How to add comments to your website with Headless Comments
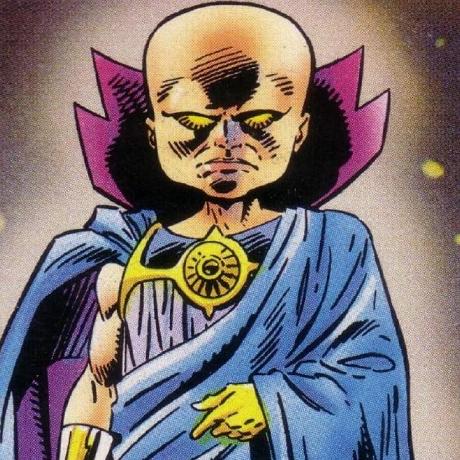
As more websites move toward headless and static site generators like Astro, developers and creators are gaining speed, security, and flexibility—but sometimes at the cost of familiar features, like comment systems that platforms like WordPress offer.
That's where Headless Comments comes in. A fully API-based commenting engine for any website or framework. In this guide, we'll walk you through how to integrate Headless Comments with Astro.
Prerequisites
For this example, we're using Astro's blog template, which can be found here: https://astro.build/themes/details/blog/.
Additionally, we'll need to install @astro/vue
and @headlesscomments/sdk
, an SDK for interacting with Headless Comments API.
Setting up Headless Comments
Creating a new website
To start this guide, we'll head to the Headless Comments' dashboard and add a new site. We'll fill in the name and URL, as well as ticking "Allow Comments". Once the form is filled in, click "create".
Adding a page
In Headless Comments, a page refers to a page, or article on your website; such as /blog/hello-world. We'll create one for a blog post in the Astro blog starter, that being /blog/hello-world/.
Navigate to the Pages table from the top navigation menu, and click the "Add Page" dropdown, then "create page" option. You'll be presented with a form. We'll fill it in with the page's title and path. The path will need to be exactly how it's shown on your website.
With that created, it's time to move onto the Astro integration!
Getting Started with Astro
Initialising the Client
To start our astro integration, we create a new folder under src
called Comments and a file within it called index
. If you're using typescript or javascript, this will end in their respective file endings.
Inside index.ts
, we'll import the Headless Comments SDK, and initialize it.
import { HeadlessComments } from "@headlesscomments/sdk"
const api = new HeadlessComments("YOUR_API_KEY", "YOUR_SITE_ID")
To initialize the client, we'll need an API key and the Site ID. The Site ID can be obtained from the Dashboard by copying the ID after /sites/
. For our API Key, click on user icon in the top right and head to Api Tokens. In here, create a new token with the following permissions:
page:read
page:create
comment:read
comment:create
Viewing Comments
Next, in /components, create a new Vue component called Comments.vue
In this file, we'll do two things; fetch existing comments on a page, and allow more comments to be submitted
For brevity, we've included a link to the Vue component from the public repo.
// Fetching comments
async function fetchComments() {
isLoaded.value = false;
// Here, we fetch the page from Headless Comments to
// check if comments are allowed.
page.value = await api.pages.get(props.path);
// If successful, and the page allows comments, fetch them
if (page.value && page.value.allow_comments) {
comments.value = (await api.comments.list(page.value.id)).data;
}
isLoaded.value = true;
}
// Posting a comment
async function submit() {
isSending.value = true;
// comment.value is { author_name, author_email, content }
const response = await api.comments.create(props.path, comment.value);
if (response.message === 'Comment ingested successfully.') {
alert('Comment submitted successfully!');
} else {
alert('Failed to submit comment. Please try again.');
}
// Clear the form
comment.value = {
author_name: "",
author_email: "",
content: ""
};
await fetchComments();
isSending.value = false;
}
onMounted(async () => {
await fetchComments();
});
Finally, in /layouts/BlogLayout
, we'll add the Comments component and pass in the current path.
layouts/BlogLayout.astro
---
import Comments from "../components/comments/Comments.vue";
const path = Astro.url.pathname;
---
<!-- Comments -->
<div class="prose">
<Comments path={path} client:load />
</div>
Testing the integration
To test our integration, submit a few comments from the /blog/hello-world post and check the /comments page in the Headless Comments dashboard.
Voila! You've just added comments to a website built with Astro 🚀
Subscribe to my newsletter
Read articles from Max Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
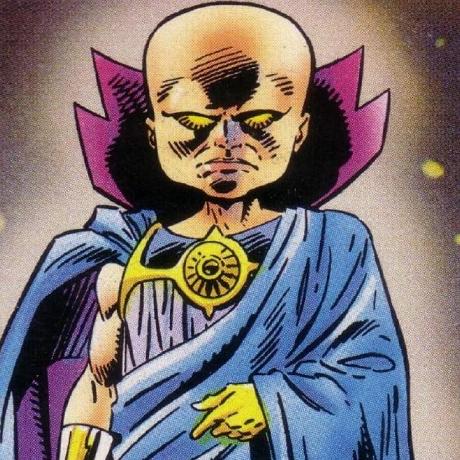