Do Developers Really Use the JavaScript Console? Understanding Its Purpose and Benefits

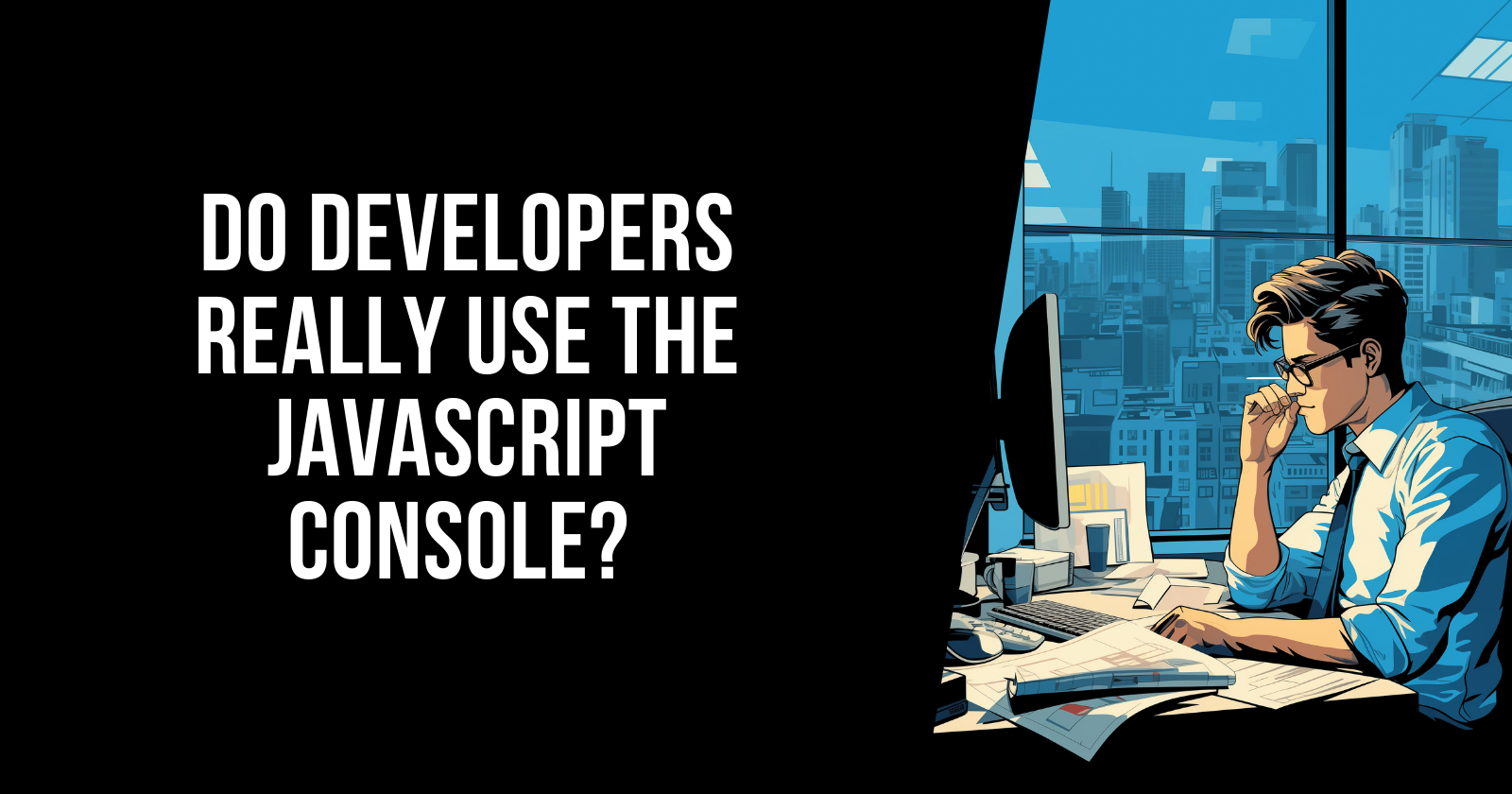
As JavaScript developers, we've all used the console
object at some point in our coding journey. But do we really understand the full capabilities of the JavaScript console
object, or are we just scratching the surface by using a small fraction of its functions in our code?
