Understanding the Global Interpreter Lock (GIL) and Its Impact on Threading in Python
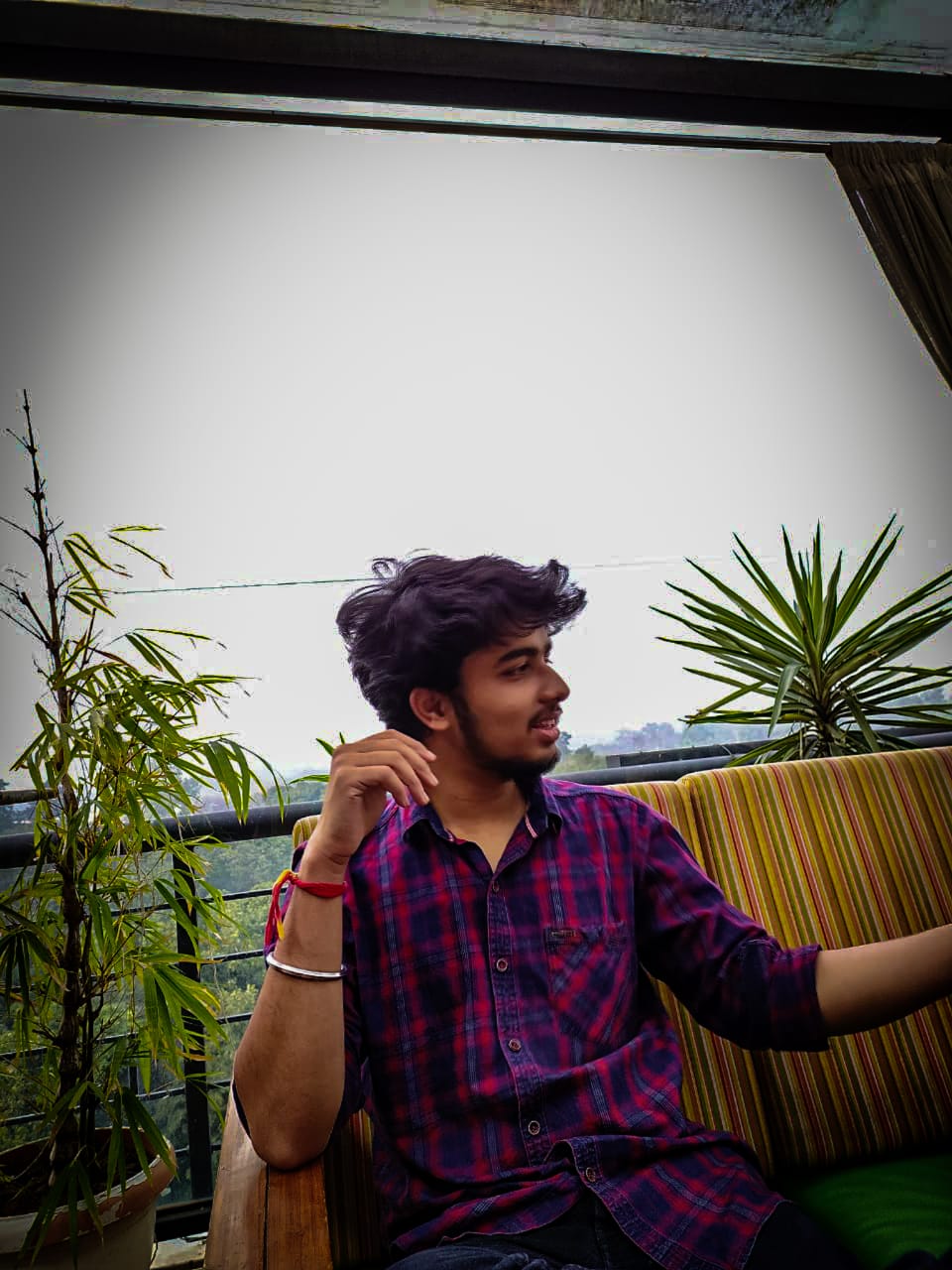

What is the GIL?
The Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecode simultaneously. The GIL exists because CPython, the reference implementation of Python, is not thread-safe. This means that, in a multi-threaded Python program, even though you may have multiple threads, only one thread can execute Python code at a time.
Thread safety:
Thread safety refers to the property of a piece of code or a data structure that guarantees safe execution by multiple threads at the same time. When code is thread-safe, it ensures that concurrent execution by multiple threads does not lead to race conditions, data corruption, or unexpected behavior.
Why is the GIL a Problem?
The GIL can be a significant limitation when using threading for CPU-bound tasks in Python. Since only one thread can execute Python bytecode at a time, the presence of the GIL means that multi-threading doesn’t provide the expected performance improvements for CPU-bound tasks. Instead of fully utilizing multiple CPU cores, the program ends up executing threads sequentially, leading to suboptimal performance.
For example, in a CPU-bound task like complex mathematical computations, threads will constantly compete for the GIL, which results in context switching between threads rather than true parallel execution. This can make your program slower rather than faster, especially on multi-core systems where true parallelism could be achieved.
