How to Create an Intelligent Image Recognition System with Python
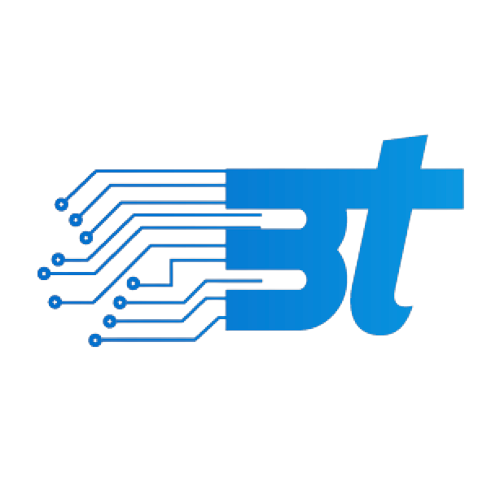
Table of contents
- Prerequisites
- Step 1: Understanding Image Recognition Basics
- Step 2: Prepare the Dataset
- Step 3: Preprocess the Data
- Step 4: Build the Convolutional Neural Network (CNN)
- Step 5: Train the Model
- Step 6: Evaluate the Model
- Step 7: Visualize the Training Process
- Step 8: Make Predictions
- Step 9: Final code
- Load and Use the Saved Model
- Predict an Image from a File Path
- Example Code to Predict an Image from a File Path
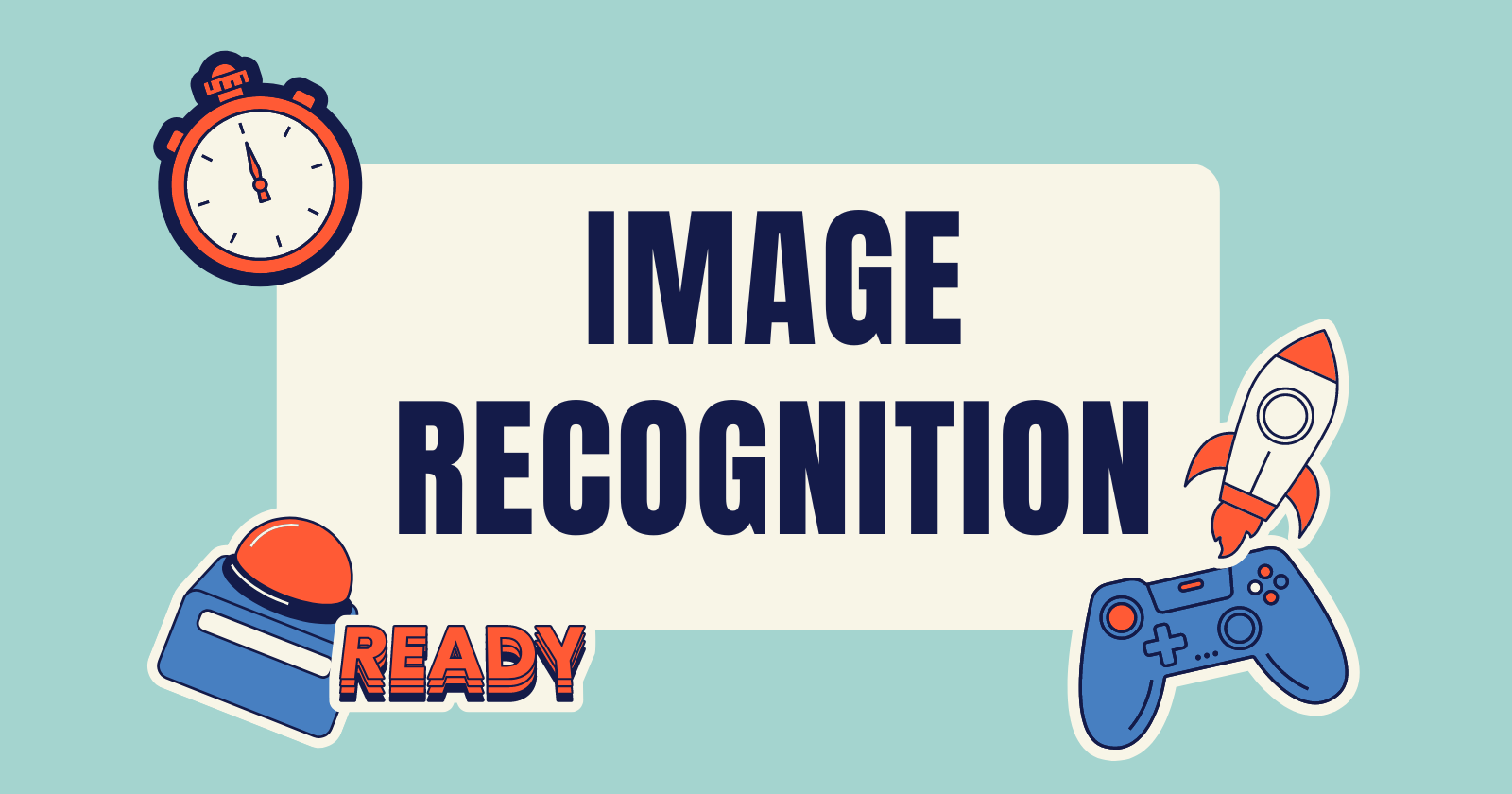
Image recognition has become a key feature in many applications, from social media platforms that tag friends in photos to autonomous vehicles that detect obstacles. Creating an intelligent image recognition system involves leveraging deep learning and computer vision techniques to identify objects, people, or even activities in images. In this guide, we'll walk through building a basic image recognition system using Python, TensorFlow, and Keras.