In the day-to-day life of a JavaScript developer, we mostly use the console
object for logging messages or checking the values of variables in our code—much like how we use the printf
function in C or C++. But does the console
object only have those basic capabilities? In reality, the JavaScript console
is much more powerful. In this blog, we will explore various use cases of JavaScript console
and learn how to fully utilize its features to improve our debugging and coding skills. By leveraging the full potential of the console
object, we can build more robust and optimized code.
What is a Javascript Console?
The JavaScript console
is a global object that provides various methods to interact with the console API, commonly used for debugging tasks. It is part of the global window
object in JavaScript. As we mentioned earlier, the console
object offers a range of methods that can significantly aid in debugging our code. In the next sections, we will explore these methods in detail and learn how to leverage them to enhance our debugging skills.
What are the Javascript Console Methods?
Let's explore all the methods of the console
object, along with their respective use cases and a small example for each method:
Message Logging Functions:
The console provides various methods for logging messages, each tailored to specific use cases. These methods help developers debug code more accurately by allowing them to log messages at different levels, measure performance, and track function calls effectively.
We can use these methods to print different messages based on their use cases, which will help us debug and understand the code more accurately. Although we can use only the
console.log()
method for logging, relying too much on it can clutter the console and make it hard to identify specific issues among many log messages. By using these various methods appropriately, we can print messages in a standardized format, making it easier to find bugs and issues. This approach improves code readability and helps maintain organized and effective debugging practices.console.log()
:The
console.log()
method is a simple logging function that is widely used by JavaScript developers. It prints messages or variable values passed into the function, making it a useful tool for debugging.console.log("Hello, world!"); const user ={ name : "John" age : 18 gender: 'Male' } console.log(user)
In the example above, we use the
console.log()
function to print a message ("Hello, world!"
) and theuser
object to the console. These messages will appear in the console's default output.console.info()
:The
console.info()
method is used to output a message at the "info" level in the JavaScript console. This method is similar toconsole.log()
, but it provides an informational icon (usually a small "i") before the message, indicating that it's informational. This type of message will only appear if the console is configured to show info-level messages.console.info("The user data has been successfully loaded."); const serverStatus = { server: "Online", uptime: "24 hours", load: "Moderate" }; console.info("Server Status:", serverStatus);
In the example above, we use
console.info
()
to log an informational message ("The user data has been successfully loaded."
) and to display theserverStatus
object. These messages will appear in the console with an "i" icon, distinguishing them from regular log messages.console.warn():
The
console.warn()
method is used to output a message at the "warning" level in the JavaScript console. Messages logged usingconsole.warn()
receive special formatting, such as yellow text and a warning icon, to make them stand out. Similar to theconsole.info()
function, warning messages will only appear if the console is configured to display warn-level messages.console.warn("This feature is deprecated and will be removed in the next release."); const userInput = ""; if (userInput === "") { console.warn("User input is empty. Default values will be used."); }
In the example above, we use
console.warn()
to log a warning about a deprecated feature and to alert about empty user input. These warnings will be displayed in the console with a yellow warning icon, making them easily noticeable.console.error()
:The
console.error()
method is used to output messages at the "error" level in the JavaScript console. Messages logged usingconsole.error()
receive special formatting, such as red text and an error icon, to make them easily noticeable. Similar to theconsole.info()
function, error messages will only appear if the console is configured to display error-level messages.console.error("Unable to connect to the server. Please try again later."); const data = null; if (!data) { console.error("Data retrieval failed: No data found."); }
In the example above, we use
console.error()
to log an error message when a server connection fails and when no data is found. These error messages will be displayed in the console with red text and an error icon, highlighting the issues that need immediate attention.console.debug():
The
console.debug()
method is used to output messages at the "debug" log level. These messages are typically used for debugging purposes and are only displayed if the console is configured to show debug-level output. This log level often corresponds to a "Debug" or "Verbose" setting, allowing developers to see detailed information while troubleshooting.const user = { id: 1, name: "John Doe" }; console.debug("User data loaded:", user); const isFeatureEnabled = false; console.debug("Feature enabled status:", isFeatureEnabled);
In the example above, we use
console.debug()
to log detailed information about theuser
object and the status of a feature flag. These debug messages will only appear in the console if the debug level logging is enabled, helping developers gain insight into the application's internal state during development.console.assert()
:Many times in our JavaScript code, we use
if
condition blocks just to log a message using theconsole.log()
function. This approach can make the code unnecessarily long and cluttered. Instead of using anif
block, we can use theconsole.assert()
function. Theconsole.assert()
function takes two arguments: the first is a boolean condition, and the second is a message or variable value that will be logged only if the condition isfalse
.const age = 15; console.assert(age >= 18, "User is not old enough to vote:", age);
In the example above, we use
console.assert()
to check if theage
is greater than or equal to 18. If the condition isfalse
(in this case, age is 15), the message"User is not old enough to vote:"
along with the actual age will be logged to the console.
