Day 29: Must-Know Jenkins Interview Questions

Table of contents
- 🟩General Questions❓
- 🔴What’s the difference between continuous integration, delivery, and deployment?
- 🔴Benefits of CI/CD
- 🔴What is Meant by CI/CD?
- 🔴What is a Jenkins Pipeline?
- 🔴How Do You Configure a Job in Jenkins?
- 🔴Where Do You Find Errors in Jenkins?
- 🔴In Jenkins, How Can You Find Log Files?
- 🔴Jenkins Workflow and Write a Script for This Workflow
- 🔴How to Create Continuous Deployment in Jenkins?
- 🔴How to Build a Job in Jenkins?
- 🔴Why Do We Use Pipelines in Jenkins?
- 🔴Is Jenkins Alone Sufficient for Automation?
- 🔴How Will You Handle Secrets in Jenkins?
- 🔴Explain the Different Stages in a CI/CD Setup
- 🔴Name Some of the Plugins in Jenkins
- 🟧Scenario-Based Questions❓
- 🟣You have a Jenkins pipeline that deploys to a staging environment. Suddenly, the deployment failed due to a missing configuration file. How would you troubleshoot and resolve this issue?
- 🟣Imagine you have a Jenkins job that is taking significantly longer to complete than expected. What steps would you take to identify and mitigate the issue?
- 🟣You need to implement a secure method to manage environment-specific secrets for different stages (development, staging, production) in your Jenkins pipeline. How would you approach this?
- 🟣Suppose your Jenkins master node is under heavy load and build times are increasing. What strategies can you use to distribute the load and ensure efficient build processing?
- 🟣A developer commits a code change that breaks the build. How would you set up Jenkins to automatically handle such scenarios and notify the relevant team members?
- 🟣You are tasked with setting up a Jenkins pipeline for a multi-branch project. How would you handle different configurations and build steps for different branches?
- 🟣How would you implement a rollback strategy in a Jenkins pipeline to revert to a previous stable version if the deployment fails?
- 🟣In a scenario where you have multiple teams working on different projects, how would you structure Jenkins jobs and pipelines to ensure efficient resource utilization and manage permissions?
- 🟣Your Jenkins agents are running in a cloud environment, and you notice that build times fluctuate due to varying resource availability. How would you optimize the performance and cost of these agents?
- 💥Conclusion💥
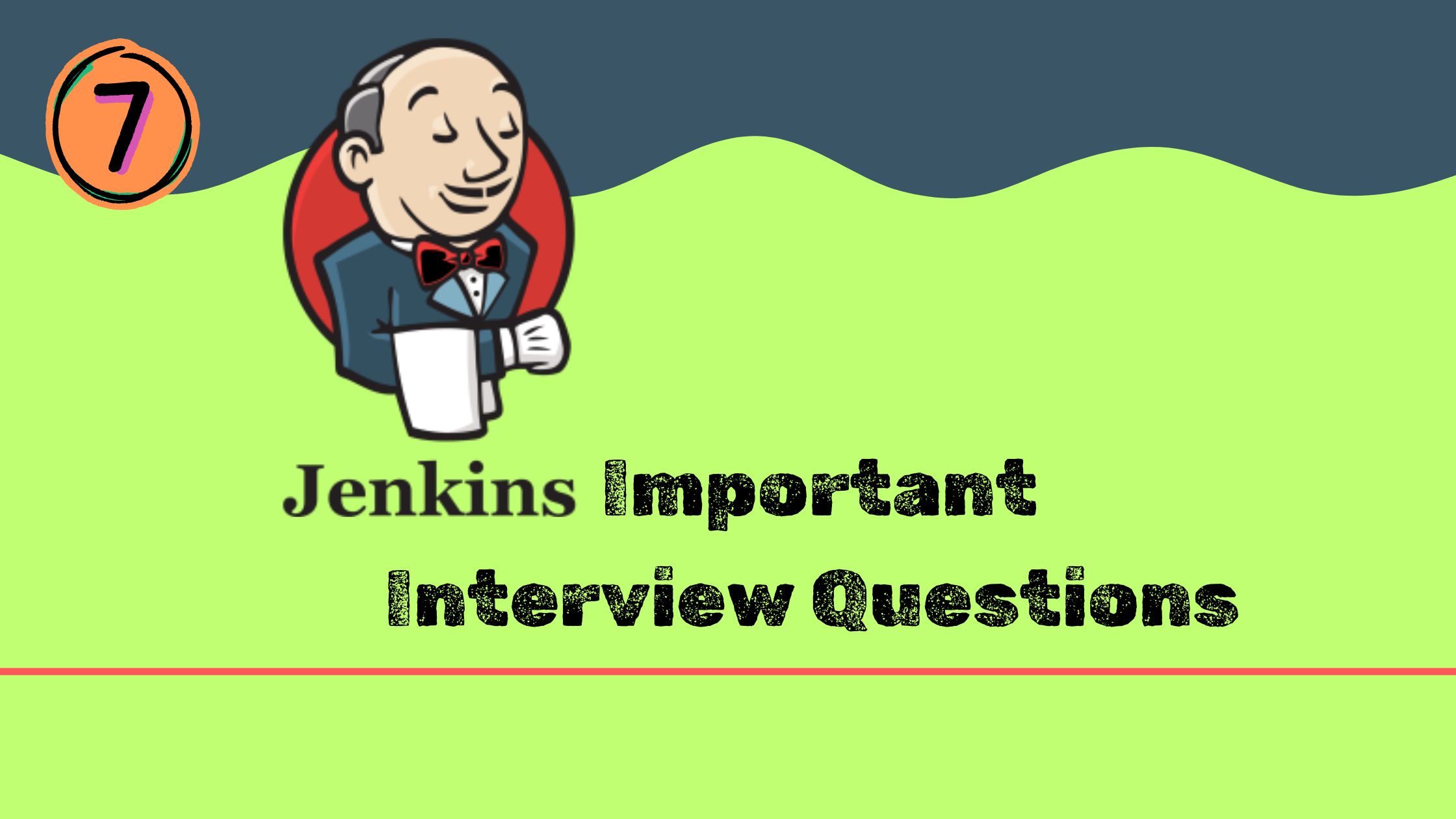
🟩General Questions❓
🔴What’s the difference between continuous integration, delivery, and deployment?
