Mastering Ether Transfer on the Ethereum Blockchain
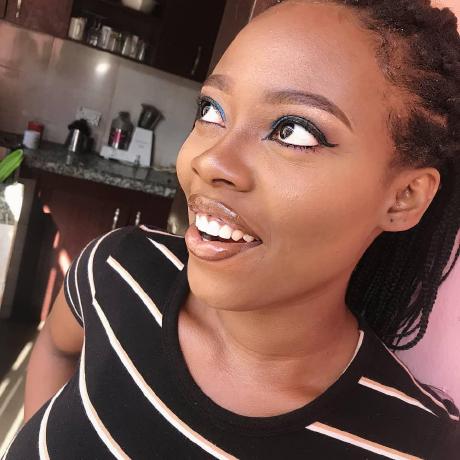
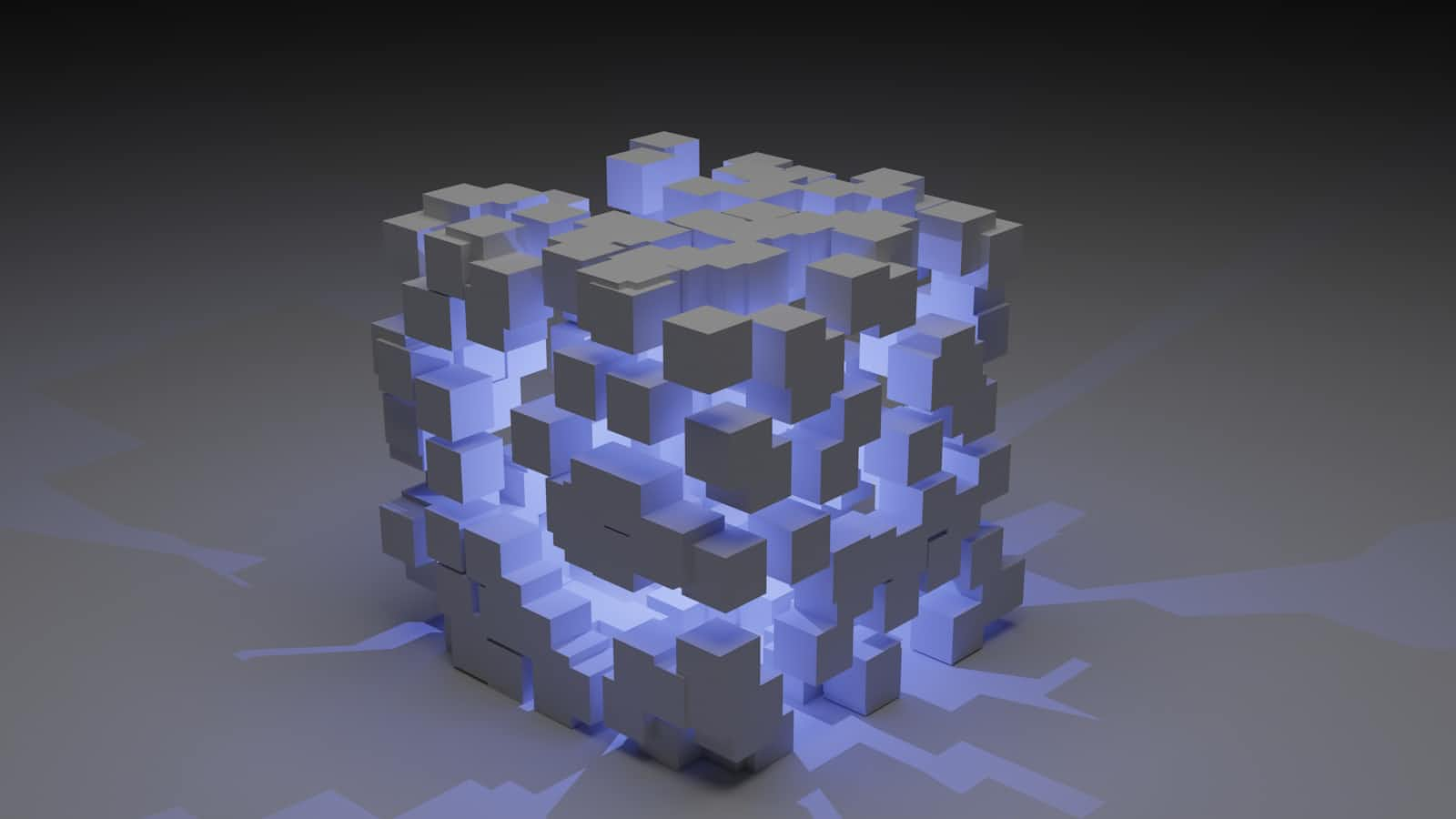
One of the fundamental operations of any blockchain is the ability to transfer its native cryptocurrency. Ethereum is no exception. Over time, new methods have been introduced to solve the problem of Ether transfer, each with its specific use cases and security implications.
