Advanced Git and GitHub: A DevOps Engineer's Guide π #Day_10
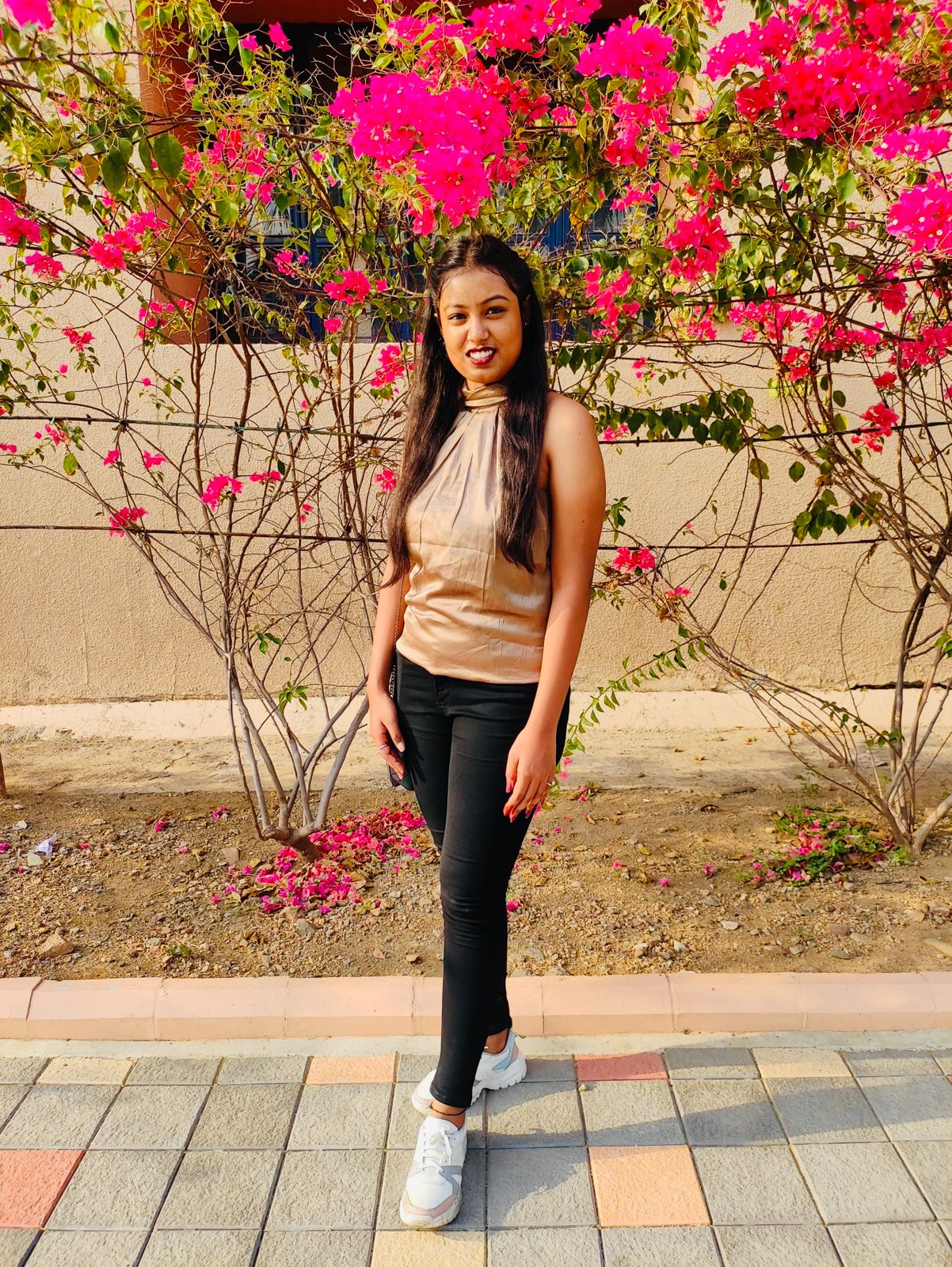
Table of contents

Advanced Git Topics
Introduction
Git is more than just a version control system; it's a tool that can significantly enhance your workflow. In this blog, we'll dive into advanced Git commands that every DevOps engineer should know. From cherry-picking commits to resolving merge conflicts, weβll cover it all with simple explanations and practical examples.
