Why Do Developers Prefer Call? A Guide to Ethereum's Send, Transfer, and Call Functions
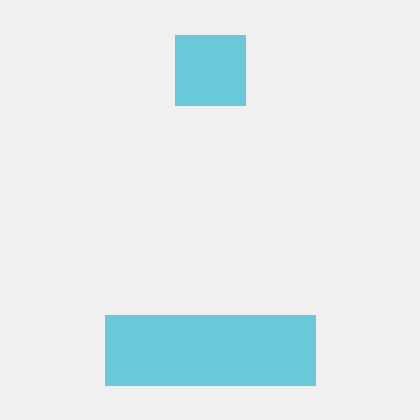
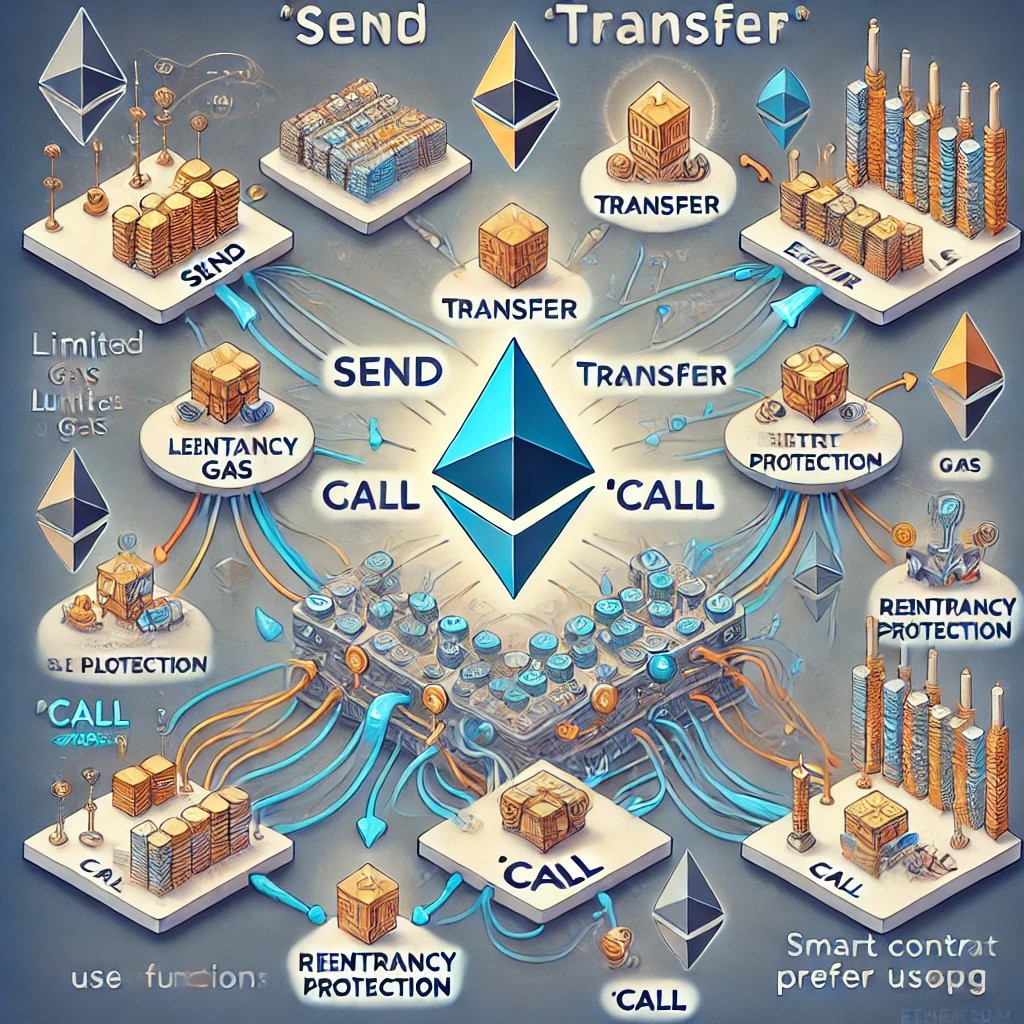
So, you're diving into the world of Ethereum smart contracts, huh? You’ve probably come across these three methods: send
, transfer
, and call
. They’re the main ways to move Ether around, but here’s the gist—they’re not all on the same level. In this article, we’re breaking it down, explaining why call
is the real MVP in the world of smart contract development.
The Basics: Meet the Squad
Before we jump into why call
is the main guy, let’s introduce the crew. In Ethereum, smart contracts need to send Ether to other contracts or regular accounts like yours. They can do this using:
send
: The chill, no-stress option—gets things done without too much hassle.transfer
: The cautious and careful one—ensures everything is in order before sending.call
: The versatile overachiever—handles all your Ether needs with extra sauce.
The Breakdown: Send vs. Transfer vs. Call
1. Send
: The Easygoing Option
First up, we have send
. Think of send
like that friend who’s always cool and laid-back just wants to get things done without too much stress.