Continuous Integration (CI): The practice of frequently merging code changes into a shared repository, where automated builds and tests are run to ensure code quality. Developers often merge their code changes into a shared repository, followed by automated builds and tests.
Continuous Delivery (CD): It extends CI by automatically deploying all code changes to a testing environment and getting them ready for a production release. This involves automated deployment of code changes to environments similar to production for testing and approval.
Continuous Deployment: This method automatically deploys every code change to the production environment, eliminating the need for manual deployment approvals.Automatic deployment of code changes to production after successful testing and approval.
🔴Benefits of CI/CD
Early Bug Detection: Automated tests in CI/CD pipelines help catch bugs early.
Faster Releases: Automates the build, test, and deployment processes, leading to quicker releases.
Improved Collaboration: Encourages collaboration among development, QA, and operations teams.
Reduced Manual Intervention: Automates repetitive tasks, giving developers more time to work on new features.
🔴What is Meant by CI/CD?
It's a set of practices that enable development teams to deliver code changes more frequently and reliably by using automation.
🔴What is a Jenkins Pipeline?
A Jenkins Pipeline is a set of plugins that help us create and manage continuous delivery pipelines in Jenkins. It lets us define a complex process with multiple steps using a simple DSL (Domain Specific Language) syntax.
Syntax
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building...'
// Add build steps here
}
}
stage('Test') {
steps {
echo 'Testing...'
// Add testing steps here
}
}
stage('Deploy') {
steps {
echo 'Deploying...'
// Add deployment steps here
}
}
}
}
🔴How Do You Configure a Job in Jenkins?
Go to Jenkins Dashboard.
Click on "New Item".
Enter a job name, select a job type (e.g., Freestyle project, Pipeline), and click "OK".
Configure the job by adding build steps, specifying the source code repository, and defining triggers (e.g., GitHub webhooks).
Save the job configuration.
Example:
Step 1: Click on
New Item
to create a pipelineStep 2: Give the pipeline name & Choose
Freestyle Project
Step 3: Give a description(optional)
Step 4: Add a build step -
Execute shell
.
🔴Where Do You Find Errors in Jenkins?
Errors can be found in the console output of the build. To access it, click on the build number and then select "Console Output" from the left-hand menu.
Example:
🔴In Jenkins, How Can You Find Log Files?
Jenkins stores logs in the $JENKINS_HOME/logs
directory. we can also find individual build logs in the job's workspace directory under $JENKINS_HOME/jobs/<job_name>/builds/<build_number>/log
.
🔴Jenkins Workflow and Write a Script for This Workflow
A typical Jenkins workflow includes stages like Build, Test, and Deploy. Here's a basic scripted pipeline example:
node {
stage('Build') {
// Build step
echo 'Building...'
}
stage('Test') {
// Test step
echo 'Testing...'
}
stage('Deploy') {
// Deploy step
echo 'Deploying...'
}
}
🔴How to Create Continuous Deployment in Jenkins?
Create a Jenkins Pipeline.
Define stages for build, test, and deploy.
Use plugins like Deploy to Container Plugin or Kubernetes Plugin to deploy to production.
🔴How to Build a Job in Jenkins?
Create a new job in Jenkins.
Configure the source code management (e.g., Git).
Define build steps (e.g., compile, test).
Set up post-build actions (e.g., deploy, notify).
🔴Why Do We Use Pipelines in Jenkins?
Pipelines let us define automated processes as code. This allows for version control, complex workflows, error handling, and better visibility of stages and their statuses.
🔴Is Jenkins Alone Sufficient for Automation?
Jenkins is a powerful tool for automation, but it may require additional tools for specific tasks, such as Docker for containerization, Ansible for configuration management, Kubernetes for orchestration, and various testing and security tools.
🔴How Will You Handle Secrets in Jenkins?
Use plugins like Credentials Plugin to securely store and manage secrets.