Prerequisites
Before we dive into the code, ensure you have the following prerequisites installed:
Python 3.x
TensorFlow
Keras (now integrated into TensorFlow)
OpenCV
NumPy
Matplotlib
Jupyter Notebook (optional for interactive development)
You can install these dependencies using pip:
pip install tensorflow opencv-python numpy matplotlib
Step 1: Understanding Image Recognition Basics
Image recognition involves classifying images into predefined categories. The core idea is to train a model that can understand patterns and features in images, such as shapes, colors, and textures, to accurately classify new images.
Step 2: Prepare the Dataset
For this guide, we'll use a popular image dataset called CIFAR-10, which contains 60,000 32x32 color images in 10 classes, with 6,000 images per class.
from tensorflow import keras
from keras.datasets import cifar10
import matplotlib.pyplot as plt
# Load the CIFAR-10 dataset
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
# Display a few images from the dataset
fig, axes = plt.subplots(1, 5, figsize=(10, 2))
for i, ax in enumerate(axes):
ax.imshow(x_train[i])
ax.axis('off')
plt.show()
Step 3: Preprocess the Data
Data preprocessing is crucial in deep learning to ensure the model learns effectively. This includes normalizing the pixel values and converting labels to one-hot encoding.
from tensorflow import keras
from keras.utils import to_categorical
from keras.datasets import cifar10
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
# Normalize pixel values to be between 0 and 1
x_train = x_train.astype('float32') / 255.0
x_test = x_test.astype('float32') / 255.0
# Convert labels to one-hot encoding
y_train = to_categorical(y_train, 10)
y_test = to_categorical(y_test, 10)
Step 4: Build the Convolutional Neural Network (CNN)
A Convolutional Neural Network (CNN) is highly effective for image recognition tasks because it can capture spatial hierarchies in images. We will build a simple CNN model using Keras.
from tensorflow import keras
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout
# Initialize the CNN
model = Sequential()
# Add convolutional layers
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)))
model.add(MaxPooling2D((2, 2)))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D((2, 2)))
model.add(Conv2D(128, (3, 3), activation='relu'))
model.add(MaxPooling2D((2, 2)))
# Flatten the layers and add dense layers
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(10, activation='softmax'))
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
Step 5: Train the Model
Training the model involves feeding the training data into the model and allowing it to learn the patterns.
# Train the model
history = model.fit(x_train, y_train, epochs=10, batch_size=64, validation_data=(x_test, y_test))
Step 6: Evaluate the Model
After training, evaluate the model's performance on the test set to see how well it generalizes to new, unseen data.
# Evaluate the model on the test data
test_loss, test_accuracy = model.evaluate(x_test, y_test)
print(f'Test accuracy: {test_accuracy:.2f}')
Step 7: Visualize the Training Process
Visualizing the training process can help us understand if the model is learning correctly and if there are any signs of overfitting.
# Plot the training and validation accuracy and loss
plt.figure(figsize=(12, 4))
plt.subplot(1, 2, 1)
plt.plot(history.history['accuracy'], label='Training Accuracy')
plt.plot(history.history['val_accuracy'], label='Validation Accuracy')
plt.title('Accuracy')
plt.legend()
plt.subplot(1, 2, 2)
plt.plot(history.history['loss'], label='Training Loss')
plt.plot(history.history['val_loss'], label='Validation Loss')
plt.title('Loss')
plt.legend()
plt.show()
Step 8: Make Predictions
Use the trained model to make predictions on new images.
import numpy as np
# Make predictions on the test set
predictions = model.predict(x_test)
# Display a few test images with their predicted and true labels
fig, axes = plt.subplots(1, 5, figsize=(10, 2))
for i, ax in enumerate(axes):
ax.imshow(x_test[i])
ax.axis('off')
ax.set_title(f"Pred: {np.argmax(predictions[i])}, True: {np.argmax(y_test[i])}")
plt.show()
Step 9: Final code
Here is the complete Python code to create an intelligent image recognition system using the CIFAR-10 dataset. This code includes loading and preprocessing the dataset, building a convolutional neural network (CNN), training the model, and evaluating its performance.
