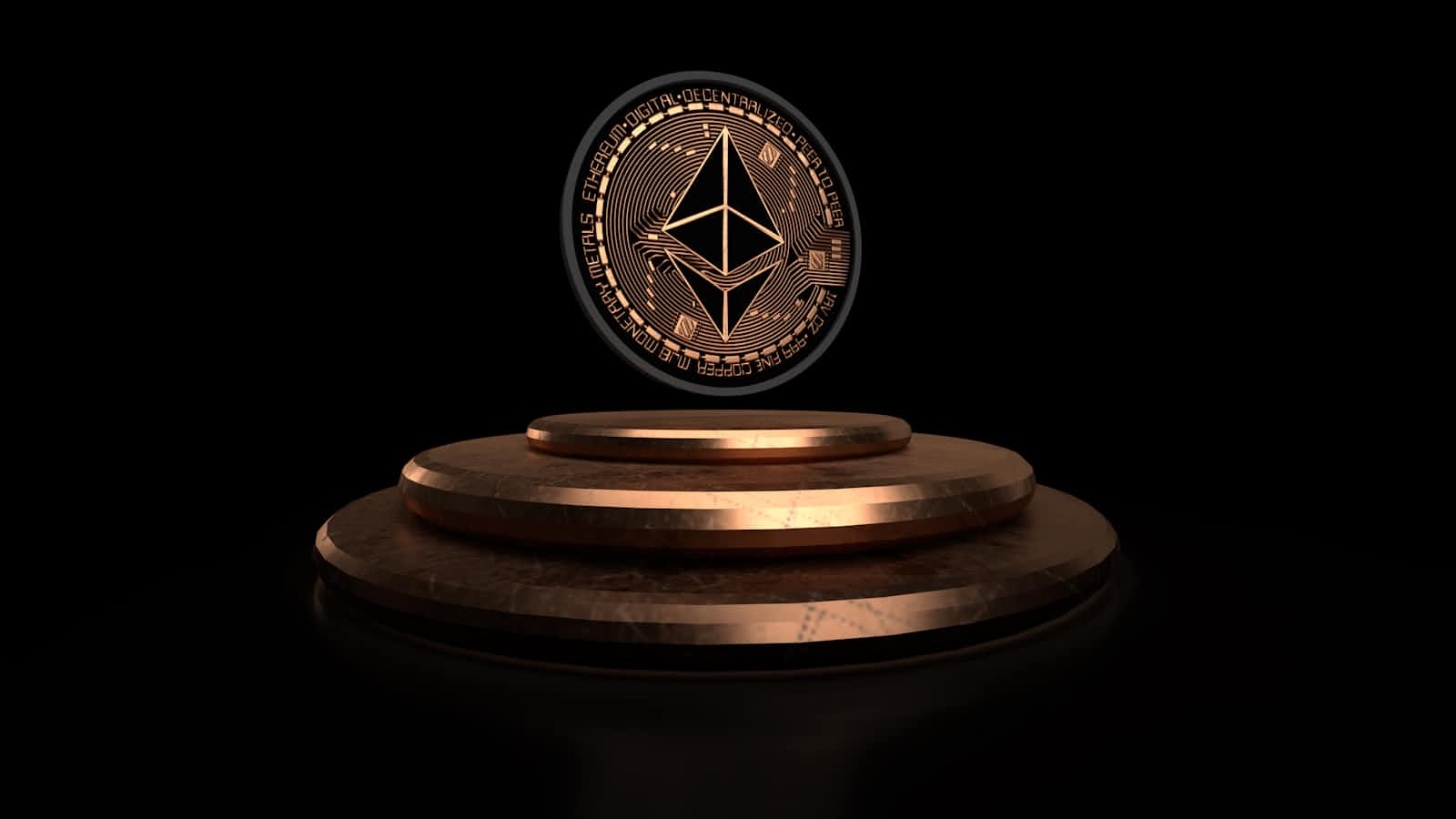
Smart contracts are self-executing contracts that allows automatic transfer of digital assets when certain conditions are met. In Solidity, when developing smart contracts, handling Ether transfers is a crucial aspect that developers must get right for both functionality and security.
This article explains the three primary methods for transferring Ether in Solidity: the.transfer()
, .send()
, and .call()
function.
Their differences, use cases, limitations, and best practices will be covered, along with detailed code snippets to help you understand how to use each method effectively.
