Understanding Buffer Overflow Attacks: A Deep Dive
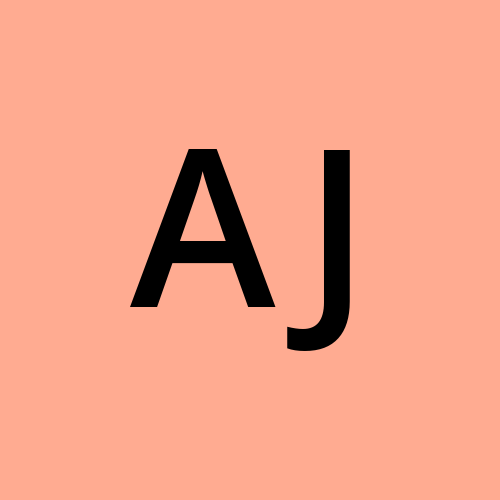
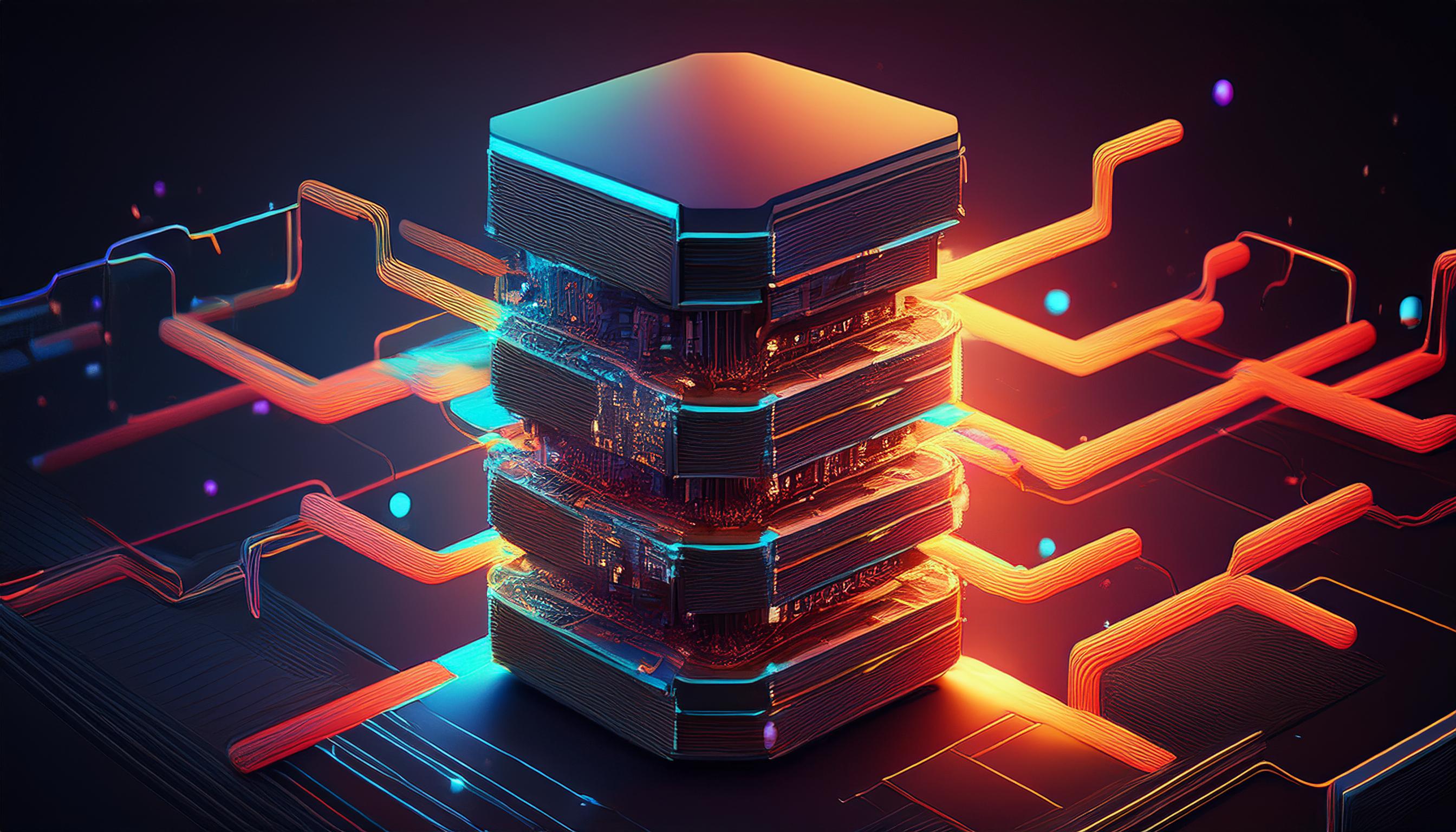
Imagine you're filling a glass with water. Normally, you'd stop pouring when the glass is full. But what if you kept pouring? The water would spill over the edges, creating a mess. In the world of cybersecurity, a similar concept exists—it's called a buffer overflow attack.