1. Git Cherry-Pick π: Selective Commit Application
Use Case: You made a critical bug fix on one branch and need to apply it to another branch without merging all the changes
Example:
git checkout feature-branch
git cherry-pick <commit-hash>
This command takes a specific commit (identified by <commit-hash>
) from one branch and applies it to the current branch. It's like picking cherriesβonly the ones you want!
2. Git Merge π: Combining Branches
Use Case: Youβve finished working on a feature in a side branch and now want to integrate it into the main branch.
Example:
git checkout main
git merge feature-branch
This command merges the changes from feature-branch
into main
. If the changes are straightforward, Git handles it automatically.
3. Git Merge Conflict βοΈ: Resolving Overlapping Changes
Use Case: Two developers made changes to the same file on different branches, and now those changes conflict.
Example:
# After a merge conflict
git status
Git will indicate the conflicting files. Open those files, resolve the conflicts by choosing the correct changes, and then:
git add <file>
git commit -m "Resolved merge conflict in <file>"
Conflicts are manually resolved by editing the file, then marking the conflict as resolved with git add
.
4. Git Stash ποΈ: Saving Uncommitted Changes Temporarily
Use Case: You're in the middle of a task, but need to switch branches without committing your work.
Example:
git stash save "WIP: working on feature X"
git checkout another-branch
# Do some work on another-branch
git checkout feature-branch
git stash pop
git stash
saves your uncommitted changes so you can work on something else and then bring those changes back with git stash pop
.
5. Git Squash π: Combining Multiple Commits
Use Case: You have multiple small commits that you want to combine into one before merging to the main branch.
Example:
git rebase -i HEAD~3
In the interactive rebase interface, mark the commits you want to squash with s
or squash
. After saving and closing the editor, Git will combine these commits.
6. Git Rearrange π: Reordering Commits
Use Case: You want to change the order of commits before pushing them to the repository.
Example:
git rebase -i HEAD~3
In the interactive rebase editor, you can rearrange the order of the commits by moving them up or down in the list.
7. Git Reset π§Ή: Reverting to a Previous State
Use Case: You want to undo some changes in your working directory or the entire commit history.
Example:
Soft Reset:
git reset --soft HEAD~1
Moves the HEAD back to the previous commit but keeps the changes in the staging area.
Mixed Reset (default):
git reset HEAD~1
Moves the HEAD back and unstages the changes.
Hard Reset:
git reset --hard HEAD~1
Completely reverts to the previous commit, removing all changes.
8. Git Clean π§½: Removing Untracked Files
Use Case: Your working directory is cluttered with untracked files that you donβt need.
Example:
git clean -f
This command removes untracked files, helping you keep your working directory tidy.
9. Git Reflog π: Recovering Lost Commits
Use Case: You accidentally reset or lost some commits and want to recover them.
Example:
git reflog
This command shows a log of all your actions in Git, allowing you to find the commit hash of lost commits and recover them.
10. Git Ignore π«: Excluding Files from Version Control
Use Case: You want to exclude files like compiled binaries or sensitive data from being tracked by Git.
Example:
Create a .gitignore
file in your repository and add the files or directories you want to ignore:
# Ignore all .log files
*.log
# Ignore the secrets directory
secrets/
Git will now ignore any files or directories listed in .gitignore
.
11. Git Rebase π: Streamlining Your Commit History
Use Case: You want to clean up your commit history before merging it into the main branch.
Example:
git checkout feature-branch
git rebase main
This command rewinds the changes in feature-branch
, applies the latest changes from main
, and then re-applies your changes on top. It makes the commit history linear and easier to read.
12. Git Fetch π: Updating Local Data from Remote Repositories
Use Case: You want to update your local repository with the latest changes from the remote repository without merging them into your current branch.
Example:
git fetch origin
This command downloads the latest changes from the remote repository but doesnβt merge them. Itβs a safe way to update your data without affecting your working branch.
13. Gist π: Sharing Code Snippets
Use Case: You want to share small snippets of code or configuration with others, without setting up a full repository.
Example:
You can create a gist directly on GitHub by visiting gist.github.com and pasting your code. Gists can be public or private, and they are a great way to share code quickly.
Advanced GitHub Topics π
To elevate your Git workflow and make the most of GitHub's robust features, let's dive into some advanced topics. These tools and features will significantly improve your efficiency, streamline your development process, and enhance collaboration within your team.
1. GitHub Actions βοΈ: Automate CI/CD Pipelines
GitHub Actions allows you to automate, customize, and execute your software development workflows directly in your GitHub repository. With GitHub Actions, you can build, test, and deploy your code right from GitHub.