Operation Time calculation Function:
After developing functionality in a project, we often need to optimize the code to scale and manage a large number of users. To determine how much time a specific block of code takes to execute, we can use console functions. These functions are widely used to optimize JavaScript code, helping developers identify performance bottlenecks and improve efficiency.
console.time()
:The
console.time()
method starts a timer you can use to track how long an operation takes. It starts a new timer with a name specified as a string, which can be used to measure the duration of a block of code.console.timeEnd():
The
console.timeEnd()
method stops the timer that was started withconsole.time()
and logs the elapsed time to the console. It takes the same string name of the timer as an argument, ensuring you can track different timers simultaneously.console.timeLog():
The
console.timeLog()
method logs the current value of the timer without stopping it. This is useful for checking how much time has elapsed at certain points within a longer process.console.timeStamp():
The
console.timeStamp()
method adds a timestamp to the browser’s performance entry buffer with an optional name. This method is often used for marking points in the code for performance analysis and debugging. Note that this method might not log anything directly in the console, but it marks the timestamps for performance profiling tools.
console.time("process");
console.timeStamp("Start");
setTimeout(() => {
console.timeLog("process", "Halfway through");
setTimeout(() => {
console.timeStamp("End");
console.timeEnd("process");
}, 1000);
}, 1000);
In Above example, we can use console.time()
to start a timer with a specific label, like "process"
, to measure how long a block of code takes. For instance, you might use console.timeStamp("Start")
to mark the beginning of a process. As the code executes, you can use console.timeLog("process", "Halfway through")
to log the elapsed time so far along with a custom message. Finally, when the process completes, console.timeStamp("End")
marks the end, and console.timeEnd("process")
stops the timer and logs the total elapsed time. This approach helps you track performance, identify bottlenecks, and analyze execution times effectively.
Counter Functions:
In JavaScript, when dealing with multiple function calls, it can be challenging to keep track of how many times each function is invoked. If you're unaware that the console provides built-in counter functions, you might resort to creating custom counters, which are often basic and temporary solutions. Instead of implementing such custom counters for each function, you can use the console counter functions.
console.count():
The
console.count()
method is a built-in method that automatically counts the number of times it is called with a specific label. This simplifies tracking function calls by eliminating the need for custom counter logic. Each timeconsole.count()
is invoked with a given label, it increments the count for that label and logs the current count to the console, making it easier to monitor how many times a particular function or code block has been executed.console.countReset():
The
console.countReset()
method is a built-in function that resets the counter for a specific label. By callingconsole.countReset()
with a given label, you can reset the count for that label back to zero. This is useful when you want to start counting from scratch for a specific function or code block, allowing for more precise tracking and analysis of function calls.
function processItem(item) {
console.count("processItem call");
if (item === "reset") {
console.countReset("processItem call");
}
}
processItem("apple");
processItem("banana");
processItem("reset");
processItem("cherry");
processItem("date");
The console.count()
and console.countReset()
methods are useful for tracking and managing function call counts in JavaScript. For instance, you can use console.count("processItem call")
to keep track of how many times the processItem
function is invoked with the label "processItem call"
. If you need to reset this count, you can call console.countReset("processItem call")
, which resets the counter for that label back to zero. For example, if processItem("apple")
and processItem("banana")
are called, the console will show counts of 1 and 2 respectively. After calling processItem("reset")
, the counter is reset, so subsequent calls like processItem("cherry")
and processItem("date")
will start from 1 again, showing updated counts.