What is a Buffer Overflow?
A buffer overflow occurs when more data is written to a block of memory, or buffer, than it can hold. In simpler terms, it's like trying to stuff too many clothes into a suitcase. When the buffer overflows, the excess data spills over into adjacent memory spaces, which can lead to unpredictable behavior and potential security vulnerabilities.
How Does a Buffer Overflow Happen?
To understand how a buffer overflow happens, let’s look at a simple example in C, a programming language often used in system programming:
#include <stdio.h>
#include <string.h>
void vulnerableFunction(char *input) {
char buffer[10];
strcpy(buffer, input);
printf("Buffer: %s\n", buffer);
}
int main() {
char largeInput[20] = "This Is Too Much Data!";
vulnerableFunction(largeInput);
return 0;
}
In this code snippet, the buffer
array can hold 10 characters. However, largeInput
contains 18 characters. When strcpy
copies largeInput
into buffer
, it doesn’t check if buffer
has enough space. The excess characters spill over into adjacent memory, potentially overwriting important data.
This is where the danger lies. If an attacker can control the input, they might be able to overwrite critical parts of the program’s memory, like the return address of a function. By doing so, they could hijack the program’s execution flow, leading to the execution of malicious code.
Real-World Example: The Morris Worm
One of the most famous early examples of a buffer overflow attack was the Morris Worm in 1988. This worm exploited a buffer overflow vulnerability in the finger
service on Unix systems, allowing it to spread rapidly across the internet. It caused significant disruption and highlighted the dangers of buffer overflow vulnerabilities.
How to Prevent Buffer Overflow Attacks
Understanding the risks is the first step in preventing buffer overflow attacks. Here are some strategies to mitigate the threat:
Use Safe Functions: Avoid functions that don’t check buffer boundaries, like
strcpy
andgets
. Instead, use safer alternatives likestrncpy
orfgets
, which allow you to specify the maximum number of characters to copy.Input Validation: Always validate input lengths before processing. Ensure that the input data fits within the allocated buffer size.
Stack Canaries: Modern compilers often include stack canaries—special values placed on the stack that, if overwritten, indicate a buffer overflow. The program can then terminate safely before any damage occurs.
Address Space Layout Randomization (ASLR): ASLR randomizes the memory addresses used by system and application processes, making it more difficult for attackers to predict the location of buffers and inject malicious code.
Code Reviews and Testing: Regularly review your code for potential vulnerabilities. Use tools like static analyzers to detect buffer overflows. Additionally, perform fuzz testing to expose potential buffer overflow vulnerabilities by inputting large, random data sets.
Conclusion: Staying Safe in a Vulnerable World
Buffer overflow attacks are a serious threat, but with careful programming practices and modern security techniques, they can be mitigated. By understanding how buffer overflows occur and taking steps to prevent them, you can protect your applications and systems from this classic yet powerful type of attack.
Always remember, in cybersecurity, vigilance and proactive defense are key. Keep your code clean, your buffers in check, and stay ahead of potential threats.
Happy Coding :)
Subscribe to my newsletter
Read articles from Akash Reddy Jammula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
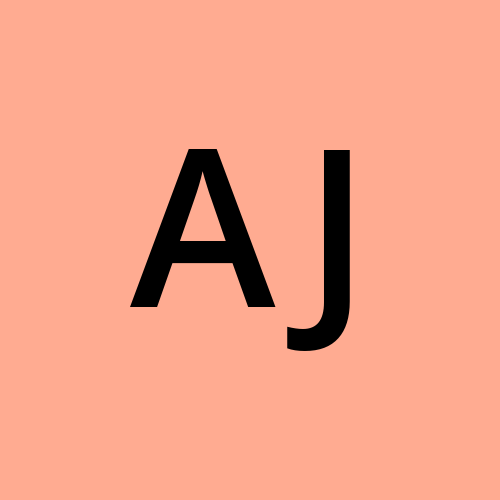
Akash Reddy Jammula
Akash Reddy Jammula
Hello 👋🏻 , I am a curiosity-driven software engineer who likes to write. I have a passion for discovering and working with cutting-edge technology.