Automate Your AWS Resource Tracking with Simple Shell Scripts
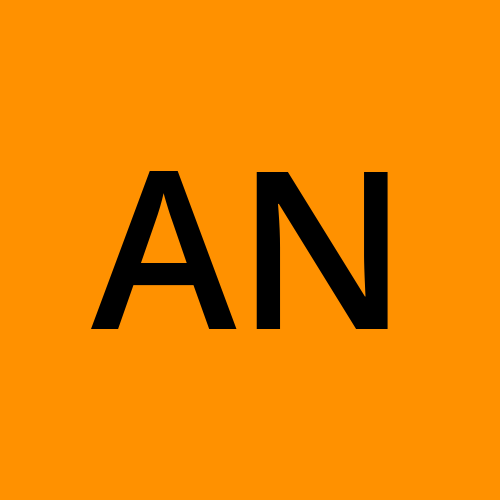
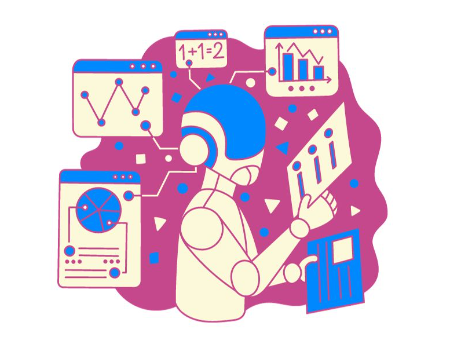
INTRODUCTION
As an AWS user, it's essential to keep track of your resources to ensure efficient resource utilization, cost optimization, and security. Manually tracking resources can be time-consuming and prone to errors. In this blog post, we'll explore how to automate AWS resource tracking using shell scripting.
Problem: AWS provides a vast array of services, and managing resources across these services can be overwhelming.
Without a proper tracking system, it's easy to lose sight of resources, leading to unnecessary costs, security vulnerabilities, and performance issues.
The Solution: We'll create a shell script that automates the process of listing AWS resources across various services, including EC2, RDS, S3, CloudFront, VPC, IAM, Route53, CloudWatch, CloudFormation, Lambda, SNS, SQS, DynamoDB, and EBS.
The Script: Here is the shell script that we'll use to automate AWS resource tracking:
#!/bin/bash
#####################################################################################
#Author: Anas
#Version: v0.0.1
#Script to automate the process of listing all the resources in an AWS account
# Below are the services that are supported by this script :
# 1. EC2
# 2. RDS
# 3. S3
# 4. CloudFront
# 5. VPC
# 6. IAM
# 7. Route53
# 8. CloudWatch
# 9. CloudFormation
# 10. Lambda
# 11. SNS
# 12. SQS
# 13. DynamoDB
# 14. VPC
# 15. EBS
#
# The script will prompt the user to enter the AWS region and the service for which the resources need to be listed
# Usage: ./aws_resource_list.sh <aws_region> <aws_service>
# Example : ./aws_resource_list.sh us-east-1 ec2
########################################################################################
#check if the required number of arguments are passed
if [ $# -ne 2]; then
echo "Usage: ./aws_resource_list.sh <aws_region> <aws_service>"
echo "Example: ./aws_resource_list.sh us-east-1 ec2"
exit 1
fi
# Assign the arguments to variable and convert service to lowercase
aws_region=$1
aws_service=$(echo "$2" | tr '[:upper:]' '[:lower:]')
# Check if the AWS CLI is installed
if ! command -v aws &> /dev/null; then
echo "AWS CLI is not installed. Please install the AWS CLI and try again."
exit 1
fi
# Check if the AWS CLI is configured
if [ ! -d ~/.aws ]; then
echo "AWS CLI is not configured. Please configure the AWS CLI and try again."
exit 1
fi
# List the resources based on the service
case $aws_service in
ec2)
echo "Listing EC2 Instances in $aws_region"
aws ec2 describe-instances --region $aws_region
;;
rds)
echo "Listing RDS Instances in $aws_region"
aws rds describe-db-instances --region $aws_region
;;
s3)
echo "Listing S3 Buckets in $aws_region"
aws s3api list-buckets # here not region bcz s3 is global service
;;
cloudfront)
echo "Listing CloudFront Distributions in $aws_region"
aws cloudfront list-distributions --region $aws_region
;;
vpc)
echo "Listing VPCs in $aws_region"
aws ec2 describe-vpcs --region $aws_region
;;
iam)
echo "Listing IAM Users in $aws_region"
aws iam list-users --region $aws_region
;;
route53)
echo "Listing Route53 Hosted Zones in $aws_region"
aws route53 list-hosted-zones --region $aws_region
;;
cloudwatch)
echo "Listing CloudWatch Alarms in $aws_region"
aws cloudwatch describe-alarms --region $aws_region
;;
cloudformation)
echo "Listing CloudFormation Stacks in $aws_region"
aws cloudformation describe-stacks --region $aws_region
;;
lambda)
echo "Listing Lambda Functions in $aws_region"
aws lambda list-functions --region $aws_region
;;
sns)
echo "Listing SNS Topics in $aws_region"
aws sns list-topics --region $aws_region
;;
sqs)
echo "Listing SQS Queues in $aws_region"
aws sqs list-queues --region $aws_region
;;
dynamodb)
echo "Listing DynamoDB Tables in $aws_region"
aws dynamodb list-tables --region $aws_region
;;
ebs)
echo "Listing EBS Volumes in $aws_region"
aws ec2 describe-volumes --region $aws_region
;;
*)
echo "Invalid service. Please enter a valid service."
exit 1
;;
esac
Prerequisites
AWS CLI installed and configured on your system
To Use the Script: simply save it to a file named aws_resource_
list.sh
, make the file executable with chmod +x aws_resource_
list.sh
, and then run it with the following command:
./aws_resource_list.sh <aws_region> <aws_service>
Replace <aws_region>
with the desired AWS region (e.g., us-east-1
) and <aws_service>
with the desired AWS service (e.g., ec2
).
Conclusion
In this blog post, we've demonstrated how to automate AWS resource tracking using shell scripting. By using this script, you can easily list AWS resources across various services, ensuring efficient resource utilization, cost optimization, and security.
Subscribe to my newsletter
Read articles from Anas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
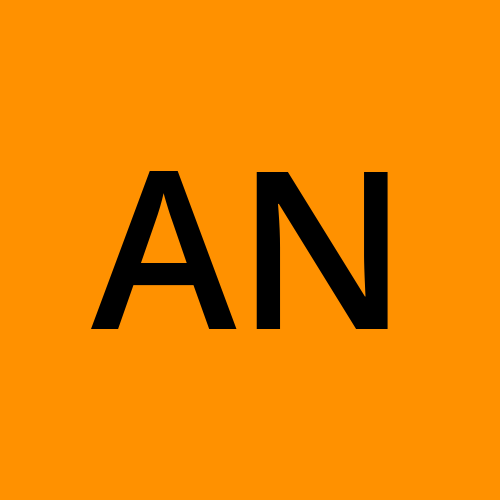