Guide For Managing Global States in React

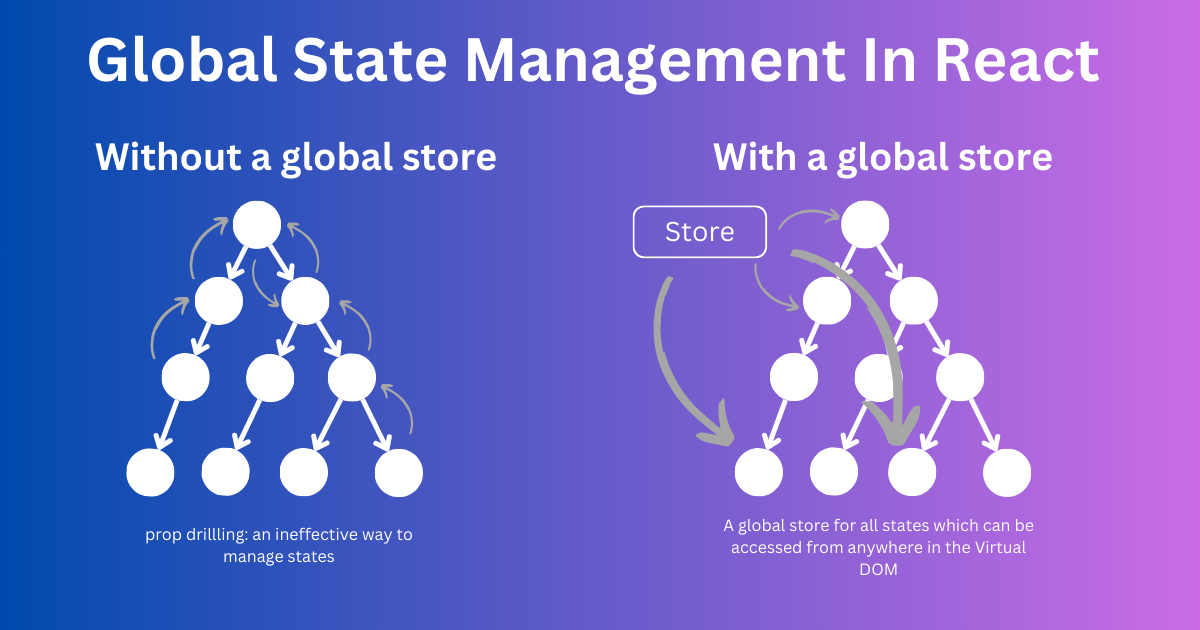
Introduction
Hi Guys! Welcome back to my blog with a guide for managing global states in react.
While building your react app you’ll find many problems when you are not using the global states in your web app. As the applications grow in complexity, managing state across different components become increasingly challenging, “prop drilling” might be manageable in small application but becomes challenging as the complexity rises.
In small applications, passing the states from one component to another as props may work but later you realize after the app grows in complexity and the codebase has become that it has become a mess and now it has become an absolute necessity to have some global states for the better management of the application.
Global state management is a technique that allows developers to keep the state synchronized across various components without having to pass props through multiple layers of the component tree. It simplifies data flow, making the application easier to maintain and scale. Effective global state management not only ensures consistency but also reduces bugs by centralizing the state in a predictable and maintainable way.
There are many powerfull libraries present like Redux, MobX, Zustand, etc. and also React provides it’s own way to managing global states in react (use Context). They provide a robust and elegant way to manage the states in the whole application seamlessly.
Brief Introduction to useContext
React’s useContext hook provides a straightforward way to share state between components without the need to pass props manually at each level of the component tree. By creating a context, you can make specific data available globally within your app, allowing any component to access it directly, no matter how deeply nested.
useContext is particularly useful for smaller applications where you only need to manage a few pieces of shared state. It works well for handling simple cases like theme toggling or user authentication, where the state isn't too complex.
However, as your app grows, the limitations of useContext become evident. For larger applications, more powerful tools like Redux offer a better approach for managing global states efficiently.