from tensorflow import keras
from keras.datasets import cifar10
from keras.utils import to_categorical
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout
from keras.optimizers import Adam
# Load the CIFAR-10 dataset
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
# Normalize pixel values to be between 0 and 1
x_train = x_train.astype('float32') / 255.0
x_test = x_test.astype('float32') / 255.0
# Convert labels to one-hot encoding
y_train = to_categorical(y_train, 10)
y_test = to_categorical(y_test, 10)
# Build the CNN model
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Conv2D(128, (3, 3), activation='relu'),
Flatten(),
Dense(128, activation='relu'),
Dropout(0.5),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer=Adam(learning_rate=0.001),
loss='categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(x_train, y_train, epochs=10, batch_size=64,
validation_data=(x_test, y_test))
# Evaluate the model on test data
test_loss, test_acc = model.evaluate(x_test, y_test, verbose=2)
print(f"Test accuracy: {test_acc:.2f}")
# Save the trained model
model.save('cifar10_cnn_model.h5')
Load and Use the Saved Model
from tensorflow import keras
from keras.models import load_model
import numpy as np
from keras.datasets import cifar10
# Load the saved model
model = load_model('cifar10_cnn_model.h5')
# Load the CIFAR-10 test dataset
(_, _), (x_test, y_test) = cifar10.load_data()
# Normalize pixel values
x_test = x_test.astype('float32') / 255.0
# Make predictions on the test data
predictions = model.predict(x_test)
# Display the predicted and actual labels for the first 10 test images
for i in range(10):
predicted_label = np.argmax(predictions[i])
actual_label = y_test[i][0]
print(
f"Test Image {i + 1}: Predicted label = {predicted_label}, Actual label = {actual_label}")
Predict an Image from a File Path
Load the Required Libraries: You will need
PIL
(Python Imaging Library) or its forkPillow
to load and process images.Load and Preprocess the Image: The image needs to be resized and normalized in the same way as the training data.
Predict the Image Class: Use the trained model to predict the class of the loaded image.
Example Code to Predict an Image from a File Path
First, make sure you have installed Pillow
, which is necessary for handling images:
pip install Pillow
Now, let's add code to load an image from a file path and make predictions:
from tensorflow import keras
from keras.models import load_model
import numpy as np
from keras.preprocessing import image
from PIL import Image
# Load the saved model
model = load_model('cifar10_cnn_model.h5')
# Function to load and preprocess an image
def load_and_preprocess_image(img_path):
# Load the image with the target size of 32x32 pixels (as CIFAR-10 images are 32x32)
img = Image.open(img_path).resize((32, 32))
# Convert the image to a numpy array
img_array = np.array(img)
# Normalize the image data to the range [0, 1]
img_array = img_array.astype('float32') / 255.0
# Expand dimensions to match the model input shape (1, 32, 32, 3)
img_array = np.expand_dims(img_array, axis=0)
return img_array
# Load and preprocess the image from the specified path
img_path = '/Applications/projects/apps/image-recognize/image.png'
processed_image = load_and_preprocess_image(img_path)
# Predict the class of the image
prediction = model.predict(processed_image)
predicted_class = np.argmax(prediction)
# CIFAR-10 class names
class_names = ['airplane', 'automobile', 'bird', 'cat', 'deer',
'dog', 'frog', 'horse', 'ship', 'truck']
# Print the predicted class
print(f"Predicted class: {class_names[predicted_class]}")
Step 10: Improve the Model
To further improve the model's performance, consider experimenting with different architectures, adding more layers, using data augmentation, or tuning hyperparameters such as learning rate and batch size.
Conclusion
With this guide, you should now have a basic understanding of how to create an image recognition system using Python and deep learning libraries like TensorFlow and Keras. Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
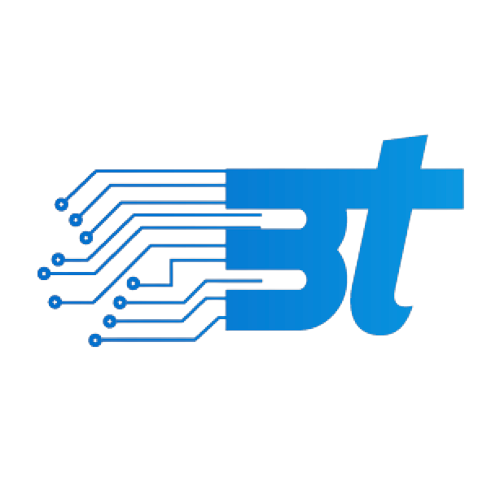
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.