Use Case: Automating a Continuous Integration (CI) pipeline.
Example: Imagine you're working on a Node.js project. Every time you push code to the
main
branch, you want to ensure that your tests pass before the code is deployed. With GitHub Actions, you can create a workflow that runs your tests automatically.yamlCopy codename: Node.js CI on: push: branches: [ main ] jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - name: Set up Node.js uses: actions/setup-node@v2 with: node-version: '14' - run: npm install - run: npm test
Real-Time Example: Your team is working on an e-commerce website. By using GitHub Actions, every time a developer pushes a change, the CI workflow automatically runs all unit tests and reports back on whether the changes broke any functionality. If all tests pass, the code can be safely deployed.
2. GitHub Branch Protection π‘οΈ: Enforce Code Quality
GitHub Branch Protection rules help maintain code quality and safeguard your important branches, like main
or production
, by enforcing standards before code can be merged.
Use Case: Ensuring that code is reviewed before merging.
Example: You want to protect your
main
branch by requiring at least one code review before any pull request (PR) is merged. Additionally, you can enforce status checks, like requiring all CI tests to pass.Real-Time Example: On a large team working on a critical project, you protect the
main
branch by requiring at least two reviews and passing all automated tests. This ensures that no buggy or unreviewed code gets merged, maintaining the stability of your codebase.
3. GitHub Pages π: Host Static Sites or Documentation
GitHub Pages is a feature that allows you to host static websites directly from your GitHub repository. It's perfect for hosting project documentation, personal websites, or even full-fledged web applications.
Use Case: Hosting project documentation.
Example: Your open-source library needs user-friendly documentation. By using GitHub Pages, you can generate and host your documentation directly from the
docs
directory of your repository.Real-Time Example: You maintain a Python library and use GitHub Pages to host a well-organized documentation site. Contributors and users can easily access the documentation at
https://username.github.io/repository-name
, helping them understand how to use your library effectively.
4. GitHub CLI π»: Manage GitHub Repositories from the Terminal
The GitHub CLI (Command Line Interface) is a powerful tool that allows you to interact with GitHub directly from your terminal. You can create issues, open pull requests, view repository details, and more without leaving your command line environment.
Use Case: Creating a pull request from the terminal.
Example: After finishing a feature branch, you want to open a pull request to merge your changes into the
main
branch. Using the GitHub CLI, you can do this directly from your terminal.gh pr create --title "Add new feature" --body "This PR adds a new feature that..."
Real-Time Example: As a developer who prefers working from the terminal, you use the GitHub CLI to open pull requests and review issues without needing to switch to a web browser, streamlining your workflow.
5. GitHub Projects π: Organize Tasks and Issues
GitHub Projects allows you to create Kanban-style boards to manage tasks, issues, and pull requests. It's a flexible project management tool that integrates directly with your GitHub repository.
Use Case: Tracking development progress with a Kanban board.
Example: Your team is developing a new feature and needs to track the progress of multiple tasks. You create a GitHub Project board, with columns for "To Do," "In Progress," and "Done." Issues and pull requests move across the board as work progresses.
Real-Time Example: Your team is working on a new mobile app. You use GitHub Projects to manage the development process, creating tasks for each feature and bug fix. As team members work on different parts of the project, the tasks move across the board, giving everyone a clear view of progress.
