Creating a Custom Hook in React: When and How to Build One

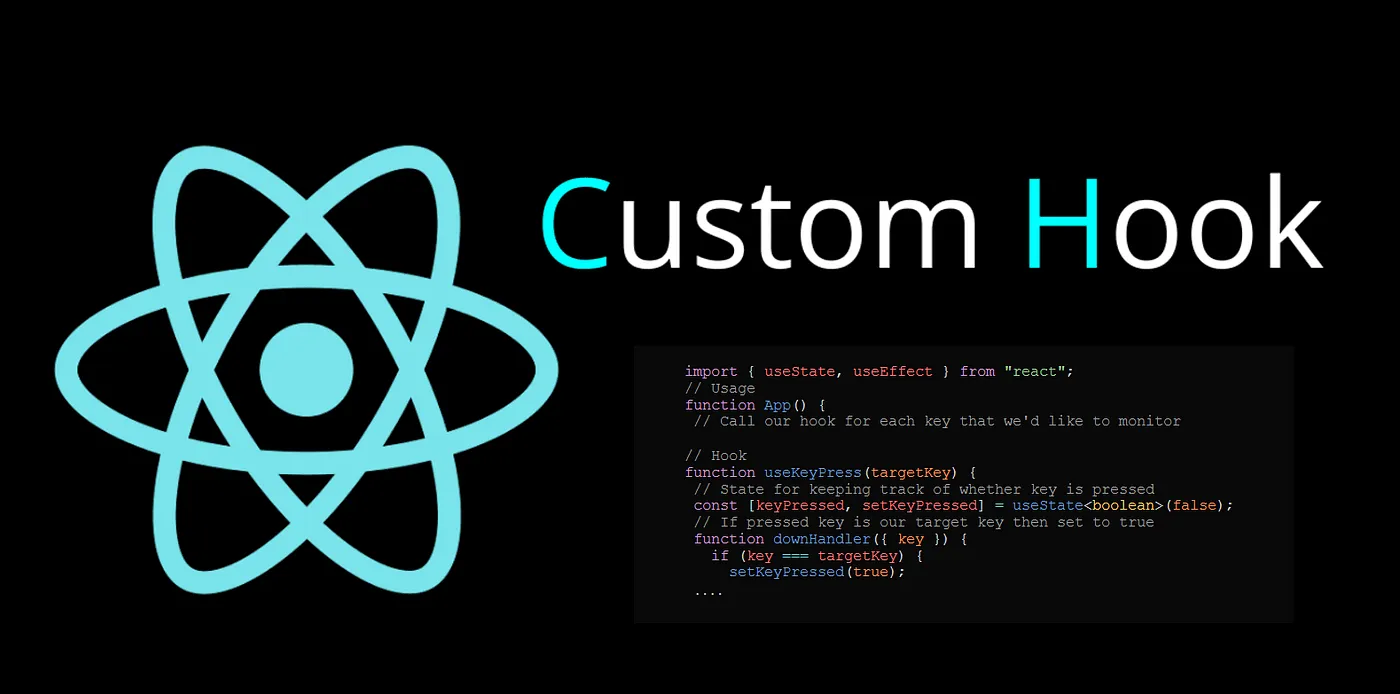
React hooks have revolutionized the way developers build functional components, offering a way to leverage state and lifecycle features without the need for class components. While React provides several built-in hooks like useState
, useEffect
, and useContext
, there are times when you need to create your own custom hooks to handle complex logic and ensure code reusability. In this blog post, we will explore when to create a custom hook and how to build one.
What is a Custom Hook?
A custom hook is a JavaScript function in React that uses one or more built-in hooks to encapsulate reusable logic. It allows you to extract component logic into reusable functions. Custom hooks can help you avoid repetition and make your code more modular and easier to maintain.
The key point to remember is that custom hooks are just normal JavaScript functions but with a convention that their names should always start with the word use
(e.g., useCustomHook
). This naming convention is important because it signals to React that the hook follows its rules for hooks (such as only calling them at the top level of a component).
When Should You Create a Custom Hook?
Reusing Stateful Logic Across Components: If you find yourself writing the same logic for managing state across multiple components, a custom hook can help you consolidate that logic in one place. This reduces redundancy and makes updates easier since changes only need to be made in one place.