How to Avoid the GIL Problem
To avoid the limitations imposed by the GIL, you can use the following approaches:
Multiprocessing: Since the GIL is only a problem within a single process, you can bypass it by using the
multiprocessing
module, which spawns separate processes for your tasks. Each process has its own GIL and memory space, allowing true parallel execution of CPU-bound tasks across multiple cores.import multiprocessing def cpu_bound_task(n): return sum(i * i for i in range(n)) if __name__ == '__main__': with multiprocessing.Pool() as pool: results = pool.map(cpu_bound_task, [10**6, 10**6, 10**6, 10**6]) print(results)
In this example, the
multiprocessing.Pool
distributes thecpu_bound_task
across multiple processes, fully utilizing multiple CPU cores and avoiding the GIL.Using Native Extensions or Cython: If you have performance-critical sections of code that are bottlenecked by the GIL, you can offload these sections to native code written in C or Cython. These extensions can release the GIL while executing, allowing other threads to run concurrently.
Asyncio for I/O-bound tasks: For I/O-bound tasks, where the main bottleneck is waiting for external resources, using
asyncio
can be more efficient than threading.Asyncio
doesn’t involve native threads and therefore doesn’t suffer from GIL-related issues.import asyncio async def io_bound_task(): await asyncio.sleep(1) return "Task complete" async def main(): tasks = [io_bound_task() for _ in range(10)] results = await asyncio.gather(*tasks) print(results) asyncio.run(main())
Here,
asyncio
allows you to manage I/O-bound tasks concurrently without the overhead and limitations of threads, providing an efficient alternative in scenarios where the GIL could be a problem.
Summary
The GIL is a critical part of CPython that ensures thread safety but can hinder the performance of multi-threaded CPU-bound tasks. By understanding the GIL’s limitations and employing alternatives like multiprocessing, native extensions, or asynchronous programming, you can write Python programs that effectively leverage concurrency and parallelism.
Subscribe to my newsletter
Read articles from Saurav Shrivastav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
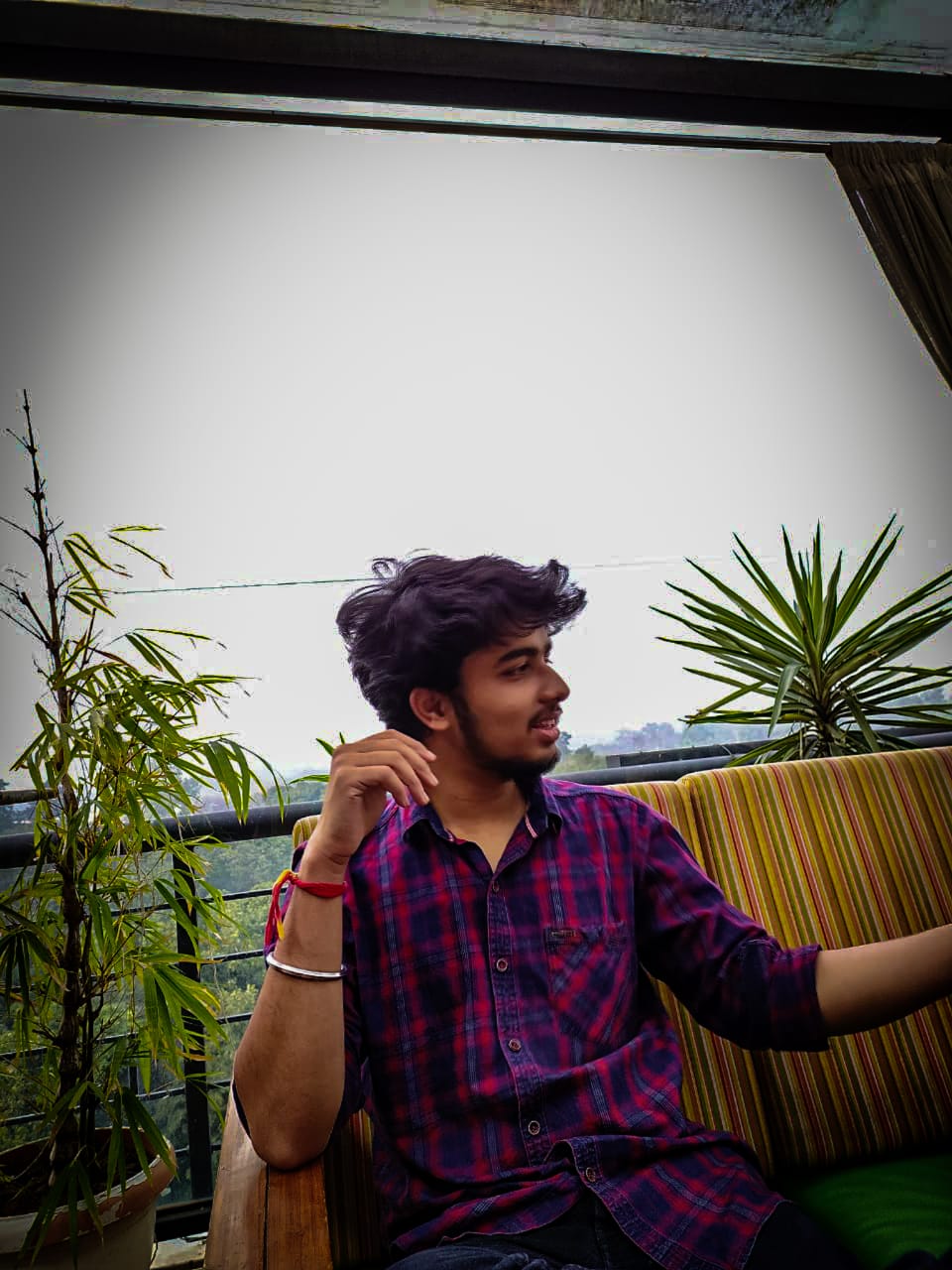