Ether Transfer Methods: The Overview
In Solidity, there are three main ways to send Ether from a smart contract to an external address or another contract:
.transfer()
: This function sends Ether with a fixed gas limit of 2300 gas units. It reverts automatically on failure..send()
: Similar to.transfer()
, but returns a boolean indicating success or failure without automatically reverting..call()
: This function is more flexible, allows specifying gas and returns a success status. It’s the preferred method due to its flexibility and explicit error handling.
Let’s dive deeper into each method with examples and detailed explanations.
1. .transfer()
function
The .transfer
function was once considered the safest way to send Ether because it only forwards 2300 gas units, theoretically enough to prevent reentrancy attacks (where a contract’s fallback function is called repeatedly in an attempt to drain funds). However, this gas limit can be a significant limitation as the Ethereum network keeps evolving.
Code Snippet: Using .transfer()
pragma solidity ^0.8.24;
contract Transfer {
function sendEtherViaTransfer(address payable _to) external payable {
// Sends Ether to the another address with a fixed gas limit of 2300 gas
_to.transfer(msg.value);
}
}
The
transfer()
function is straightforward: it sends the Ether amount specified bymsg.value
to therecipient
.Gas Limit: This function imposes a fixed gas limit of 2300 gas units, which is enough for basic operations like logging an event.
If the transfer fails (that is, if the recipient runs out of gas or explicitly reverts), the transaction is automatically reverted, ensuring that no funds are lost.
Limitations of this function
Fixed Gas Limit: The fixed gas limit can cause transactions to fail if the recipient’s fallback function is more complex or requires more gas.
Deprecated for Security: As the Ethereum ecosystem keeps evolving, this limited gas approach is no longer sufficient to guarantee security or functionality, making
.transfer()
less suitable for most modern contracts.
2. .send()
function
Similarly to .transfer()
, the .send
function works almost the same, the key difference is that it returns a boolean indicating whether the transfer was successful. It helps in order to handle failures explicitly rather than relying on automatic reversion.
Example: Using .send()
pragma solidity ^0.8.24;
contract Send {
function sendEtherViaSend(address payable _to) external payable {
// Sends Ether to the address with 2300 gas, returns a boolean
bool sent = recipient.send(msg.value);
require(sent, "Sending failed");
}
}
The
send
function sends Ether to therecipient
with a fixed gas limit of 2300 gas units.if the transfer was successful, it returns
true
orfalse
if it fails (e.g., due to insufficient gas or explicit revert).This method requires explicit error handling using the return value, allowing developers to control the behavior if the transfer fails.
Limitations:
Fixed Gas Limit: Like
.transfer
,.send
also has the 2300 gas limit, which can be a challenge for contracts requiring more complex fallback operations.Manual Error Handling: The developer must handle the failure case explicitly, which can add complexity to the contract code.
3. .call()
function
Presently, the .call()
is the preferred method for sending Ether in Solidity due to its flexibility. It allows you to specify the amount of gas to forward, handle errors explicitly, and is not limited by the 2300 gas unit.
Example: Using .call()
pragma solidity ^0.8.24;
contract Call {
function sendEtherViaCall(address payable _to) public payable {
(bool sucess, bytes memory data) = _to.call{value: msg.value}(""); // Returns false on failure
require(success, "Failed to send Ether");
}
}
The
call()
function allows sending Ether without a gas limit constraint, enabling the recipient to perform complex operations.It returns a tuple with a boolean
success
indicating whether the call was successful and any data returned from the call.Explicit error handling with
require(success, "Failed to send Ether");
provides more control and flexibility compared to.transfer()
and.send()
.
Advantages:
Gas Flexibility: You can specify how much gas to forward, making it suitable for complex fallback functions in the recipient contract.
Explicit Error Handling: You have full control over handling errors, which is essential for building smart contracts that are secure and resilient.
Best Security Practice: When combined with proper reentrancy protections (like the Checks-Effects-Interactions pattern or
ReentrancyGuard
),.call()
is the most secure and recommended method for sending Ether.
Receiving Ether in Solidity: receive()
and fallback()
Functions
If a contract is meant to receive Ether, it must implement at least one of the following functions: receive()
or fallback()
. These functions determine how a contract handles incoming Ether and whether it can accept plain Ether transfers or data-bearing transactions.
1. receive() external payable
function
The receive()
function is specifically designed to handle plain Ether transfers sent directly to the contract without any accompanying data.