To know more about use Context , refer to React Official Documentation
Redux : The Go-to Solution for Global State Management
Redux has gain a lot of popularity and is used widely in the world for Managing Global States in React.
Redux offers a structured and robust approach to managing global states in more intricate apps. It’s a predictable state container that centralizes the application’s state into a single store, making it easier to manage and debug.
One of the core principles of Redux is that the state is immutable, meaning it cannot be modified directly. Instead, actions are dispatched, and reducers determine how the state should change in response. This structured approach brings clarity and predictability to state changes, ensuring that you always know how your state is evolving.
Key Concepts of Redux
There are some key concepts in redux that is very important to know to efficiently manage global states in react.
- Store: A Single source of Truth
This is where all your states of the application resides. Any component in the tree can have access to these states without needing to pass any props into the component. This centralized approach solves the problem of state management across multiple components, making the application more predictable and easier to debug.Any component can subscribe to the updates which will happen in the store ensuring when the state changes the component will re-render to reflect those changes.
- Actions: The Only Way to Update State
Actions are basically plain javascript object containing properties telling the reducers what to update.
const addItemAction = {
type: 'ADD_ITEM',
payload: { id: 1, name: 'New Item' }
};
It mainly contains a type
property which defines what event has occurred. In the above example there is an addItemAction which has type of ADD_ITEM
and the action may also contain payload which can be used to update the global store.
- Reducers: Pure Functions to Update State
Reducers are pure functions that take two arguments: the current state and an action. Based on the type of action, reducers return a new state object. Since reducers are pure, they don't mutate the original state but return a new version of the state instead. This immutability is a core principle in Redux, ensuring that you can track and manage the state predictably.
function itemReducer(state = [], action) {
switch (action.type) {
case 'ADD_ITEM':
return [...state, action.payload];
default:
return state;
}
}
As in the above example, for a action with type of ‘ADD_ITEM’ this will return a new object with the payload added with the previous items.
- Dispatching Actions: Triggering State Changes
In Redux, state changes can only occur through the dispatching of actions. Dispatching is the process of sending the action to the redux store for the reducer to process it and update the states in the store.
This is where the user can dispatch the actions by clicking a button or by submitting a form.
dispatch({ type: 'ADD_ITEM', payload: { id: 1, name: 'New Item' } });
Why Choose Redux for Large Applications?
Redux offers numerous advantages, particularly for larger applications to manage global state when it becomes increasingly complex. Here are some key benefits of using Redux:
- Predictable State Management
One of the biggest advantages of Redux is its predictable state behavior. Since state changes can only happen through actions and reducers, you always know how the state is updated. This strict unidirectional data flow ensures that state transitions are clear and predictable, making it easier to track changes over time. Each piece of the state is updated in a controlled, reliable manner, reducing the risk of bugs caused by unexpected changes.
- Centralized Control with a Single Store
With Redux, the entire application’s state is stored in a single, centralized place—the Redux store. This means that any component in the app can access the state directly, without needing to pass props down multiple levels of components. This centralization improves maintainability, as you only need to manage one state tree, even as the app grows. It also makes debugging simpler since the entire state is easily accessible and observable.
- Time-Travel Debugging and DevTools Integration
Redux offers powerful development tools, including time-travel debugging, which allows you to track and revert state changes. With Redux DevTools, you can inspect every dispatched action, view the state before and after the action, and even "time-travel" to previous states. This capability is incredibly useful when debugging, as you can see the exact series of actions that led to a specific bug or issue. It gives you full visibility into how your application’s state is evolving over time.
- Middleware for Handling Asynchronous Logic
Redux supports middleware, which allows you to extend its functionality beyond simple synchronous actions. Middleware like redux-thunk or redux-saga enables you to handle asynchronous operations such as API calls or logging. This means you can cleanly manage asynchronous logic in your app, such as fetching data from a server, while keeping the core state management logic focused and concise. Middleware acts as a powerful extension point to integrate third-party libraries or handle complex tasks that go beyond synchronous state updates.