Credentials can be accessed securely in pipeline scripts using the credentials()
method.
withCredentials([string(credentialsId: 'my-secret', variable: 'SECRET')]) {
echo "Using secret: ${SECRET}"
}
🔴Explain the Different Stages in a CI/CD Setup
Source: Triggered by a commit to the version control system(GitHub).
Build: Compiling the code.
Test: Running automated tests.
Deploy: Deploying to the staging or production environment.
Monitor: Checking application health and performance.
🔴Name Some of the Plugins in Jenkins
Git Plugin: Integrates Jenkins with Git repositories.
Pipeline Plugin: Enables the creation of Jenkins pipelines.
Maven Plugin: Build Maven projects.
Docker Plugin: Provides support for using Docker containers.
Kubernetes Plugin: Integrate with Kubernetes clusters.
Credentials Plugin: Manages and stores credentials securely.
Slack Notification Plugin: Sends build notifications to Slack channels.
🟧Scenario-Based Questions❓
🟣You have a Jenkins pipeline that deploys to a staging environment. Suddenly, the deployment failed due to a missing configuration file. How would you troubleshoot and resolve this issue?
Check the Jenkins console output for error details.
Verify the existence of the configuration file in the repository.
Ensure that environment variables or credentials are correctly set.
Update the pipeline script to handle missing files gracefully and send alerts.
Retry the deployment.
🟣Imagine you have a Jenkins job that is taking significantly longer to complete than expected. What steps would you take to identify and mitigate the issue?
Review the console output to identify bottlenecks.
Analyze system resource usage (CPU, memory) on Jenkins nodes.
Optimize the build process (e.g., parallel execution, caching).
Scale horizontally by adding more agents or vertically by increasing resource limits.
🟣You need to implement a secure method to manage environment-specific secrets for different stages (development, staging, production) in your Jenkins pipeline. How would you approach this?
Use Jenkins Credentials Plugin to store secrets securely.
Use environment variables to distinguish between environments.
Access credentials in the pipeline using the
withCredentials()
method.
🟣Suppose your Jenkins master node is under heavy load and build times are increasing. What strategies can you use to distribute the load and ensure efficient build processing?
Use a Jenkins master-agent setup to move builds to agent nodes.
Set up agents on different servers or use cloud-based agents.
Balance the load by distributing jobs across multiple agents.
🟣A developer commits a code change that breaks the build. How would you set up Jenkins to automatically handle such scenarios and notify the relevant team members?
Set up automatic build triggers on commits.
Use the
post
section in Jenkins Pipeline to handle build failures.Configure Jenkins to send email or Slack notifications on failure.
post { failure { mail to: 'devteam@example.com', subject: "Build Failed: ${env.JOB_NAME}", body: "Check the Jenkins console output for more details." } }
Set up a webhook to trigger automated actions (e.g., rollback, code analysis) when a build fails.
🟣You are tasked with setting up a Jenkins pipeline for a multi-branch project. How would you handle different configurations and build steps for different branches?
Use the Jenkins Multibranch Pipeline Plugin.
Define a
Jenkinsfile
with conditional stages based on the branch name.
pipeline {
agent any
stages {
stage('Build') {
when {
branch 'master'
}
steps {
echo 'Building master branch...'
}
}
stage('Test') {
when {
branch 'Dev'
}
steps {
echo 'Testing develop branch...'
}
}
}
}
🟣How would you implement a rollback strategy in a Jenkins pipeline to revert to a previous stable version if the deployment fails?
Use versioning for builds and maintain artifacts.
Implement a rollback stage in the pipeline to deploy the last successful build if the current deployment fails.
pipeline {
agent any
stages {
stage('Deploy') {
steps {
script {
try {
// Deployment code
} catch (Exception e) {
echo 'Deployment is failed, rolling back...'
// Rollback code
}
}
}
}
}
}
🟣In a scenario where you have multiple teams working on different projects, how would you structure Jenkins jobs and pipelines to ensure efficient resource utilization and manage permissions?
Create separate Jenkins instances or folders for different teams.
Use roles and permissions to control (RBAC) access to jobs and pipelines.
Implement tagging or labelling to organize jobs and pipelines.
Consider using a shared library to define common pipeline steps.
🟣Your Jenkins agents are running in a cloud environment, and you notice that build times fluctuate due to varying resource availability. How would you optimize the performance and cost of these agents?
Use auto-scaling to adjust the number of agents based on demand.
Use spot instances or preemptible instances to lower costs.
Optimize job configurations to minimize resource usage.
Consider using caching or parallel execution to improve build performance.
💥Conclusion💥
Jenkins is a powerful tool in the DevOps toolkit, helping teams automate their CI/CD workflows efficiently. Knowing these common Jenkins questions and scenarios can help us get ready for DevOps interviews and improve our automation skills. Remember, practicing and applying these ideas in real-world situations will boost our expertise and make you a valuable member of any DevOps team.
Subscribe to my newsletter
Read articles from Ketan Hiray directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ketan Hiray
Ketan Hiray
💼 Senior Software Engineer 🚀 Enthusiast in DevOps Engineering