Triggered When: The contract receives Ether with an empty
calldata
(i.e., no function call data).Purpose: To allow the contract to accept simple Ether transfers without any additional processing or function calls.
Requirements: Must be marked
external
andpayable
to accept Ether.
pragma solidity ^0.8.24;
contract EtherReceiver {
// This function is called when the contract receives plain Ether
receive() external payable {
// Logic to handle incoming Ether
}
}
2. fallback() external payable
function
The fallback()
function acts as a catch-all mechanism for handling transactions that don’t match any existing function in the contract, including those that contain data or those that have no matching function signature.
Triggered When:
The contract receives a message with data that does not match any other function’s signature.
The contract receives Ether, but it does not have a
receive()
function.
Purpose: To handle transactions with data or unmatched function identifiers and to serve as a default function for accepting Ether when no other suitable function exists.
Requirements: Must be marked
external
and can be markedpayable
if it needs to accept Ether.
pragma solidity ^0.8.24;
contract FallbackReceiver {
// This function is called when the contract receives data or if no other function matches
fallback() external payable {
// Logic to handle unexpected calls or data-bearing transactions
}
}
3. Interaction between receive()
and fallback()
If a contract has both
receive()
andfallback()
functions:receive()
is called when the contract receives plain Ether with no data.fallback()
is called when the transaction includes data or when the function signature does not match any available functions.
If only
fallback()
exists: Thefallback()
function handles all transactions, whether they involve plain Ether or data-bearing calls.If neither function exists: This means the contract cannot receive Ether through direct transfers and will revert the transaction (it will throw an exception).
Error Handling in Solidity: revert
, assert
, and require
Solidity provides three primary error-handling mechanisms: revert()
, assert()
, and require()
. Each serves a specific purpose and is used in different scenarios to manage contract behavior when something goes wrong.
For the sake of this article, our focus will be on the require()
function and how it is used to handle errors
1. require()
function
The require()
function is used to validate conditions such as function inputs, contract state variables, or return values from external calls. If the condition is not met, require()
reverts the transaction with an optional error message, and any unused gas is refunded.
Example: Using require()
pragma solidity ^0.8.24;
contract RequireExample {
uint public minimumDeposit = 1 ether;
function deposit(uint amount) external payable {
// Ensure that the amount is at least the minimum required deposit
require(amount >= minimumDeposit, "Deposit amount is too low");
}
}
Explanation:
In this example,
require
checks that the deposit amount meets the minimum requirement. If the condition is not met, it reverts with the message "Deposit amount is too low."Usage:
require
is ideal for validating inputs, ensuring conditions are met before proceeding, and protecting against undesirable contract states.Gas Usage: Like
revert
,require
refunds the remaining gas, making it an efficient way to handle validation checks.The
require()
function is used for validating inputs, contract states, or conditions that must be true for execution to proceed. It reverts the transaction with a message and refunds unused gas.
By using these error-handling mechanisms appropriately, smart contracts behaviour can be predictable and secure, enhancing the overall robustness and reliability of your Solidity code.
Recommendations and Practices
When choosing how to send Ether in Solidity, consider the following best practices:
Use
.call()
function for flexibility and control. It is generally recommended because it allows you to specify gas, handle errors explicitly, and provides greater control over the execution flow of your contract.Implement reentrancy guards when using
.call()
function (e.g., using OpenZeppelin’sReentrancyGuard
or the Checks-Effects-Interactions pattern) to mitigate reentrancy attacks.Avoid
.transfer()
and.send()
for complex contracts: Due to the fixed 2300 gas limit, avoid.transfer
and.send
if the receiving contract might need more gas for its fallback or receive function.Always handle errors explicitly when using
.call()
function to ensure that your contract behaves as expected in case of failures.
Conclusion
Understanding how to properly transfer Ether in Solidity is crucial for any developer working with Ethereum smart contracts. While .transfer()
and .send()
were initially popular for their simplicity and perceived safety, the limitations of their fixed gas limits have led to a shift towards the more flexible and secure .call()
function. By adopting .call()
and following best practices like explicit error handling and implementing reentrancy guards, developers can ensure that their contracts handle Ether transfers safely and efficiently.
Whether you’re a seasoned Solidity developer or just starting out, mastering these transfer functions and their appropriate use cases will significantly enhance the security and robustness of your smart contracts.
Subscribe to my newsletter
Read articles from Michael John directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