This article delves into the three primary methods—send
, transfer
, and call
—exploring their unique characteristics, advantages, and potential pitfalls.
The 'send' method
The send method is the oldest method of carrying out Ether transfer from a contract account. This method sends the specified amount of ether and a fixed gas limit of 2300 gas to the receiving contract. This gas limit is only enough for logging the transaction or emitting an event and it becomes a problem when the receiving contract requires more gas to execute complex logic. The send method does not throw an exception when it fails. Instead, it returns a status of false without reverting any state changes. This requires manual error handling.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.7;
contract SendEther {
function sendEtherViaSend(address payable _to) public payable {
bool success = _to.send(msg.value); // Returns false on failure
require(success, "Failed to send Ether");
}
}
The 'transfer' method
The transfer method was introduced as a safer alternative to 'send' following the DAO hack. Like 'send', 'transfer' has a fixed gas limit of 2300 gas that is forwarded to the receiving contract. While it benefits from protection against reentrancy attacks due to the small gas limit, it also shares the limitation of being unable to transfer ether to contracts that require complex computation. The major way it differs from 'send' is in its ability to revert all state changes when the transaction fails.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.7;
contract SendEther {
function sendEtherViaTransfer(address payable _to) public payable {
_to.transfer(msg.value); // Automatically reverts on failure
}
}
The 'call' method
The 'call' method is a low-level function that offers greater flexibility than 'send' and 'transfer'. By default, it forwards all the remaining gas in the transaction to the receiving contract. However, it also allows you to specify the amount of gas to be forwarded. This flexibility ensures that recipient contracts that require complex computations can also receive ether. But it has the downside of being the most susceptible to reentrancy attacks, this downside can easily be overcome when it is used in combination with Checks-Effects-Interaction pattern and reentrancy guards. The 'call' method is similar to 'send' because it requires manual error handling; state changes are not reverted if the transaction fails.
The 'call' method is the recommended way of transferring ether due to several reasons:
The call method allows for the customization of gas limit. Being able to specify the amount of gas forwarded, allows the recipient contract to execute complex computation without running out of gas.
The call method returns a boolean indicating if the transfer was successful or not, allowing the developer to implement custom error handling especially in situations where reverted state changes are undesirable.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.7;
contract SendEther {
function sendViaCall(address payable _to) public payable {
(bool success, bytes memory data) = _to.call{value: msg.value}(""); // Returns false on failure
require(success, "Failed to send Ether");
}
}
Conclusion
Understanding the differences between the send
, transfer
, and call
methods is crucial for secure and efficient Ether transfers in Ethereum smart contracts. Each method has its own strengths and weaknesses, and the choice of which to use depends on the specific requirements of your contract.
Use
send
when you need a simple transfer and are prepared to handle potential failures manually.Use
transfer
for safer, automatic reversion on failure, but be mindful of its fixed gas limitation.Use
call
for maximum flexibility, especially when interacting with complex contracts, but always implement secure coding practices to protect against reentrancy attacks.
By carefully selecting the appropriate method and following best practices, you can minimize risks and ensure that your smart contracts function as intended in the ever-evolving Ethereum ecosystem.
Subscribe to my newsletter
Read articles from Favour Abangwu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
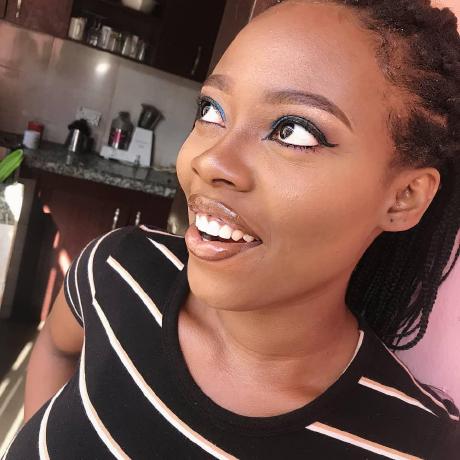