Understanding the Node.js Event Loop: The Heartbeat of Asynchronous Programming
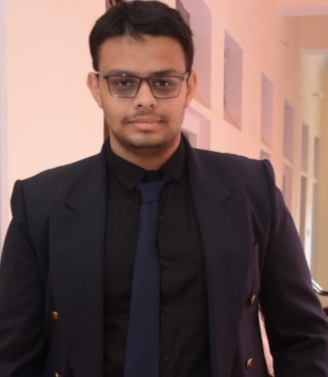
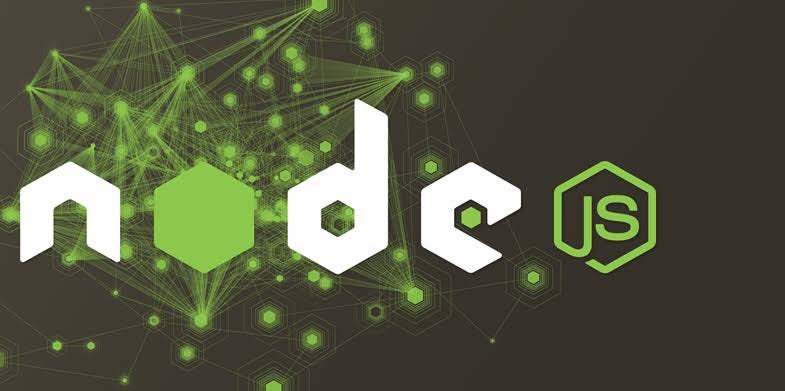
When I started diving deeper into the world of Node.js, I quickly realized that its performance and scalability didn't just come from using JavaScript on the server side. Instead, it was the non-blocking, event-driven architecture, powered by the event loop, that truly set Node.js apart.
In this blog, I want to share my insights into the Node.js event loop—how it works, why it's critical for performance, and some key takeaways for optimizing your Node.js applications.
What is the Event Loop?
The event loop is a fundamental part of Node.js that allows it to handle numerous tasks concurrently without creating multiple threads for each request. It’s like the central hub that orchestrates various tasks—executing callbacks, handling events, managing I/O operations, and more.
Here’s how it works at a high level:
Call Stack: The call stack is where the JavaScript code execution happens. It works on a LIFO (Last In, First Out) principle. When a function is invoked, it’s pushed onto the stack, and when it returns, it’s popped off.
Event Loop: The event loop monitors both the call stack and the callback queue. If the call stack is empty, it picks functions from the callback queue and pushes them onto the call stack for execution.
Callback Queue: This queue holds the functions that are ready to be executed after the current operation completes. For example, callbacks from asynchronous operations like setTimeout, network requests, or I/O tasks are placed here.
Task Phases: The event loop works in different phases (timers, pending callbacks, idle, poll, check, close callbacks), each handling specific types of tasks. Understanding these phases helps in writing efficient code and debugging issues.
My First Encounter with the Event Loop
Early in my Node.js journey, I was tasked with building a server that could handle a high volume of incoming HTTP requests. Initially, everything was running smoothly during the development phase, but as I started testing with more concurrent users, I noticed some delays and unexpected behavior.
This led me to dive deeper into the event loop's workings. By understanding how the event loop processes tasks, I was able to identify bottlenecks and restructure my code to better utilize Node.js's asynchronous capabilities.
The Event Loop in Action: A Simple Example
To illustrate how the event loop works, consider this simple example:
console.log("Start");
setTimeout(() => {
console.log("Timeout callback");
}, 0);
Promise.resolve().then(() => {
console.log("Promise callback");
});
console.log("End");
What’s the output?
End
Promise callback
Timeout callback
Here’s why:
Synchronous Execution First: The
console.log("Start")
andconsole.log("End")
are executed first because they are synchronous.Microtasks Before Macrotasks:
Promise.resolve().then(...)
adds its callback to the microtask queue, which gets executed before the macrotask queue wheresetTimeout
callbacks are placed.Timeout Callback: Finally, the
setTimeout
callback executes, demonstrating how the event loop prioritizes tasks.
Key Takeaways for Optimizing Node.js Applications
Through my experience and understanding of the event loop, I’ve identified a few best practices to keep in mind:
Avoid Blocking the Event Loop: Long-running, synchronous operations can block the event loop, preventing other callbacks from executing. Use asynchronous APIs whenever possible to ensure the event loop remains unblocked.
Microtasks vs. Macrotasks: Be mindful of how tasks are queued. Microtasks (like Promise callbacks) have higher priority and can execute before macrotasks (like setTimeout callbacks). Overusing microtasks can starve the event loop, leading to performance degradation.
Use setImmediate for Immediate Tasks: If you need to execute code asynchronously but with high priority,
setImmediate
is a good choice. It places the callback in the check phase of the event loop, which runs after the poll phase, making it faster than setTimeout with zero delay.Monitor and Profile: Use Node.js built-in tools like the
--inspect
flag and external profiling tools to monitor the event loop’s behavior. This can help identify bottlenecks and understand how different phases are being utilized.Leverage Worker Threads: For CPU-intensive tasks, consider using worker threads to offload processing without blocking the event loop. This allows you to maintain the non-blocking nature of Node.js while handling more intensive computations.
Conclusion
Understanding the Node.js event loop has been a game-changer for me. It’s not just a technical detail; it’s the heart of how Node.js handles concurrency and performance. By writing code that works well with the event loop, you can build scalable and efficient applications.
Remember, the event loop is what allows Node.js to handle thousands of concurrent connections with a single thread. By keeping the event loop free and optimizing the flow of tasks, you can unlock the full potential of Node.js and build applications that are both responsive and robust.
In your next Node.js project, keep an eye on the event loop. Understand how your tasks are scheduled and executed, and you’ll be well on your way to becoming a Node.js performance guru. Happy coding!
Subscribe to my newsletter
Read articles from SANKALP HARITASH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
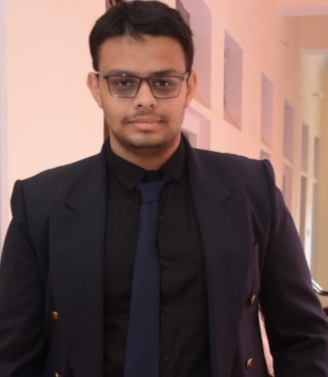
SANKALP HARITASH
SANKALP HARITASH
Hey 👋🏻, I am , a Software Engineer from India. I am interested in, write about, and develop (open source) software solutions for and with JavaScript, ReactJs. 📬 Get in touch Twitter: https://x.com/SankalpHaritash Blog: https://sankalp-haritash.hashnode.dev/ LinkedIn: https://www.linkedin.com/in/sankalp-haritash/ GitHub: https://github.com/SankalpHaritash21 📧 Sign up for my newsletter: https://sankalp-haritash.hashnode.dev/newsletter