Pros:
Simple and Fast:
Send
is quick, using just 2300 gas units—enough for basic tasks.No Drama: If something goes wrong,
send
just returnsfalse
and keeps it moving. No big deal.
Cons:
Limited Gas: 2300 gas units? That’s like having enough airtime to make a short call, but not enough to chat for long.
Manual Fixes: If
send
fails, it’s on you to sort it out. If you miss it, well, that’s your headache.
When to Use:
- For simple, no-fuss transactions where the other side just needs to receive the Ether and move on.
Example Code:
pragma solidity ^0.8.0;
contract ExampleSend {
function sendEther(address payable recipient) public payable {
// Sending Ether with the send function
bool success = recipient.send(msg.value);
// Handle failure case
if (!success) {
// Optionally revert the transaction or handle the failure
revert("Send failed");
}
}
}
Bottom Line: Send
is like your basic Nokia phone—reliable for the essentials, but when it’s time to do more, you know you need an upgrade.
2. Transfer
: The Careful One
Next, we have transfer
. Transfer
is like that friend who’s always double-checking everything—doesn’t leave anything to chance.
Pros:
Automatic Reversion: If something doesn’t go as planned,
transfer
will stop everything and revert the transaction. No messy aftermath.Trustworthy: You can always count on
transfer
to handle the situation properly.
Cons:
Gas Limit: Like
send
,transfer
also works with just 2300 gas units—so don’t expect it to handle anything complex.Not Flexible: If you need to tweak gas or do something special,
transfer
isn’t the one for that.
When to Use:
- When you need a safe and straightforward Ether transfer, and you don’t want to worry about things going wrong.
Example Code:
pragma solidity ^0.8.0;
contract ExampleTransfer {
function transferEther(address payable recipient) public payable {
// Sending Ether with the transfer function
recipient.transfer(msg.value);
// No need to handle failure explicitly as transfer reverts automatically on failure
}
}
Bottom Line: Transfer
is reliable and gets the job done, but if you need more flexibility, you might want to explore other options.
3. Call
: The All-Star
Now let’s talk about call
, the real star of the show. Call
is like that friend who can do everything—need more gas? Got it. Want to send data along with Ether? No problem. Call
is your go-to for handling all the complex stuff.
Pros:
Unlimited Gas:
Call
isn’t stuck with the 2300 gas limit—give it all the gas it needs to get the job done.Versatile:
Call
can send Ether, data, and even call functions on other contracts. It’s the all-in-one solution.Custom Error Handling: If something goes wrong,
call
doesn’t panic. It lets you handle the situation however you want.
Cons:
Responsibility: With great power comes great responsibility. You’ve got to handle failures yourself, and if you’re not careful, things can get messy.
Complexity:
Call
isn’t as straightforward as the others, so you’ll need to put in a bit more work.
When to Use:
- Anytime you need more control over your transactions or when dealing with complex interactions.
Example Code:
pragma solidity ^0.8.0;
contract ExampleCall {
function callEther(address payable recipient) public payable {
// Sending Ether with the call function
(bool success, ) = recipient.call{value: msg.value, gas: 5000}("");
// Handle failure case
if (!success) {
// Optionally revert the transaction or handle the failure
revert("Call failed");
}
}
function callWithFunction(address payable recipient, bytes memory data) public payable {
// Sending Ether and calling a function on the recipient contract
(bool success, ) = recipient.call{value: msg.value}(data);
// Handle failure case
if (!success) {
// Optionally revert the transaction or handle the failure
revert("Call with function failed");
}
}
}
Bottom Line: Call
is the go-to choice for anyone looking to do more than just basic transactions. It’s powerful and flexible, but make sure you’re ready to handle the extra responsibility.
Why Call
is the Real MVP
So why does call
get all the hype? Here’s the lowdown:
Flexibility:
Call
lets you adjust gas, send data, and handle complex interactions without any hassle.Control: You’re in charge—handle errors and failures your way, making it safer in the long run.
Future-Proofing: As smart contracts evolve and get more complicated,
call
is ready to adapt and handle whatever comes next.
Final Thoughts
Understanding the differences between send
, transfer
, and call
is like knowing when to grab Jollof, when to go for Amala, and when to settle with Pounded Yam. Each has its own vibe, but in the world of smart contracts, call
is the one that gives you the most control and flexibility. So next time you’re building your contract, think about what you need—quick and easy, safe and reliable, or powerful and versatile—and choose your method accordingly.
Subscribe to my newsletter
Read articles from Samuel Aleonomoh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
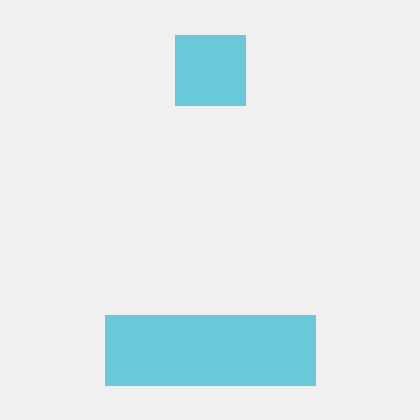