- Separation of Concerns
Redux enforces a clear separation of concerns by keeping the view layer (React components) separate from the state logic (Redux). Components don't need to manage the state directly; instead, they dispatch actions and receive the state as props. This separation keeps the UI code cleaner and more focused on rendering, while Redux handles the heavy lifting of state management in the background. It makes it easier to test individual components and state logic separately.
- Consistent Data Flow Across Components
In a large application, managing data consistency between components can be challenging. Redux ensures that all components share the same source of truth. Since every component reads from the centralized store, you can rest assured that no part of your app is out of sync. This is especially useful when multiple components rely on the same data, such as user authentication or application settings.
- Scalability for Large Applications
Redux shines when working with large-scale applications where managing global states will become complex. Its architecture is designed to handle substantial apps with intricate state management needs. With the clear structure of actions, reducers, and the store, adding new features, updating existing ones, or integrating third-party libraries becomes easier to manage and scale.
By using Redux, you can manage even the most complex state in a scalable, maintainable way, ensuring that your React application remains organized as it grows.
Redux
Vs useContext
In this guide we’ve discussed about Redux and useContext in brief. Both are great to manage global states but both have different use cases which depends on the size and complexity of the app. Here are some key points to consider which could help you to choose one for your app:
When to use useContext
The useContext hook is built directly into React, making it a simple and lightweight solution for passing state between components without needing to drill props. It works well when:
Simple Global State: If your application only needs to manage a small number of global values, such as theme settings, user authentication status, or other basic data that doesn't change frequently.
Minimal State Logic: useContext is great when you don't need complex state updates or side effects. It excels when state is relatively static or doesn't require advanced manipulation.
Small or Medium-Sized Apps: In smaller applications or projects with less frequent state changes, useContext provides a more straightforward solution, as it’s part of React's core features without the need for additional libraries.
Example use cases for useContext:
Managing a dark mode toggle.
Handling basic user authentication and roles.
Sharing configuration settings like language preferences.
However, as your app grows in complexity, you might find that useContext alone can become difficult to manage, especially with large, dynamic data flows.
When To Use Redux
Redux is best suited for larger, more complex applications where state management requires more structure and control. It provides a centralized store for all the states in your app, making it easier to manage state that affects many components. Use Redux when:
Complex State Logic: If your application deals with numerous pieces of global state that are frequently updated, or if the state relies on complex logic, Redux’s strict structure will make it easier to manage.
Asynchronous Operations: Redux excels at handling asynchronous logic like fetching data from an API. Middleware such as redux-thunk or redux-saga makes handling side effects straightforward.
Debugging and State History: Redux provides powerful debugging tools, such as time-travel debugging with Redux DevTools, allowing you to inspect every state change and replay actions. This is useful for identifying bugs or performance issues.
Large Applications: As your app grows in size, with many components relying on shared state, Redux offers a more scalable solution. It helps maintain consistency and ensures that updates are predictable across your entire application.
Example use cases for Redux:
Handling complex data like e-commerce cart items, where multiple components rely on shared state.
Managing a large form across different pages with validations.
Applications with complex data fetching and caching strategies.
When to Use Both
Rarely in some situations, you can use both Redux and useContext together. For example, you might use useContext for lightweight, static global state (like a theme or language context) and Redux for managing more dynamic, frequently changing state (like user data or API responses). This approach balances simplicity and flexibility while still providing the power of Redux when needed.
Conclusion
Effective global state management is crucial for building scalable and maintainable React applications. As your app grows in complexity, managing state across multiple components can become challenging. Choosing the right solution for global state management ensures your app remains predictable and easy to debug.
For simpler use cases, React’s useContext provides a straightforward way to share state globally without adding extra complexity. However, when your application scales, and state management becomes more demanding, Redux offers a powerful and structured solution. It centralizes the state, provides a predictable flow for state updates, and offers advanced tools for managing asynchronous actions and debugging.
Ultimately, understanding your app's needs and complexity will help you decide which approach is best suited for managing global states. Whether you go with useContext for simplicity or Redux for robust state handling, mastering global state management is key to creating efficient, scalable applications in React.
Subscribe to my newsletter
Read articles from SURVEER directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