console group functions:
As developers, we often use various console logging methods to print messages in different parts of our code. However, this can lead to difficulty in identifying which messages correspond to which code blocks. To address this issue, the console object provides grouping methods. By using
console.group
()
,console.groupCollapsed()
, andconsole.groupEnd()
, you can group related log messages together. This organization makes it easier to trace and debug specific code blocks, allowing for quicker identification and resolution of issues compared to the traditional approach.console.group():
The
console.group()
method creates a new inline group in the console. It indents all subsequent console messages untilconsole.groupEnd()
is called. This is useful for grouping related log messages together, making it easier to manage and analyze logs related to a specific part of your code.console.groupEnd():
The
console.groupEnd()
method closes the most recently opened group created byconsole.group()
orconsole.groupCollapsed()
. It ends the indentation for that group, and subsequent log messages will not be indented.console.groupCollapsed():
he
console.groupCollapsed()
method creates a new group in the console, similar toconsole.group
()
, but with the group initially collapsed. This means that the group’s logs are hidden by default, and you have to expand the group manually in the console to view the messages. This is useful for keeping the console output tidy when you have a lot of log messages.
console.group("User Details");
console.log("Name: John Doe");
console.log("Age: 30");
console.groupCollapsed("Address");
console.log("City: New York");
console.log("State: NY");
console.groupEnd();
console.group("Contacts");
console.log("Email: john.doe@example.com");
console.log("Phone: (123) 456-7890");
console.groupEnd();
In this example, console.group("User Details")
starts a new group labeled "User Details" and indents all logs within it. Inside this group, console.groupCollapsed("Address")
creates a collapsed subgroup labeled "Address," so its contents are hidden by default. After console.groupEnd()
closes the "Address" group, console.group
("Contacts")
starts another group for contact details. Each console.groupEnd()
call ends the corresponding group, returning the log indentation to its previous level. This organization helps keep related logs together and makes it easier to navigate and understand the console output.
Special Functions of Console:
console.table():
The
console.table()
method displays data in a tabular format, which is especially useful for visualizing arrays or objects. It provides a clear, structured view of data with columns and rows.const users = [ { name: "Alice", age: 25, city: "New York" }, { name: "Bob", age: 30, city: "San Francisco" }, { name: "Charlie", age: 35, city: "Chicago" } ]; console.table(users);
In this example,
console.table(users)
outputs theusers
array as a table in the console, allowing you to see each user’s details in a clear, tabular format.console.dir():
The
console.dir()
method displays an interactive list of the properties of a specified JavaScript object. This method is useful for inspecting the structure of objects and their properties in depth.const person = { name: "John Doe", age: 30, address: { street: "123 Main St", city: "Los Angeles" } }; console.dir(person);
In this example,
console.dir(person)
provides a detailed, expandable view of theperson
object, including its properties and nested objects, in the console. This helps in inspecting and debugging complex objects.console.trace():
he
console.trace()
method outputs a stack trace to the console, showing the path the code execution took to reach the point whereconsole.trace()
was called. It’s useful for debugging by tracking the sequence of function calls.function foo() { bar(); } function bar() { console.trace("Trace log"); } foo();
In this example, calling
console.trace("Trace log")
inside thebar()
function outputs a stack trace that shows the sequence of function calls leading tobar()
, which includesfoo()
as the caller. This helps in tracing the flow of execution and debugging the code path.
We’ve explored various methods of the console object. While many developers are familiar with basic methods like console.log()
, they might not fully utilize the other powerful methods available. By incorporating methods such as console.table()
, console.dir()
, console.trace()
, and console grouping functions into your coding practice, you can create more robust and optimized code. These methods enhance debugging capabilities, making your code easier to understand and maintain for other developers.
In conclusion, I encourage you to experiment with these console methods, review their outputs, and integrate them into your daily coding routine. This practice will sharpen your debugging skills and help you build more efficient and comprehensible code.
Subscribe to my newsletter
Read articles from Jaydev Jadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Jaydev Jadav
Jaydev Jadav
I am a passionate software developer specializing in Software development. With a strong focus on programming, development, cloud technologies, Artificial Intelligent, Machine Learning and application integration