Example: A custom hook to handle form input state in various components.
Simplifying Complex Components: Sometimes, a single component can become cluttered with too much logic—state management, side effects, event handlers, and more. In these cases, you can extract specific logic into a custom hook, making the component cleaner and easier to understand.
Example: A component that fetches data, updates the UI, and handles loading/error states might use a custom hook for the data-fetching logic.
Encapsulating Side Effects: Hooks like
useEffect
are often used to handle side effects such as data fetching or interacting with browser APIs. When these effects need to be shared among multiple components, custom hooks can encapsulate the side effect logic and make it reusable.Example: A custom hook to handle scrolling behavior or window resizing.
Abstraction of Third-Party APIs or Libraries: When you're working with third-party APIs (like Firebase, GraphQL, etc.) or external libraries, it’s a good idea to abstract this logic into a custom hook. This keeps your component code clean and reduces the amount of third-party logic cluttering your components.
Example: A custom hook for handling Firebase authentication across multiple pages.
How to Create a Custom Hook
Let’s walk through the process of building a custom hook in React by creating a simple data-fetching hook. This hook will encapsulate the logic of fetching data from an API and managing loading and error states.
Step 1: Identify the Logic to Extract
We want to create a hook that can be reused in multiple components to fetch data from an API. The hook will manage the following:
API call logic.
Loading state.
Error handling.
Step 2: Create the Custom Hook
import { useState, useEffect } from 'react';
function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
async function fetchData() {
try {
setLoading(true);
const response = await fetch(url);
if (!response.ok) {
throw new Error('Error fetching data');
}
const result = await response.json();
setData(result);
} catch (err) {
setError(err.message);
} finally {
setLoading(false);
}
}
fetchData();
}, [url]);
return { data, loading, error };
}
Step 3: Use the Custom Hook
Now that we have created the useFetch
hook, let’s use it inside a functional component.
function DataDisplayComponent() {
const { data, loading, error } = useFetch('https://api.example.com/data');
if (loading) {
return <div>Loading...</div>;
}
if (error) {
return <div>Error: {error}</div>;
}
return (
<div>
<h1>Data:</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
Step 4: Refactor and Reuse
The beauty of this approach is that now you can reuse useFetch
in any component that needs to fetch data from an API. For example, you can use it in another component to fetch data from a different endpoint:
function AnotherComponent() {
const { data, loading, error } = useFetch('https://api.example.com/other-data');
if (loading) {
return <div>Loading...</div>;
}
if (error) {
return <div>Error: {error}</div>;
}
return (
<div>
<h1>Other Data:</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
Best Practices for Creating Custom Hooks
Start with Built-in Hooks: Before creating a custom hook, make sure you are leveraging the built-in hooks like
useState
,useEffect
,useReducer
, etc., as much as possible. These are highly optimized and should be your first choice.Name Hooks Intuitively: Always follow the
use
prefix convention. This helps React identify hooks, and it also makes your custom hook easily recognizable as a hook by other developers.Keep it Simple and Focused: A custom hook should focus on one task or purpose. If your hook starts doing too many things, it may become difficult to manage. Consider splitting it into smaller, focused hooks.
Don't Break the Rules of Hooks: Custom hooks must follow the same rules as regular hooks. They should not be called conditionally or inside loops. Always ensure they are invoked at the top level of your component functions.
Return Only What’s Necessary: A custom hook should return only the values or functions necessary for the component that uses it. This keeps the API of the hook clean and easy to understand.
Conclusion
Custom hooks in React are a powerful way to make your code more modular, reusable, and maintainable. By encapsulating logic into a custom hook, you can simplify your components, reduce code duplication, and keep your application code clean. Whether you're managing state, side effects, or abstracting third-party APIs, creating custom hooks is a best practice that can improve your React projects.
Next time you find yourself repeating code or facing a complex component, consider refactoring with a custom hook!
Subscribe to my newsletter
Read articles from akash javali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

akash javali
akash javali
A passionate 'Web Developer' with a Master's degree in Electronics and Communication Engineering who chose passion as a career. I like to keep it simple. My goals are to focus on typography, and content and convey the message that you want to send. Well-organized person, problem solver, & currently a 'Senior Software Engineer' at an IT firm for the past few years. I enjoy traveling, watching TV series & movies, hitting the gym, or online gaming.