Understanding CommonJS (CJS) and ES Modules (ESM) in JavaScript

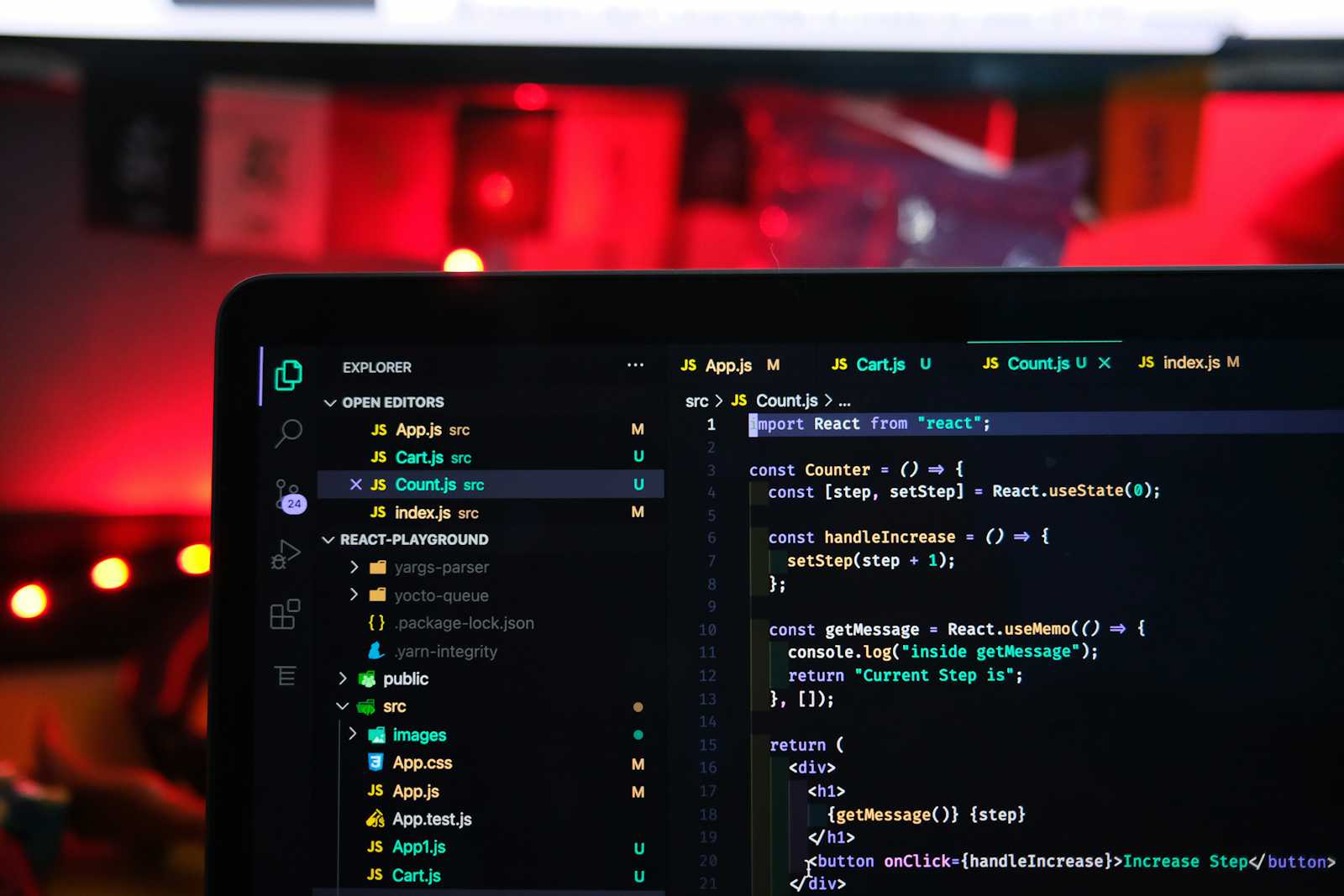
Let's understand what module is!
In the world of JavaScript, modules play a crucial role in organizing and structuring our code. They allow us to break down complex applications into manageable pieces, promote reusability, and enhance maintainability. Let’s explore two important types of modules: CommonJS (CJS) and ES Modules (ESM).
Common JS modules VS. ES Modules
CommonJS (CJS) Modules (The Old Guard)
Syntax:
From module1: module.exports = {abc, xyz}
To module2: const {abc , xyz} = require("./abcxyz.js")
//FirstModule.js
function FirstModule(a,b){
console.log("sum is " + (a + b))
}
module.exports = {FistModule};
//SecModule.js
const {Sum} = require("./FirstModule");
FirstModule(10,20);
Node.js Default: CommonJS is the default module system in Node.js.
Synchronous Execution: CJS modules load synchronously, which means they block execution until the module is fully loaded.
Non-Strict Mode: Code within CJS modules runs in non-strict mode by default.
ES Modules (ESM) - The Modern Approach
Syntax:
From module1: export default xyz;
To module2: import xyz from "./xyz.js";
// ModuleFirst.js
function ModuleFirst(a, b){
const m = a * b;
console.log(m);
}
export default ModuleFirst;
//ModuleSecond.js
import ModuleFirst from "./ModuleFirst"
ModuleFirst(10 , 20);
Browser and React Default: ES Modules are the default in modern browsers and React applications.
Asynchronous Loading: ESM loads asynchronously, allowing for better performance.
Strict Mode: Code within ESM runs in strict mode, catching potential errors early.
Strict Mode vs. Non-Strict Mode
Strict Mode (ESM): Variables must have a defined data type, and accessing undeclared variables results in an error.
Non-Strict Mode (CJS): Variables can be accessed regardless of whether they have a data type or are defined.
In the ever-evolving landscape of JavaScript, understanding module systems is crucial. Whether you’re diving into the legacy world of CommonJS or embracing the modern elegance of ES Modules, remember that both have their strengths and use cases. Choose wisely based on your project’s needs and keep exploring the fascinating world of code organization and reusability.
Happy coding, and may your modules always be modular! 🚀
Subscribe to my newsletter
Read articles from Akriti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Akriti
Akriti
“Innovative developer with a passion for continuous learning and expertise in cutting-edge technologies. Proficient in frontend development, particularly ReactJS and Tailwind CSS, and currently expanding my skills in Node.js and backend development. Enthusiastic about creating seamless user experiences and optimizing performance. Always eager to explore new tools and frameworks to stay ahead in the tech world.”