Conclusion
Mastering advanced Git and GitHub topics enhances your version control skills and streamlines collaboration. From efficient commit management with Git commands to automating workflows and enforcing code quality with GitHub, these tools empower you to handle complex scenarios with ease. Embrace these features to boost productivity and elevate your development process. π
Subscribe to my newsletter
Read articles from Vaishnavi Modakwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
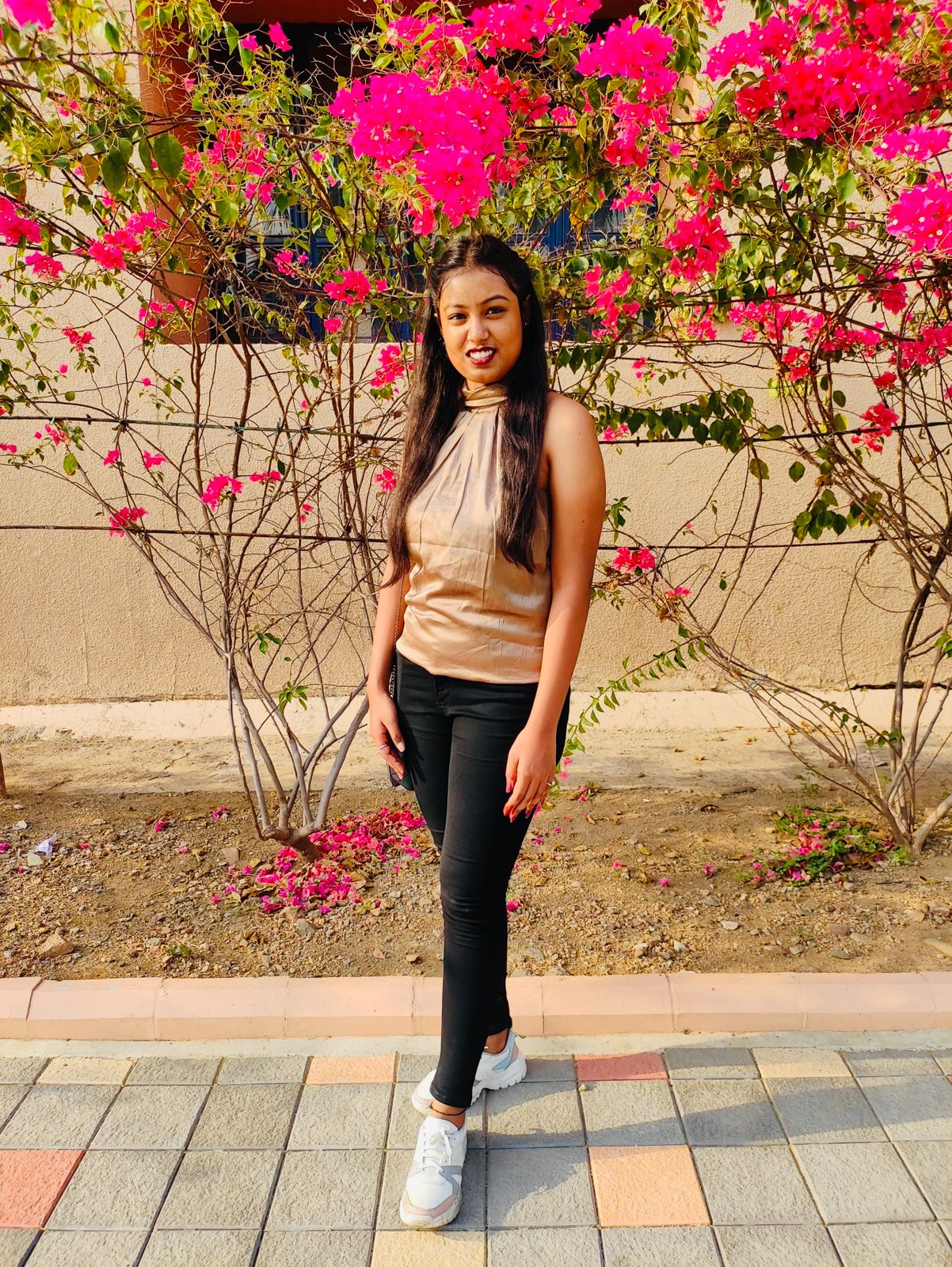
Vaishnavi Modakwar
Vaishnavi Modakwar
π Hi there! I'm Vaishnavi Modakwar, a dedicated DevOps and Cloud Engineer with 2 years of hands-on experience in the tech industry. My journey in DevOps has been fueled by a passion for optimizing and automating processes to deliver high-quality software efficiently. Skills: Cloud Technologies: AWS, Azure. Languages: Python, YAML, Bash Scripting. Containerization: Docker, ECS, Kubernetes. IAC: Terraform, Cloud Formation. Operating System: Linux and MS Windows. Tools: Jenkins, Selenium, Git, GitHub, Maven, Ansible. Monitoring: Prometheus, Grafana. I am passionate about demystifying complex DevOps concepts and providing practical tips on automation and infrastructure management. I believe in continuous learning and enjoy keeping up with the latest trends and technologies in the DevOps space. π On my blog, you'll find tutorials, insights, and stories from my tech adventures. Whether you're looking to learn about CI/CD pipelines, cloud infrastructure, or containerization, my goal is to share knowledge and inspire others in the DevOps community. Let's Connect: I'm always eager to connect with like-minded professionals and enthusiasts. Feel free to reach out for discussions, collaborations, or feedback. Wave me at vaishnavimodakwar@gmail.com